|
PHP Image Effect Class - php ย่อรูปตามความกว้างเป็นหลัก , ย่อตามความสูงเป็นหลัก |
PHP Upload images resize-effect class php ย่อรูปตามความกว้างเป็นหลัก , ย่อตามความสูงเป็นหลัก , ย่อตามความสูงเป็นหลักโดยไม่เกินความกว้างที่กำหนด , ครอปรูป เอฟเฟครูป (การหมุนรูป , การใส่ลายน้ำ , การใส่เอฟเฟคเหมือน photoshop เช่น GRAYSCALE,NEGATE, EDGEDETECT,EMBOSS,GAUSSIAN,SELECTIVE, MEAN_REMOVAL, BRIGHTNESS ,CONTRAST, PENCIL, CRAYON)
ตัว class นี้สามารถเอาไปประยุกต์ใช้ได้ฟรีๆครับ เอาไปปรับแต่ง ได้ตามสบาย อาจจะยังไม่ดีที่สุด แต่ก็สามารถใช้งานได้ดีในระดับนึง หาก ไม่ถึกใจรูปแบบการส่งค่าตัวแปร หรือฟังก์ ชั่นยังไม่ถูกใจก็แก้ได้ตามสบายครับ เอาไปพัฒนาเพิ่มเติมได้เลย
คลาสนี้รองรับรูป .jpg ,.gif ,.png เท่านั้นครับ
Code
<?
/*
*Ex:
<?php
if( $_POST['upload']=='Upload' ) {
$image = new ImageAffect($_FILES['phhoto']['tmp_name']);
$image->resizeToWidth(150);
$my_image=$image->save('resize/test');
echo "<img src=' $my_image' >";
} else {
?>
<form action="" name="frm-photo" method="post" enctype="multipart/form-data">
<input type="file" name="phhoto" id="phhoto" />
<input type="submit" name="upload" value="Upload" />
</form>
<?php
}
?>
*
*/
ini_set('memory_limit', '512M');
set_time_limit ( 45 );
class ImageAffect {
private $image;
private $new_height;
private $new_width;
private $new_image;
private $image_height;
private $image_width;
private $image_type;
private $cropX=0;
private $cropY=0;
private $arrIsImg=array(".jpg",".jpeg",".gif",".png");
private $load_error=false;
private $error_status="";
private $phpVersion=5;
private $rotateAngle=0;
private $imAffect=array(
"ROTATE"=>array(false,"Angle"=>0),
"GRAY"=>false,
"NEGATE"=>false,
"EDGEDETECT"=>false,
"EMBOSS"=>false,
"GAUSSIAN"=>false,
"SELECTIVE"=>false,
"MEAN_REMOVAL"=>false,
"BRIGHTNESS"=>false,
"CONTRAST"=>false,
"PENCIL"=>false,
"CRAYON"=>false,
"EMBOSS2"=>false,
"SHARPEN"=>false,
"BLUR"=>false,
"UNSHAPE_MASK"=>false,
"COLOR"=>array(true,"r"=>0,"g"=>0,"b"=>0)
);
//Auto load for php >= 5
function __construct($filename) {
$this->phpVersion=(float)phpversion();
$this->load($filename);
}
//Auto load for php <5
function ImageAffect($filename){
$this->phpVersion=(float)phpversion();
$this->load($filename);
}
/*
Load image
parram: (string)filename
return : boolean
*/
public function load($filename="") {
if(file_exists($filename)){
$image_info = getimagesize($filename);
$this->getImgType($image_info);
#$this->image_type = ".".strtolower(end(explode(".",$filename)));
if(in_array($this->image_type,$this->arrIsImg)){
if( $this->image_type == ".jpg" || $this->image_type==".jpeg") {
$this->image = imagecreatefromjpeg($filename);
}elseif( $this->image_type == ".gif" ) {
$this->image = imagecreatefromgif($filename);
}elseif( $this->image_type == ".png") {
$this->image = imagecreatefrompng($filename);
}elseif( $this->image_type == ".bmp") {
$this->image = @imagecreatefromwbmp($filename);
}else{
$this->image = imagecreatefromjpeg($filename);
}
}else{
$this->error_status = "File: $filename is not image jpg | gif | png | bmp";
$this->load_error=true;
return false;
exit;
}
if($this->image){
$this->error_status="";
$this->load_error=false;
return true;
}else{
$this->error_status="File: $filename is create failed";
$this->load_error=true;
return false;
}
}else{
$this->error_status = "File: $filename is not found";
