|
jQuery กับ Ajax และ MySQL ส่งค่าจาก Form และจัดเก็บลงใน MySQL และการรับ-ส่งด้วย JSON |
jQuery กับ Ajax และ MySQL ส่งค่าจาก Form และจัดเก็บลงใน MySQL และการรับ-ส่งด้วย JSON บทความนี้จะเป็นตัวอย่างการใช้ jQuery กับ Ajax ในการรับค่า Input จาก Form ส่งค่าทั้งหมดด้วยคำคำสั่ง $("#frmMain").serialize() ซึ่งจะสะดวกในการที่จะส่งค่าไปยัง PHP ได้ในครั้งเดียว และหลังจากที่ PHP ได้รับค่าจะสามารถอ่านโดยใช้คำสั่ง $_POST เพื่อนำค่าที่ได้ไป Insert ลงใน MySQL Database และหลังจากที่ Insert ลงใน Database เรียบร้อยแล้วก็จะส่งค่า JSON กลับมายัง jQuery เพื่อให้รู้ว่าสถานะการ Insert เป็นอย่างไร ด้วยการใช้ header('Content-Type: application/json'); และ JSON Object ซึ่งฝั่งของ jQuery จะรู้ทันทีว่าค่าที่ถูกส่งมาเป็น JSON ด้วยการอ่านจาก Object ID นั้นๆ
jQuery กับ Ajax และ MySQL
jQuery กับ Ajax ใช้ Serialize() ส่งค่า Form อ่านค่า JSON (Content-Type: application/json)
เพื่อความเข้าจเกี่ยวกับ jQuery Ajax และ JSON ควรอ่านบทความนี้ให้เข้าใจก่อน
MySQL Table
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
จากนั้นให้เขียน Code ที่เป็น jQuery กับ Ajax และ PHP ดังนี้
post.html
<html>
<head>
<title>ThaiCreate.Com Tutorials</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
</head>
<body>
<form action="" name="frmMain" id="frmMain" method="post">
<table width="284" border="1">
<tr>
<th width="120">CustomerID</th>
<td width="238"><input type="text" name="txtCustomerID" id="txtCustomerID" size="5"></td>
</tr>
<tr>
<th width="120">Name</th>
<td><input type="text" name="txtName" id="txtName" size="20"></td>
</tr>
<tr>
<th width="120">Email</th>
<td><input type="text" name="txtEmail" id="txtEmail" size="20"></td>
</tr>
<tr>
<th width="120">CountryCode</th>
<td><input type="text" name="txtCountryCode" id="txtCountryCode" size="2"></td>
</tr>
<tr>
<th width="120">Budget</th>
<td><input type="text" name="txtBudget" id="txtBudget" size="5"></td>
</tr>
<tr>
<th width="120">Used</th>
<td><input type="text" name="txtUsed" id="txtUsed" size="5"></td>
</tr>
</table>
<br>
<input type="button" name="btnSend" id="btnSend" value="Send">
</form>
<script type="text/javascript">
$(document).ready(function() {
$("#btnSend").click(function() {
$.ajax({
type: "POST",
url: "save.php",
data: $("#frmMain").serialize(),
success: function(result) {
if(result.status == 1) // Success
{
alert(result.message);
}
else // Err
{
alert(result.message);
}
}
});
});
});
</script>
</body>
</html>

save.php
<?php
header('Content-Type: application/json');
$serverName = "localhost";
$userName = "root";
$userPassword = "root";
$dbName = "mydatabase";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "INSERT INTO customer (CustomerID, Name, Email, CountryCode, Budget, Used)
VALUES ('".$_POST["txtCustomerID"]."','".$_POST["txtName"]."','".$_POST["txtEmail"]."'
,'".$_POST["txtCountryCode"]."','".$_POST["txtBudget"]."','".$_POST["txtUsed"]."')";
$query = mysqli_query($conn,$sql);
if($query) {
echo json_encode(array('status' => '1','message'=> 'Record add successfully'));
}
else
{
echo json_encode(array('status' => '0','message'=> 'Error insert data!'));
}
mysqli_close($conn);
?>
คำอธิบาย
เมื่อ PHP รับค่าจาก Ajax จะทำการ Insert ลงใน MySQL Database และมีการ Return ค่าที่เป็น JSON กลับไปว่าทำการ Insert สำเร็จหรือไม่ ถ้าสำเร็จ
สำเร็จ
status = 1
message = Record add successfully
ไม่สำเร็จ
status = 0
message = Error insert data!
เมื่อ jQuery ได้รับค่าจะสามารถอ่านค่า json ได้เลย เช่น result.status และ result.message
ผลลัพธ์ที่ได้
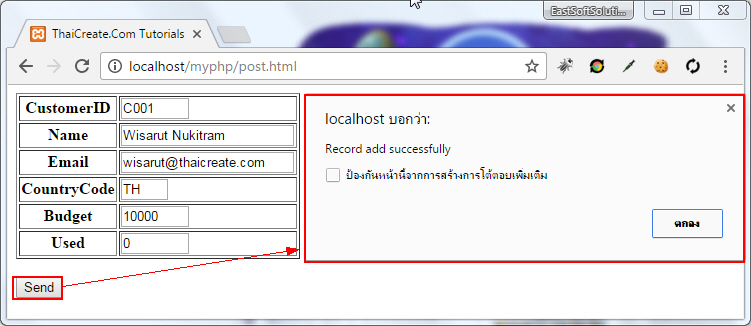
การ Insert ข้อมูลด้วย Ajax

ข้อมูลเข้าสู่ฐานข้อมูล MySQL Database
.
|
|
|
|
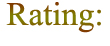 |
|
|
|
By : |
TC Admin
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
  |
|
Create Date : |
2017-02-23 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|