|
C# DataGridView + OpenFileDialog แบบบ้านๆ |
C# DataGridView + OpenFileDialog แบบบ้านๆ วันนี้จะขอนำเสนอ การเพิ่ม DataGridViewTextBoxColumn แบบมีปุ่มกด พอดีมีวันหนึ่ง QC document มาบอกว่า ตารางอยากให้กดเลือกไฟล์ได้เลย ตอนแรกก็งงแต่คิดไปคิดมามันก็น่าจะทำได้นี่นา ก็เลยลองหาว่าธี เพิ่ม DataGridViewTextBoxColumn แบบมีปุ่มกด เพื่อ select path ได้
โดยผมให้เป็น DataGridViewFilePathColumn ครับ โค๊ดบ้านๆมีดังนี้
Code (C#)
using System.Drawing;
using System.Windows.Forms;
#region _DataGridViewFilePathColumn
/// <summary>
/// Hosts a collection of DataGridViewTextBoxCell cells.
/// </summary>
public class DataGridViewFilePathColumn : DataGridViewColumn
{
public enum PathType { directory, file };
public PathType pathtype = PathType.file;
public DataGridViewFilePathColumn()
: base(new DataGridViewFilePathCell())
{
}
public override DataGridViewCell CellTemplate
{
get
{
return base.CellTemplate;
}
set
{
if (null != value &&
!value.GetType().IsAssignableFrom(typeof(DataGridViewFilePathCell)))
{
throw new InvalidCastException("must be a DataGridViewFilePathCell");
}
base.CellTemplate = value;
}
}
}
/// <summary>
/// Displays editable text information in a DataGridView control. Uses
/// PathEllipsis formatting if the column is smaller than the width of a
/// displayed filesystem path.
/// </summary>
public class DataGridViewFilePathCell : DataGridViewTextBoxCell
{
Button browseButton;
Dictionary<Color, SolidBrush> brushes = new Dictionary<Color, SolidBrush>();
protected virtual SolidBrush GetCachedBrush(Color color)
{
if (this.brushes.ContainsKey(color))
return this.brushes[color];
SolidBrush brush = new SolidBrush(color);
this.brushes.Add(color, brush);
return brush;
}
protected virtual bool RightToLeftInternal
{
get
{
return this.DataGridView.RightToLeft == RightToLeft.Yes;
}
}
protected override void OnClick(DataGridViewCellEventArgs e)
{
base.OnClick(e);
if (this.DataGridView.CurrentCell == this )
{
string file = Convert.ToString(base.Value);
if (!System.IO.File.Exists(file))
{
MessageBox.Show("ไม่พบไฟล์ " + file);
}
else
{
System.Diagnostics.Process.Start(file);
}
}
}
protected override void Paint(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, DataGridViewElementStates cellState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts)
{
if (cellStyle == null) { throw new ArgumentNullException("cellStyle"); }
this.PaintPrivate(graphics, clipBounds, cellBounds, rowIndex, cellState, formattedValue, errorText, cellStyle, advancedBorderStyle, paintParts);
}
protected Rectangle PaintPrivate(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, DataGridViewElementStates cellState, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts)
{
System.Diagnostics.Debug.WriteLine(string.Format("Painting Cell row {0} for rowindex {2} with rectangle {1}", this.RowIndex, cellBounds, rowIndex));
SolidBrush cachedBrush;
Rectangle empty = Rectangle.Empty;
if (((paintParts & DataGridViewPaintParts.Border) != DataGridViewPaintParts.None)) { this.PaintBorder(graphics, clipBounds, cellBounds, cellStyle, advancedBorderStyle); }
Rectangle rectangle2 = this.BorderWidths(advancedBorderStyle);
Rectangle borderedCellRectangle = cellBounds;
borderedCellRectangle.Offset(rectangle2.X, rectangle2.Y);
borderedCellRectangle.Width -= rectangle2.Right;
