|
PHP Sorting Columns In MySQL คลิกที่ Column แล้วจัดเรียงข้อมูลด้วย PHP กับ MySQL |
PHP Sorting Column In MySQL ตัวอย่าง PHP ดึงข้อมูลจาก MySQL แล้วกำหนดให้ส่วนหัวของ Header Column สามารถ Sort จัดเรียงตาม Keyword ทีได้คลิกเลือกจาก Header ของ Column
เริ่มต้นด้วยการสร้างตารางชื่อ customer
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM;
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'EN', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
นำคำสั่ง SQL นี้ไปสร้างตาราง
คำอธิบายการทำงาน
หลักการจัดเรียงจาก PHP กับ MySQL ไม่ยาก เพียงเราส่งค่าตัวแปรจาก Link ที่มีการคลิกจาก Header ของ Column เช่น
<a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CustomerID">CustomerID</a>
จาก Code จะเห็นว่ามีการส่งตัวแปร sort ผ่าน $_GET มีค่าเป็น CustomerID ซึ่งเราจะเอาค่าตัวแปรนี้ไปทำการ sort เพื่อจัดเรียงใน SQL Statement และกรณีที่ไม่มีค่าก็ให้กำหนดค่า Default ตามตัวอย่าง
$strSort = $_GET["sort"];
if($strSort == "")
{
$strSort = "CustomerID";
}
ตัวอย่างที่ 1 การแสดงข้อมูลและคลิกที่ Header และทำการจัดเรียงข้อมูลตาม Column นั้น ๆ
PHPmySQLSort.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$strSort = $_GET["sort"];
if($strSort == "")
{
$strSort = "CustomerID";
}
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer ORDER BY ".$strSort." ASC";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CustomerID">CustomerID</a></div></th>
<th width="98"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Name">Name</a></div></th>
<th width="198"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Email">Email</a></div></th>
<th width="97"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CountryCode">CountryCode</a></div></th>
<th width="59"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Budget">Budget</a></div></th>
<th width="71"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Used">Used</a></div></th>
</tr>
<?php
while($objResult = mysql_fetch_array($objQuery))
{
?>
<tr>
<td><div align="center"><?php echo $objResult["CustomerID"];?></div></td>
<td><?php echo $objResult["Name"];?></td>
<td><?php echo $objResult["Email"];?></td>
<td><div align="center"><?php echo $objResult["CountryCode"];?></div></td>
<td align="right"><?php echo $objResult["Budget"];?></td>
<td align="right"><?php echo $objResult["Used"];?></td>
</tr>
<?php
}
?>
</table>
<?php
mysql_close($objConnect);
?>
</body>
</html>
Screenshot
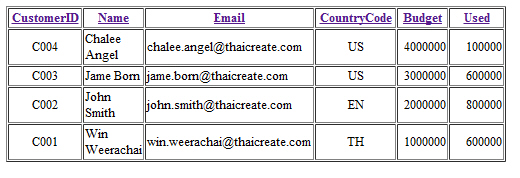

ตัวอย่างที่ 2 การแสดงข้อมูลและคลิกที่ Header และทำการจัดเรียงข้อมูลตาม Column นั้น ๆ แต่มีในส่วนของการแบ่งหน้า (Pagination เข้ามาด้วย)
PHPmySQLSortPagination.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer ";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
$Num_Rows = mysql_num_rows($objQuery);
$Per_Page = 2; // Per Page
$Page = $_GET["Page"];
if(!$_GET["Page"])
{
$Page=1;
}
$Prev_Page = $Page-1;
$Next_Page = $Page+1;
$Page_Start = (($Per_Page*$Page)-$Per_Page);
if($Num_Rows<=$Per_Page)
{
$Num_Pages =1;
}
else if(($Num_Rows % $Per_Page)==0)
{
$Num_Pages =($Num_Rows/$Per_Page) ;
}
else
{
$Num_Pages =($Num_Rows/$Per_Page)+1;
$Num_Pages = (int)$Num_Pages;
}
$strSort = $_GET["sort"];
if($strSort == "")
{
$strSort = "CustomerID";
}
$strSQL .=" order by ".$strSort." ASC LIMIT $Page_Start , $Per_Page";
$objQuery = mysql_query($strSQL);
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CustomerID">CustomerID</a></div></th>
<th width="98"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Name">Name</a></div></th>
<th width="198"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Email">Email</a></div></th>
<th width="97"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CountryCode">CountryCode</a></div></th>
<th width="59"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Budget">Budget</a></div></th>
<th width="71"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Used">Used</a></div></th>
</tr>
<?php
while($objResult = mysql_fetch_array($objQuery))
{
?>
<tr>
<td><div align="center"><?php echo $objResult["CustomerID"];?></div></td>
