|
C# Extension Methods แบบ บ้านๆ |
วันนี้ ผม จะมาสอนจระเข้ให้ว่ายน้ำกันครับ
ทุกคนทีเขียนโปรแกรม ก็มักจะเจอว่าเวลาเราเรียกใช้ตัวแปร ต่างๆ แล้วพิมพ์ จุดแล้วมักจะเจอว่ามี method หรือ function ขึ้นมาให้เลือกแบบนี้
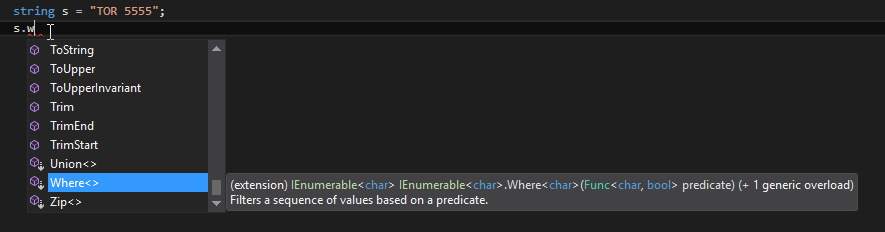
ขอให้อ่านเรื่อง Modifiersซักหน่อยก่อนที่จะอ่าน เนื้อหาต่อไป นะครับ
https://docs.microsoft.com/en-us/dotnet/csharp/language-reference/keywords/modifiers
หากเข้าใจเรื่อง Modifiers แบบ คร่าวๆ แล้ว ก็ไปต่อได้ครับ
ตัว Whereจะเป็น extension methods จะสังเกตว่ามันจะมี(extensions) อยู่ด้วย
ผมก็คงต้องให้อ่าน เรื่อง extension methods ดูอีกละครับ
https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/classes-and-structs/extension-methods
ถ้าจะให้พูดแบบบ้านๆ ก็คือ
2.static class มันคือ คลาส ที่เราเรียกใช้ได้เลย ไม่ต้องมานั่งประกาศตัวแปร
public class
Code (C#)
Form1 f = New Form1();
f.Text = "TOR 5555";
หรือ
Code (C#)
New Form1().Text = "TOR 5555";
แต่ถ้าเป็น static class
Code (C#)
string.Format("0.00",2.2351d);
2. extension methods มันก็เป็น methods ที่จะเรียกใช้ได้ผ่าน ชนิดของตัวแปรนั้นๆ
ตัว Where จะอยู่ใน Enumerable.cs
https://referencesource.microsoft.com/#system.core/System/Linq/Enumerable.cs,37c6daad6e403a3b
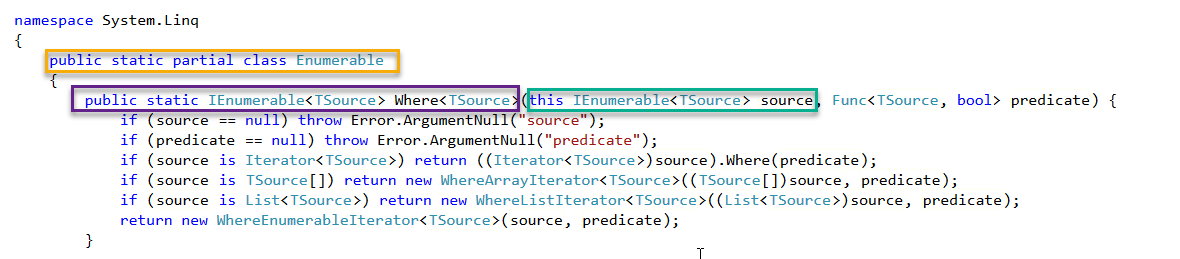
จะเห็นชัดว่า คลาสนี้เป็น static class จาก
Code (VB.NET)
public static partial class Enumerable
และเป็น static methods จาก
Code (C#)
public static IEnumerable<TSource> Where<TSource>
ที่จะส่งกลับค่าออกมาเป็น IEnumerable<TSource>
แค่นี้ยังไม่ทำให้เป็น extension methods ครับ จนกว่าเราจะ เพิ่ม this
ที่หน้าตัวแปร ที่เราต้องการ แบบนี้
Code (C#)
this IEnumerable<TSource> source
จะเห็นว่า เมื่อ เราเพิ่ม this หน้า IEnumerable<TSource> source จะทำให้เป็น extension methods ขึ้นมาทันที
จากนี้ เราจะมาลอง สร้าง extension methods ของเราเอง ครับ
โดยมีขั้นตอน ประมาณนี้
1. สร้าง static class ขึ้นมาก่อน
Code (C#)
public static class Tor_extension
{
}
2. สร้าง static methods ขึ้นมา
Code (C#)
public static class Tor_extension
