|
เริ่มต้นสร้าง PHP Lib กันดีกว่า 2 |
เกริ่นนำ
เขียนไฟล์มั่นยุ่งยากพอสมควร นะครับยิ่งถ้าต้องการให้มันเขียน หลายจุดหรือหลายครั้ง ก็คงต้องนั่งกุมขมับกันหรือไม่ก็เสยผมไม่รู้กี่รอบ เอาเป็นว่า หัวแทบล้านเลยก็ว่าได้ ดังนั้นจึงเกิดคลาส File ตัวนี้ขึ้นมาให้เลือกหยิบใช้ได้งานได้ง่ายมากขึ้น
Class File
File.php
<?php
/**
* Class File
* PHP5+
*
*/
define ( "FILE_EMPTY", (- 1), true );
define ( "FILE_OPENED", 1, true );
define ( "FILE_CLOSED", 0, true );
class File {
public $s_file_name;
public $r_file_handle;
public $i_line;
public $i_state;
public function __construct($s_fileName = '') {
$this->s_file_name = ( string ) $s_fileName;
$this->r_file_handle = null;
$this->i_line = FILE_EMPTY;
$this->i_state = FILE_CLOSED;
}
public function openFile($mod = 'r') {
switch ($mod) {
case 'r' :
$s_mode = 'r';
break;
case 'rb' :
$s_mode = 'rb';
break;
case 'w' :
$s_mode = 'w';
break;
case 'a' :
$s_mode = 'a';
break;
case 'r+' :
$s_mode = 'r+';
break;
case 'w+' :
$s_mode = 'w+';
break;
case 'a+' :
$s_mode = 'a+';
break;
default :
$s_mode = 'r';
break;
}
if ($this->i_state === FILE_CLOSED) {
$fopen = @fopen ( $this->s_file_name, $s_mode );
if ($fopen !== false) {
$this->i_state = FILE_OPENED;
$this->r_file_handle = $fopen;
} else {
print "ERROR : " . $this->s_file_name . " file can't found./ fle can't open. !!!";
$this->i_state = FILE_CLOSED;
exit ();
}
}
}
/**
* return date/time of last modify
*
* @return string
*/
public function lastModifyDate() {
return filemtime ( $this->s_file_name );
}
/**
* Write data to blank file at 1024 bytes per time.
*
* @param String $s_data
* @param Integer $i_byte
*/
public function writeTxt($s_data, $i_byte = 1024) {
if ($this->i_state == FILE_OPENED) {
fwrite ( $this->r_file_handle, $s_data, $i_byte );
} else {
print "ERROR : " . $this->s_file_name . " file can't found./ fle can't open. !!!";
exit ();
}
}
/**
* return size (byte) of file.
*
* @return integer
*/
public function sizeFile() {
return filesize ( $this->s_file_name );
}
/**
* return last access date/time
*
* @return string
*/
public function lastAccress() {
return fileatime ( $this->s_file_name );
}
/**
* return type of file (file or dir)
*
* @return string
*/
public function fileType() {
return filetype ( $this->s_file_name );
}
/**
* return data from file
*
* @param integer $i_byte
* @return string
*/
public function readTxt($i_byte = 1024) {
$s_data = '';
if ($this->i_state === FILE_OPENED) {
while ( ! feof ( $this->r_file_handle ) ) {
$s_data .= fgets ( $this->r_file_handle, $i_byte );
}
fseek ( $this->r_file_handle, 0 );
} else {
print "ERROR : " . $this->s_file_name . " file can't found./ fle can't open. !!!";
exit ();
}
return $s_data;
}
/**
* return number of line.
*
*/
public function countLine() {
$i_line = 0;
if ($this->i_state === FILE_OPENED) {
while ( ! feof ( $this->r_file_handle ) ) {
$i_line = $i_line + 1;
fgets ( $this->r_file_handle, 1024 );
}
fseek ( $this->r_file_handle, 0 );
} else {
print "ERROR : " . $this->s_file_name . " file can't found./ fle can't open. !!!";
exit ();
}
return $i_line;
}
/**
* return data by in line number.
