ASP.NET กับ JSON และการรับ-ส่งข้อมูล JSON ผ่าน Web Service (VB.NET , C#) |
|
|
|
ASP.NET กับ JSON และการรับส่งข้อมูล JSON ผ่าน Web Service (VB.NET , C#) ใน ASP.NET การรับส่ง Web Service สามารถทำการ Return ค่าจาก Web Service ในรูปแบบต่าง ๆ ได้ทันที เช่น String , Array หรือพวก DataSet / DataTable ซึ่งถ้าในฝั่ง Client เป็นพัฒนาด้วย .NET เช่นเดียวกัน ก็ไม่ใช่ปัญหาที่จะใช้การรับส่งแบบนั้น แต่ในกรณ๊ Client เป็นภาษาอื่น เช่น PHP , JSP หรืออื่น ๆ หรือใช้ JavaScript ในการแสดงผลจาก Web Service ของ ASP.NET ก็จะเป็นการยากที่จะอ่านค่าตัวแปรเหล่านั้นให้เข้าใจ ฉะนั้นการนำ JSON มาใช้ประโยชน์เป็นสื่อการในการรับส่งข้อมูล ระว่าง Server กับ Client ก็เป็นทางเลือกที่ดี และง่ายต่อการอ่านค่าที่ส่งมาในรูปแบบของ JSON
Screenshot
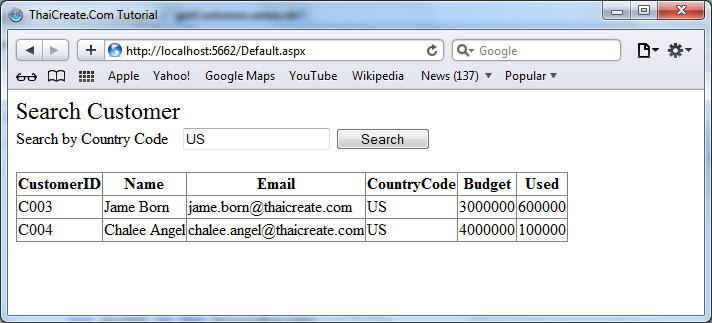
jQuery Ajax กับ JSON ทำความเข้าใจ การรับส่งข้อมูล JSON ผ่าน jQuery กับ Ajax
ก่อนอ่านบทความนี้ควรอ่าน 4 บทความนี้ก่อน
บทความนี้เป็นภาคต่อของบทความก่อน ๆ หน้านี้ บางส่วนอาจจะไม่ได้อธิบายให้ละเอียด ถ้าไม่เข้าใจแนะนำให้อ่านบทความที่ได้แนะนำ 4 บทความ
Database ของ SQL Server
USE [mydatabase]
GO
/****** Object: Table [dbo].[customer] Script Date: 05/01/2012 16:44:29 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[customer](
[CustomerID] [varchar](4) NOT NULL,
[Name] [varchar](50) NULL,
[Email] [varchar](50) NULL,
[CountryCode] [varchar](2) NULL,
[Budget] [float] NULL,
[Used] [float] NULL,
CONSTRAINT [PK_customer] PRIMARY KEY CLUSTERED
(
[CustomerID] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
SET ANSI_PADDING OFF
INSERT INTO customer VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO customer VALUES ('C002', 'John Smith', '[email protected]', 'EN', 2000000, 800000);