{
public static void Testextensions(string s)
{
MessageBox.Show(s + " by TOR");
}
}

ในขึ้นนี้ เราสามารถเรียกใช้ คลาส Tor_extension แบบ ไม่ต้องประกาศตัวแปรได้แล้ว
และเรียกใช้ Testextensions ใน Tor_extension แต่มันยังไม่เป็น extension methods
2. เพิ่ม this หน้า ตัวแปร
Code (C#)
public static class Tor_extension
{
public static void Testextensions(this string s)
{
MessageBox.Show(s + " by TOR");
}
}
คราวนี้ เราก็ใช้ Testextensions แบบ extension methods ได้แล้ว ครับ
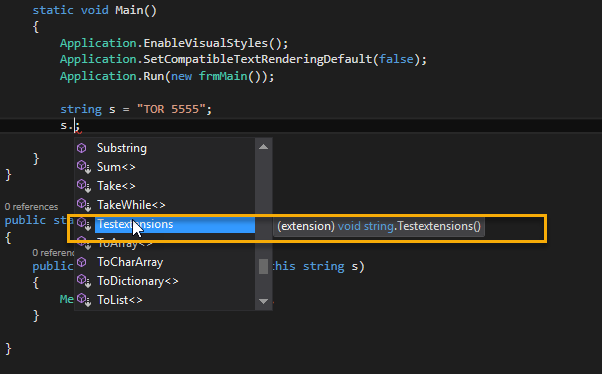
เห็นไม๊ครับว่ามัน ง๊ายง่าย
มันจะมีประโยชน์ให้ยุ่งทำไมน่ะหรือครับ
ถ้าเราศึกษามันแล้ว จะเห็นว่ามันใช้งานได้หลายอย่างครับ
ยกตัวอย่าง extension methods ที่ใช้กับ DataGridView นะครับ
กรณี เรามี DataGridView เยอะๆ เราก็เขียน extension methods ไว้จัดการ พวก สี ฟอนต์ format ประมาณนี้
Code (C#)
namespace TORServices.ExtensionTor
{
public static class extGridview
{
public static void SetDefaultCellStyleColor(this System.Windows.Forms.DataGridView dgv,
int ColumnIndex)
{
string s1 = "";
string s2 = "";
System.Drawing.Color Color1 = System.Drawing.Color.Beige;
System.Drawing.Color Color2 = System.Drawing.Color.LightGreen;
System.Drawing.Color cl = Color1;
for (int i = 0; i < dgv.Rows.Count - 1; i++)
{
if (i == 0)
{
dgv.Rows[i].DefaultCellStyle.BackColor = cl;
}
else
{
s1 = dgv[ColumnIndex, i].Value.ToString().Replace(" ", "").ToUpper();
s2 = dgv[ColumnIndex, i - 1].Value.ToString().Replace(" ", "").ToUpper();
cl = (s1 == s2) ? cl : (cl != Color1) ? Color1 : Color2;
dgv.Rows[i].DefaultCellStyle.BackColor = cl;
}
}
}
public static void SetDefaultCellStyle(this System.Windows.Forms.DataGridView dgv,
bool RowHeadersVisible = true,DataGridViewSelectionMode _SelectionMode = DataGridViewSelectionMode.FullRowSelect )
{
System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle1 = new System.Windows.Forms.DataGridViewCellStyle();
dataGridViewCellStyle1.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(255)))), ((int)(((byte)(192)))));
