(C#) ASP.NET SQL Server & System.Data.Odbc |
(C#) ASP.NET SQL Server & System.Data.Odbc เป็นตัวอย่างการใช้งานฐานข้อมูล Microsoft SQL Server กับ Odbc ด้วย System.Data.Odbc ในการเพิ่ม ลบ แก้ไข ข้อมูลใน GridView
Language Code : VB.NET || C#
Connection String
Driver={SQL Server};Server=localhost;Database=database;UID=sa;PWD=;
AspNetOdbcSQLServer.aspx
<%@ Page Language="C#" Debug="true" %>
<%@ import Namespace="System.Data" %>
<%@ import Namespace="System.Data.Odbc" %>
<script runat="server">
OdbcConnection objConn;
OdbcCommand objCmd;
String strSQL;
void Page_Load(Object sender, EventArgs e)
{
String strConnString;
strConnString = "Driver={SQL Server};Server=localhost;Database=mydatabase;UID=sa;PWD=;";
objConn = new OdbcConnection(strConnString);
objConn.Open();
if(!Page.IsPostBack)
{
BindData();
}
}
void BindData()
{
strSQL = "SELECT * FROM customer";
OdbcDataReader dtReader;
objCmd = new OdbcCommand(strSQL, objConn);
dtReader = objCmd.ExecuteReader();
//*** BindData to GridView ***//
myGridView.DataSource = dtReader;
myGridView.DataBind();
dtReader.Close();
dtReader = null;
}
void Page_UnLoad()
{
objConn.Close();
objConn = null;
}
void modEditCommand(Object sender , GridViewEditEventArgs e)
{
myGridView.EditIndex = e.NewEditIndex;
myGridView.ShowFooter = false;
BindData();
}
void modCancelCommand(Object sender, GridViewCancelEditEventArgs e)
{
myGridView.EditIndex = -1;
myGridView.ShowFooter = true;
BindData();
}
void modDeleteCommand(Object sender, GridViewDeleteEventArgs e)
{
strSQL = "DELETE FROM customer WHERE CustomerID = '" + myGridView.DataKeys[e.RowIndex].Value + "'";
objCmd = new OdbcCommand(strSQL, objConn);
objCmd.ExecuteNonQuery();
myGridView.EditIndex = -1;
BindData();
}
void myGridView_RowCommand(Object source , GridViewCommandEventArgs e)
{
if (e.CommandName == "Add")
{
//*** CustomerID ***//
TextBox txtCustomerID = (TextBox)myGridView.FooterRow.FindControl("txtAddCustomerID");
//*** Email ***//
TextBox txtName = (TextBox)myGridView.FooterRow.FindControl("txtAddName");
//*** Name ***//
TextBox txtEmail = (TextBox)myGridView.FooterRow.FindControl("txtAddEmail");
//*** CountryCode ***//
TextBox txtCountryCode = (TextBox)myGridView.FooterRow.FindControl("txtAddCountryCode");
//*** Budget ***//
TextBox txtBudget = (TextBox)myGridView.FooterRow.FindControl("txtAddBudget");
//*** Used ***//
TextBox txtUsed = (TextBox)myGridView.FooterRow.FindControl("txtAddUsed");
strSQL = "INSERT INTO customer (CustomerID,Name,Email,CountryCode,Budget,Used) " +
" VALUES ('" + txtCustomerID.Text + "','" + txtName.Text + "','" + txtEmail.Text + "' " +
" ,'" + txtCountryCode.Text + "','" + txtBudget.Text + "','" + txtUsed.Text + "') ";
objCmd = new OdbcCommand(strSQL, objConn);
objCmd.ExecuteNonQuery();
BindData();
}
}
void modUpdateCommand(Object sender,GridViewUpdateEventArgs e)
{
//*** CustomerID ***//
TextBox txtCustomerID = (TextBox)myGridView.Rows[e.RowIndex].FindControl("txtEditCustomerID");
//*** Email ***//
TextBox txtName = (TextBox)myGridView.Rows[e.RowIndex].FindControl("txtEditName");
//*** Name ***//
TextBox txtEmail = (TextBox)myGridView.Rows[e.RowIndex].FindControl("txtEditEmail");
//*** CountryCode ***//
TextBox txtCountryCode = (TextBox)myGridView.Rows[e.RowIndex].FindControl("txtEditCountryCode");
//*** Budget ***//