INSERT INTO customer VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO customer VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
นำคำสั่ง SQL นี้ไปสร้าง Database ในฝั่งของ Web Service Server
สำหรับบทความนี้จะเป็นตัวอย่างการค้นข้อมูลในตารางที่ชื่อว่า customer ใช้ฐานข้อมูล SQL Server และออกแบบ Form เหมือนใน Screenshot มีการค้นหาข้อมูลลูกค้าจาก CountryCode โดยใน Client จะส่ง CountryCode ไปยังฝั่ง Server ผ่าน Web Service และ Web Service จะ Return ค่า JSON กลับมายัง Client

เมื่อสร้างเสร็จจะได้โครงสร้างและข้อมูลดังรูป
Code เต็ม ๆ
Web Service ฝั่ง Server
สร้าง Application เป็น Web Service ด้วย ASP.NET ตั้งชื่อไฟล์เป็น getCustomer.asmx มีคำสั่งดังนี้
- VB.NET
getCustomer.asmx.vb
Imports System.Web.Services
Imports System.Web.Services.Protocols
Imports System.ComponentModel
Imports System.Data
Imports System.Data.SqlClient
Imports Newtonsoft.Json
' To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
' <System.Web.Script.Services.ScriptService()> _
<System.Web.Services.WebService(Namespace:="http://tempuri.org/")> _
<System.Web.Services.WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)> _
<ToolboxItem(False)> _
Public Class getCustomer
Inherits System.Web.Services.WebService
<WebMethod()> _
Public Function resultCustomer(ByVal strCountry As String) As String
Dim objConn As New SqlConnection
Dim objCmd As New SqlCommand
Dim dtAdapter As New SqlDataAdapter
Dim ds As New DataSet
Dim dt As DataTable
Dim strConnString As String
Dim strSQL As New StringBuilder
strConnString = "Server=localhost;UID=sa;PASSWORD=;database=mydatabase;Max Pool Size=400;Connect Timeout=600;"
strSQL.Append(" SELECT * FROM customer ")
strSQL.Append(" WHERE CountryCode LIKE '%" & strCountry & "%' ")
