|
C# การใช้ DateTimePicker เพื่อใช้เปรียบเทียบวันเวลาแบบบ้านๆ |
C# การใช้ DateTimePicker เพื่อใช้เปรียบเทียบวันเวลาแบบบ้านๆ หลังจากผ่านการตรวจ(Audit) ในห้องปฎิบัติการผ่านด้วย(ไม่)ดี ได้ CAR ซักโหลเห็นจะได้
ผมมองเห็นว่าโปรแกรมที่ผมเขียนจะไม่สะดวกในการ preview ดูหากมีข้อมูลเยอะๆ
ซึ่งส่วนใหญ่เป็นข้อมูลบันทึกประจำวัน
ผมจึงได้ดึง dateTimePicker ลงในฟอร์มแล้วให้ User เลือก preview ตามวัน เวลา ได้ง่ายขึ้น
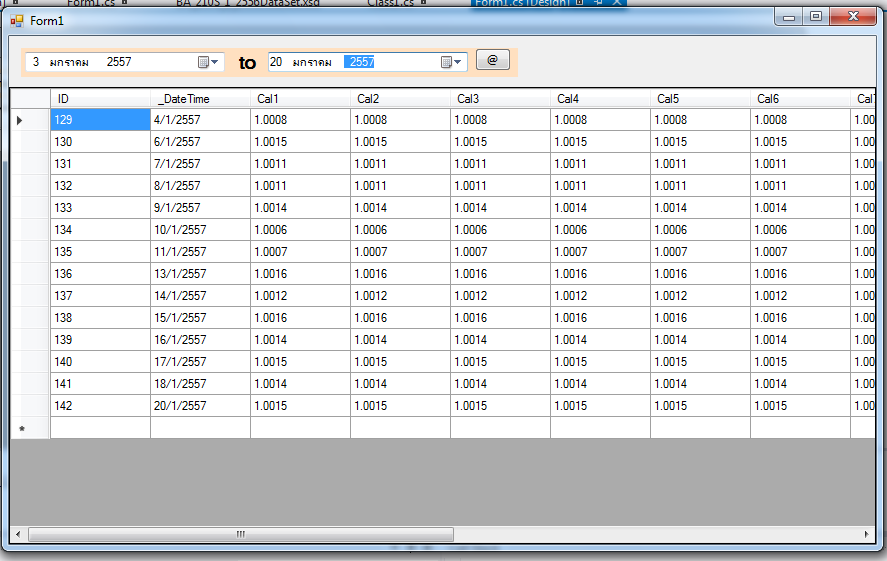
แต่ถ้าจะให้ดึง dateTimePicker ลงในฟอร์ม ทุก ฟอร์ม แล้วมานั่งแก้ค่า คงเสียเวลาไปเยอะ
ดังนั้นผมจึงเขียน UserControl ตัวหนึ่งขึ้นมาเพื่อให้สะดวกในการ เลือก preview ตามวัน เวลา ได้ง่ายขึ้น
หน้าตาก็เหมือนในรูปด้านบนครับ

ส่วนโค้ด
Code (C#)
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System;
using System.ComponentModel;
namespace TORServices.Forms
{
public partial class refDate : UserControl
{
private System.Windows.Forms.DateTimePicker dateTimePicker1;
private System.Windows.Forms.DateTimePicker dateTimePicker2;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Label label1;
private void InitializeComponent()
{
this.dateTimePicker1 = new System.Windows.Forms.DateTimePicker();
this.dateTimePicker2 = new System.Windows.Forms.DateTimePicker();
this.label1 = new System.Windows.Forms.Label();
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// dateTimePicker1
//
this.dateTimePicker1.Location = new System.Drawing.Point(4, 4);
this.dateTimePicker1.Name = "dateTimePicker1";
this.dateTimePicker1.Size = new System.Drawing.Size(200, 20);
this.dateTimePicker1.TabIndex = 0;
this.dateTimePicker1.ValueChanged += new System.EventHandler(this.dateTimePicker1_ValueChanged);
//
// dateTimePicker2
//
this.dateTimePicker2.Location = new System.Drawing.Point(247, 4);
this.dateTimePicker2.Name = "dateTimePicker2";
this.dateTimePicker2.Size = new System.Drawing.Size(200, 20);
this.dateTimePicker2.TabIndex = 1;
this.dateTimePicker2.ValueChanged += new System.EventHandler(this.dateTimePicker1_ValueChanged);
//
// label1
//
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("Microsoft Sans Serif", 14.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(222)));
this.label1.Location = new System.Drawing.Point(214, 2);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(27, 24);
this.label1.TabIndex = 3;
this.label1.Text = "to";
//
// button1
//
this.button1.Location = new System.Drawing.Point(454, 0);
this.button1.Name = "button1";
this.button1.Text = "@";
this.button1.Size = new System.Drawing.Size(36, 23);
this.button1.TabIndex = 2;
this.button1.UseVisualStyleBackColor = true;
//
// refDate
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(224)))), ((int)(((byte)(192)))));
this.Controls.Add(this.label1);
this.Controls.Add(this.button1);
this.Controls.Add(this.dateTimePicker2);
this.Controls.Add(this.dateTimePicker1);
this.Name = "refDate";
this.Size = new System.Drawing.Size(497, 29);
this.Resize += new System.EventHandler(this.refDate_Resize);
this.ResumeLayout(false);
this.PerformLayout();
}
public refDate()
{
InitializeComponent();
}
private EventHandler onValueChanged;
[
Localizable(true),
RefreshProperties(RefreshProperties.Repaint),
]
public string CustomFormat
{
get
{
return dateTimePicker1.CustomFormat;
}
set
{
if (DateTimeFormat == DateTimePickerFormat.Custom)
{
dateTimePicker1.CustomFormat = value;
dateTimePicker2.CustomFormat = value;
}
}
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.DefaultValue(DateTimePickerFormat.Long)]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("Open Dialog is dispalyed and on success the contents of the Cell is replaced with the new path.")]