dataGridViewCellStyle1.SelectionBackColor = System.Drawing.Color.Yellow;
dataGridViewCellStyle1.SelectionForeColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(0)))), ((int)(((byte)(192)))));
dataGridViewCellStyle1.Font = new System.Drawing.Font("Microsoft Sans Serif", 10);
System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle2 = new System.Windows.Forms.DataGridViewCellStyle();
dataGridViewCellStyle2.BackColor = System.Drawing.Color.White;
dataGridViewCellStyle2.SelectionBackColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(255)))), ((int)(((byte)(192)))));
dataGridViewCellStyle2.SelectionForeColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(0)))), ((int)(((byte)(64)))));
dataGridViewCellStyle2.Font = new System.Drawing.Font("Microsoft Sans Serif", 10);
dgv.AlternatingRowsDefaultCellStyle = dataGridViewCellStyle1;
dgv.RowsDefaultCellStyle = dataGridViewCellStyle2;
dgv.RowHeadersVisible = RowHeadersVisible;
dgv.SelectionMode = _SelectionMode;
}
}
}
เวลาใช้งานก็แค่
Code (C#)
postcodeViewDataGridView.SetDefaultCellStyle();
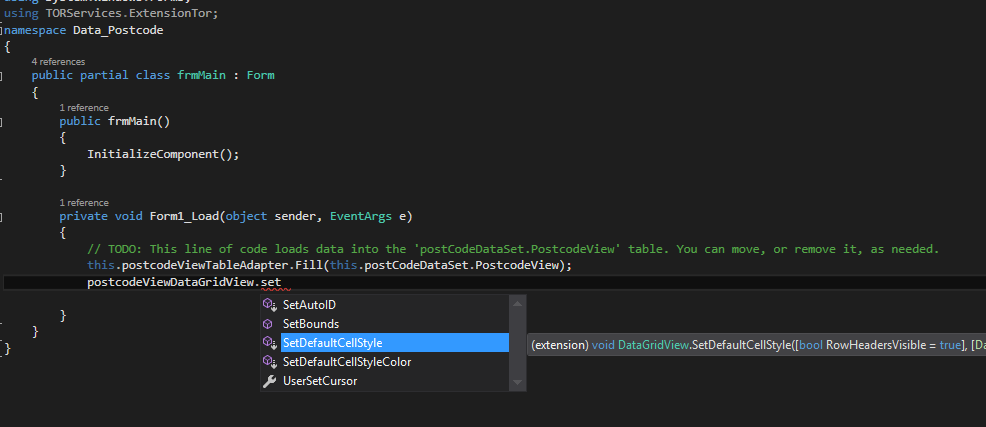
จุดที่ควรจับ คือ เราอยากให้ datatype ไหน ทำอะไรได้บ้าง ครับ
อย่างเช่น ผมอยากให้ double มัน ยกกำลังได้ ซึ่ง จริงๆแล้ว เราต้องใช้
Code (C#)
System.Math.Pow(2.5, 5.2);
เราก็ลองเขียน extension ประมาณนี้
Code (C#)
public static class Tor_extension
{
public static void Testextensions(this string s)
{
MessageBox.Show(s + " by TOR");
}
public static double Power(this double a, double b)
{
return System.Math.Pow(a, b);
}
}
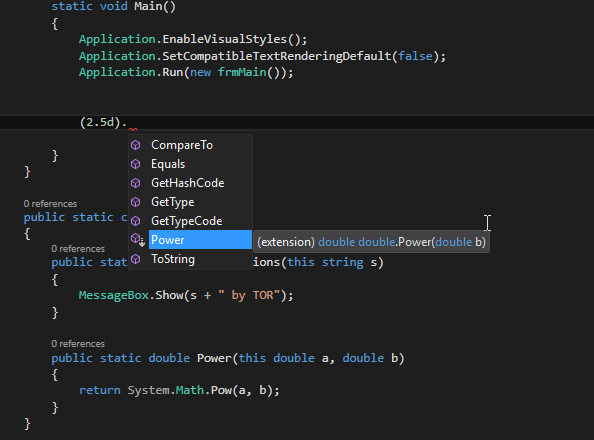
ผมหวังว่าบทความนี้จะเป็นประโยชน์กับคนที่ฝึกเขียนโปรแกรม ไม่มากก็น้อยนะครับ

|
|
|
|
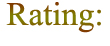 |
|
|
|
By : |
TOR_CHEMISTRY
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
 |
|
Create Date : |
2019-04-29 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|