(C#) ASP.NET ListBox & DataBinding |
(C#) ASP.NET ListBox & DataBinding เป็นการใช้ ListBox อ่านข้อมูลจาก Database การกำหนดเงื่อนไขแบบ SelectionMode="Multiple" ตัวอย่างนี้ผมได้ยกตัวอย่างการใช้บน DataTable,TableRows,SortedList การเพิ่ม/ลบ Item และการใช้งานอื่น ๆ
Language Code : VB.NET || C#
Framework : 1,2,3,4
AspNetListBoxDataBind.aspx
<%@ Import Namespace="System.Data"%>
<%@ Import Namespace="System.Data.OleDb"%>
<%@ Page Language="C#" Debug="true" %>
<script runat="server">
void Page_Load(object sender, EventArgs e)
{
if(!Page.IsPostBack)
{
ListBoxDataTable();
ListBoxDataTableRows();
ListBoxSortedList();
ListBoxAddInsertItem();
}
}
//*** ListBox & DataTable ***//
void ListBoxDataTable()
{
OleDbConnection objConn;
OleDbDataAdapter dtAdapter;
DataTable dt = new DataTable();
String strConnString;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source="+
Server.MapPath("database/mydatabase.mdb")+"";
objConn = new OleDbConnection(strConnString);
objConn.Open();
String strSQL;
strSQL = "SELECT * FROM country";
dtAdapter = new OleDbDataAdapter(strSQL, objConn);
dtAdapter.Fill(dt);
dtAdapter = null;
objConn.Close();
objConn = null;
//*** ListBox ***//
this.myListBox1.DataSource = dt;
this.myListBox1.DataTextField = "CountryName";
this.myListBox1.DataValueField = "CountryCode";
this.myListBox1.DataBind();
//*** Default Value ***//
myListBox1.SelectedIndex = myListBox1.Items.IndexOf(myListBox1.Items.FindByValue("TH")); //*** By DataValueField ***//
//myListBox1.SelectedIndex = myListBox1.Items.IndexOf(myListBox1.Items.FindByText("Thailand")); //*** By DataTextField ***//
}
//*** ListBox & TableRows ***//
void ListBoxDataTableRows()
{
DataTable dt = new DataTable();
DataRow dr;
//*** Column ***//
dt.Columns.Add("Sex");
dt.Columns.Add("SexDesc");
//*** Rows ***//
dr = dt.NewRow();
dr["Sex"] = "M";
dr["SexDesc"] = "Man";
dt.Rows.Add(dr);
//*** Rows ***//
dr = dt.NewRow();
dr["Sex"] = "W";
dr["SexDesc"] = "Woman";
dt.Rows.Add(dr);
//*** ListBox ***//
this.myListBox2.DataSource = dt;
this.myListBox2.DataTextField = "SexDesc";
this.myListBox2.DataValueField = "Sex";
this.myListBox2.DataBind();
//*** Default Value ***//
myListBox2.SelectedIndex = myListBox2.Items.IndexOf(myListBox2.Items.FindByValue("W")); //*** By DataValueField ***//
//myListBox2.SelectedIndex = myListBox2.Items.IndexOf(myListBox2.Items.FindByText("Woman")); //*** By DataTextField ***//
}
//*** ListBox & SortedList ***//
void ListBoxSortedList()
{
SortedList mySortedList = new SortedList();
mySortedList.Add("M","Man");
mySortedList.Add("W","Woman");
//*** ListBox ***//
this.myListBox3.DataSource = mySortedList;
this.myListBox3.DataTextField = "Value";
this.myListBox3.DataValueField = "Key";
this.myListBox3.DataBind();
//*** Default Value ***//
myListBox3.SelectedIndex = myListBox3.Items.IndexOf(myListBox3.Items.FindByValue("W")); //*** By DataValueField ***//
//myListBox3.SelectedIndex = myListBox3.Items.IndexOf(myListBox3.Items.FindByText("Woman")); //*** By DataTextField ***//
}
//*** Add/Insert Items ***//
void ListBoxAddInsertItem()
{
SortedList mySortedList = new SortedList();
mySortedList.Add("M","Man");
mySortedList.Add("W","Woman");
//*** ListBox ***//
this.myListBox4.DataSource = mySortedList;
this.myListBox4.DataTextField = "Value";
this.myListBox4.DataValueField = "Key";
this.myListBox4.DataBind();
//*** Add & Insert New Item ***//
String strText,strValue;
//*** Insert Item ***//
strText = "";
strValue = "";
ListItem InsertItem = new ListItem(strText, strValue);
myListBox4.Items.Insert(0, InsertItem);
//*** Add Items ***//
strText = "Guy";
strValue = "G";
ListItem AddItem = new ListItem(strText, strValue);
myListBox4.Items.Add(AddItem);
//*** Default Value ***//
myListBox4.SelectedIndex = myListBox4.Items.IndexOf(myListBox4.Items.FindByValue("")); //*** By DataValueField ***//
//myListBox4.SelectedIndex = myListBox4.Items.IndexOf(myListBox4.Items.FindByText("")); //*** By DataTextField ***//
}
void Button1_OnClick(object sender, EventArgs e)
{
this.lblText1.Text = this.myListBox1.SelectedItem.Value; //*** Or this.myListBox1.SelectedItem.Text ***'
this.lblText2.Text = this.myListBox2.SelectedItem.Value; //*** Or this.myListBox2.SelectedItem.Text ***//
//*** SelectionMode="Multiple" ***//
int i;
this.lblText3.Text = "";
for(i = 0 ; i <= this.myListBox3.Items.Count - 1; i ++)
{
if(this.myListBox3.Items[i].Selected)
{
this.lblText3.Text = this.lblText3.Text + "," + this.myListBox3.Items[i].Value; //*** Or this.myListBox3.Items[i].Text ***//
}
}
this.lblText4.Text = this.myListBox4.SelectedItem.Value; //*** Or this.myListBox4.SelectedItem.Text ***//
}
</script>
<html>
<head>
<title>ThaiCreate.Com ASP.NET - ListBox & DataBind</title>
</head>
<body>
<form id="form1" runat="server">
<asp:ListBox id="myListBox1" runat="server"></asp:ListBox>
<asp:ListBox id="myListBox2" runat="server"></asp:ListBox>
<asp:ListBox id="myListBox3" SelectionMode="Multiple" runat="server"></asp:ListBox>
<asp:ListBox id="myListBox4" runat="server"></asp:ListBox>
<asp:Button id="Button1" onclick="Button1_OnClick" runat="server" Text="Button"></asp:Button>
<hr />
<asp:Label id="lblText1" runat="server"></asp:Label><br />
<asp:Label id="lblText2" runat="server"></asp:Label><br />
<asp:Label id="lblText3" runat="server"></asp:Label><br />
<asp:Label id="lblText4" runat="server"></asp:Label><br />
</form>
</body>
</html>
Screenshot
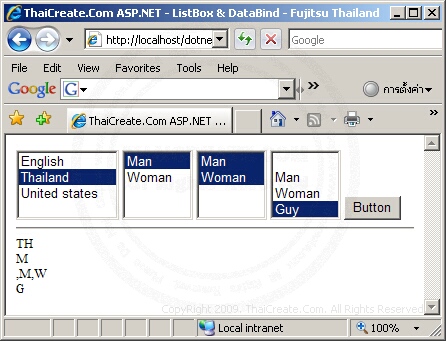
ASP.NET & AccessDataSource and Listbox
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2008-11-15 06:50:08 /
2017-03-28 20:46:30 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|