|
โค้ดสำหรับโหลดไฟล์จากเว็บ one2up แบบบ้านๆ |
โค้ดสำหรับโหลดไฟล์จากเว็บ one2up แบบบ้านๆ
สืบเนื่องจากที่ผมได้งมศึกษาเกี่ยวกับ RegularExpressions มานานพอสมควร
และต้องขอขอบคุณ
http://regexhero.net/tester/
หลังจากที่ลองผิดลองถูกอยู่หลายวันก็เริ่มคล่อง
ถึงเวลาที่จะต้องเอามาใช้งานกันซักที
พอดีที่แล็บจะจัดเลี้ยงปีใหม่วันที่ 16/01/2558(น่าจะเรียกจัดเลี้ยงปีเก่าคงเหมาะ)
ผมจึงต้องค้นหาเพลง และ คาราโอเกะ เพื่อเปิดในงาน
เว็บที่ผมชอบมากอีกเว็บก็คือ
http://www.one2up.com/
โหลดฟรีโหลดง่ายใช่คล่องดี
แต่เสียอย่างเดียวเวลาโหลดมาต้องมานั่งเปลี่ยนชื่อใหม่
ผมจึงคิดว่าน่าจะมีโปรแกรม getlink rename ให้เรียบร้อยก่อนโหลด
ว่าดังนั้นจึงลองวิชาเกี่ยวกับ RegularExpressions ซะเลย
ขั้นตอนแบบบ้านๆมีดังนี้ครับ
1.getHTML ออกมาก่อน
2.getcontent_ID ,getfilename ออกมา
3.เอา URL content_ID มา getlink แบบนี้
4.ส่งให้ IDM โหลดมันโลด
มีแค่นี้ครับ
getHTML
string getHTML(string url)
{
string html = "";
try
{
System.Net.HttpWebRequest request = (System.Net.HttpWebRequest)System.Net.HttpWebRequest.Create(url.Trim());
System.Net.HttpWebResponse response = (System.Net.HttpWebResponse)request.GetResponse();
System.IO.StreamReader sr = new System.IO.StreamReader(response.GetResponseStream());
html = sr.ReadToEnd();
sr.Close();
response.Close();
}
catch { }
return html;
}
ต่อมาก็ getcontent_ID ,getfilename
Code (C#)
System.Text.RegularExpressions.Regex regexListcontent_ID = new System.Text.RegularExpressions.Regex(@"(?:<div.*?(view_content.php\?content_ID=[0-9]+)\"".*?<b>(.*?)<.*?ขนาดไฟล์(.*?)<br>)", System.Text.RegularExpressions.RegexOptions.Singleline | System.Text.RegularExpressions.RegexOptions.CultureInvariant);
