|
Route::In Laravel PHP Framework Part 2 |
Route::In PHP Laravel Framework Part 2 วันนี้มาต่อเรื่องที่ค้างไว้เมื่อวานนะครับ เราจะมาพูดถึงเรื่องการกรอง URL กัน Route ของ Laravel มี ฟังก์ชัน filter ให้ ซึ่งจะมีประโยชน์ในการตรวจสอบสิทธิ์และเพิ่มการจัดการสิทธิ์การเข้าถึง เช่น เมื่อมีการเรียกฟังก์ชัน admin_create เรามาดูตัวอย่างกัน
เริ่มแรกเราก็สร้าง filter ขึ้นมาก่อน
Code (PHP)
Route::filter('filter', function()
{ return Redirect::to('home'); });
ทีนี้เราก็แทรก ไว้ใน route ได้เลย
Code (PHP)
Route::get('blocked', array('before' => 'filter', function()
{ return View::make('blocked'); }));
เราสามารถกำหนดให้ filter ทำงานก่อนหรือหลัง ได้ อย่างในตัวอย่าง เรากำหนดให้เมื่อ มีการเรียก download controller หลังจากนั้นก็เรียก log controller
Code (PHP)
Route::get('download', array('after' => 'log', function()
{ //// }));
เราสามารถแทรกหลายๆ controller ลงไปได้อีก อย่างในตัวอย่าง ก่อนที่จะเรียก create เราจะให้ class auth กับ csrf ทำงานเพื่อตรวจสอบสิทธิ์ และกรองค่าที่ไม่ปลอดภัยก่อน
Code (PHP)
Route::get('create', array('before' => 'auth|csrf', function()
{ // }));
ความพิเศษอีกอย่างคือเราสามารถส่ง parameter ไปพร้อมกับตอนเรียกใช้งาน controller ได้เลย
Code (PHP)
Route::get('panel', array('before' => 'role:admin', function()
{ //// }));
Pattern Filters
บางครั้งเราต้องการตรวจสอบสิทธิ์ของผู้ใช้งาน ก่อนจะเข้าถึง ฟังชันที่เกี่ยวกับการจัดการระบบ
เราต้องกำหนดแบบนี้เลยครับ
Code (PHP)
Route::filter('pattern: admin/*', 'auth');
จะเหมือนตัวอย่างข้างบน ทุกการเรียกใช้ ฟังชันใน controller admin จะถูกดึงเข้า ไปตรวจใน controller auth ก่อน
Code (PHP)
Route::filter('pattern: admin/*', array('name' => 'auth', function()
{ //// }));
Global Filters
เราสามารถสร้างตัวกรองระดับที่ทุกการเรียกใช้จะเข้ามาตรวจใน route นี้ก่อนโดยใน laravel มีตัวอย่างนำมาให้แล้ว ตัวไฟล์จะอยู่ที่ application/routes.php
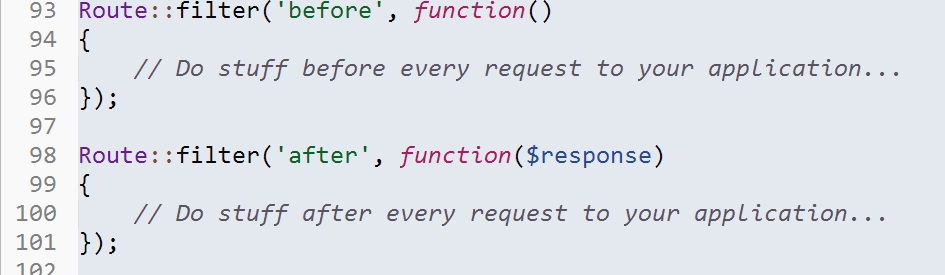
Route Groups
อยากสร้างตัวกรองเป็นแบบกลุ่มก็สร้างได้เลยครับ ลักษณะจะเหมือนจับฟังชันแม่ฟังชันลูก
Code (PHP)
Route::group(array('before' => 'auth'), function()
{
Route::get('panel', function(){});
Route::get('dashboard', function(){});
});
Named Routes
เป็นการสร้างชื่อเล่นให้กับ route ครับ ลักษณะจะเป็นแบบใน MYSQL ที่เราใช้คำว่า as ทำให้ชื่อ filed สั้นลง ใน larval ก็เช่นเดียวกัน เมื่อเราเรียกใช้ home ก็จะลิ้งไปยัง admin/index นะครับ
สร้าง named route:
Code (PHP)
Route::get('admin/index', array('as' => 'home', function()