TextBox txtBudget = (TextBox)myGridView.Rows[e.RowIndex].FindControl("txtEditBudget");
//*** Used ***//
TextBox txtUsed = (TextBox)myGridView.Rows[e.RowIndex].FindControl("txtEditUsed");
strSQL = "UPDATE customer SET CustomerID = '" + txtCustomerID.Text + "' " +
" ,Name = '" + txtName.Text + "' " +
" ,Email = '" + txtEmail.Text + "' " +
" ,CountryCode = '" + txtCountryCode.Text + "' " +
" ,Budget = '" + txtBudget.Text + "' " +
" ,Used = '" + txtUsed.Text + "' " +
" WHERE CustomerID = '" + myGridView.DataKeys[e.RowIndex].Value + "'";
objCmd = new OdbcCommand(strSQL, objConn);
objCmd.ExecuteNonQuery();
myGridView.EditIndex = -1;
myGridView.ShowFooter = true;
BindData();
}
</script>
<html>
<head>
<title>ThaiCreate.Com ASP.NET - System.Data.Odbc</title>
</head>
<body>
<form id="form1" runat="server">
<asp:GridView id="myGridView" runat="server" AutoGenerateColumns="False"
ShowFooter="True"
DataKeyNames="CustomerID"
OnRowEditing="modEditCommand"
OnRowCancelingEdit="modCancelCommand"
OnRowDeleting="modDeleteCommand"
OnRowUpdating="modUpdateCommand"
OnRowCommand="myGridView_RowCommand">
<Columns>
<asp:TemplateField HeaderText="CustomerID">
<ItemTemplate>
<asp:Label id="lblCustomerID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCustomerID" size="5" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCustomerID" size="5" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label id="lblName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditName" size="10" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddName" size="10" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Email">
<ItemTemplate>
<asp:Label id="lblEmail" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditEmail" size="20" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddEmail" size="20" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="CountryCode">
<ItemTemplate>
<asp:Label id="lblCountryCode" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCountryCode" size="2" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCountryCode" size="2" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Budget">
<ItemTemplate>
<asp:Label id="lblBudget" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBudget" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBudget" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Used">
<ItemTemplate>
<asp:Label id="lblUsed" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditUsed" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddUsed" size="6" runat="server"></asp:TextBox>
<asp:Button id="btnAdd" runat="server" Text="Add" CommandName="Add"></asp:Button>
</FooterTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="True" CancelText="Cancel" DeleteText="Delete" EditText="Edit" UpdateText="Update" HeaderText="Modify" />
<asp:CommandField ShowDeleteButton="True" HeaderText="Delete" />
</Columns>
</asp:GridView>
</form>
</body>
</html>
Screenshot
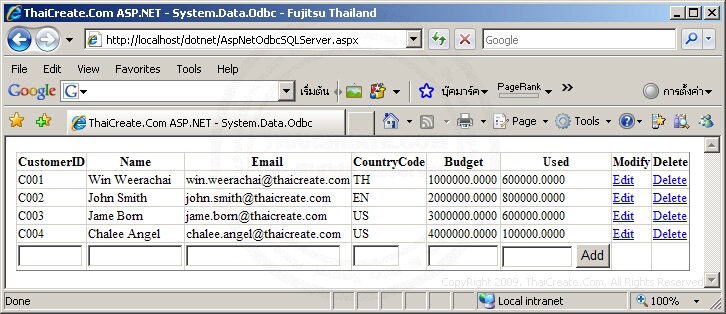
ASP.NET System.Data.Odbc - Connection String
ASP.NET Microsoft SQL Server - System.Data.SqlClient
ASP.NET System.Data.Odbc - Parameter Query
ในส่วนของดาวน์โหลดผมได้เขียนไวสำหรับใช้งานบน Visual Studio 2005 (Framework 2.0) ไว้ด้วยครับ สามารถดาวน์โหลดได้จากข้างล่าง
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2008-12-01 10:53:54 /
2017-03-29 11:03:40 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|