void getListHTML(string strsearch, int _min, int _max)
{
for (int i = _min; i <= _max; i++)
{
getListHTML(new object[] { "http://www.one2up.com/list_content.php?page="+i+"&txt_Search="+strsearch+"&radio_type=content_Name" });
System.Threading.Thread.Sleep(100);
}
}
void getListHTML(string URL)
{
getListHTML(new object[] { URL });
}
void getListHTML(object obj)
{
string url = (string)((object[])obj)[0];
this.Invoke(new Action(() => { this.Text = url; }));
foreach (System.Text.RegularExpressions.Match match in regexListcontent_ID.Matches(getHTML(url)))
{
dataGridView1.Invoke(new Action(() => { dataGridView1.Rows.Add(true, "http://www.one2up.com/" + match.Groups[1].Value, match.Groups[2].Value, match.Groups[3].Value, GetLinkByID("http://www.one2up.com/" + match.Groups[1].Value)); }));
System.Threading.Thread.Sleep(100);
}
}
ต่อมาก็ getlink
Code (C#)
System.Text.RegularExpressions.Regex regexLinkFile = new System.Text.RegularExpressions.Regex("(?:\"txt_URL\" id=\"txt_URL\" value)=[\"|']?(.*?)[\"|'|>]", System.Text.RegularExpressions.RegexOptions.Singleline | System.Text.RegularExpressions.RegexOptions.CultureInvariant);
string GetLinkByID(string URL_ID)
{
return (URL_ID == null || URL_ID == string.Empty) ? "" : regexLinkFile.Matches(getHTML(URL_ID))[0].Groups[1].Value;
}
ส่วน IDM command line
