|
Class create table php by DownsTream
ไฟล์ dbManagement.class.php
Code
<?php
#################################################################################################
# --- Please do not delete this header ----
# DS dbManagement v1.0.0
# Class Name : dbManagement
# Version : v1.0.0
# Requirement : PHP5 >
# Build Date : December 4, 2009 - Friday
# Developer : Narong - [email protected]
# Licence : Free License (c) 2009
#
#################################################################################################
/*
--
-- โครงสร้างตาราง `test_table`
--
CREATE TABLE `test_table` (
`id` int(11) NOT NULL auto_increment,
`name` varchar(50) default NULL,
`surname` varchar(50) default NULL,
`email` varchar(50) default NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=22 ;
--
-- dump ตาราง `test_table`
--
INSERT INTO `test_table` VALUES (1, 'name1', 'surname1', 'name1@mail');
INSERT INTO `test_table` VALUES (2, 'name2', 'surname2\r\n', 'name2@mail');
INSERT INTO `test_table` VALUES (3, 'name3', 'surname3', 'name3@mail');
INSERT INTO `test_table` VALUES (4, 'name4', 'surname4', 'name4@mail');
INSERT INTO `test_table` VALUES (5, 'name5', 'surname5', 'name5@mail');
INSERT INTO `test_table` VALUES (6, 'name6', 'surname6', 'name6@mail');
INSERT INTO `test_table` VALUES (7, 'name7', 'surname7', 'name7@mail');
INSERT INTO `test_table` VALUES (8, 'name8', 'surname8', 'name8@mail');
INSERT INTO `test_table` VALUES (9, 'name9', 'surname9', 'name9@mail');
INSERT INTO `test_table` VALUES (10, 'name10', 'surname10', 'name10@mail');
INSERT INTO `test_table` VALUES (11, 'name11', 'surname11', 'name11@mail');
INSERT INTO `test_table` VALUES (12, 'name12', 'surname12', 'name12@mail');
INSERT INTO `test_table` VALUES (13, 'name13', 'surname13', 'name13@mail');
INSERT INTO `test_table` VALUES (14, 'name14', 'surname14', 'name14@mail');
INSERT INTO `test_table` VALUES (15, 'name15', 'surname15', 'name15@mail');
INSERT INTO `test_table` VALUES (16, 'name16', 'surname16', 'name16@mail');
INSERT INTO `test_table` VALUES (17, 'name17', 'surname17', 'name17@mail');
INSERT INTO `test_table` VALUES (18, 'name18', 'surname18', 'name18@mail');
INSERT INTO `test_table` VALUES (19, 'name19', 'surname19', 'name19@mail');
INSERT INTO `test_table` VALUES (20, 'name20', 'surname20', 'name20@mail');
INSERT INTO `test_table` VALUES (21, 'name21', 'surname21', 'name21@mail');
*/
# defines database connection data
define('DB_HOST', 'localhost');
define('DB_USER', 'root');
define('DB_PASS', '1234');
define('DB_DATABASE', 'test');
define('DB_CHARSET', 'UTF8');
class dbManagement {
# stored database connection
protected $mysqli;
protected $result;
protected $obj;
protected $retarr = array();
# constructor opens database connection
public function __construct() {
$this->mysqli = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_DATABASE);
$this->mysqli->set_charset(DB_CHARSET);
}
# query data from database
public function query($command) {
return $this->mysqli->query($command);
}
# insert data to database
public function insert($command) {
return $this->mysqli->query($command);
}
# update data on database
public function update($command) {
return $this->mysqli->query($command);
}
# delete data from database
public function delete($command) {
return $this->mysqli->query($command);
}
public function num_rows($command) {
return mysqli_num_rows($command);
}
# destructor closes database connection
public function __destruct() {
$this->mysqli->close();
}
}
?>
ไฟล์ create_table.class.php
Code
<?php
#################################################################################################
# --- Please do not delete this header ----
# DS CreateTable v1.0.0
# Class Name : createTable
# Version : v1.0.0
# Requirement : PHP5 >
# Build Date : December 4, 2009 - Friday
# Developer : Narong - [email protected]
# Licence : Free License (c) 2009
#
#################################################################################################
# database configuration
require_once('dbManagement.class.php');
class createTable extends dbManagement {
protected $table;
protected $headers = array();
protected $data = array();
protected $attr = array();
protected $css_class;
protected $endl = "\n";
protected $tab_lv1 = ' ';
protected $tab_lv2 = ' ';
protected $tab_lv3 = ' ';
# set table attribute
public function setTableAttribute($attribute) {
$this->attr = $attribute;
}
# set table header
public function setTableHeader($header) {
$this->headers = $header;
}
# set table body
public function setTableBody($data) {
$this->data = $data;
}
# print table
public function getTable() {
# open table tag
$this->table = '<table id="'.$this->attr[0].'" border="1" width="'.
