|
Laravel::Raw & Fluent Query Builder มาดูการย่อคำสั่ง Sql ให้เหลือบรรทัดเดียวในสไตน์ ของ Laravel |
Laravel::Raw & Fluent Query Builder มาดูการย่อคำสั่ง Sql ให้เหลือบรรทัดเดียวในสไตน์ ของ Laravel มาต่อกันด้้วยการทำให้การคิวรี้ที่แสนจะยืดยาวแบบเดิม สั้นลงให้เหลือบรรทัดเดียวกัน
RAW QUERIES คือการคิวรีแบบยัด sql ลงไปตรงๆเลยครับ
การ select
Code (PHP)
$users = DB::query('select * from users');
Select โดยผูกข้อมูลเข้าไปด้วย
Code (PHP)
$users = DB::query('select * from users where name = ?', array('test'));
ยัดข้อมูลลง database (binding คือการยัดข้อมูลเข้าไปในฟังชันนะครับ )
Code (PHP)
$success = DB::query('insert into users values (?, ?)', $bindings);
แก้ไขข้อมูล ผูกข้อมูลลงไปด้วย
Code (PHP)
$affected = DB::query('update users set name = ?', $bindings);
ลบข้อมูล
Code (PHP)
$affected = DB::query('delete from users where id = ?', array(1));
รูปแบบต่างๆของการคิวรี่ ตัวอย่างข้างล่างก็เป็นฟังก์ชันมาตรฐานขอ Framework ทั่วไป ในรูปแบบของ laravel ครับ
เลือกข้อมูลแถวแรก
Code (PHP)
$user = DB::first('select * from users where id = 1');
เลือกข้อมูลแถวเดียวกรณีเราเลือกเป็น column
Code (PHP)
$email = DB::only('select email from users where id = 1');
FLUENT QUERY BUILDER
คือการย่อฟังชันต่างๆของ sql ลงมาเหลือแค่ฟังชันเดียว โดยเราต้องเริ่มด้วยการเลือกตารางที่เราต้องการจะคิวรี่ก่อนทุกครั้งนะครับ
Code (PHP)
$query = DB::table('users');
การดึงข้อมูล เหมือน select * from users ครับ
Code (PHP)
$users = DB::table('users')->get();
เลือกแถวแรกของตาราง users ครับ
Code (PHP)
$user = DB::table('users')->first();
เลือกโดยใช้เงื่อนไขคือ id
Code (PHP)
$user = DB::table('users')->find($id);
เห็นไมครับว่าตัวอย่างข้างล่างนี้เข้าใจง่ายมาก เลือกข้อมูลจากตาราง User โดย id = 1 เอาคอลัมน์ email เท่านั้น
Code (PHP)
$email = DB::table('users')->where('id', '=', 1)->only('email');
เลือกเฉพาะคอลัม id กับ EMAIL โดยใส่ asให้มันด้วครับ
Code (PHP)
$user = DB::table('users')->get(array('id', 'email as user_email'));
เลือกข้อมูลที่ไม่ซ้ำขึ้นมาครับ
Code (PHP)
$user = DB::table('users')->distinct()->get();
Building Where Clauses where and or_where เลือกข้อมูลโดยมีเงื่อนไขคือ id = 1 หรือ email = email@[email protected]
Code (PHP)
return DB::table('users')
->where('id', '=', 1)
->or_where('email', '=', '[email protected]')
->first();
เลือกโดย id มากกว่า 1 หรือ name มีคำว่า Taylor อยู่ด้วยครับ
Code (PHP)
return DB::table('users')
->where('id', '>', 1)
->or_where('name', 'LIKE', '%Taylor%')
->first();
Whare = and , or where = or ใน sql นะครับ
where_in, where_not_in, or_where_in, and or_where_not_in
มาเป็นชุดเลยครับ
Code (PHP)
DB::table('users')->where_in('id', array(1, 2, 3))->get();
Where in คือ เงื่อนไขที่กำหนดว่าต้องมีข้อมูลที่เรากำหนดอยู่ด้วย
Code (PHP)
DB::table('users')->where_not_in('id', array(1, 2, 3))->get();