$this->load_error=true;
return false;
}
}
/*
* Save image
* param :
(string)filename =save image name
(string)image_type = save image type (.jpg or .gif or .png or .bmp)
(int)$compression
(int)$permissions = permission directory (null or 775)
return error text or file name
*/
public function save($filename="", $image_type="", $compression=75, $permissions=null) {
if($this->load_error!=false){return $this->error_status; exit;}
if($image_type==""){$image_type=$this->image_type;}
if(strpos($filename,".")==0){$filename.=$this->image_type;}
if( $image_type == ".jpg" || $this->image_type==".jpeg") {
imagejpeg($this->image,$filename,$compression);
}elseif( $image_type == ".gif" ) {
imagegif($this->image,$filename);
}elseif( $image_type == ".png") {
imagepng($this->image,$filename);
}elseif( $image_type == ".bmp") {
imagewbmp($this->image,$filename);
}
if( $permissions != null) {
chmod($filename,$permissions);
}
@imagedestroy($this->image);
@imagedestroy($this->new_image);
return $filename;
}
public function output($image_type="") {
if($this->load_error!=false){return $this->error_status; exit;}
if($image_type==""){$image_type=$this->image_type;}
if( $image_type == ".jpg" || $this->image_type==".jpeg") {
imagejpeg($this->image);
} elseif( $image_type == ".gif" ) {
imagegif($this->image);
} elseif( $image_type == ".png") {
imagepng($this->image);
}elseif( $image_type == ".bmp") {
imagewbmp($this->image);
}
}
private function getImgType($img_info=array()){
if($img_info[2]==1){
$this->image_type =".gif";
}elseif($img_info[2]==2){
$this->image_type =".jpg";
}elseif($img_info[2]==3){
$this->image_type =".png";
}elseif($img_info[2]==4){
$this->image_type =".swf";
}elseif($img_info[2]==5){
$this->image_type =".psd";
}elseif($img_info[2]==6){
$this->image_type =".bmp";
}elseif($img_info[2]==15){
$this->image_type =".bmp";
}else{
$this->image_type ="";
}
}
function getWidth() {
return imagesx($this->image);
}
function getHeight() {
return imagesy($this->image);
}
public function resizeToHeight($height=100) {
if($this->load_error!=false){return $this->error_status; exit;}
$ratio = $height / $this->getHeight();
$width = $this->getWidth() * $ratio;
$this->resize($width,$height);
}
public function resizeToWidth($width=100) {
if($this->load_error!=false){return $this->error_status; exit;}
$ratio = (float)$width / $this->getWidth();
$height = $this->getheight() * $ratio;
$this->resize($width,$height);
}
public function resizeCrop($width=100,$height=100){
if($this->load_error!=false){return $this->error_status; exit;}
$size[0]=$this->getWidth();
$size[1]=$this->getHeight();
$thumb_w=$width;
$thumb_h=round($width*$size[1]/$size[0]);
if($thumb_h<$height){
$tmp_h=$height-$thumb_h;
#resize
$thumb_w =$thumb_w + $tmp_h;
$thumb_h=$thumb_h + $tmp_h;
#crop
$this->cropX=$tmp_h;
$this->cropY=0;
#echo "save1 ($thumb_w x $thumb_h) resze (".($thumb_w + $tmp_h )." x ".($thumb_h + $tmp_h).") crop ($cropX x $cropY)";
}elseif($thumb_h>$height){
$tmp_h=$thumb_h-$height;
#crop
$this->cropX=0;
$this->cropY=$tmp_h;
#echo "save2 ($thumb_w x $thumb_h) crop ($cropX x $cropY)";
}else{
$this->cropX=0;
$this->cropY=0;
#echo "save3 ($thumb_w x $thumb_h) => (".($thumb_w + $tmp_h )." x ".($thumb_h + $tmp_h).")";
}
$this->new_image = imagecreatetruecolor($width, $height);
