|
PHP - Web Service กับ MySQL Database รับส่งค่า Result ผ่านเว็บเซอร์วิส (NuSoap) |
PHP - Web Service กับ MySQL Database รับส่งค่า Result ผ่านเว็บเซอร์วิส (NuSoap) บทความนี้เป็นภาคต่อของ PHP กับ Web Service และการ Return ค่า Array และจำเป็นเลยที่ขาดไม่ได้สำหรับผู้ที่กำลังศึกษาการเขียนโปรแกรม PHP กับ Web Service เพราะจะเป็นการประยุกต์การใช้งานกับ MySQL Database โดยในตัวอย่างนี้ทาง Web Service ของ Server จะมีฐานข้อมูล MySQL Database ชื่อว่า customer ซึ่งประกอบด้วยฟิวด์ CustomerID, Name, Email, CountryCode, Budget, Used ตามตัวอย่างในรูป
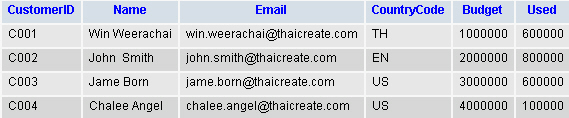
เปิดบริการให้ Client ทำการ Reqeust ข้อมูลเข้ามา โดยส่งรหัสประเทศ( CountryCode) ของลูกค้าเข้ามาใน Web Service และ Web Service จะทำการ Return ค่ารายชื่อลูกค้าทั้งหมดที่อยู่ในประเทศนั้น ๆ กลับไปยัง Client เช่น Client ทำการส่ง CountryCode = US
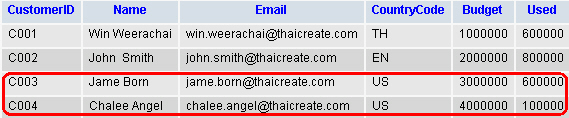
ในตัวอย่างมีรหัสประเทศ CountryCode = US อยู่ 2 รายชื่อ
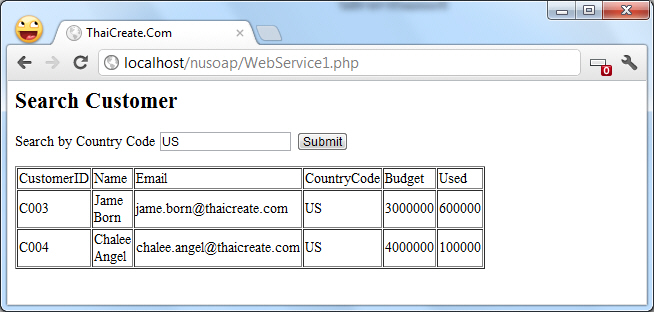
นี่คือหน้าจอ Web Service ฝั่ง Client และข้อมูลที่ Web Service ฝั่ง Server ได้ส่งกลับมา โดยข้อมูลที่ได้จะอยู่ในรูปแบบของ Array มี 2 มิติ
รูปแบบข้อมูลที่ Server ส่งกลับมา
array(2) {
[0]=>
array(6) {
["CustomerID"]=>
string(4) "C003"
["Name"]=>
string(9) "Jame Born"
["Email"]=>
string(24) "[email protected]"
["CountryCode"]=>
string(2) "US"
["Budget"]=>
float(3000000)
["Used"]=>
float(600000)
}
[1]=>
array(6) {
["CustomerID"]=>
string(4) "C004"
["Name"]=>
string(12) "Chalee Angel"
["Email"]=>
string(27) "[email protected]"
["CountryCode"]=>
string(2) "US"
["Budget"]=>
float(4000000)
["Used"]=>
float(100000)
}
}
โครงสร้างข้อมูลที่ส่งกลับไปยัง Client ซึ่งทาง Client สามารถใช้คำสั่ง foreach ในการแสดงค่าจาก Array
ก่อนอ่านบทความนี้ควรอ่าน 3 บทความนี้ก่อน
ฐานข้อมูลฝั่ง Server
REATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'EN', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
นำคำสั่ง SQL นี้ไปสร้าง Database ในฝั่งของ Web Server
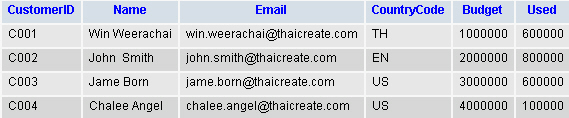
เมื่อสร้างเสร็จจะได้โครงสร้างและข้อมูลดังรูป
สำหรับบทความนี้ใช้ Library ของ NuSoap สามารถดาวน์โหลดได้ที่
หรือจะดาวน์โหลดได้จากในส่วนท้ายของบทความ
Code เต็ม ๆ
Web Service ฝั่ง Server
WebServiceServer.php
<?php
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "http://localhost/nusoap/WebServiceServer.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("getCustomer");
//Add ComplexType
