 |
|
|
 |
 |
|
ใช้ sql server 2005 express ครับ
|
 |
 |
 |
 |
Date :
2009-02-19 22:22:36 |
By :
mamme |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ASP.NET & Search Record ดู VB.NET ไปก่อนน่ะครับ C# คงจะมีภายในเร็ว ๆ นี้ครับ
|
 |
 |
 |
 |
Date :
2009-02-20 00:10:17 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มาแล้วครับ C#
อันนี้ผมเคยทำไว้ เป็นการ search หา customer น่ะ
แต่ก่อนจะเอาไปใช้รบกวนศึกษา พวก event ของ gridview นิดนึงนะครับ
Code (C#)
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Text;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
public partial class AdminCustSearch : System.Web.UI.Page
{
DataSet ds = new DataSet();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
string strConn1 = "Data Source=MIXAR-PC;Initial Catalog=StockProductInfo;Integrated Security=True";
SqlConnection Conn1 = new SqlConnection(strConn1);
if (Conn1.State == ConnectionState.Open)
{
Conn1.Close();
}
Conn1.ConnectionString = strConn1;
Conn1.Open();
string sqlCustType;
sqlCustType = "SELECT DISTINCT CustType_ID, CustType_Name ";
sqlCustType += "FROM tabCustomerType ";
sqlCustType += "WHERE (CustType_Status LIKE 'yes')";
sqlCustType += "ORDER BY CustType_Name";
SqlDataAdapter da1 = new SqlDataAdapter(sqlCustType, Conn1);
DataSet ds1 = new DataSet();
da1.Fill(ds1, "CustType");
ddlCustomerType.DataSource = ds1.Tables["CustType"];
ddlCustomerType.DataValueField = "CustType_Name";
ddlCustomerType.DataTextField = "CustType_Name";
ddlCustomerType.DataBind();
ListItem firstItem = new ListItem("Select...", "Select...");
ddlCustomerType.Items.Insert(0, firstItem);
Conn1.Close();
}
}
protected void btnSearch_Click(object sender, EventArgs e)
{
string strConn2 = "Data Source=MIXAR-PC;Initial Catalog=StockProductInfo;Integrated Security=True";
SqlConnection Conn2 = new SqlConnection(strConn2);
if (Conn2.State == ConnectionState.Open)
{
Conn2.Close();
}
Conn2.ConnectionString = strConn2;
Conn2.Open();
string sqlCustSearch;
sqlCustSearch = "SELECT DISTINCT masCustomer.Cust_ID AS CustID, ";
sqlCustSearch += "masCustomer.Cust_FName AS Firstname, ";
sqlCustSearch += "masCustomer.Cust_LName AS Lastname, ";
sqlCustSearch += "masCustomer.Cust_Tel AS Telephone, ";
sqlCustSearch += "tabCustomerType.CustType_Name AS Type ";
sqlCustSearch += "FROM masCustomer, tabCustomerType ";
sqlCustSearch += "WHERE (masCustomer.CustType_ID = tabCustomerType.CustType_ID)";
if (ddlCustomerType.SelectedItem.Value != "Select...")