{
return "Hello World";
}));
สร้างลิ้งโดยใช้ named route ที่เราสร้างข้างบน
Code (PHP)
$url = URL::to_route('home');
สร้างการส่งกลับโดยใช้ลิ้งที่เราสร้าง
Code (PHP)
return Redirect::to_route('home');
บางครั้งเราก็ต้องการตรวจสอบที่มาของรีเควสก็ตรวจได้ง่ายๆแบบในตัวอย่างเลยครับ
Code (PHP)
if (Request::route()->is('home'))
{
// The "home" route is handling the request!
}
HTTPS Routes
บางครั้งเราต้องใช้ https ก็สามารถใส่ option เสริมเข้าไปแบบในตัวอย่างได้เลยครับ
Code (PHP)
Route::get('login', array('https' => true, function()
{return View::make('login');}));
หรือจะใช้ฟังก์ชัน secure ของ route ได้เลยครับ
Code (PHP)
Route::secure('GET', 'login', function()
{
return View::make('login');
});
Bundle Routes
Bundle คือส่วนขยายของ laravel นั้นเองครับ ในตัวอย่างนี้เราจะกำหนดค่า url ให้ bundle นะครับ ไฟล์ที่จะแก้นั้นอยู่ที่
applications/bundle.php
Code (PHP)
return array(
'admin' => array('handles' => 'admin'),
);
Handles จะบอกให้ laravel โหลด bundle ชื่อว่า admin ขึ้นมาเมื่อเรียกใช้ admin
แล้วเราก็มาสร้าง route ให้กับ bundle ที่ไฟล์ route.php เลยครับ
Code (PHP)
Route::get('(:bundle)', function()
{
return 'Welcome to the Admin bundle!';
});
Controller Routing
ใน laravel นั้นเราต้องสร้าง route ให้ controller ด้วยนะครับ ไม่สร้างแปลว่า ไม่อนุญาตให้เข้าถึง controller นั้น ตัวอย่างข้างล่างเป็นการสร้าง Route ให้ controller ทั้งหมด
Code (PHP)
Route::controller(Controller::detect());
Controller::detect จะส่งกลับค่า controller ทั้ง app เลย
สร้าง Route ให้ controller ใน bundle
Code (PHP)
Route::controller(Controller::detect('admin'));
สร้าง Route ให้ method
Code (PHP)
Route::get('welcome', 'home@index');
เก็บ log ด้วยเลยก็ได้
Code (PHP)
Route::get('welcome', array('after' => 'log', 'uses' => 'home@index'));
สร้างชื่อเล่นให้ method index ในcontroller home ให้ชื่อเล่นว่า home.welcome เมื่ิอมีการเรียก parameter welcome
Code (PHP)
Route::get('welcome', array('as' => 'home.welcome', 'uses' => 'home@index'));
CLI Route Testing
เราสามารถทดสอบ Route ที่เราสร้างผ่าน command line ได้ผ่าน คำสั่ง artisan เลยผลที่ออกมาจะเหมือน ใช้คำสั่ง var_dump
Code (PHP)
php artisan route:call get api/user/1
|
|
|
|
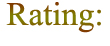 |
|
|
|
By : |
taqman
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
 |
|
Create Date : |
2013-05-09 |
|
Download : |
No files |
|
|
|
|