objConn.ConnectionString = strConnString
With objCmd
.Connection = objConn
.CommandText = strSQL.ToString()
.CommandType = CommandType.Text
End With
dtAdapter.SelectCommand = objCmd
dtAdapter.Fill(ds)
dt = ds.Tables(0)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
Dim json = JsonConvert.SerializeObject(dt, Formatting.Indented)
Return json
End Function
End Class
- C#
getCustomer.asmx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using System.Data.SqlClient;
using System.Text;
using Newtonsoft.Json;
namespace WebService_Server
{
/// <summary>
/// Summary description for getCustomer
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class getCustomer : System.Web.Services.WebService
{
[WebMethod]
public string resultCustomer(string strCountry)
{
SqlConnection objConn = new SqlConnection();
SqlCommand objCmd = new SqlCommand();
SqlDataAdapter dtAdapter = new SqlDataAdapter();
DataSet ds = new DataSet();
DataTable dt = null;
string strConnString = null;
StringBuilder strSQL = new StringBuilder();
strConnString = "Server=localhost;UID=sa;PASSWORD=;database=mydatabase;Max Pool Size=400;Connect Timeout=600;";
strSQL.Append(" SELECT * FROM customer ");
strSQL.Append(" WHERE CountryCode LIKE '%" + strCountry + "%' ");
objConn.ConnectionString = strConnString;
var _with1 = objCmd;
_with1.Connection = objConn;
_with1.CommandText = strSQL.ToString();
_with1.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
dt = ds.Tables[0];
dtAdapter = null;
objConn.Close();
objConn = null;
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
}
}
}
คำอธิบาย
จาก Code ใน Web Service จะมี Method ชื่อว่า resultCustomer ทำการรับค่า Parameter ชื่อว่า strCountry ทำการค้นหาข้อมูลในตารางที่่ชื่อว่า customer และจะได้ข้อมูลในรูปแบบของ DataTable และจะมีการเข้ารหัสเป็น JSON Code เพื่อทำการ Return ค่าไปยัง Client