https://www.internetdownloadmanager.com/support/command_line.html
ทีนี้ก็ออกแบบหน้าตาแบบบ้านๆมาใช้งาน
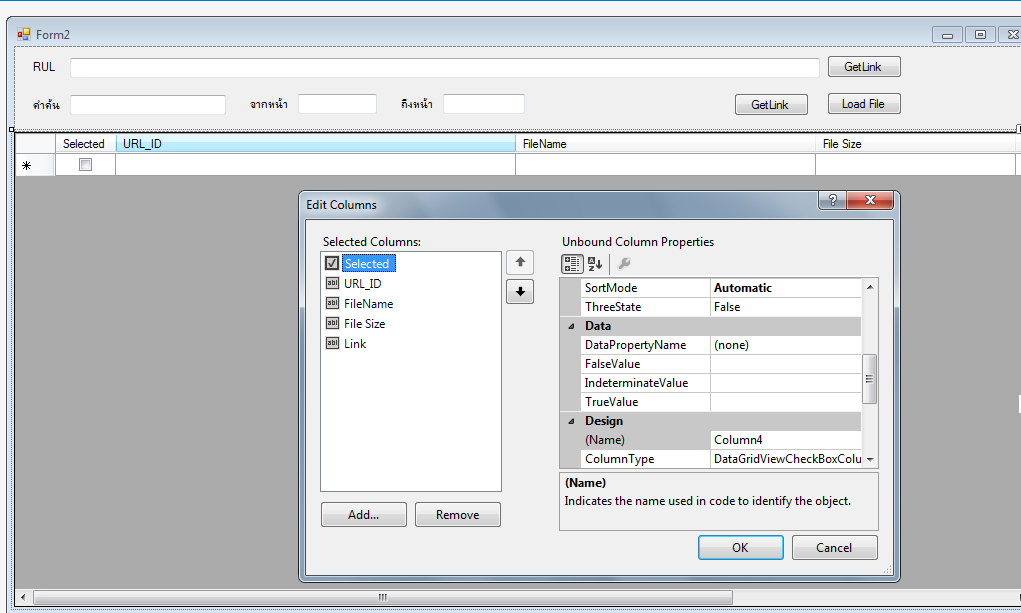
ส่วนโค้ดบ้านๆทั้งหมดก็ประมาณนี้ครับ
Code (C#)
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System;
namespace LoadOne2UpIDM
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
System.Text.RegularExpressions.Regex regexListcontent_ID = new System.Text.RegularExpressions.Regex(@"(?:<div.*?(view_content.php\?content_ID=[0-9]+)\"".*?<b>(.*?)<.*?ขนาดไฟล์(.*?)<br>)", System.Text.RegularExpressions.RegexOptions.Singleline | System.Text.RegularExpressions.RegexOptions.CultureInvariant);
System.Text.RegularExpressions.Regex regexLinkFile = new System.Text.RegularExpressions.Regex("(?:\"txt_URL\" id=\"txt_URL\" value)=[\"|']?(.*?)[\"|'|>]", System.Text.RegularExpressions.RegexOptions.Singleline | System.Text.RegularExpressions.RegexOptions.CultureInvariant);
System.Text.RegularExpressions.Regex regexFileName = new System.Text.RegularExpressions.Regex("(?:content=\"Other->)(.*?)[\"|'|>|/]+", System.Text.RegularExpressions.RegexOptions.Singleline | System.Text.RegularExpressions.RegexOptions.CultureInvariant);
string pathIDM = @"C:\Program Files\Internet Download Manager\IDMan.exe";
string getHTML(string url)
{
string html = "";
try
{
System.Net.HttpWebRequest request = (System.Net.HttpWebRequest)System.Net.HttpWebRequest.Create(url.Trim());
System.Net.HttpWebResponse response = (System.Net.HttpWebResponse)request.GetResponse();