$server->wsdl->addComplexType(
'DataList',
'complexType',
'struct',
'all',
'',
array(
'CustomerID' => array('name' => 'CustomerID', 'type' => 'xsd:string'),
'Name' => array('name' => 'Name', 'type' => 'xsd:string'),
'Email' => array('name' => 'Email', 'type' => 'xsd:string'),
'CountryCode' => array('name' => 'CountryCode', 'type' => 'xsd:string'),
'Budget' => array('name' => 'Budget', 'type' => 'xsd:float'),
'Used' => array('name' => 'Used', 'type' => 'xsd:float')
)
);
//Add ComplexType
$server->wsdl->addComplexType(
'DataListResult',
'complexType',
'array',
'',
'SOAP-ENC:Array',
array(),
array(
array('ref'=>'SOAP-ENC:arrayType','wsdl:arrayType'=>'tns:DataList[]')
),
'tns:DataList'
);
//Register our method and argument parameters
$varname = array(
'strCountry' => "xsd:string"
);
// Register service and method
$server->register('resultCustomer', // method name
$varname, // input parameters
array('return' => 'tns:DataListResult'));
function resultCustomer($strCountry)
{
$objConnect = mysql_connect("localhost","root","") or die(mysql_error());
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer WHERE 1 AND CountryCode like '%".$strCountry."%' ";
$objQuery = mysql_query($strSQL) or die (mysql_error());
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
return $resultArray;
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
Web Service ฝั่ง Client
สร้างไฟล์สำหรับ Client เพื่อทดสอบเรียก Web Sevice
WebServiceClient.php
<html>
<head>
<title>ThaiCreate.Com</title>
</head>
<body>
<form name="frmMain" method="post" action="">
<h2>Search Customer</h2>
Search by Country Code
<input type="text" name="txtCountry" value="<?php echo $_POST["txtCountry"];?>">
<input type="submit" name="Submit" value="Submit">
</form>
<?php
if($_POST["txtCountry"] != "")
{
include("lib/nusoap.php");
$client = new nusoap_client("http://localhost/nusoap/WebServiceServer.php?wsdl",true);
$params = array(
'strCountry' => $_POST["txtCountry"]
);
$data = $client->call('resultCustomer', $params);
//echo '<pre>';
//var_dump($data);
//echo '</pre><hr />';
if(count($data) == 0)
{
echo "Not found data!";
}
else
{
?>
<table width="500" border="1">
<tr>
<td>CustomerID</td>
<td>Name</td>
<td>Email</td>
<td>CountryCode</td>
<td>Budget</td>
<td>Used</td>
</tr>
<?php
foreach ($data as $result) {
?>
<tr>