เพิ่มเติม จะเห็นว่าใรการใช้ json = JsonConvert.SerializeObject(dt, Formatting.Indented) ซึ่งเป็น Open Source Library ของ JSON.NET ที่สามารถดาวน์โหลดมาใช้งานฟรี เพื่อช่วยให้การแปลงพวก DataTable , Array เป็นข้อความในรูปแบบของ JSON ได้ง่ายยิ่งขึ้น และในทางกลับกันทางฝังของ Client ก็สามารถใช้ JSON.NET เพื่อทำการ DeCode ข้อความ JSON ได้เช่นเดียวกัน
Download JSON.NET Library
http://json.codeplex.com/
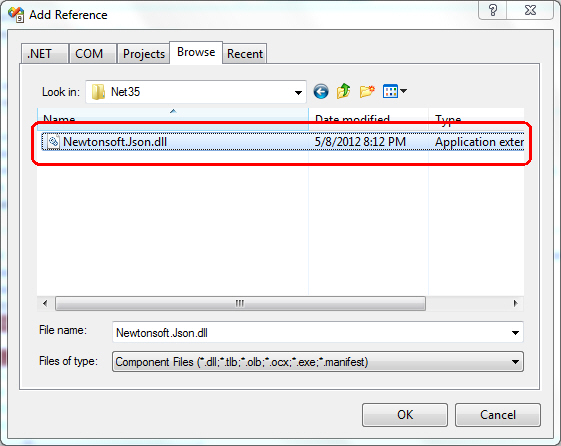
การ Add Reference คลาส Library ของ JSON.NET
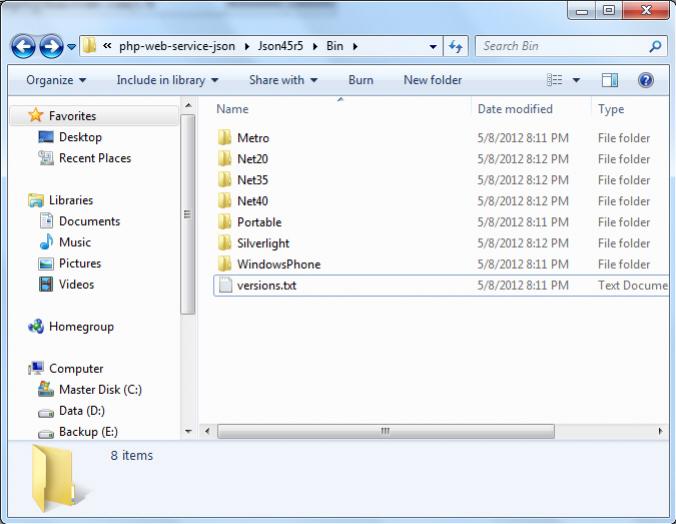
อย่าลืมเลือก Version ให้ถูกต้องด้วย
หลังจากทำตามขั้นตอนเรียบร้อย ก็ทำการ Save Project และ Build และทำการรันเพื่อทดสอบโปรแกรม
เมื่อทำการรันเพื่อดูผลลัพธ์
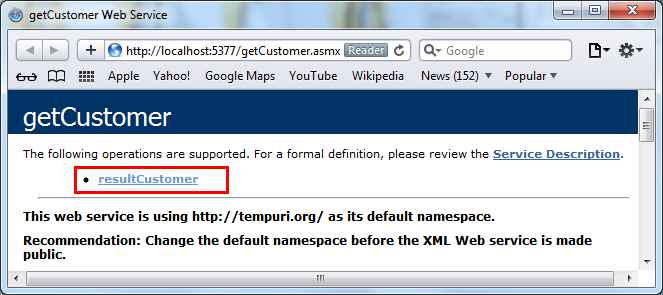
แสดง method ของ Web Service
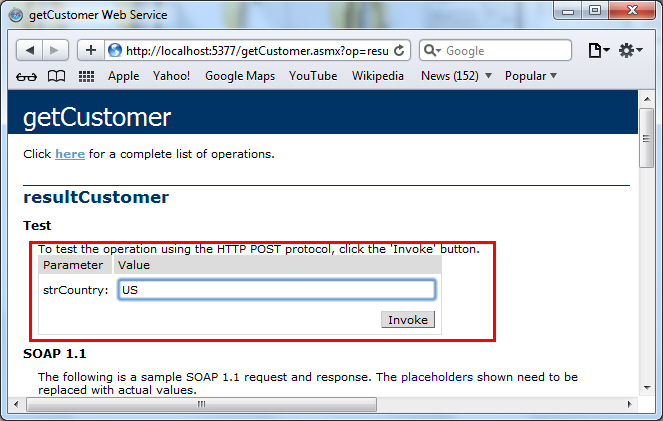
ลองทดสอบกรอกรหัส CountryCode เป็น US
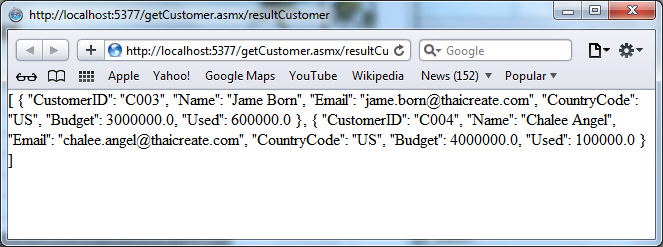
สิ่งที่ Web Service ทำการ return ค่ามาเป็น JSON Code
มุมมองของ JSON
[
{"CustomerID":"C003","Name":"Jame Born","Email":"[email protected]","CountryCode":"US","Budget":"3000000","Used":"600000"}
,{"CustomerID":"C004","Name":"Chalee Angel","Email":"[email protected]","CountryCode":"US","Budget":"4000000","Used":"100000"}
]
Web Service ฝั่ง Client ด้วย ASP.NET
ทดสอบการเรียก Web Service (ASP.NET) จาก Web Service ของ ASP.NET ที่ส่งค่ากลับมาในรูปแบบของ JSON สำหรับการอ่านค่า JSON ในฝั่งของ Client ให้ใช้ Library ของ JSON.NET เช่นเดียวกัน
ตัวอย่างการสร้าง WebPage และการ Add Web Reference ด้วย ASP.NET
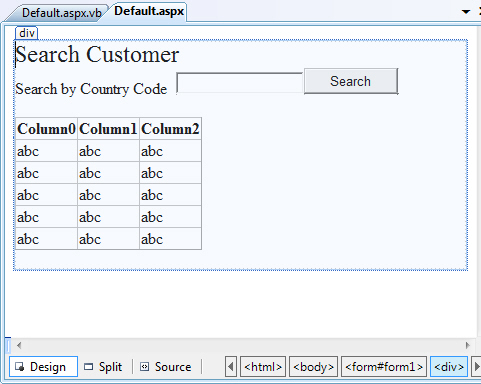
ออกแบบ Form ในหน้า WebPage ของ ASP.NET
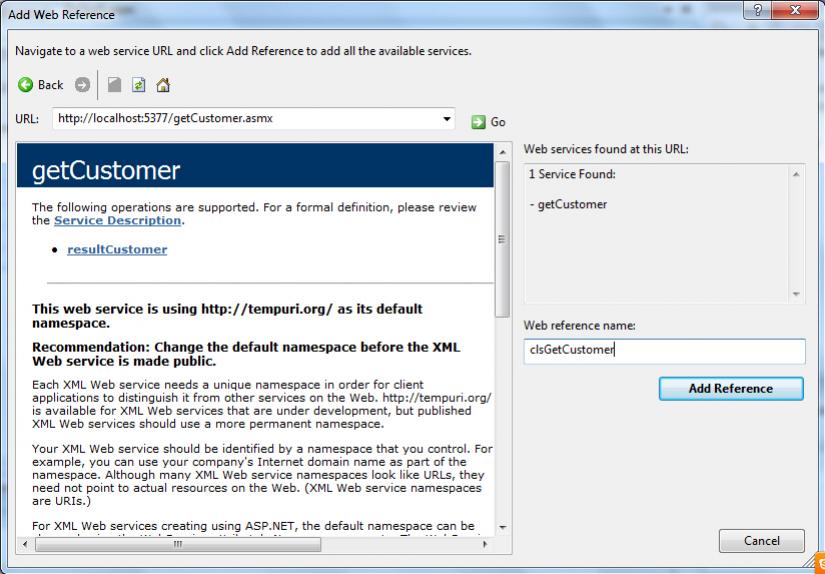