Where not in คือ ต้องไม่มีข้อมูลตรงตามเงื่อนไขที่เรากำหนดครับ
Code (PHP)
DB::table('users')
->where('email', '=', '[email protected]')
->or_where_in('id', array(1, 2, 3))
->get();
Or where in คือ จะมีข้อมูลตรงตามเงื่อนไขที่เรากำหนดหรือจะไม่มีก็ได้
Code (PHP)
DB::table('users')
->where('email', '=', '[email protected]')
->or_where_not_in('id', array(1, 2, 3))
->get();
ตัวอย่างข้างบน เลือกข้อมูลทั้งหมดจากตาราง users โดยเงื่อนไขคือ email = [email protected] หรือ id ต้องไม่มีค่าในอาเรย์
where_null, where_not_null, or_where_null, and or_where_not_null
เป็นชุดในการตรวจหาค่าว่างนะครับ
Code (PHP)
return DB::table('users')->where_null('updated_at')->get();
เลือกข้อมูลจากตาราง users โดยเลือกที่แถวที่คอลัม updated_at เป็นค่าว่าง
Code (PHP)
return DB::table('users')->where_not_null('updated_at')->get();
เหมือนกับข้างบนครับแต่ updated_at ต้องไม่ว่าง
Code (PHP)
return DB::table('users')
->where('email', '=', '[email protected]')
->or_where_null('updated_at')
->get();
เลือกข้อมูลทั้งหมดจากตาราง users โดยเงื่อนไขคือ email = [email protected]
หรือคอลัม updated_at เป็นค่าว่าง
Code (PHP)
return DB::table('users')
->where('email', '=', '[email protected]')
->or_where_not_null('updated_at')
->get();
ลือกข้อมูลทั้งหมดจากตาราง users โดยเงื่อนไขคือ email = [email protected]
หรือคอลัม updated_at ไม่เป็นค่าว่าง
Nested Where Clauses การเลือกโดยเงื่อนไขแบบเป็นช่วงครับ
Code (PHP)
$users = DB::table('users')
->where('id', '=', 1)
->or_where(function($query)
{
$query->where('age', '>', 25);
$query->where('votes', '>', 100);
})
->get();
ตัวอย่างข้างบนจะสร้าง sql ออกมาแบบนี้ครับ
Code (PHP)
SELECT * FROM "users" WHERE "id" = ? OR ("age" > ? AND "votes" > ?)
Dynamic Where Clauses ทำเงื่อนไขที่ยุ่งยากให้ง่ายขึ้นในสไตน์ของ laravel
Code (PHP)
$user = DB::table('users')->where_email('[email protected]')->first();
เลือกแถวแรกโดย email เท่ากับ [email protected]'Code (PHP)
$user = DB::table('users')->where_email_and_password('[email protected]', 'secret')
เห็น ชื่อฟังก์ชันไหมครับ เราตั้งเงื่อนไขโดยใช้สองคอลัมพร้อมกันเลยCode (PHP)
$user = DB::table('users')->where_id_or_name(1, 'Fred');
จับวิธีการคิวรี่ไปใส่ในชื่อของฟังชันเลย สะดวกสั้นไหมละครับ ดูนานๆไปทำให้ผมนึกถึง ruby หละสิ
เชื่อมตาราง การเชื่อมตารางของ laravel ครับ ข้างล่างเราจอยตาราง users เข้ากับ phone โดยใช้ user.id กับ phone.user_idแล้วเลือก คอลัม user.email กับ phone.number มา
Code (PHP)
DB::table('users')
->join('phone', 'users.id', '=', 'phone.user_id')
->get(array('users.email', 'phone.number'));
จะใช้ left join ก็เหมือนกันเลยครับ
DB::table('users')
->left_join('phone', 'users.id', '=', 'phone.user_id')
->get(array('users.email', 'phone.number'));
สามารถ join ได้หลายๆ ครั้งโดยสร้างฟังชันมาซ้อนครับ
Code (PHP)
DB::table('users')
->join('phone', function($join)
{
$join->on('users.id', '=', 'phone.user_id');