<td><?php echo $result["CustomerID"];?></td>
<td><?php echo $result["Name"];?></td>
<td><?php echo $result["Email"];?></td>
<td><?php echo $result["CountryCode"];?></td>
<td><?php echo $result["Budget"];?></td>
<td><?php echo $result["Used"];?></td>
</tr>
<?php
}
}
}
?>
</body>
</html>
Screenshot
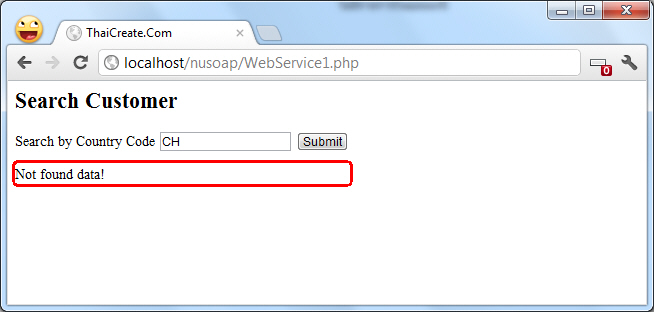
กรณีที่ไม่พบข้อมูล
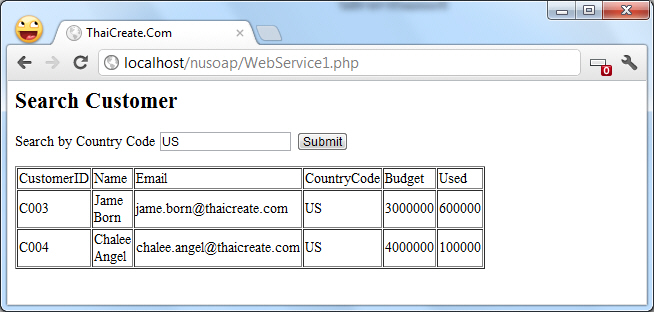
ข้อมูลที่พบข้อมูล
เพิ่มเติม
กรณีที่ใช้ var_dump($data) เพื่อแสดงข้อมูล ก็จะได้ result ที่อยู่ในรูปแบบของ array ดัวตัวอย่าง
echo '<pre>';
var_dump($data);
echo '</pre><hr />';
Result ที่ Server ส่งกลับมา
array(2) {
[0]=>
array(6) {
["CustomerID"]=>
string(4) "C003"
["Name"]=>
string(9) "Jame Born"
["Email"]=>
string(24) "[email protected]"
["CountryCode"]=>
string(2) "US"
["Budget"]=>
float(3000000)
["Used"]=>
float(600000)
}
[1]=>
array(6) {
["CustomerID"]=>
string(4) "C004"
["Name"]=>
string(12) "Chalee Angel"
["Email"]=>
string(27) "[email protected]"
["CountryCode"]=>
string(2) "US"
["Budget"]=>
float(4000000)
["Used"]=>
float(100000)
}
}
ทดสอบกับ .NET ด้วย ASP.NET
สำหรับพื้นฐาน ASP.NET เรียก Web Service ของ PHP สามารถอ่านได้ที่นี่
Go to : PHP สร้าง Web Service และใช้ .NET เรียก Web Service ของ PHP (ASP.NET and Windows Form)
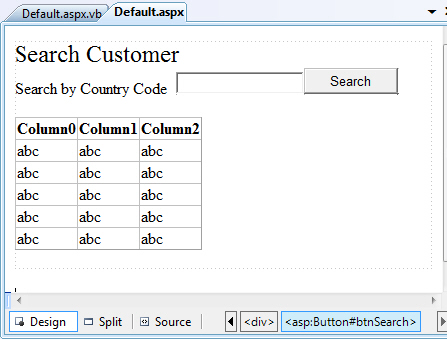
สร้างหน้า WebPage เหมือนในรูป ประกอบด้วย Label , Textbox , Button และ GridView เหมือนในรูปประกอบ
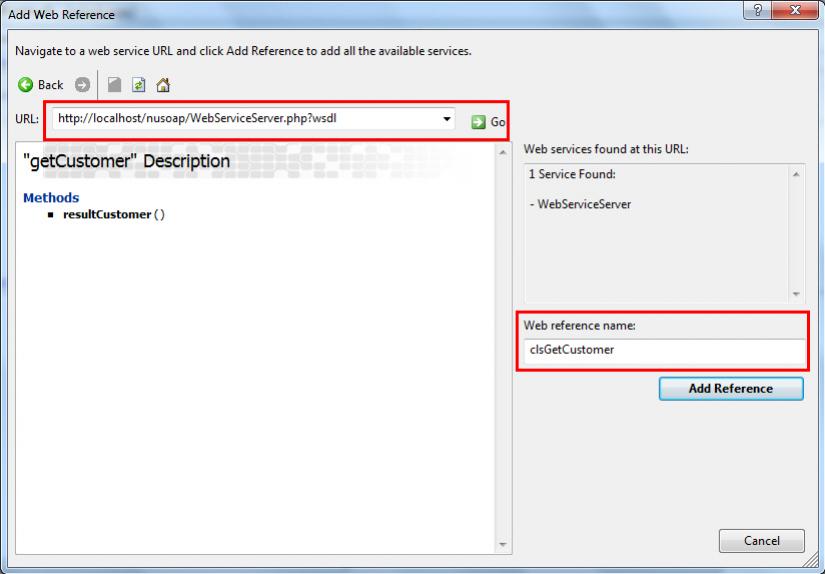
Add หน้า Web Service กรอก URL ให้ถูกต้อง
Code สำหรับภาษา VB.NET และ C#
- VB.NET
Protected Sub btnSearch_Click(ByVal sender As Object, ByVal e As EventArgs) Handles btnSearch.Click
Dim cls As New clsGetCustomer.getCustomer
GridView1.DataSource = cls.resultCustomer(Me.txtCountry.Text)
GridView1.DataBind()
End Sub
- C#
protected void btnSearch_Click(object sender, EventArgs e)
{
clsGetCustomer.getCustomer cls = new clsGetCustomer.getCustomer();
GridView1.DataSource = cls.resultCustomer(this.txtCountry.Text);
GridView1.DataBind();
}
อธิบายเพิ่มเติม
จาก Code จะเห็นว่าสามารถรับค่าจาก Web Service และโยน DataSource ให้กับ GridView ได้เลย โดยไม่ต้องการประกาศตัวแปรหรือทำการ DirectCast แต่อย่างใด
หรือถ้าต้องการ Loop ข้อมูลจาก Web Service ให้อยู่ในรูปแบบของ DataTable ก็สามารถทำได้เช่นเดียวกัน
- VB.NET
Protected Sub DataTableToGridView()
Dim cls As New clsGetCustomer.getCustomer
Dim myArr As Array = DirectCast(cls.resultCustomer(Me.txtCountry.Text), Array)
