|
DataGridView / ComboBox (DataGridViewComboBoxColumn) สร้าง DataSource ให้กับ ComboBox (VB.Net , C#) |
DataGridView / ComboBox (DataGridViewComboBoxColumn) สร้าง DataSource ให้กับ ComboBox (VB.Net , C#) ในหัวข้อนี้เราจะมาเรียนรู้วิธีการสร้าง ComboBox ใน DataGridView ของ Windows Form Application ซึ่งโดยปกติทั่วไปแล้ว Column/Rows ใน DataGridView เราจะใช้เป็น Textbox ในหัวข้อนี้เราจะมาเรียนรู้วิธีการสร้าง ComboBox ใน DataGridView ของ Windows Form Application ซึ่งโดยปกติทั่วไปแล้ว Column/Rows ใน DataGridView เราจะใช้เป็น Textbox แต่อาจจะมีข้อมูลบางประเภทที่ไม่ใช่แบบ Input แต่จะเป็น Select List หรือเราเรียกว่า ComboBox / DropDownList สำหรับวิธีการสร้างนั้นสามารถใช้วิธีการสร้าง DataSource แบบ Wizard ผ่าน Tools ของ Visual Studio แล้วนำค่า DataSource ไปกำหนดค่าให้กับ ComboBox ได้เลย หรือจะใช้วิธีการสร้าง ComboBox มาเปล่า ๆ แล้วค่อยไป Bind ข้อมูลให้ทีหลังก็ได้ ในตัวอย่างนี้มีทั้ง VB.Net และ C#
DataGridView / ComboBox (DataGridViewComboBoxColumn) and Default Selected
ตอนที่ 1 : DataGridView (Win Form) สร้าง Custom แบบ Column/Header และการ Summary ผลรวม (VB.Net,C#)
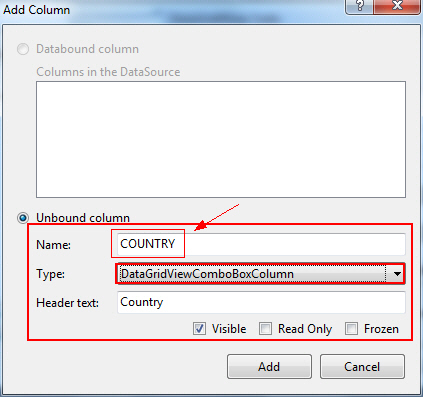
เพิ่ม Column ขึ้นมาใหม่ชื่อว่า Country โดยเลือกเป็นแบบ DataGridViewComboBoxColumn กำหนด Name และ Header text ดังรูป
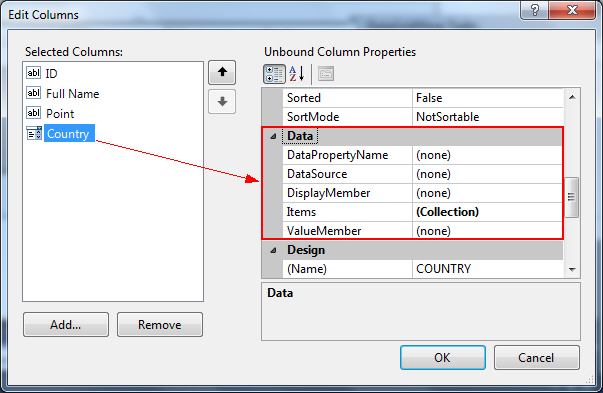
ในกรณีที่เรามี DataSource จาก Wizard พวก DataSet สามารถกำหนดค่า DataSource, ValueMember, DisplayMember และ DataPropertyName ได้เลย ซึ่งตัว DataGridView จะทำการ BindData ข้อมูลและ Default Selected ค่าใน ComboBox ให้อัตโนมัติ แต่ในบทความนี้ผมจะมากำหนดค่ามันทีหลัง และเขียน Code เอง
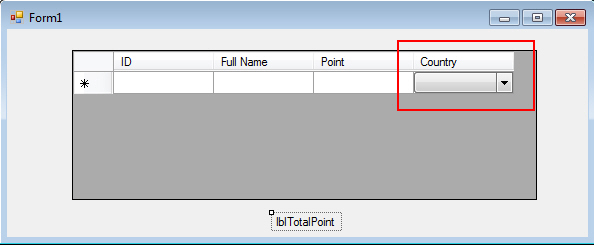
DataGridViewComboBoxColumn ของคอลัมบ์ Country
Code ของ VB.Net
Private Sub Form1_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
'*** Master Data Table ***'
' Create Datable
Dim dtTable As DataTable = New DataTable("myTable")
' Create Column Header
dtTable.Columns.Add(New DataColumn("ColID", GetType(Integer)))
dtTable.Columns.Add(New DataColumn("ColFullName", GetType(String)))
dtTable.Columns.Add(New DataColumn("ColPoint", GetType(Integer)))
dtTable.Columns.Add(New DataColumn("ColCountryCode", GetType(String)))
' Add Rows
dtTable.Rows.Add(1, "Weerachai Nukitram", 10, "TH")
dtTable.Rows.Add(2, "Wisarut Nukitram", 20, "US")
dtTable.Rows.Add(3, "Wipa Nukitram", 30, "TH")
'*** Country Data Table ***'
' Create Datable
Dim dtCountry As DataTable = New DataTable("myCountry")
dtCountry.Columns.Add(New DataColumn("ColCountryCode", GetType(String)))
dtCountry.Columns.Add(New DataColumn("ColCountryName", GetType(String)))
' Add Rows
dtCountry.Rows.Add("TH", "Thailand")
dtCountry.Rows.Add("US", "United States")
' Bind to DataGridView
Me.myDataGridView.AutoGenerateColumns = False
Me.myDataGridView.AllowUserToAddRows = False
Me.myDataGridView.DataSource = dtTable
' Bind ComboBox
Me.COUNTRY.DataPropertyName = "Name"
Me.COUNTRY.DataSource = dtCountry
Me.COUNTRY.ValueMember = "ColCountryCode"
Me.COUNTRY.DisplayMember = "ColCountryName"
' Loop defult selected from Datatable
For Each row As DataGridViewRow In Me.myDataGridView.Rows
Dim sel As DataRow = dtTable.Select("ColID = '" & row.Cells("ID").Value & "' ").FirstOrDefault()
If Not (IsNothing(sel)) Then