$this->attr[1].'">'.$this->endl;
#begin table head
$this->table .= $this->tab_lv1.'<thead>'.$this->endl;
$this->table .= $this->tab_lv2.'<tr>'.$this->endl;
foreach($this->headers as $val) {
$this->table .= $this->tab_lv3.'<th>'.$val.'</th>'.$this->endl;
}
$this->table .= $this->tab_lv2.'</tr>'.$this->endl;
$this->table .= $this->tab_lv1.'</thead>'.$this->endl;
#end table head
# begin table body
$this->table .= $this->tab_lv1.'<tbody>'.$this->endl;
foreach($this->data as $index=>$rows) {
($index%2 == 1) ? $this->css_class = $this->attr[2]
: $this->css_class = $this->attr[3];
$this->table .= $this->tab_lv2.'<tr '.$this->css_class.'>'.$this->endl;
foreach ($rows as $key=>$val)
$this->table .= $this->tab_lv3.'<td>'.$rows[$key].'</td>'.$this->endl;
$this->table .= $this->tab_lv2.'</tr>'.$this->endl;
}
$this->table .= $this->tab_lv1.'</tbody>'.$this->endl;
# end table body
# close table tag
$this->table .= '</table>'.$this->endl;
echo $this->table;
}
# print array
public function show($arr) {
echo '<pre>'; print_r($arr); echo '</pre>';
}
}
?>
ไฟล์ kgPager.class.php
Code
<?php
#################################################################################################
#
# KG Pager v2.0.1
# Class Name : KG Pager Class
# Version : 2.0.1
# Requirement : PHP4 >
# Build Date : December 17, 2007 - Monday
# Developer : Muharrem ERİN (TÜRKİYE) - [email protected] - muharremerin.com - mhrrmrnr.com - kisiselgunce.com
# Licence : GNU General Public License (c) 2007
#
#################################################################################################
// pager class
class kgPager {
var $total_records = NULL;
var $start = NULL;
var $scroll_page = NULL;
var $per_page = NULL;
var $total_pages = NULL;
var $current_page = NULL;
var $page_links = NULL;
// total pages and essential variables
function total_pages ($pager_url, $total_records, $scroll_page, $per_page, $current_page) {
$this -> url = $pager_url;
$this -> total_records = $total_records;
$this -> scroll_page = $scroll_page;
$this -> per_page = $per_page;
if (!is_numeric($current_page)) {
$this -> current_page = 1;
}else{
$this -> current_page = $current_page;
}
if ($this -> current_page == 1) $this -> start = 0; else $this -> start = ($this -> current_page - 1) * $this -> per_page;
$this -> total_pages = ceil($this -> total_records / $this -> per_page);
}
// page links
function page_links ($inactive_page_tag, $pager_url_last) {
if ($this -> total_pages <= $this -> scroll_page) {
if ($this -> total_records <= $this -> per_page) {
$loop_start = 1;
$loop_finish = $this -> total_pages;
}else{
$loop_start = 1;
$loop_finish = $this -> total_pages;
}
}else{
if($this -> current_page < intval($this -> scroll_page / 2) + 1) {
$loop_start = 1;
$loop_finish = $this -> scroll_page;
}else{
$loop_start = $this -> current_page - intval($this -> scroll_page / 2);
$loop_finish = $this -> current_page + intval($this -> scroll_page / 2);
if ($loop_finish > $this -> total_pages) $loop_finish = $this -> total_pages;
}
}
for ($i = $loop_start; $i <= $loop_finish; $i++) {
if ($i == $this -> current_page) {
$this -> page_links .= '<span '.$inactive_page_tag.'>'.$i.'</span>';
}else{
$this -> page_links .= '<span><a href="'.$this -> url.$i.$pager_url_last.'">'.$i.'</a></span>';
}
}
}
// previous page
function previous_page ($previous_page_text, $pager_url_last) {
if ($this -> current_page > 1) {
$this -> previous_page = '<span><a href="'.$this -> url.($this -> current_page - 1).$pager_url_last.'">'.$previous_page_text.'</a></span>';
}
}
// next page
function next_page ($next_page_text, $pager_url_last) {
if ($this -> current_page < $this -> total_pages) {
$this -> next_page = '<span><a href="'.$this -> url.($this -> current_page + 1).$pager_url_last.'">'.$next_page_text.'</a></span>';
}
}
// first page
function first_page ($first_page_text, $pager_url_last) {
if ($this -> current_page > 1) {
$this -> first_page = '<span><a href="'.$this -> url.'1'.$pager_url_last.'">'.$first_page_text.'</a></span>'; // :)
}
}
// last page
function last_page ($last_page_text, $pager_url_last) {
if ($this -> current_page < $this -> total_pages) {
$this -> last_page = '<span><a href="'.$this -> url.$this -> total_pages.$pager_url_last.'">'.$last_page_text.'</a></span>';
}
}
// pages functions set