Dim dt As DataTable
Dim dr As DataRow
dt = New DataTable()
dt.Columns.Add("CustomerID")
dt.Columns.Add("Name")
dt.Columns.Add("Email")
dt.Columns.Add("CountryCode")
dt.Columns.Add("Budget")
dt.Columns.Add("Used")
For Each arr In myArr
dr = dt.NewRow()
dr("CustomerID") = DataBinder.Eval(arr, "CustomerID").ToString()
dr("Name") = DataBinder.Eval(arr, "Name").ToString()
dr("Email") = DataBinder.Eval(arr, "Email").ToString()
dr("CountryCode") = DataBinder.Eval(arr, "CountryCode").ToString()
dr("Budget") = DataBinder.Eval(arr, "Budget").ToString()
dr("Used") = DataBinder.Eval(arr, "Used").ToString()
dt.Rows.Add(dr)
Next
GridView1.DataSource = dt.DefaultView
GridView1.DataBind()
End Sub
- C#
protected void DataTableToGridView()
{
clsGetCustomer.getCustomer cls = new clsGetCustomer.getCustomer();
Array myArr = (Array)cls.resultCustomer(this.txtCountry.Text);
DataTable dt = null;
DataRow dr = null;
dt = new DataTable();
dt.Columns.Add("CustomerID");
dt.Columns.Add("Name");
dt.Columns.Add("Email");
dt.Columns.Add("CountryCode");
dt.Columns.Add("Budget");
dt.Columns.Add("Used");
foreach (object arr in myArr)
{
dr = dt.NewRow();
dr["CustomerID"] = DataBinder.Eval(arr, "CustomerID").ToString();
dr["Name"] = DataBinder.Eval(arr, "Name").ToString();
dr["Email"] = DataBinder.Eval(arr, "Email").ToString();
dr["CountryCode"] = DataBinder.Eval(arr, "CountryCode").ToString();
dr["Budget"] = DataBinder.Eval(arr, "Budget").ToString();
dr["Used"] = DataBinder.Eval(arr, "Used").ToString();
dt.Rows.Add(dr);
}
GridView1.DataSource = dt.DefaultView;
GridView1.DataBind();
}
Screenshot
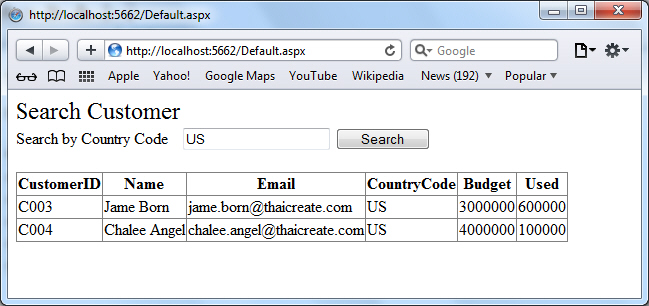
สำหรับ Code ทั้ง PHP Web Service รวมทั้ง ASP.NET by C# or VB.NET สามารถดาวน์โหลดได้จากที่นี่
Download Code !!
บทความอื่น ๆ ที่เกี่ยวข้อง
Go to : PHP Create - Call Web Service สร้างและเรียกเว็บเซอร์วิส ด้วย PHP (NuSoap and Soap)
Go to : PHP สร้าง Web Service และใช้ .NET เรียก Web Service ของ PHP (ASP.NET and Windows Form)
Go to : วิธีการสร้าง PHP กับ Web Service และ Return Array ไปยัง Client ด้วย NuSoap
Go to : php กับ web service ขอคำปรึกษาเรื่องการ return array ใน webservice ครับ
|
|
|
|
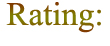 |
|
|
|
By : |
TC Admin
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
   |
|
Create Date : |
2012-05-18 |
|
Download : |
No files |
|
|
|
|