System.IO.StreamReader sr = new System.IO.StreamReader(response.GetResponseStream());
html = sr.ReadToEnd();
sr.Close();
response.Close();
}
catch { }
return html;
}
void getListHTML(string strsearch, int _min, int _max)
{
for (int i = _min; i <= _max; i++)
{
getListHTML(new object[] { "http://www.one2up.com/list_content.php?page="+i+"&txt_Search="+strsearch+"&radio_type=content_Name" });
System.Threading.Thread.Sleep(100);
}
}
void getListHTML(string URL)
{
getListHTML(new object[] { URL });
}
void getListHTML(object obj)
{
string url = (string)((object[])obj)[0];
this.Invoke(new Action(() => { this.Text = url; }));
foreach (System.Text.RegularExpressions.Match match in regexListcontent_ID.Matches(getHTML(url)))
{
dataGridView1.Invoke(new Action(() => { dataGridView1.Rows.Add(true, "http://www.one2up.com/" + match.Groups[1].Value, match.Groups[2].Value, match.Groups[3].Value, GetLinkByID("http://www.one2up.com/" + match.Groups[1].Value)); }));
System.Threading.Thread.Sleep(100);
}
}
string GetLinkByID(string URL_ID)
{
return (URL_ID == null || URL_ID == string.Empty) ? "" : regexLinkFile.Matches(getHTML(URL_ID))[0].Groups[1].Value;
}
void LoadOne2UpByID()
{
for (int i = 0; i < dataGridView1.RowCount - 1; i++)
{
if ((bool)dataGridView1[0, i].Value == true && dataGridView1[2, i].Value.ToString() != "xxx")
{
System.Diagnostics.Process.Start(pathIDM, "/a /n /d " + dataGridView1[4, i].Value.ToString() + " /f \"" + dataGridView1[2, i].Value.ToString() + "\"");