{
sqlCustSearch += "AND (tabCustomerType.CustType_Name = '" + ddlCustomerType.SelectedItem.Text + "')";
}
if (txtCustID.Text != "")
{
sqlCustSearch += "AND (masCustomer.Cust_ID LIKE '" + txtCustID.Text + "%')";
}
if (txtFirstname.Text != "")
{
sqlCustSearch += "AND (masCustomer.Cust_FName LIKE '" + txtFirstname.Text + "%')";
}
if (txtLastname.Text != "")
{
sqlCustSearch += "AND (masCustomer.Cust_LName LIKE '" + txtLastname.Text + "%')";
}
if (txtTelephone.Text != "")
{
sqlCustSearch += "AND (masCustomer.Cust_Tel LIKE '" + txtTelephone.Text + "%')";
}
SqlDataAdapter da3 = new SqlDataAdapter(sqlCustSearch, Conn2);
da3.Fill(ds, "Customers");
dgvCustResult.DataSource = ds.Tables["Customers"]; //เอาไปโชว์ในดาต้ากริดวิว
dgvCustResult.DataBind();
if (dgvCustResult.Rows.Count <= 0)
{
Response.Write("<script type='text/javascript'>alert('Search not found!!');</script>");
ddlCustomerType.SelectedValue = "Select...";
txtCustID.Text = "";
txtFirstname.Text = "";
txtLastname.Text = "";
txtTelephone.Text = "";
}
else
{
dgvCustResult.Visible = true;
}
Conn2.Close();
}
//method นี้ ผมมีปุ่ม select เป็น button field ไว้สำหรับดึงค่า ไปโชว์อีกเพจอะครับ
protected void dgvCustResult_SelectedIndexChanged(object sender, EventArgs e)
{
string custID, custFName, custLName, custName;
custID = dgvCustResult.SelectedRow.Cells[0].Text;
custFName = dgvCustResult.SelectedRow.Cells[1].Text;
custLName = dgvCustResult.SelectedRow.Cells[2].Text;
custName = custFName + " " + custLName;
Session["custFullName"] = custName;
Session["custID"] = custID;
Response.Redirect("AdminNewOrder.aspx");
}
}
แอด เอ็ม มา ถามได้ครับ
ถ้าตอบได้จะช่วยตอบ
[email protected]
|
 |
 |
 |
 |
Date :
2009-02-20 08:41:06 |
By :
mixarstudio |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
<%@ Import Namespace="System.Data"%>
<%@ Import Namespace="System.Data.SqlClient"%>
<%@ Page Language="C#" Debug="true" %>
<script runat="server">
String strKeyWord;
void Page_Load(object sender,EventArgs e)
{
strKeyWord = this.txtKeyWord.Text;
}
void BindData()
{
SqlConnection objConn = new SqlConnection();
SqlCommand objCmd = new SqlCommand();
SqlDataAdapter dtAdapter = new SqlDataAdapter();
DataSet ds = new DataSet();
String strConnString,strSQL;
strConnString = "Server=localhost;Uid=sa;PASSWORD=;database=mydatabase;Max Pool Size=400;Connect Timeout=600;";
strSQL = "SELECT * FROM customer WHERE (Name like '%"+ strKeyWord +"%' OR Email like '%"+ strKeyWord +"%') ";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL ;
objCmd.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
//*** BindData to GridView ***//
myGridView.DataSource = ds;
myGridView.DataBind();
dtAdapter = null;
objConn.Close();
objConn = null;
}
void myGridView_RowDataBound(Object s, GridViewRowEventArgs e)
{
//*** CustomerID ***//
Label lblCustomerID = (Label)(e.Row.FindControl("lblCustomerID"));
if (lblCustomerID != null)
{
lblCustomerID.Text = (string)DataBinder.Eval(e.Row.DataItem, "CustomerID");
}
//*** Email ***//
Label lblName = (Label)(e.Row.FindControl("lblName"));
if (lblName != null)
{
lblName.Text = (string)DataBinder.Eval(e.Row.DataItem, "Name");
}
//*** Name ***//
Label lblEmail = (Label)(e.Row.FindControl("lblEmail"));
if (lblEmail != null)
{