borderedCellRectangle.Height -= rectangle2.Bottom;
Point currentCellAddress = base.DataGridView.CurrentCellAddress;
bool isFirstCell = (currentCellAddress.X == base.ColumnIndex) && (currentCellAddress.Y == rowIndex);
bool flagisFirstCellAndNotEditing = isFirstCell && (base.DataGridView.EditingControl != null);
bool thisCellIsSelected = (cellState & DataGridViewElementStates.Selected) != DataGridViewElementStates.None;
cachedBrush = ((((paintParts & DataGridViewPaintParts.SelectionBackground) != DataGridViewPaintParts.None) && thisCellIsSelected) && !flagisFirstCellAndNotEditing) ? GetCachedBrush(cellStyle.SelectionBackColor) : GetCachedBrush(cellStyle.BackColor);
if (((((paintParts & DataGridViewPaintParts.Background) != DataGridViewPaintParts.None)) && ((cachedBrush.Color.A == 0xff) && (borderedCellRectangle.Width > 0))) && (borderedCellRectangle.Height > 0))
{
graphics.FillRectangle(cachedBrush, borderedCellRectangle);
}
if (cellStyle.Padding != Padding.Empty)
{
if (RightToLeftInternal)
{
borderedCellRectangle.Offset(cellStyle.Padding.Right, cellStyle.Padding.Top);
}
else
{
borderedCellRectangle.Offset(cellStyle.Padding.Left, cellStyle.Padding.Top);
}
borderedCellRectangle.Width -= cellStyle.Padding.Horizontal;
borderedCellRectangle.Height -= cellStyle.Padding.Vertical;
}
if (((isFirstCell) && (!flagisFirstCellAndNotEditing && ((paintParts & DataGridViewPaintParts.Focus) != DataGridViewPaintParts.None))) && ((ShowFocusCues && base.DataGridView.Focused) && ((borderedCellRectangle.Width > 0) && (borderedCellRectangle.Height > 0))))
{
ControlPaint.DrawFocusRectangle(graphics, borderedCellRectangle, Color.Empty, cachedBrush.Color);
}
Rectangle cellValueBounds = borderedCellRectangle;
string text = formattedValue as string;
if ((text != null) && (!flagisFirstCellAndNotEditing))
{
int y = (cellStyle.WrapMode == DataGridViewTriState.True) ? 1 : 2;
borderedCellRectangle.Offset(0, y);
borderedCellRectangle.Width = borderedCellRectangle.Width;
borderedCellRectangle.Height -= y + 1;
if ((borderedCellRectangle.Width > 0) && (borderedCellRectangle.Height > 0))
{
TextFormatFlags flags = TextFormatFlags.PathEllipsis;
if (((paintParts & DataGridViewPaintParts.ContentForeground) != DataGridViewPaintParts.None))
{
if ((flags & TextFormatFlags.SingleLine) != TextFormatFlags.GlyphOverhangPadding) { flags |= TextFormatFlags.EndEllipsis; }
DataGridViewFilePathColumn filePathColumn = (DataGridViewFilePathColumn)this.DataGridView.Columns[ColumnIndex];
if (this.RowIndex >= 0)
{
bool changed = false;
if ((browseButton.Width != Math.Max(10, borderedCellRectangle.Width / 4)) && (browseButton.Width != 20))
{
System.Diagnostics.Trace.WriteLine(string.Format("browseButton Width was incorrect:{0} for given rectangle:{1}", browseButton.Width, borderedCellRectangle));
browseButton.Width = Math.Max(10, borderedCellRectangle.Width / 4);
browseButton.Width = Math.Min(browseButton.Width, 20);
changed = true;
}
if (browseButton.Height != (borderedCellRectangle.Height + 4))
{
System.Diagnostics.Trace.WriteLine(string.Format("browseButton Height was incorrect:{0} for given rectangle:{1}", browseButton.Height, borderedCellRectangle));
browseButton.Height = borderedCellRectangle.Height + 4;
changed = true;
}