System.Threading.Thread.Sleep(200);
}
}
}
private void button1_Click(object sender, EventArgs e)
{
//โหลดจาก URL
getListHTML(textBox1.Text);
}
private void button2_Click(object sender, EventArgs e)
{
//ให้โหลดจาก คำค้น แบบระบุหน้า ถ้ามีหน้าเดียว ก็ 1 ถึง 1 ประมาณนี้ครับgetListHTML(textBox2.Text,int.Parse(textBox3.Text),int.Parse(textBox4.Text));
}
private void button3_Click(object sender, EventArgs e)
{
//สั่งโหลดจาก dataGridView1 ที่ slected ไว้
new System.Threading.Thread(LoadOne2UpByID).Start();
}
}
}
เอา Threading ออกมาใช้เพื่อไม่ให้สะดุดเวลาทำงานครับ
ทำแบบนี้เราก็ GetLink,Filename มาลงใน dataGridView แล้วก็เลือกได้ว่าจะเอาหรือไม่เอาไฟล์ไหน
ก่อนสั่งโหลดไงละครับ
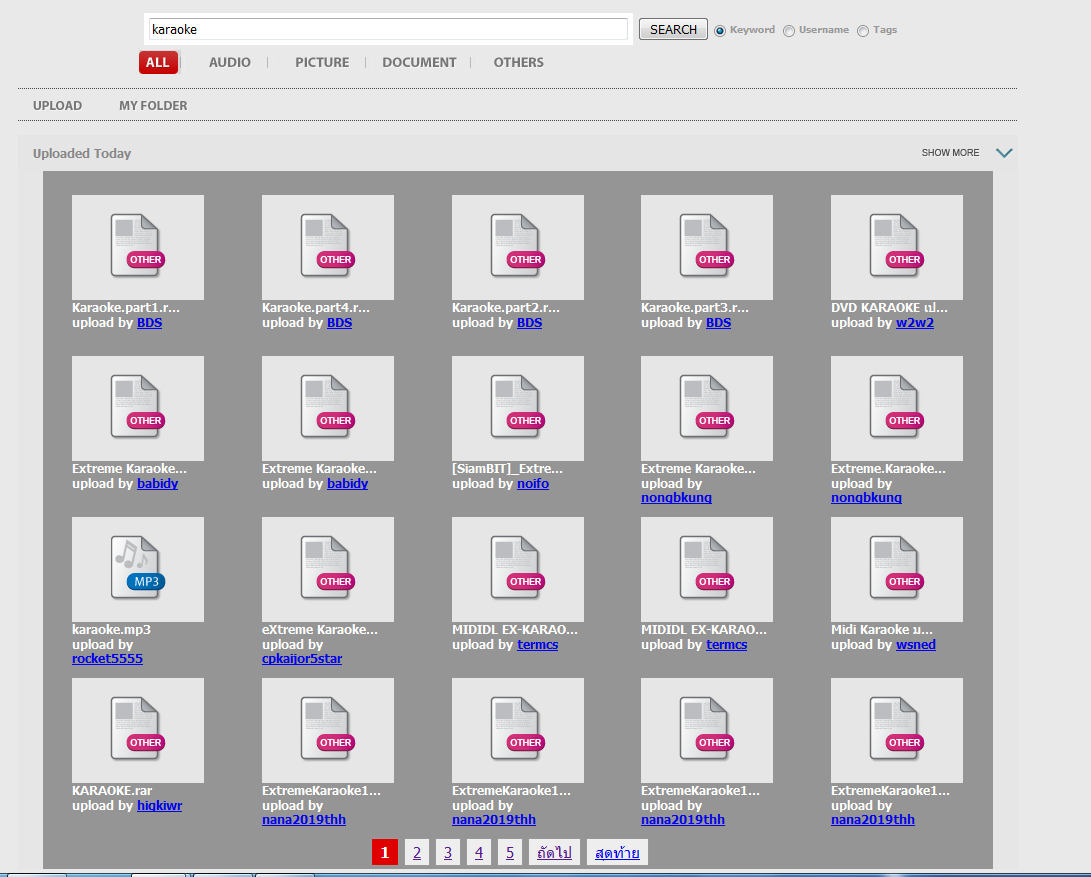
ตัวอย่างผมค้นหา karaoke มีซัก 5 หน้าได้ครับ
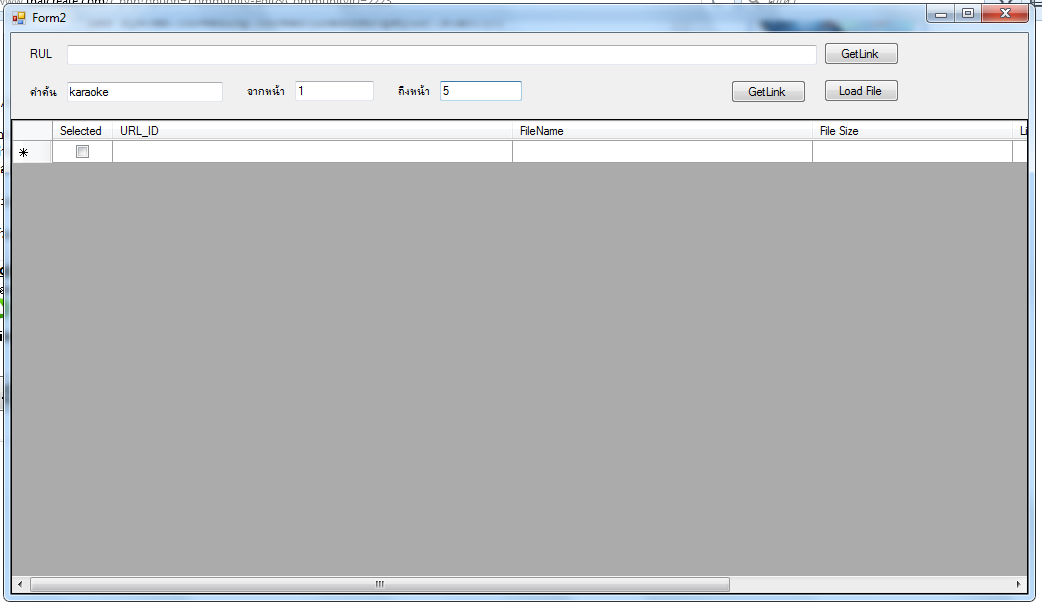
คลิกที่ getlink(button2_Click) แล้วก็รอจนกว่าจะนำข้อมูลเข้าใน dataGridView1 เสร็จ
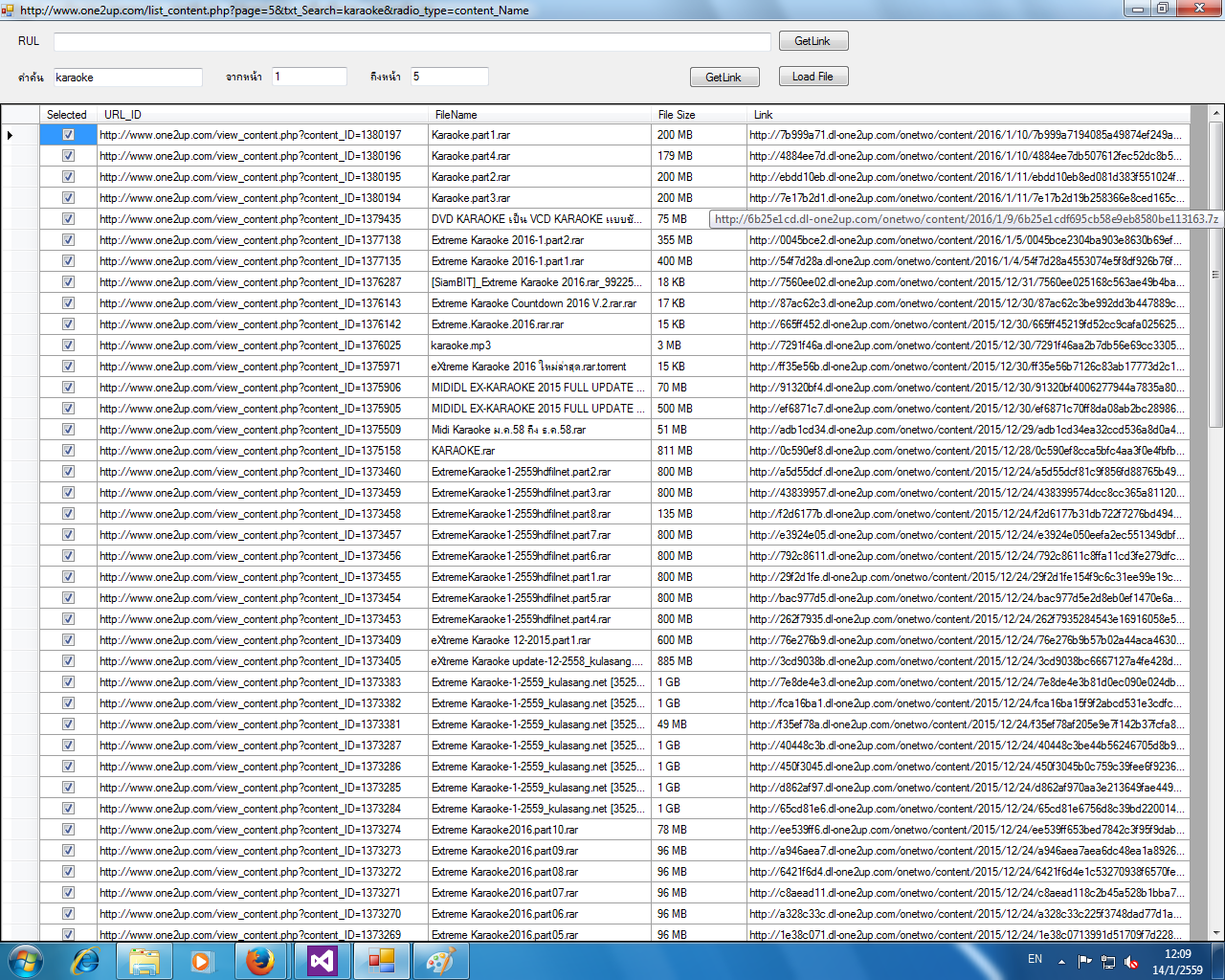
จากนั้นก็เลือกเอาไฟล์ที่ต้องการรึไม่ก็เลือกหมดซะเลย(โลภ)
เสรจแล้วก็คลิก Load File(button3_Click)
แค่นี้ก็เป็นอันเสร็จพิธี ที่เหลือก็แค่เอาออกมาตัดเพลงซ้ำซึ่งผมเขียนไว้นานแล้ว
โค้ดสำหรับโหลดไฟล์จากเว็บ one2up แบบบ้านๆ ก็จบลงเพียงเท่านี้ครับ
จากความรู้และสมองแบบบ้านๆทำให้ได้โค้ดแบบบ้านมาใช้งานครับ

|
|
|
|
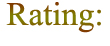 |
|
|
|
By : |
TOR_CHEMISTRY
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2016-01-14 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|