*
* @param integer $line
* @param integer $i_byte
* @return string
*/
public function readLine($line = 1, $i_byte = 1024) {
$i_count_line = self::countLine ();
$s_at_Line = '';
if ($this->i_state === FILE_OPENED) {
if ($i_count_line >= $line) {
for($i = 1; $i < $i_count_line; $i ++) {
$s_at_Line = fgets ( $this->r_file_handle, $i_byte );
if ($i == $line) {
break;
}
}
fseek ( $this->r_file_handle, 0 );
}
} else {
print "ERROR : " . $this->s_file_name . " file can't found./ fle can't open. !!!";
exit ();
}
return $s_at_Line;
}
public function getStartCFGBlock($s_block) {
$i = 1;
$pattern = '/(^\[' . $s_block . '\])/i';
while ( ! feof ( $this->r_file_handle ) ) {
$s_data = fgets ( $this->r_file_handle, 1024 );
if (preg_match ( $pattern, $s_data )) {
print $i . " : " . $s_data;
}
$i ++;
}
fseek ( $this->r_file_handle, 0 );
return $s_data;
}
/**
* return data from file for browser.
*
* @return string
*/
public function readHtmlBreakLine($i_byte = 1024) {
$s_data = '';
if ($this->i_state === FILE_OPENED) {
while ( ! feof ( $this->r_file_handle ) ) {
$s_data .= fgets ( $this->r_file_handle, $i_byte );
$s_data .= '<br>';
}
fseek ( $this->r_file_handle, 0 );
} else {
print "ERROR : " . $this->s_file_name . " file can't found./ fle can't open. !!!";
exit ();
}
return $s_data;
}
/**
* rename file.
*/
public function renameFile($s_name_old_file, $s_name_new_file = '') {
if ($s_name_new_file != '' && $s_name_old_file != '') {
$b_ = rename ( $s_name_old_file, $s_name_new_file );
return $b_;
} else {
return false;
}
}
/**
* delete file.
*/
public function deleteFile($s_name_file = '') {
if ($s_name_file != '') {
$b_ = unlink ( $s_name_file );
return $b_;
} else {
return false;
}
}
/**
*
*/
public function fpassthru(){
return fpassthru($this->r_file_handle);
}
/**
* close file connection.
*
*/
public function closeFile() {
if (fclose ( $this->r_file_handle )) {
$this->r_file_handle = null;
$this->i_state = 0;
}
}
}
?>[/code]
ตัวอย่างการเขียนไฟล์
write_file_class.php
<?php
include_once "File.php"; // นำเข้าไฟล์ File.php
$o_log_file = new File();
$o_log_file->s_file_name = "my_file.txt";
$o_log_file->openFile('a+'); //เปิดไฟล์แบบเขียนต่อท้าย หาไฟล์ไม่เจอสร้างให้ใหม่
$o_log_file->writeTxt("111111111111111111");
$o_log_file->writeTxt("222222222222222222");
$o_log_file->writeTxt("333333333333333333");
$o_log_file->writeTxt("444444444444444444");
$o_log_file->writeTxt("555555555555555555");
$o_log_file->closeFile();
?>
######################################################
*** การอ่านไฟล์ จะต้องมีไฟล์อยู่จริง ตามตำแหน่งที่ระบุ นะครับไม่งั้น error บาน
ตัวอย่างการอ่านไฟล์ ทั้งไฟล์
read_file_class.php
[code]<?php
include_once "File.php"; // นำเข้าไฟล์ File.php
$o_log_file = new File();
$o_log_file->s_file_name = "my_file.txt";
$o_log_file->openFile(); //เปิดไฟล์แบบเขียนต่อท้าย หาไฟล์ไม่เจอสร้างให้ใหม่
print "อ่านทั้งไฟล์<br>";
print $o_log_file->readTxt(1024);// ส่งค่ากลับออกมา สามารถเก็บไว้ในตัวแปรได้ แต่อันนี้ให้แสดงเลย
print "<br>";
print "อ่านเป็นแถว แสดงข้อมูลออกมาเฉพาะแถวที่กำหนด<br>";
print $o_log_file->readLine(3,1024); // อ่านข้อมูลเป็นแถว โดยระบุแถวที่เราต้องการ ได้ ส่งค่ากลับมาเหมือนกัน
$o_log_file->closeFile();
?>
ผลลัพธ์
ขอแสดงผลแบบควบสองโปรแกรมเลยนะครับ ทั้งเขียน และอ่านไฟล์สองแบบเลย
คลิกเพื่อดู ผลลัพธ์ที่ได้
|
|
|
|
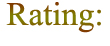 |
|
|
|
By : |
เอส
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2009-05-03 |
|
Download : |
No files |
|
|
|
|