|
(C#) จัดการ CheckBox ให้เลือก Checkbox เฉพาะตามการแสดงตารางที่ค้นหาข้อมูลในปัจจุบันครับ |
บทความเรื่อง (C#) จัดการ CheckBox ให้เลือก Checkbox เฉพาะตามการแสดงตารางที่ค้นหาข้อมูลในปัจจุบัน เป็นตัวอย่างการจัดการ CheckBox ให้เลือก Checkbox เฉพาะตามการแสดงตารางที่ค้นหาข้อมูลในปัจจุบัน ในรูปแบบ Code ภาษา C# ครับ
ซึ่งที่มาของการทำบทความนี้ ได้แรงบันดาลใจมาจากบทความ และ Source Code ดังนี้ครับ
หัวใจของ Code อยู่ที่ "jQuery :visible Selector"
$(window).load(function(){
$("#checkAll").change(function () {
$("input:checkbox:visible").prop('checked', $(this).prop("checked"));
});
});
Source Code
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="CheckLoopQR3.Default" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title></title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript" src="//code.jquery.com/jquery-1.6.4.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<style>
#myInput {
font-size: 16px;
padding: 6px 20px 6px 10px;
border: 1px solid #ddd;
margin-bottom: 3px;
}
</style>
<script type="text/javascript">
$(window).load(function(){
$("#checkAll").change(function () {
$("input:checkbox:visible").prop('checked', $(this).prop("checked"));
});
});
</script>
<script>
function myFunction() {
var input, filter, table, tr, td, i, txtValue;
input = document.getElementById("myInput");
filter = input.value.toUpperCase();
table = document.getElementById("CheckBox1");
tr = table.getElementsByTagName("tr");
for (i = 0; i < tr.length; i++) {
td = tr[i].getElementsByTagName("td")[0];
if (td) {
txtValue = td.textContent || td.innerText;
if (txtValue.toUpperCase().indexOf(filter) > -1) {
tr[i].style.display = "";
} else {
tr[i].style.display = "none";
}
}
}
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div class="container">
<h2>QR Code Generator</h2>
<div class="row">
<div class="col-md-4">
<div class="form-group">
<label>Please Input Data</label>
<div class="input-group">
<asp:TextBox ID="txtQRCode" runat="server" CssClass="form-control"></asp:TextBox>
<div class="input-group-prepend">
<asp:Button ID="btnGenerate" runat="server" CssClass="btn btn-secondary" Text="Generate" OnClick="btnGenerate_Click" />
</div>
</div>
</div>
</div>
</div>
<label>Input Number to Search </label>
<input type="text" id="myInput" onkeyup="myFunction()"><br />
<asp:Button ID="btnSelect" runat="server" CssClass="btn btn-secondary" Text="Display Text" OnClick="btnSelect_Click" /><br /><br />
<asp:PlaceHolder ID="PlaceHolder1" runat="server"></asp:PlaceHolder>
<asp:CheckBox ID="checkAll" runat="server" Font-Size="Large"/><asp:Label id="checkTextAll" runat="server" Font-Size="Large"></asp:Label><br /><br />
