|
PHP MySQL Display Row No and Line Number แสดงเลขหน้ากำกับแถว |
PHP MySQL Display Row No and Line Number ตัวอย่าง PHP ดึงข้อมูลจาก MySQL แล้วมีการแสดงเลขหน้ากำหนดแถว Row No หรือบรรทัด Line Number นั้น ๆ ว่าเป็นข้อมูล Record ที่เท่าไหร่
เริ่มต้นด้วยการสร้างตารางชื่อ customer
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM;
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'EN', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
นำคำสั่ง SQL นี้ไปสร้างตาราง
ตัวอย่างที่ 1 การแสดงข้อมูลและแสดงหมายเลขกำกับแถว ในตัวอย่างที่ 1 สามารถสร้างตัวแปร $i และเพิ่มค่า +1 ($i++) ในแต่ล่ะ Loop ได้เลย
PHPmySQLRowNo.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
?>
<table width="585" border="1">
<tr>
<th width="42"> <div align="center">No </div></th>
<th width="102"> <div align="center">CustomerID </div></th>
<th width="70"> <div align="center">Name </div></th>
<th width="118"> <div align="center">Email </div></th>
<th width="94"> <div align="center">CountryCode </div></th>
<th width="55"> <div align="center">Budget </div></th>
<th width="58"> <div align="center">Used </div></th>
</tr>
<?php
$i = 1;
while($objResult = mysql_fetch_array($objQuery))
{
?>
<tr>
<td><div align="center"><?php echo $i;?></div></td>
<td><div align="center"><?php echo $objResult["CustomerID"];?></div></td>
<td><?php echo $objResult["Name"];?></td>
<td><?php echo $objResult["Email"];?></td>
<td><div align="center"><?php echo $objResult["CountryCode"];?></div></td>
<td align="right"><?php echo $objResult["Budget"];?></td>
<td align="right"><?php echo $objResult["Used"];?></td>
</tr>
<?php
$i++;
}
?>
</table>
<?php
mysql_close($objConnect);
?>
</body>
</html>
Screenshot
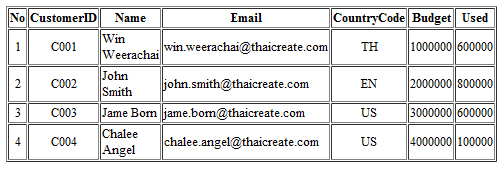

ตัวอย่างที่ 2 กรณีที่มีการแบ่งหน้า และมีการคลิกไปยังหน้าถัดไปตัวแปร $i จะต้องมีค่าเริ่มต้นตามจำนวนหน้า ตาม Logic
if($Page > 1)
{
$i = ($Per_Page * ($Page-1)) + 1;
}
Code ทั้งหมด
PHPmySQLPaginationRowNo.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer ";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
$Num_Rows = mysql_num_rows($objQuery);
$Per_Page = 2; // Per Page
$Page = $_GET["Page"];
if(!$_GET["Page"])
{
$Page=1;
}
$Prev_Page = $Page-1;
$Next_Page = $Page+1;
$Page_Start = (($Per_Page*$Page)-$Per_Page);
if($Num_Rows<=$Per_Page)
{
$Num_Pages =1;
}
else if(($Num_Rows % $Per_Page)==0)
{
$Num_Pages =($Num_Rows/$Per_Page) ;
}
else
{
$Num_Pages =($Num_Rows/$Per_Page)+1;
$Num_Pages = (int)$Num_Pages;
}
$strSQL .=" order by CustomerID ASC LIMIT $Page_Start , $Per_Page";
$objQuery = mysql_query($strSQL);
?>
<table width="600" border="1">
<tr>
<th width="20"> <div align="center">No </div></th>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
$i = 1;
if($Page > 1)
{
$i = ($Per_Page * ($Page-1)) + 1;
}
while($objResult = mysql_fetch_array($objQuery))
{
?>
<tr>
<td><div align="center"><?php echo $i;?></div></td>
<td><div align="center"><?php echo $objResult["CustomerID"];?></div></td>
<td><?php echo $objResult["Name"];?></td>
<td><?php echo $objResult["Email"];?></td>
<td><div align="center"><?php echo $objResult["CountryCode"];?></div></td>
<td align="right"><?php echo $objResult["Budget"];?></td>
<td align="right"><?php echo $objResult["Used"];?></td>
</tr>
<?php
$i++;
}
?>
</table>
<br>
Total <?php echo $Num_Rows;?> Record : <?php echo $Num_Pages;?> Page :
<?php
if($Prev_Page)
{
echo " <a href='$_SERVER[SCRIPT_NAME]?Page=$Prev_Page'><< Back</a> ";
}
for($i=1; $i<=$Num_Pages; $i++){
if($i != $Page)
{
echo "[ <a href='$_SERVER[SCRIPT_NAME]?Page=$i'>$i</a> ]";
}
else
{
echo "<b> $i </b>";
}
}
if($Page!=$Num_Pages)
{
echo " <a href ='$_SERVER[SCRIPT_NAME]?Page=$Next_Page'>Next>></a> ";
}
mysql_close($objConnect);
?>
</body>
</html>
Screenshot
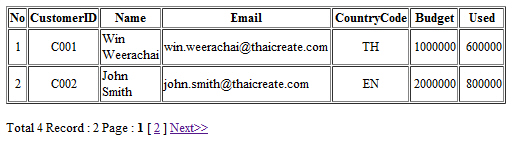
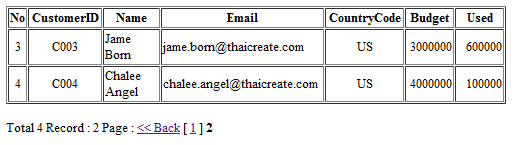
บทความที่เกี่ยวข้อง
Go to : PHP MySQL List Record
Go to : PHP MySQL List Record Paging/Pagination
Go to : PHP MySQL Pagination ทำแบ่งหน้าด้วย PHP กับ MySQL ย่อจำนวนหน้า ในกรณีที่มีจำนวนหน้าเยอะ ๆ
|
|
|
By : |
TC Admin
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
  |
|
Create Date : |
2011-01-27 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|