if($this->image_type==".png" or $this->image_type==".gif"){
imagealphablending($this->new_image, false);
imagesavealpha($this->new_image,true);
$transparent = imagecolorallocatealpha($this->new_image, 255, 255, 255, 127);
imagefilledrectangle($this->new_image, 0, 0, $thumb_w, $thumb_h, $transparent);
}
imagecopyresampled($this->new_image, $this->image, 0, 0, $this->cropX, $this->cropY, $thumb_w, $thumb_h, $this->getWidth(), $this->getHeight());
$this->image = $this->new_image;
}
/*
param: scale = %
*/
public function scale($scale=50) {
if($this->load_error!=false){return $this->error_status; exit;}
$width = $this->getWidth() * $scale/100;
$height = $this->getheight() * $scale/100;
$this->resize($width,$height);
}
function resize($width=100,$height=100) {
if($this->load_error!=false){return $this->error_status; exit;}
$this->new_image = imagecreatetruecolor($width, $height);
if($this->image_type==".png" or $this->image_type==".gif"){
imagealphablending($this->new_image, false);
imagesavealpha($this->new_image,true);
$transparent = imagecolorallocatealpha($this->new_image, 255, 255, 255, 127);
imagefilledrectangle($this->new_image, 0, 0, $width, $height, $transparent);
}
imagecopyresampled($this->new_image, $this->image, 0, 0, $this->cropX, $this->cropY, $width, $height, $this->getWidth(), $this->getHeight());
$this->image = $this->new_image;
}
private function array_flatten($array) {
(array)$tempArray = array();
foreach ( $array as $value ) {
if ( is_array($value) ) {
$tempArray = array_merge($tempArray, $this->array_flatten($value));
} else {
$tempArray[] = $value;
}
}
return $tempArray;
}
// Creates the divisor value dynamically, and passes offset
private function makeFilter($resource, $matrix, $offset=1) {
global $$resource;
(float)$divisor = array_sum($this->array_flatten($matrix));
return imageconvolution($resource, $matrix, $divisor, $offset) ? true : false;
}
public function rotateImage($rotateAngle=0){
if((int)$rotateAngle>=-360 && (int)$rotateAngle<=360){
$this->image = imagerotate($this->image, (int)$rotateAngle, -1);
imagealphablending($this->image, true);
imagesavealpha($this->image, true);
}
}
public function setEffect($imAffect=array()){
if($this->phpVersion>=5){
if($this->imAffect["GRAY"]==true){
imagefilter($this->image, IMG_FILTER_GRAYSCALE);
}elseif($imAffect["COLOR"]==true){
imagefilter($this->image, IMG_FILTER_COLORIZE, $imAffect["color"]["r"], $imAffect["color"]["g"], $imAffect["color"]["b"]);
}elseif($imAffect["NEGATE"]==true){
imagefilter($this->image,IMG_FILTER_NEGATE);
}elseif($imAffect["EDGEDETECT"]==true){
imagefilter($this->image,IMG_FILTER_EDGEDETECT);
}elseif($imAffect["EMBOSS"]==true){
imagefilter($this->image,IMG_FILTER_EMBOSS);
}elseif($imAffect["GAUSSIAN"]==true){
imagefilter($this->image,IMG_FILTER_GAUSSIAN_BLUR);
}elseif($imAffect["SELECTIVE"]==true){
imagefilter($this->image,IMG_FILTER_SELECTIVE_BLUR);
}elseif($imAffect["MEAN_REMOVAL"]==true){
imagefilter($this->image,IMG_FILTER_MEAN_REMOVAL);
}elseif($imAffect["BRIGHTNESS"]==true){
imagefilter($this->image, IMG_FILTER_BRIGHTNESS, 20);// ระบุเปอร์เซ็นต์การลดความเข้มของรูป
}elseif($imAffect["CONTRAST"]==true){
imagefilter($this->image,IMG_FILTER_CONTRAST,30); // ระบุเปอร์เซ็นต์การลดความสว่างของรูป
}elseif($imAffect["PENCIL"]==true){