<td><?php echo $objResult["Name"];?></td>
<td><?php echo $objResult["Email"];?></td>
<td><div align="center"><?php echo $objResult["CountryCode"];?></div></td>
<td align="right"><?php echo $objResult["Budget"];?></td>
<td align="right"><?php echo $objResult["Used"];?></td>
</tr>
<?php
}
?>
</table>
<br>
Total <?php echo $Num_Rows;?> Record : <?php echo $Num_Pages;?> Page :
<?php
if($Prev_Page)
{
echo " <a href='$_SERVER[PHP_SELF]?Page=$Prev_Page&sort=$strSort'><< Back</a> ";
}
for($i=1; $i<=$Num_Pages; $i++){
if($i != $Page)
{
echo "[ <a href='$_SERVER[PHP_SELF]?Page=$i&sort=$strSort'>$i</a> ]";
}
else
{
echo "<b> $i </b>";
}
}
if($Page!=$Num_Pages)
{
echo " <a href ='$_SERVER[PHP_SELF]?Page=$Next_Page&sort=$strSort'>Next>></a> ";
}
mysql_close($objConnect);
?>
</body>
</html>
Screenshot
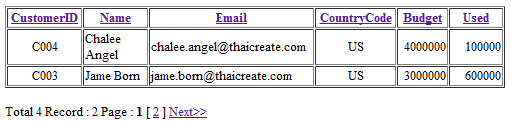
ตัวอย่างที่ 3 กรณีที่คลิกที่หัว Column อีกครั้ง จะสลับจาก ASC เป็น DESC หรือจาก DESC เป็น ASC
PHPmySQLSortPagination.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer ";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
$Num_Rows = mysql_num_rows($objQuery);
$Per_Page = 2; // Per Page
$Page = $_GET["Page"];
if(!$_GET["Page"])
{
$Page=1;
}
$Prev_Page = $Page-1;
$Next_Page = $Page+1;
$Page_Start = (($Per_Page*$Page)-$Per_Page);
if($Num_Rows<=$Per_Page)
{
$Num_Pages =1;
}
else if(($Num_Rows % $Per_Page)==0)
{
$Num_Pages =($Num_Rows/$Per_Page) ;
}
else
{
$Num_Pages =($Num_Rows/$Per_Page)+1;
$Num_Pages = (int)$Num_Pages;
}
$strSort = $_GET["sort"];
if($strSort == "")
{
$strSort = "CustomerID";
}
$strOrder = $_GET["order"];
if($strOrder == "")
{
$strOrder = "ASC";
}
$strSQL .=" order by ".$strSort." ".$strOrder." LIMIT $Page_Start , $Per_Page";
$objQuery = mysql_query($strSQL);
$strNewOrder = $strOrder == 'DESC' ? 'ASC' : 'DESC';
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CustomerID&order=<?php echo $strNewOrder?>">CustomerID</a></div></th>
<th width="98"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Name&order=<?php echo $strNewOrder?>">Name</a></div></th>
<th width="198"><div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Email&order=<?php echo $strNewOrder?>">Email</a></div></th>
<th width="97"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=CountryCode&order=<?php echo $strNewOrder?>">CountryCode</a></div></th>
<th width="59"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Budget&order=<?php echo $strNewOrder?>">Budget</a></div></th>
<th width="71"> <div align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?sort=Used&order=<?php echo $strNewOrder?>">Used</a></div></th>
</tr>
<?php
while($objResult = mysql_fetch_array($objQuery))
{
?>
<tr>
<td><div align="center"><?php echo $objResult["CustomerID"];?></div></td>
<td><?php echo $objResult["Name"];?></td>
<td><?php echo $objResult["Email"];?></td>
<td><div align="center"><?php echo $objResult["CountryCode"];?></div></td>
<td align="right"><?php echo $objResult["Budget"];?></td>
<td align="right"><?php echo $objResult["Used"];?></td>
</tr>
<?php
}
?>
</table>
<br>
Total <?php echo $Num_Rows;?> Record : <?php echo $Num_Pages;?> Page :
<?php
if($Prev_Page)
{
echo " <a href='$_SERVER[PHP_SELF]?Page=$Prev_Page&sort=$strSort'><< Back</a> ";
}
for($i=1; $i<=$Num_Pages; $i++){
if($i != $Page)
{
echo "[ <a href='$_SERVER[PHP_SELF]?Page=$i&sort=$strSort'>$i</a> ]";
}
else
{
echo "<b> $i </b>";
}
}
if($Page!=$Num_Pages)
{
echo " <a href ='$_SERVER[PHP_SELF]?Page=$Next_Page&sort=$strSort'>Next>></a> ";
}
mysql_close($objConnect);
?>
</body>
</html>

บทความที่เกี่ยวข้อง
Go to : PHP MySQL List Record
Go to : PHP MySQL List Record Paging/Pagination
Go to : PHP MySQL Pagination ทำแบ่งหน้าด้วย PHP กับ MySQL ย่อจำนวนหน้า ในกรณีที่มีจำนวนหน้าเยอะ ๆ
Go to : PHP MySQL Display Row No and Line Number แสดงเลขหน้ากำกับแถว
|
|
|
By : |
TC Admin
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
  |
|
Create Date : |
2011-05-26 |
|
Download : |
No files |
|
Sponsored Links |
|
|
|
|
|
|