Add URL ของ ASP.NET Web Service
ขั้นตอนการ Add Reference คลาส dll ของ JSON.NET เข้ามาใน Project
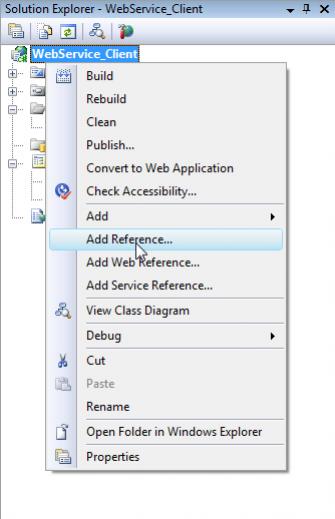
คลิกขวาที่ Project เลือก Add Reference ดังรูป
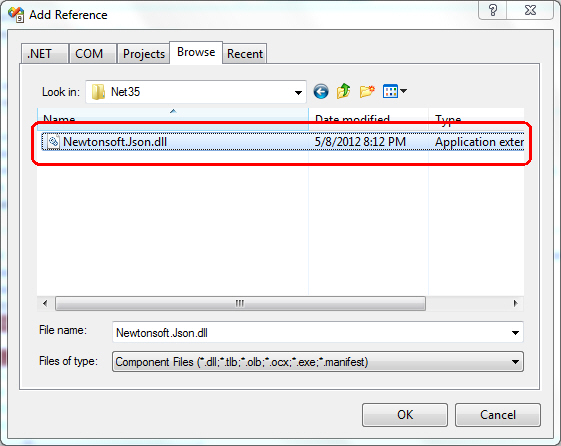
เลือก dll ของ JSON.NET ตาม Version ที่ได้ดาวน์โหลดมา
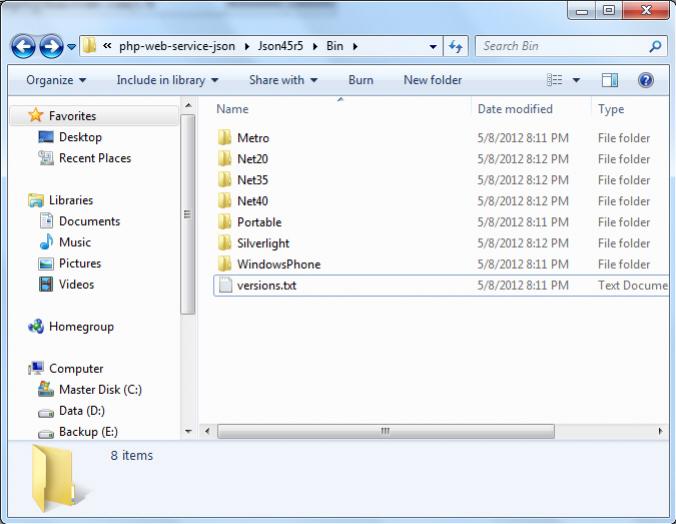
ในไฟล์ที่ดาวน์โหลดมาจะมีอยู่หลาย Version เช่น 2.0 , 3.5 , และ 4.5 (ใน 3.5 จะต้องเป็น Service Pack 1)
Screen Default.aspx
- สำหรับ VB.NET
Default.aspx.vb
Imports System.Data
Imports Newtonsoft.Json
Partial Public Class _Default
Inherits System.Web.UI.Page
Public Function DerializeDataTable(ByVal strJSON As String) As DataTable
Dim dt As DataTable
dt = JsonConvert.DeserializeObject(Of DataTable)(strJSON)
Return dt
End Function
Protected Sub btnSearch_Click(ByVal sender As Object, ByVal e As EventArgs) Handles btnSearch.Click
Dim cls As New clsGetCustomer.getCustomer
Dim strJSON = cls.resultCustomer(Me.txtCountry.Text)
GridView1.DataSource = DerializeDataTable(strJSON)
GridView1.DataBind()
End Sub
End Class
- สำหรับ C#
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using Newtonsoft.Json;
namespace WebService_Client
{
public partial class _Default : System.Web.UI.Page
{
public DataTable DerializeDataTable(string strJSON)
{
DataTable dt = null;
dt = JsonConvert.DeserializeObject<DataTable>(strJSON);
return dt;
}
protected void btnSearch_Click(object sender, EventArgs e)
{
clsGetCustomer.getCustomer cls = new clsGetCustomer.getCustomer();
string strJSON = cls.resultCustomer(this.txtCountry.Text).ToString();
GridView1.DataSource = DerializeDataTable(strJSON);
GridView1.DataBind();
}
}
}
คำอธิบาย