$matrix = array(array(-4.4, -1, -3.7), array(-2,22,-2), array(-2.9, -0.6, -2.9));
imageconvolution($this->image, $matrix, 1, 10); //imageconvolution($src, $filter, $filter_div, $offset);
}elseif($imAffect["CRAYON"]==true){
$matrix = array(array(-4.4, -4, -3.7), array(-2,22,-2), array(-2.9, -0.4, -2.9));
imageconvolution($this->image, $matrix, 1, 10);
}elseif($imAffect["EMBOSS2"]==true){
$matrix = array(array(-3.4, 20, -2.9), array(-2,17,-2.9), array(-1.9, -20, -3.2));
imageconvolution($image, $matrix, 10, 50);
}elseif($imAffect["SHARPEN"]==true){
$matrix = array( array(-1,-1,-1),
array(-1,16,-1),
array(-1,-1,-1) );
$this->makeFilter($this->image, $matrix);
}elseif($imAffect["BLUR"]==true){
$matrix = array( array(-10,-1,-10),
array(-10,-1,-10),
array(-10,-1,-10) );
$this->makeFilter($this->image, $matrix);
}elseif($imAffect["UNSHAPE_MASK"]==true){
$matrix = array( array(0,-10,0),
array(0,-100,0),
array(0,70,0) );
$this->makeFilter($this->image, $matrix);
}
}else{
if($imAffect["GRAY"]==true){
$imgw = imagesx($this->image);
$imgh = imagesy($this->image);
for ($i=0; $i<$imgw; $i++){
for ($j=0; $j<$imgh; $j++){
// get the rgb value for current pixel
$rgb = imagecolorat($this->image, $i, $j);
// extract each value for r, g, b
$rr = ($rgb >> 16) & 0xFF;
$gg = ($rgb >> 8) & 0xFF;
$bb = $rgb & 0xFF;
// get the Value from the RGB value
$g = round(($rr + $gg + $bb) / 3);
// grayscale values have r=g=b=g
$val = imagecolorallocate($this->image, $g, $g, $g);
// set the gray value
imagesetpixel ($this->image, $i, $j, $val);
}
}
}elseif($imAffect["COLOR"]==true){
#
}elseif($imAffect["NEGATE"]==true){
#
}elseif($imAffect["EDGEDETECT"]==true){
#
}elseif($imAffect["EMBOSS"]==true){
#
}elseif($imAffect["GAUSSIAN"]==true){
#
}elseif($imAffect["SELECTIVE"]==true){
#
}elseif($imAffect["MEAN_REMOVAL"]==true){
#
}elseif($imAffect["BRIGHTNESS"]==true){
#
}elseif($imAffect["CONTRAST"]==true){
#
}elseif($imAffect["PENCIL"]==true){
#
}elseif($imAffect["CRAYON"]==true){
#
}elseif($imAffect["EMBOSS2"]==true){
#
}elseif($imAffect["SHARPEN"]==true){
#
}elseif($imAffect["BLUR"]==true){
#
}elseif($imAffect["UNSHAPE_MASK"]==true){
#
}
}
}
public function watermark($watermarkfile, $posx="0", $posy="0") {
if (file_exists($watermarkfile)) {
$wmsize = getimagesize($watermarkfile);
if ($wmsize != false) {
$width = $wmsize[0];
$height = $wmsize[1];
$imgtype_xxx = $wmsize[2];// convert from number(xxx) to ext by image_type_to_extension($imgtype_xxx)
// value of xxx 1=gif/2=jpeg/3=png/4=swf/5=psd/6=bmp/7=tiff /8=tiff?/9=jpc/10=jp2/11=jpx/12=jb2/13=swf/14=iff/15=bmp/16=xbm/
$mime = $wmsize['mime'];// eg. image/gif, image/jpeg
}
if ("1" == $imgtype_xxx) {
// gif
$watermark_read = imagecreatefromgif($watermarkfile);
imagecopy($this->image, $watermark_read, $posx, $posy, 0, 0, $width, $height);
//imagesavealpha($this->new_image, true);
} elseif ($imgtype_xxx == "2") {
// jpeg
$watermark_read = imagecreatefromjpeg($watermarkfile);
imagecopy($this->image, $watermark_read, $posx, $posy, 0, 0, $width, $height);
} elseif ($imgtype_xxx == "3") {
// png
$watermark_read = imagecreatefrompng($watermarkfile);
imagealphablending($this->image, true);// add this for transparent watermark thru image. if not add transparent from watermark can see thru background under image.