$join->or_on('users.id', '=', 'phone.contact_id');
})
->get(array('users.email', 'phone.number'));
การเรียงลำดับผลการค้นหา การเรียงลำดับก็ง่ายๆ เหมือนในตัวอย่างเลยครับ
Code (PHP)
return DB::table('users')->order_by('email', 'desc')->get();
จะเรียงยังไง ได้ตามใจเราเลยครับ
Code (PHP)
return DB::table('users')
->order_by('email', 'desc')
->order_by('name', 'asc')
->get();
Skip & Take Limt ใน sql ใน laravel ใช้ take นะครับ
Code (PHP)
return DB::table('users')->take(10)->get();
ถ้าต้องการเรียง offset ใช้ skip เลยครับ
Code (PHP)
return DB::table('users')->skip(10)->get();
Aggregates
จะใช้ฟังชันในการบวกลบคูณหารที่เราคุ้นเคยใน sql แบบเดิม เราก็ไม่ต้องไปจำใหม่แบบใน framework อื่นๆ เลยครับ laravel เอาใจเราโดยการตั้งชื่อให้
Code (PHP)
$min = DB::table('users')->min('age');
$max = DB::table('users')->max('weight');
$avg = DB::table('users')->avg('salary');
$sum = DB::table('users')->sum('votes');
$count = DB::table('users')->count();
เรายังสามารถจำกัดการคืนค่าโดยใช้ where แบบในตัวอย่างเลยครับ
Code (PHP)
$count = DB::table('users')->where('id', '>', 10)->count();
Expressions บางครั้งเราต้องการใช้ฟังก์ชันสำเร็จรูปใน sql laravel ก็เตรียมมาแล้วครับ
Code (PHP)
DB::table('users')->update(array('updated_at' => DB::raw('NOW()')));
ฟังก์ชัน Raw จะทำการแทรกส่วนที่ต้องใช้ในการคำนวนให้ครับ Code (PHP)
DB::table('users')->update(array('votes' => DB::raw('votes + 1')));
เขาเตรียมให้เราไว้หมดเลยครับสบายจริงๆ อย่างข้างล่างมีฟังก์ชันที่ใช้เพิ่มค่าของ column ให้เลย
Code (PHP)
DB::table('users')->increment('votes');
DB::table('users')->decrement('votes');
การเพิ่มข้อมูล การเพิ่มข้อมูลต้องใส่ลงไปเป็น array นะครับ ค่าที่ส่งกลับมาก็เป็น true หรือ false
Code (PHP)
DB::table('users')->insert(array('email' => '[email protected]'));
ใช้ฟังก์ชัน insert_get_id จะทำการเพิ่มข้อมูลให้กับฟิว id เลยครับ
Code (PHP)
$id = DB::table('users')->insert_get_id(array('email' => '[email protected]'));
การแก้ไขข้อมูล เหมือนกับการเพิ่มเลยครับ เปลี่ยนแค่ insert เป็น update
Code (PHP)
$affected = DB::table('users')->update(array('email' => '[email protected]'));
จะแก้ไข้หลายแถวก็ใช้ where เลยครับ
$affected = DB::table('users')
->where('id', '=', 1)
->update(array('email' => '[email protected]'));
การลบข้อมูล จะลบก็ง่ายๆ ครับเหมือนในตัวอย่างเลย
Code (PHP)
$affected = DB::table('users')->where('id', '=', 1)->delete();
จะให้สั้นได้อีกก็ได้เลยครับ
Code (PHP)
$affected = DB::table('users')->delete(1);
จบแล้วครับบทนี้ ยาวไหมครับ บทหน้าผมจะมาทำให้ ตัวอย่างข้างบนนี้สั้นลงได้อีก โดย Model ของ laravel ที่ชื่อว่า Eloquent(อีโลเคว้น) ชื่ออ่านยากดีจัง นะครับ

|
|
|
|
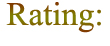 |
|
|
|
By : |
taqman
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2013-05-14 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|