จาก Code จะ import ตัว namespace ของ Newtonsoft.Json เข้ามาใน Class และมีการเรียกใช้ JsonConvert.DeserializeObject<DataTable>(strJSON) เพื่อแปลงคา JSON ที่ถูกส่งมาจาก ASP.NET Web Service ฝั่ง Server ให้อยู่ในรูปแบบ DataTable พร้อม ๆ กับโยนค่า DataSource ให้กับ GridView
Screenshot
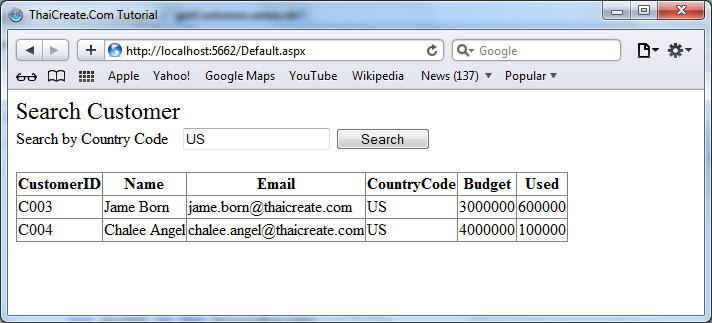
Web Service ฝั่ง Client ด้วย PHP
สำหรับ PHP จะใช้ Library ของ NuSoap ในการแปลงข้อความ JSON ที่ส่งมาจาก ASP.NET Web Service
Library ของ NuSoap สามารถดาวน์โหลดได้ที่
http://sourceforge.net/projects/nusoap/
WebServiceClient.php
<html>
<head>
<title>ThaiCreate.Com</title>
</head>
<body>
<form name="frmMain" method="post" action="">
<h2>Search Customer</h2>
Search by Country Code
<input type="text" name="txtCountry" value="<?=$_POST["txtCountry"];?>">
<input type="submit" name="Submit" value="Submit">
</form>
<?
if($_POST["txtCountry"] != "")
{
include("lib/nusoap.php");
$client = new nusoap_client("http://localhost:5377/getCustomer.asmx?wsdl",true);
$params = array(
'strCountry' => $_POST["txtCountry"]
);
$data = $client->call('resultCustomer', $params);
//echo '<pre>';
//var_dump(json_decode($data["resultCustomerResult"]));
//echo '</pre><hr />';
$mydata = json_decode($data["resultCustomerResult"],true); // json decode from web service
if(count($mydata) == 0)
{
echo "Not found data!";
}
else
{
?>
<table width="500" border="1">
<tr>
<td>CustomerID</td>
<td>Name</td>
<td>Email</td>
<td>CountryCode</td>
<td>Budget</td>
<td>Used</td>
</tr>
<?
foreach ($mydata as $result) {
?>
<tr>
<td><?=$result["CustomerID"];?></td>
<td><?=$result["Name"];?></td>
<td><?=$result["Email"];?></td>
<td><?=$result["CountryCode"];?></td>
<td><?=$result["Budget"];?></td>
<td><?=$result["Used"];?></td>
</tr>
<?