function pager_set ($pager_url, $total_records, $scroll_page, $per_page, $current_page, $inactive_page_tag, $previous_page_text, $next_page_text, $first_page_text, $last_page_text) {
$this -> total_pages($pager_url, $total_records, $scroll_page, $per_page, $current_page);
$this -> page_links($inactive_page_tag, $pager_url_last);
$this -> previous_page($previous_page_text, $pager_url_last);
$this -> next_page($next_page_text, $pager_url_last);
$this -> first_page($first_page_text, $pager_url_last);
$this -> last_page($last_page_text, $pager_url_last);
}
}
?>
ไฟล์ index.php
Code
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Class Create Table</title>
<style type="text/css">
body { font-size:12px; font-family:Tahoma, Geneva, sans-serif; }
#ex { border-collapse:collapse; border-color:#bbb; font-size:11px; font-family:Tahoma, Geneva, sans-serif; }
#ex thead { background-color:#666; color:#FFF; }
#ex thead th { background-color:#666; color:#FFF; height:30px; border-color:#eee;}
#ex tbody td { text-align:center; height:25px; padding-left:10px; padding-right:10px; border-color:#bbb;}
#ex tbody td span.name { color:#444; }
#ex tbody td span.sname { color:#444; }
#ex tbody tr:hover { background-color:#F93;}
.odd { background-color:#fff; }
.even { background-color:#eee; }
.pager_links a { text-decoration:none; color:#ff3300; background:#fff; border:1px solid #e0e0e0;
padding:1px 4px 1px 4px; margin:2px; }
.pager_links a:hover { text-decoration:none; color:#3399ff; background:#f2f2f2; border:1px solid #3399ff;
padding:1px 4px 1px 4px; margin:2px; }
.current_page { border:1px solid #333; padding:1px 4px 1px 4px; margin:2px; color:#333; }
a:link { text-decoration: none; }
a:hover { text-decoration: none; color: #FFF; }
a:active { text-decoration: none; color: #F00; }
</style>
</head>
<?php
require_once('create_table.class.php');
require_once('kgPager.class.php');
# create table object
$table = new createTable();
# string query
$command = 'SELECT * FROM test_table';
# get object result from database
$result = $table->query($command);
$total_records =$table->num_rows($result); // จำนวน record ทั้งหมด
$scroll_page = 5; // จำนวน scroll page
$per_page = 5; // จำนวน record ต่อหนึ่ง page
$current_page = $_GET['page']; // หน้าปัจจุบัน
$pager_url = 'index.php?page='; // url page ที่ต้องการเรียก
$inactive_page_tag = 'class="current_page"'; //
$previous_page_text = '< ก่อนหน้า'; // หน้าก่อนหน้า
$next_page_text = 'หน้าถัดไป >'; // หน้าถัดไป
$first_page_text = '<< หน้าแรก'; // ไปหน้าแรก
$last_page_text = 'หน้าสุดท้าย >>'; // ไปหน้าสุดท้าย
$kgPagerOBJ = & new kgPager();
$kgPagerOBJ -> pager_set($pager_url, $total_records, $scroll_page, $per_page, $current_page, $inactive_page_tag, $previous_page_text, $next_page_text, $first_page_text, $last_page_text);
echo '<p><strong>จำนวนทั้งหมด : </strong>';
echo $kgPagerOBJ -> total_pages;
echo ' หน้า</p>';
$result = $table->query($command." ORDER BY id DESC LIMIT ".$kgPagerOBJ -> start.", ".$kgPagerOBJ -> per_page."");
echo '<p class="pager_links">';
echo isset($kgPagerOBJ->first_page) ? $kgPagerOBJ->first_page : '';
echo isset($kgPagerOBJ->previous_page) ? $kgPagerOBJ->previous_page : '';
echo isset($kgPagerOBJ->page_links) ? $kgPagerOBJ->page_links : '';
echo isset($kgPagerOBJ->next_page) ? $kgPagerOBJ->next_page : '';
echo isset($kgPagerOBJ->last_page) ? $kgPagerOBJ->last_page : '';
echo '</p>';
# fetch object result store to array data
while($rs = $result->fetch_object()) {
$data[] = array(
$rs->id,
'<span class="name">'.$rs->name.'</span>',
'<span class="sname">'.$rs->surname.'</span>',
'<a href="mailto:[email protected]">'.$rs->email.'</a>',
'<a href="javascript:alert(\''.$rs->id.'\');"><img src="images/icons/User-Edit.png" border="0" /></a>',
'<a href="javascript:alert(\''.$rs->id.'\');"><img src="images/icons/User-Cancel.png" border="0" /></a>');
}
# show array data
# $table->show($data);
# parameter of attribute
$att = array('ex', '', 'class="odd"', 'class="even"');
# parameter of header
$head = array('ID', 'Name', 'Surname', 'Email', 'Edit','Delete');
# call member function to set table attribute
$table->setTableAttribute($att);
# call member function to set table header
$table->setTableHeader($head);
# call member function to set table body
$table->setTableBody($data);
# print table
$table->getTable();
?>
<body>
</body>
</html>
Output

|
|
|
|
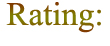 |
|