row.Cells("COUNTRY").Value = sel.Item("ColCountryCode")
End If
Next
' Summary Total Point
Dim iTotal As Integer
For Each row As DataGridViewRow In Me.myDataGridView.Rows
iTotal = iTotal + row.Cells("POINT").Value
Next
Me.lblTotalPoint.Text = String.Format("Total Point : {0}", iTotal)
End Sub
Code ของ C#
private void Form1_Load(object sender, EventArgs e)
{
//*** Master Data Table ***'
// Create Datable
DataTable dtTable = new DataTable("myTable");
//Create Column Header
dtTable.Columns.Add(new DataColumn("ColID", typeof(int)));
dtTable.Columns.Add(new DataColumn("ColFullName", typeof(string)));
dtTable.Columns.Add(new DataColumn("ColPoint", typeof(int)));
dtTable.Columns.Add(new DataColumn("ColCountryCode", typeof(string)));
// Add Rows
dtTable.Rows.Add(1, "Weerachai Nukitram", 10, "TH");
dtTable.Rows.Add(2, "Wisarut Nukitram", 20, "US");
dtTable.Rows.Add(3, "Wipa Nukitram", 30, "TH");
//*** Country Data Table ***'
// Create Datable
DataTable dtCountry = new DataTable("myCountry");
dtCountry.Columns.Add(new DataColumn("ColCountryCode", typeof(string)));
dtCountry.Columns.Add(new DataColumn("ColCountryName", typeof(string)));
// Add Rows
dtCountry.Rows.Add("TH", "Thailand");
dtCountry.Rows.Add("US", "United States");
// Bind to DataGridView
this.myDataGridView.AutoGenerateColumns = false;
this.myDataGridView.AllowUserToAddRows = false;
this.myDataGridView.DataSource = dtTable;
// Bind ComboBox
this.COUNTRY.DataPropertyName = "Name";
this.COUNTRY.DataSource = dtCountry;
this.COUNTRY.ValueMember = "ColCountryCode";
this.COUNTRY.DisplayMember = "ColCountryName";
// Loop defult selected from Datatable
foreach (DataGridViewRow row in this.myDataGridView.Rows)
{
DataRow sel = dtTable.Select("ColID = '" + row.Cells["ID"].Value.ToString() + "' ").FirstOrDefault();
if (sel != null)
{
row.Cells["COUNTRY"].Value = sel["ColCountryCode"].ToString();
}
}
// Summary Total Point
int iTotal = 0;
foreach (DataGridViewRow row in this.myDataGridView.Rows)
{
iTotal = iTotal + Convert.ToInt32(row.Cells["POINT"].Value);
}
this.lblTotalPoint.Text = String.Format("Total Point : {0}", iTotal);
}
คำอธิบาย
' Bind ComboBox
Me.COUNTRY.DataPropertyName = "Name"
Me.COUNTRY.DataSource = dtCountry
Me.COUNTRY.ValueMember = "ColCountryCode"
Me.COUNTRY.DisplayMember = "ColCountryName"
เป็นการ Bind ข้อมูลของ Country แสดงใน ComboBox โดยอ้างถึงคอลัมบ์ชื่อว่า "COUNTRY"
' Loop defult selected from Datatable
For Each row As DataGridViewRow In Me.myDataGridView.Rows
Dim sel As DataRow = dtTable.Select("ColID = '" & row.Cells("ID").Value & "' ").FirstOrDefault()
If Not (IsNothing(sel)) Then
row.Cells("COUNTRY").Value = sel.Item("ColCountryCode")
End If
Next
เป็นการ Loop ข้อมูลใน DataGridView ของ Column ที่ชื่อว่า ID ซึ่งเป็น Key มีค่าเป็นอะไร แล้วนำ Key ไปค้นหาใน DataTable ว่า Rows นั้น CountryCode มีค่าเป็นอะไร ซึ่งจะนำมาใส่เป็นค่า Default ให้กับ ComboBox
Screenshot
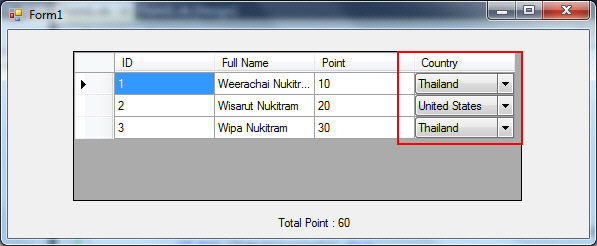
ผลลัพธ์ที่ได้ ComboBox บน DataGridView
.
|
|
|
|
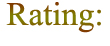 |
|
|
|
By : |
TC Admin
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2015-09-24 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|