}
}
}
?>
</body>
</html>
คำอธิบาย
จาก Code ฝั่งของ Client มีการส่งค่า Country เพื่อไปค้นหาข้อมูลใน ASP.NET Web Service ฝั่ง Server และฝั่ง Server จะส่งข้อมูลกลับมาในรูปแบบของ JSON ซึ่งเมื่อ Client ได้ค่า JSON กลับมาก็ใช้การ decode ด้วย function ของ php ที่ชื่อว่า json_decode() โดยเมื่อผ่านการ decode ตัวแปรที่ได้จะอยู่ในรูปแบบของ Array และสามารถใช้ loop foreach หรืออื่น ๆ เพื่อทำอ่านการค่าออกมา
Screenshot
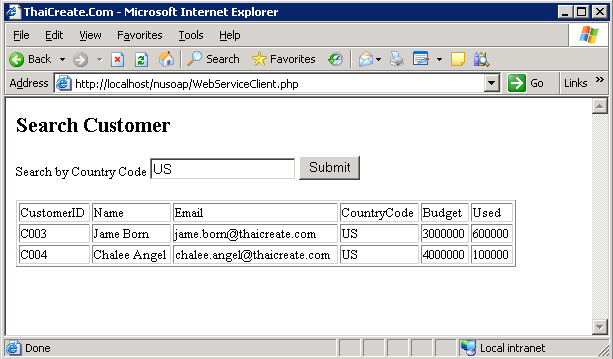
ดาวน์โหลด Code ทั้งหมด ทั้งที่เป็น ASP.NET Web Service และ ASP.NET by VB.NET or C# และ PHP
Download Code !!
บทความอื่น ๆ ที่เกี่ยวข้อง
Go to : ASP.NET เรียก PHP กับฐานข้อมูล MySQL ผ่าน Web Service และการรับส่งค่าผ่านเว็บเซอร์วิส
Go to : PHP และ JSON กับ Web Service การรับส่งข้อมูลจาก MySQL ในรูปแบบของ JSON
Go to : jQuery Ajax กับ JSON ทำความเข้าใจ การรับส่งข้อมูล JSON ผ่าน jQuery กับ Ajax
Go to : วิธีการสร้าง PHP กับ Web Service และ Return Array ไปยัง Client ด้วย NuSoap
Go to : PHP - Web Service กับ MySQL Database รับส่งค่า Result ผ่านเว็บเซอร์วิส (NuSoap)
Go to : การทำ Login Form และ Update MySQL Data ผ่าน Web Service ด้วย PHP (NuSoap)
Go to : PHP Create - Call Web Service สร้างและเรียกเว็บเซอร์วิส ด้วย PHP (NuSoap and Soap)
Go to : PHP สร้าง Web Service และใช้ .NET เรียก Web Service ของ PHP (ASP.NET and Windows Form)
Go to : php กับ web service ขอคำปรึกษาเรื่องการ return array ใน webservice ครับ
|
|
|
|
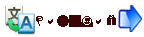 |
|
|
|
|
|
|
|
|
|
|
|
By : |
TC Admin
|
|
Score Rating : |
- |
|
Create Date : |
2012-05-26 08:38:26 |
|
Download : |
No files |
|
|
|
|
Related Links |
|
|
|
|
|
.NET Smart Device เขียนโปรแกรมบน Smartphone, Pocket PC , Windows CE , Window Mobile 5-6, Hand Held,...
.NET Smart Device Project เขียนโปรแกรมบน Smartphone, Pocket PC , Windows CE , Window Mobile 5-6, Hand Held,...
Rating :    
Update :
2017-03-24 21:17:48 View :
42,251 |
|
| |
|
|
|
|