lblEmail.Text = (string)DataBinder.Eval(e.Row.DataItem, "Email");
}
//*** CountryCode ***//
Label lblCountryCode = (Label)(e.Row.FindControl("lblCountryCode"));
if (lblCountryCode != null)
{
lblCountryCode.Text = (string)DataBinder.Eval(e.Row.DataItem, "CountryCode");
}
//*** Budget ***//
Label lblBudget = (Label)(e.Row.FindControl("lblBudget"));
if (lblBudget != null)
{
lblBudget.Text = DataBinder.Eval(e.Row.DataItem, "Budget").ToString();
}
//*** Used ***//
Label lblUsed = (Label)(e.Row.FindControl("lblUsed"));
if (lblUsed != null)
{
lblUsed.Text = DataBinder.Eval(e.Row.DataItem, "Used").ToString();
}
}
void btnSearch_Click(Object sender, EventArgs e)
{
BindData();
}
</script>
<html>
<head>
<title>ThaiCreate.Com ASP.NET - SQL Server</title>
</head>
<body>
<form id="form1" runat="server">
<asp:Label id="lblKeyword" runat="server" text="Keyword"></asp:Label>
<asp:TextBox id="txtKeyWord" runat="server"></asp:TextBox>
<asp:Button id="btnSearch" onclick="btnSearch_Click" runat="server" Text="Search"></asp:Button>
<br />
<br />
<asp:GridView id="myGridView" runat="server"
AutoGenerateColumns="False" onRowDataBound="myGridView_RowDataBound">
<HeaderStyle backcolor="#cccccc"></HeaderStyle>
<AlternatingRowStyle backcolor="#e8e8e8"></AlternatingRowStyle>
<Columns>
<asp:TemplateField HeaderText="CustomerID">
<ItemTemplate>
<asp:Label id="lblCustomerID" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label id="lblName" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Email">
<ItemTemplate>
<asp:Label id="lblEmail" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="CountryCode">
<ItemTemplate>
<asp:Label id="lblCountryCode" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Budget">
<ItemTemplate>
<asp:Label id="lblBudget" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Used">
<ItemTemplate>
<asp:Label id="lblUsed" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</form>
</body>
</html>
Ref : (C#) ASP.NET SQL Server Search Record
|
 |
 |
 |
 |
Date :
2009-09-24 21:29:46 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอบคุนสำหรับข้อมูลดีๆ
|
 |
 |
 |
 |
Date :
2009-10-08 12:13:56 |
By :
tandanai |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
w'
|
 |
 |
 |
 |
Date :
2009-12-24 10:50:34 |
By :
byeday |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รันไม่ผ่านเลย
|
 |
 |
 |
 |
Date :
2009-12-24 13:55:43 |
By :
byeday |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ค่ามันไม่ตรงกัน
|
 |
 |
 |
 |
Date :
2009-12-24 13:57:12 |
By :
byeday |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Error อะไร ครับ
|
 |
 |
 |
 |
Date :
2009-12-24 14:14:12 |
By :
ksillapapan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอบคุณสำหรับข้อมูลต่างๆ
|
 |
 |
 |
 |
Date :
2010-03-22 15:00:09 |
By :
winnamo |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอบคุณคาบ
|
 |
 |
 |
 |
Date :
2011-10-05 19:00:15 |
By :
k.singha |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
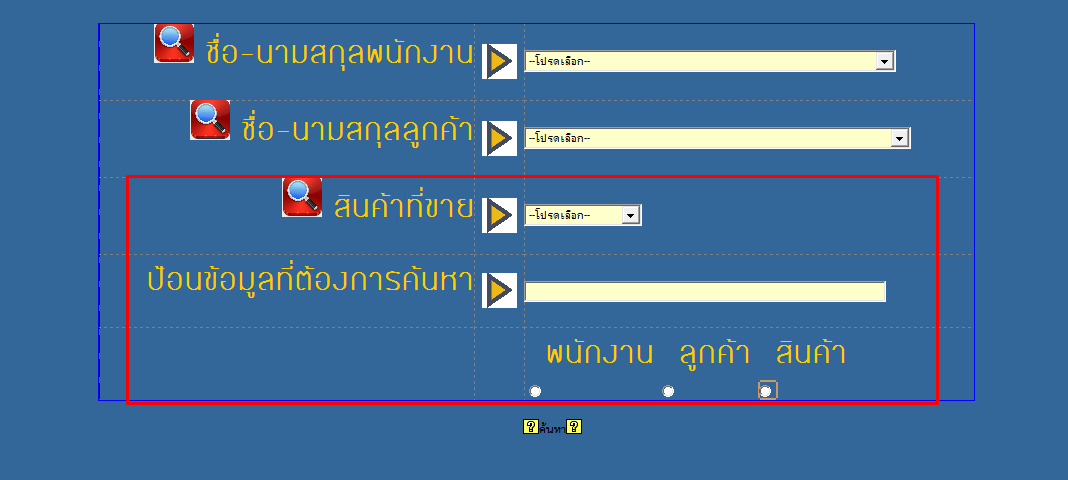
|
 |
 |
 |
 |
Date :
2017-05-30 12:18:10 |
By :
จินดา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อยากได้โค้ดค้นหาแบบ radio อ่ะค่ะ
|
 |
 |
 |
 |
Date :
2017-05-30 12:19:36 |
By :
จินดา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|