imagecopy($this->image, $watermark_read, $posx, $posy, 0, 0, $width, $height);
} elseif ($imgtype_xxx == "15") {
// bmp
$watermark_read = imagecreatefromwbmp($watermarkfile);
imagecopy($this->image, $watermark_read, $posx, $posy, 0, 0, $width, $height);
} else {
@imagedestroy($watermark_read);
return false;
}
} else {
@imagedestroy($watermark_read);
return false;
}
@imagedestroy($watermark_read);
return $this->image;
}// watermark
public function setWatermark($watermarkfile, $position="center") {
if (file_exists($watermarkfile)) {
//Image size
$this->image_width=$this->getWidth();
$this->image_height=$this->getHeight();
// find watermark size
$wmsize = getimagesize($watermarkfile);
if ($wmsize != false) {
$width = $wmsize[0];
$height = $wmsize[1];
}
// end find watermark size
if ($position == "topleft") {
if (($this->image_width+5) > $width && ($this->image_height+5) > $height) {
$posx = "5";
$posy = "5";
} else {
$posx = "0";
$posy = "0";
}
} elseif ($position == "top") {
$center_of_image = round($this->image_width/2);// width
$center_of_watermark = round($width/2);// width
$posx = ($center_of_image-$center_of_watermark);
if(($this->image_height) > ($height+5)) {
$posy = "5";
} else {
$posy = "0";
}
} elseif ($position == "topright") {
if ($this->image_width > ($width+5)) {
$posx = $this->image_width-($width+5);
} else {
$posx = $this->image_width-$width;
}
if ($this->image_height > ($height+5)) {
$posy = "5";
} else {
$posy = "0";
}
} elseif ($position == "left") {
if ($this->image_width-($width+5) > "0") {
$posx = "5";
} else {
$posx = "0";
}
$middle_of_image = round($this->image_height/2);// height
$middle_of_watermark = round($height/2);// height
$posy = ($middle_of_image-$middle_of_watermark);
} elseif ($position == "center") {
$center_of_image = round($this->image_width/2);// width
$center_of_watermark = round($width/2);// width
$posx = ($center_of_image-$center_of_watermark);
$middle_of_image = round($this->image_height/2);// height
$middle_of_watermark = round($height/2);// height
$posy = ($middle_of_image-$middle_of_watermark);
} elseif ($position == "right") {
if ($this->image_width > ($width+5)) {
$posx = $this->image_width-($width+5);
} else {
$posx = $this->image_width-$width;
}
$middle_of_image = round($this->image_height/2);// height
$middle_of_watermark = round($height/2);// height
$posy = ($middle_of_image-$middle_of_watermark);
} elseif ($position == "bottomleft") {
if ($this->image_width-($width+5) > "0") {
$posx = "5";
} else {
$posx = "0";
}
if ($this->image_height-($height+5) > "0") {
$posy = $this->image_height-($height+5);
} else {
$posy = $this->image_height-($height);
}
} elseif ($position == "bottom") {
$center_of_image = round($this->image_width/2);// width
$center_of_watermark = round($width/2);// width
$posx = ($center_of_image-$center_of_watermark);
if ($this->image_height-($height+5) > "0") {
$posy = $this->image_height-($height+5);
} else {
$posy = $this->image_height-($height);
}
} elseif ($position == "bottomright") {
if ($this->image_width > ($width+5)) {
$posx = $this->image_width-($width+5);
} else {
$posx = $this->image_width-$width;
}
if ($this->image_height-($height+5) > "0") {
$posy = $this->image_height-($height+5);
} else {
$posy = $this->image_height-($height);
}
} else {
$this->destroy();
return false;
}
} else {
$this->destroy();
return false;
}
return $this->watermark($watermarkfile, $posx, $posy);
}
}
?>
test
$img[0]="002.jpg";
$img[1]="031.jpg";
$img[2]="N800.png";
$img[3]="001.jpg";
$img[4]="010.jpg";
echo "<br><br>#################### Old image ####################<br><br>";
echo "<img src='images/010.jpg'>";
echo "<br><br>#################### New image ####################<br><br>";
//Resize only ย่อรูปอย่างเดียวเป็นขนาด กว้าง 250px ความสูงอัตโนมัติ
$image = new ImageAffect("images/".$img[4]);
$image->resizeToWidth(250);
$dd=$image->save('resize/picturess');
echo "<img src='$dd'>";
//Resize && set green color // ย่อรูปยึดขนาดความกว้างเป็นหลัก ที่ขนาด 250px ความสูงปรับอัตโนมัติ และปรับรูปใหม่เป็นสีเขียว
$image = new ImageAffect("images/".$img[4]);
$image->resizeToWidth(250);
$image->setEffect(array("COLOR"=>true,"color"=>array("r"=>0,"g"=>120,"b"=>0)));
$dd=$image->save('resize/picture');
echo "<img src='$dd' >";
//Resize 50% && set effect pencel && rotate image -90 degree && set watermark // ย่อรูป ใส่เอฟเฟคเหมือนภาพวาดการ์ตูน หมุน -90 องศา และใส่ลายน้ำ
$image = new ImageAffect("images/".$img[4]);
$image->scale(50);
$image->setEffect(array("PENCIL"=>true));
$image->rotateImage(-90);
$image->setWatermark("images/watermark.png","bottomright");
$dd2=$image->save('resize/picture3.jpg');
echo "<img src='resize/picture3.jpg'>";
//ย่อและขยายรูป ให้มีขนาด กว้าง 200px สูง 300px
$image = new ImageAffect("images/".$img[4]);
#$image->load("images/".$img[4]);
$image->resizeCrop(200,300);
$ddsd=$image->save('resize/picturessddd');
echo "<img src='$ddsd' >";
echo " w: $width h: $height t: $type a: $attr";*/
?>
Screenshot

|
|
|
|
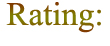 |
|