<asp:CheckBoxList ID="CheckBox1" runat="server" Border="1"
BorderColor="LightGray" Font-Size="Large"></asp:CheckBoxList>
</div>
</form>
</body>
</html>
Default.aspx.cs
using System;
using System.Drawing;
using System.IO;
using ZXing;
using ZXing.QrCode;
using System.Web.UI.WebControls;
using System.Web.UI.HtmlControls;
namespace CheckLoopQR3
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
this.checkTextAll.Text = " Check All";
}
protected void btnSelect_Click(object sender, EventArgs e)
{
string code = txtQRCode.Text;
long num = Convert.ToInt64(code);
int i;
for (i = 1; i < 6; i++)
{
num *= i;
CheckBox1.Items.Add(new ListItem(" " + num));
}
}
protected void btnGenerate_Click(object sender, EventArgs e)
{
if (CheckBox1.SelectedItem == null)
{
Response.Redirect("Default.aspx");
}
string[] texture = { "Selected Text 1 -> ", "Selected Text 2 -> ", "Selected Text 3 -> ",
"Selected Text 4 -> ", "Selected Text 5 -> "};
string[] texture2 = { " is Checkbox 1.", " is Checkbox 2.", " is Checkbox 3.",
" is Checkbox 4.", " is Checkbox 5."};
foreach (ListItem listItem in CheckBox1.Items)
{
if (listItem.Selected)
{
int a = CheckBox1.Items.IndexOf(listItem);
a = a + 1;
string code = listItem.Text;
CheckBox1.Visible = false;
checkAll.Visible = false;
checkTextAll.Visible = false;
QrCodeEncodingOptions options = new QrCodeEncodingOptions();
options = new QrCodeEncodingOptions
{
DisableECI = true,
CharacterSet = "UTF-8",
Width = 150,
Height = 150,
Margin = 0,
};
var barcodeWriter = new BarcodeWriter();
barcodeWriter.Format = BarcodeFormat.QR_CODE;
barcodeWriter.Options = options;
System.Web.UI.WebControls.Image imgBarCode = new System.Web.UI.WebControls.Image();
imgBarCode.Height = 150;
imgBarCode.Width = 150;
Label lblvalues = new Label();
lblvalues.Text += texture[a - 1] + listItem.Text + texture2[a - 1];
lblvalues.Font.Size = FontUnit.Large;
using (Bitmap bitMap = barcodeWriter.Write(code))
{
using (MemoryStream ms = new MemoryStream())
{
bitMap.Save(ms, System.Drawing.Imaging.ImageFormat.Png);
byte[] byteImage = ms.ToArray();
imgBarCode.ImageUrl = "data:image/png;base64," + Convert.ToBase64String(byteImage);
}
PlaceHolder1.Controls.Add(imgBarCode);
PlaceHolder1.Controls.Add(new HtmlGenericControl("br"));
PlaceHolder1.Controls.Add(lblvalues);
PlaceHolder1.Controls.Add(new HtmlGenericControl("br"));
}
}
else
{
//do something else
}
}
}
}
}
Screenshot
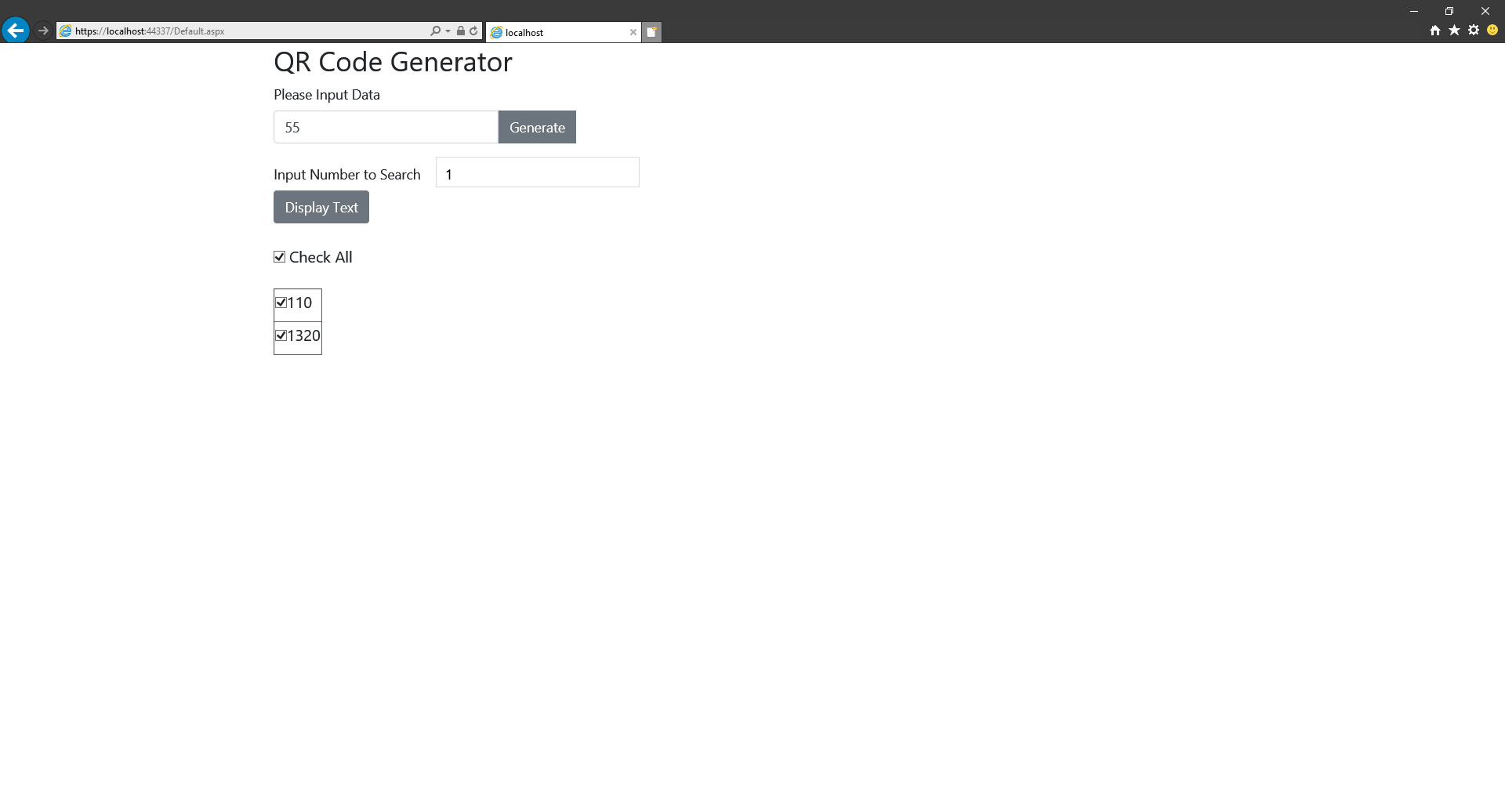
|
|
|
|
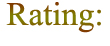 |
|
|
|
By : |
doanga1
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
|
|
Create Date : |
2019-12-21 |
|
Download : |
No files |
|
|
|
|