public DateTimePickerFormat DateTimeFormat
{
get { return dateTimePicker1.Format; }
set { dateTimePicker1.Format = value; dateTimePicker2.Format = value; }
}
public DateTime DateStart
{
get { return dateTimePicker1.Value; }
set { dateTimePicker1.Value = value; }
}
public DateTime DateFinal
{
get { return dateTimePicker2.Value; }
set { dateTimePicker2.Value = value; }
}
public event EventHandler ButtonRefreshClick
{
add
{
button1.Click += value;
}
remove
{
button1.Click -= value;
}
}
public event EventHandler ValueChanged
{
add
{
onValueChanged += value;
}
remove
{
onValueChanged -= value;
}
}
protected virtual void OnValueChanged(EventArgs eventargs)
{
if (onValueChanged != null)
{
onValueChanged(this, eventargs);
}
}
private void refDate_Resize(object sender, EventArgs e)
{
this.Size = new Size(497, 29);
}
private void dateTimePicker1_ValueChanged(object sender, EventArgs e)
{
if (onValueChanged != null)
{
onValueChanged(this, e);
}
}
}
}
เราสามารถ ลากลงฟอร์ม เพื่อใช้งานได้เลย
โดยจะมี คุณสมบัติ CustomFormat,DateTimeFormat เพื่อให้ปรับแก้เหมือนกับ dateTimePicker
DateStart,DateFinal เพื่อกำหนดวันเริ่มต้น และ วันสิ้นสุดดังกล่าว
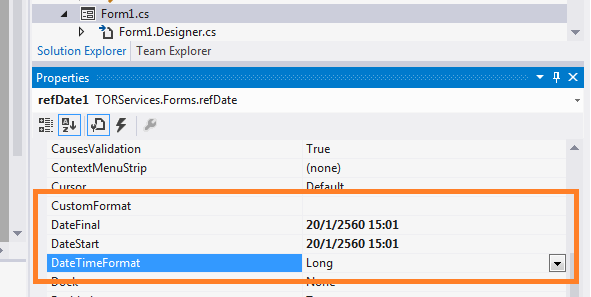
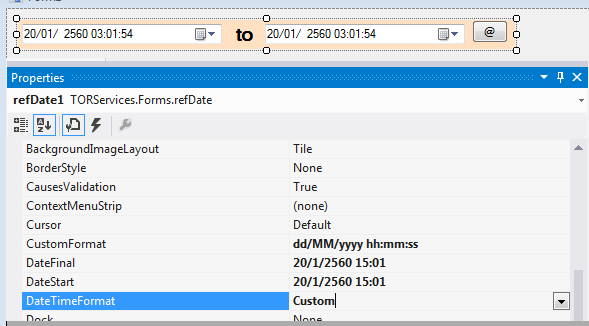
มี Event ButtonRefreshClick กรณีที่ User คลิกปุ่ม
Event ValueChanged กรณีที่ DateStart,DateFinal เปลี่ยนค่ากันด้วยเช่นกันครับ

Code (C#)
private void refDate1_ValueChanged(object sender, EventArgs e)
{
//Preview by Date
this.aLS_F_717TableAdapter.FillByDate(this.bA_210S_1_2556DataSet.ALS_F_717,refDate1.DateStart,refDate1.DateFinal);
}
private void refDate1_ButtonRefreshClick(object sender, EventArgs e)
{
//Preview All Data
this.aLS_F_717TableAdapter.Fill(this.bA_210S_1_2556DataSet.ALS_F_717);
}
เพียงแค่นี้เราก็ไม่ต้องมานั่งเพิ่ม dateTimePicker และ จัดค่าต่างๆให้เสียเวลาแล้วครับ
พอดีผมเขียนแล้วเห็นว่ามีประโยชน์เผื่อบางท่านสนใจ จึงนำมาแชร์ให้ใช้ๆกันครับ
|
|
|
|
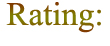 |
|
|
|
By : |
TOR_CHEMISTRY
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2017-01-20 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|