|
PHP Class split page แบ่งหน้า PHP & MySQL |
Pagination.class.php
<?php
/**
* Pagination.class.php
* Last Modify: May 2012
* By Narong Rammanee
*/
class Pagination
{
/**
* Previous page constant
* @var string
*/
const PREV = '‹ Prev';
/**
* First page constant
* @var string
*/
const FIRST = '« First';
/**
* Next page constant
* @var string
*/
const NEXT = 'Next ›';
/**
* Last page constant
* @var string
*/
const LAST = 'Last »';
/**
* All page
* @var interger
*/
public $totalPage;
/**
* SQL offset
* @var interger
*/
public $offset;
/**
* URL page
* @var unknown_type
*/
private $link;
/**
* All row of data
* @var integer
*/
private $records;
/**
* Current page
* @var interger
*/
private $curPage;
/**
* Per page
* @var integer
*/
private $perPage;
/**
* Scroll page
* @var integer
*/
private $scroll = 18;
/**
* Initialization
* @param string link
* @param integer records
* @param integer curPage
* @param integer perPage
*/
public function __construct($link, $records, $curPage, $perPage)
{
$this->link = $link;
$this->records = $records;
$this->curPage = $curPage;
$this->perPage = $perPage;
$this->totalPage = ($this->records) ? ceil($this->records / $this->perPage) : 0;
$this->curPage = (!is_numeric($this->curPage)) ? 1 : $this->curPage;
$this->offset = ($this->totalPage <= 1) ? 0 : ($this->curPage - 1) * $this->perPage;
}
/**
* Create Pagination
*
* @return void
*/
public function render()
{
if(!$html = self::compilePagination())
throw new Exception( 'Error obtaining Pagination!' );
echo $html;
}
/**
* define private 'compilePagination()' method
*
* @return complete Pagination
*/
private function compilePagination()
{
$html = '<p class="css-pager">';
if($this->totalPage > 1 && $this->curPage > 1) {
$html .= '<a href="' . $this->link . '1">' . self::FIRST . '</a>';
}
if($this->totalPage > 1 && $this->curPage > 1) {
$html .= '<a href="' . $this->link . ($this->curPage - 1) . '">' . self::PREV . '</a>';
}
if($this->totalPage <= $this->scroll) {
if($this->records <= $this->perPage) {
$startPage = 1;
$stopPage = $this->totalPage;
} else {
$startPage = 1;
$stopPage = $this->totalPage;
}
} else {
if($this->curPage < intval($this->scroll / 2) + 1) {
$startPage = 1;
$stopPage = $this->scroll;
} else {
$startPage = $this->curPage - intval($this->scroll / 2);
$stopPage = $this->curPage + intval($this->scroll / 2);
if($stopPage > $this->totalPage) $stopPage = $this->totalPage;
}
}
if ($this->totalPage > 1) {
for ($i = $startPage; $i <= $stopPage; $i++) {
if ($i == $this->curPage) {
$html .= '<span class="current_page">' . $i . '</span>';
} else {
$html .= '<a href="'.$this->link.$i.'">' . $i . '</a>';
}
}
}
if($this->curPage < $this->totalPage) {
$html .= '<a href="' . $this->link . ($this->curPage + 1) . '">' . self::NEXT . '</a>';
}
if($this->curPage < $this->totalPage) {
$html .= '<a href="' . $this->link . $this->totalPage . '">' . self::LAST . '</a>';
}
return $html.'</p>';
}
}
index.php
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<title>Pagination</title>
<meta http-equiv="content-type" content="text/html;charset=utf-8" />
<meta name="generator" content="Geany 0.19.1" />
<link href="css/default.css" rel="stylesheet" type="text/css" />
</head>
<body>
<?php
require_once dirname(__FILE__) . '/classes/Pagination.class.php';
$records = 200;
$link = 'index.php?p=';
$current = $_REQUEST['p'] ? $_REQUEST['p'] : 1;
$perpage = 5;
for ($i = ((($current-1)*$perpage)+1); $i <= $current*$perpage; $i++) {
$data[$i] = $i * 1001;
}
$pager = new Pagination($link, $records, $current, $perpage);
try {
$pager->render();
echo '<pre>'; print_r($data); echo '</pre>';
$pager->render();
}
catch(Exception $e) { echo $e->getMessage(); }
?>
</body>
</html>
default.css
@CHARSET "UTF-8";
body {
font-family: "Trebuchet MS", Tahoma, Verdana, Arial, Helvetica, sans-serif;
font-size: 11px;
color: #4f6b72;
background: #E6EAE9
}
.pager {
padding: 5px 0 5px 0
}
.css-pager a {
text-decoration: none;
color: #666;
background: #F4F4F4;
border: 1px solid #e0e0e0;
padding: 2px 5px;
margin: 2px;
font-weight: 700;
font-size: 11px
}
.css-pager a:hover {
text-decoration: none;
color: #fff;
background: #0A85CB;
border: 1px solid #3af;
padding: 2px 5px;
margin: 2px
}
.current_page {
background-color: #0A85CB;
border: 1px solid #3af;
padding: 2px 5px;
margin: 2px;
color: #fff;
font-weight: 700;
font-size: 11px
}

|
|
|
|
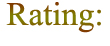 |
|
|
|
By : |
ranarong
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2009-08-17 |
|
Download : |
(0.0033 MB) |
|
Sponsored Links |
|
|
|
|
|
|