Point loc = new Point();
loc.X = borderedCellRectangle.X + borderedCellRectangle.Width - browseButton.Width;
loc.Y = borderedCellRectangle.Y - 4;
if (browseButton.Location != loc)
{
System.Diagnostics.Trace.WriteLine(string.Format("browseButton location was incorrect:{0} for given rectangle:{1} with loc: {2}", browseButton.Location, borderedCellRectangle, loc));
browseButton.Location = loc;
changed = true;
}
if (changed)
browseButton.Invalidate();
if (!this.DataGridView.Controls.Contains(browseButton))
this.DataGridView.Controls.Add(browseButton);
borderedCellRectangle.Width -= browseButton.Width;
}
}
TextRenderer.DrawText(graphics, text, cellStyle.Font, borderedCellRectangle, thisCellIsSelected ? cellStyle.SelectionForeColor : cellStyle.ForeColor, flags);
}
}
if ((base.DataGridView.ShowCellErrors) && ((paintParts & DataGridViewPaintParts.ErrorIcon) != DataGridViewPaintParts.None))
{
if ((!string.IsNullOrEmpty(errorText) && (cellValueBounds.Width >= 20)) && (cellValueBounds.Height >= 0x13))
{
Rectangle iconBounds = this.GetErrorIconBounds(graphics, cellStyle, rowIndex);
if ((iconBounds.Width >= 4) && (iconBounds.Height >= 11))
{
iconBounds.X += cellBounds.X;
iconBounds.Y += cellBounds.Y;
Bitmap errorBitmap = new Bitmap(typeof(DataGridViewCell), "DataGridViewRow.error.bmp");
errorBitmap.MakeTransparent();
if (errorBitmap != null)
{
lock (errorBitmap)
{
graphics.DrawImage(errorBitmap, iconBounds, 0, 0, 12, 11, GraphicsUnit.Pixel);
}
}
}
}
}
return empty;
}
public bool ShowFocusCues
{
get { return true; }
}
protected bool ApplyVisualStylesToHeaders
{
get
{
if (Application.RenderWithVisualStyles)
{
return this.DataGridView.EnableHeadersVisualStyles;
}
return false;
}
}
private void DataGridView_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (base.Value.ToString().Length > 0)
{
System.Diagnostics.Process.Start(base.Value.ToString());
}
}
void browseButton_Click(object sender, EventArgs e)
{
DataGridViewFilePathColumn filePathColumn = (DataGridViewFilePathColumn)this.DataGridView.Columns[ColumnIndex];
if (filePathColumn.pathtype == DataGridViewFilePathColumn.PathType.file)
{
OpenFileDialog fdg = new OpenFileDialog();
fdg.ShowDialog();
base.Value = (fdg.FileName.Length > 0) ? fdg.FileName : base.Value;
}
else
{
FolderBrowserDialog fdg = new FolderBrowserDialog();
fdg.ShowDialog();
base.Value = (fdg.SelectedPath.Length > 0) ? fdg.SelectedPath : base.Value;
}
}
public DataGridViewFilePathCell()
: base()
{
browseButton = new Button();
browseButton.Text = "…";
browseButton.Click += new EventHandler(browseButton_Click);
}
}
#endregion
หลังจากนั้นก็เพิ่ม DataGridView ในฟอร์ม
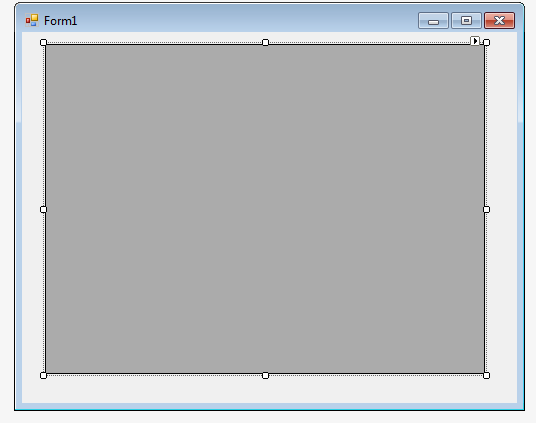
จากนั้นก็ Add column โดยเลือกเป็น DataGridViewFilePathColumn
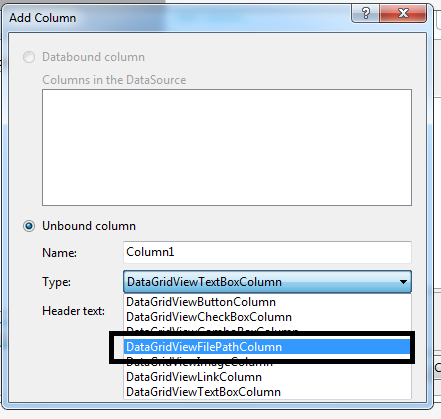
เพียงแค่นี้พอเรา รันโปรแกรมขึ้นมาก็สามารถใช้ DataGridViewFilePathColumn ได้เลยครับ
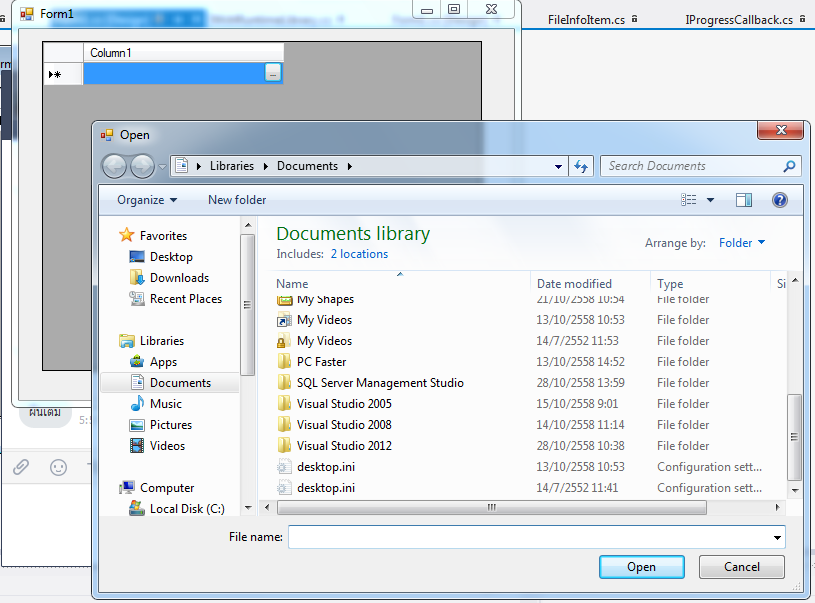
C# datagridview openfile แบบบ้านๆ ก็ขอจบลงเพียงเท่านี้ครับ เนื่องจากใช้ความรู้แบบบ้านๆ จึงได้โค้ด และ หลักการแบบบ้านๆ มาเขียนบทความแบบบ้านๆ ให้อ่านกันครับ

|
|
|
|
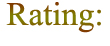 |
|
|
|
By : |
TOR_CHEMISTRY
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
  |
|
Create Date : |
2015-10-28 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|