 |
|
พอดีอยากจะทำ Menu ที่สามารถแก้ไขข้อมูลได้ง่ายครับแต่ติดปัญหาเวลาเราเพิ่ม Node เข้าไปใน Provider ท่านใดมีประสบการณ์ช่วยหน่อยนะครับมีภาพครับ
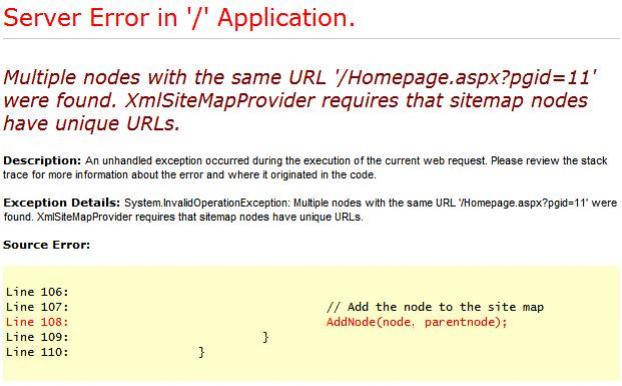
Code (C#)
using System;
using System.Web;
using System.Data.SqlClient;
using System.Collections.Specialized;
using System.Configuration;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
/// <summary>SqlSiteMapProvider</summary>
public class SqlSiteMapProvider : StaticSiteMapProvider
{
static readonly string _errmsg1 = "Missing connectionStringName attribute";
static readonly string _errmsg2 = "Duplicate node ID";
SiteMapNode _root = null;
string _connect;
public override void Initialize (string name, NameValueCollection attributes)
{
base.Initialize (name, attributes);
if (attributes == null)
throw new ConfigurationErrorsException (_errmsg1);
_connect = attributes["connectionStringName"];
if (String.IsNullOrEmpty (_connect))
throw new ConfigurationErrorsException(_errmsg1);
}
[MethodImpl(MethodImplOptions.Synchronized)]
public override SiteMapNode BuildSiteMap()
{
// Return immediately if this method has been called before
if (_root != null)
return _root;
// Create a dictionary for temporary node storage and lookup
Dictionary<int, SiteMapNode> nodes = new Dictionary<int, SiteMapNode> (16);
// Query the database for site map nodes
using(SqlConnection connection = new SqlConnection (ConfigurationManager.ConnectionStrings[_connect].ConnectionString))
{
connection.Open ();
SqlCommand command = new SqlCommand ("SELECT ID, Title, Description,'~/Homepage.aspx?pgid='+ Url as Url, Roles, Parent FROM SiteMap ORDER BY ID", connection);
SqlDataReader reader = command.ExecuteReader ();
int id = reader.GetOrdinal("ID");
int url = reader.GetOrdinal ("Url");
int title = reader.GetOrdinal ("Title");
int desc = reader.GetOrdinal("Description");
int roles = reader.GetOrdinal ("Roles");
int parent = reader.GetOrdinal("Parent");
if (reader.Read())
{
// Create the root SiteMapNode
_root = new SiteMapNode(this, reader.GetInt32(id).ToString(), reader.IsDBNull(url) ? null : reader.GetString(url),
reader.GetString(title), reader.IsDBNull(desc) ? null : reader.GetString(desc));
if (!reader.IsDBNull(roles))
{
string rolenames = reader.GetString(roles).Trim ();
if (!String.IsNullOrEmpty(rolenames))
{
string[] rolelist = rolenames.Split(new char[] { ',', ';' }, 512);
_root.Roles = rolelist;
}
}
// Add "*" to the roles list if no roles are specified
if (_root.Roles == null)
_root.Roles = new string[] { "*" };
// Record the root node in the dictionary
if (nodes.ContainsKey (reader.GetInt32(id)))
throw new ConfigurationErrorsException(_errmsg2); // ConfigurationException pre-Beta 2
nodes.Add(reader.GetInt32(id), _root);
// Add the node to the site map
AddNode(_root, null);
// Build a tree of SiteMapNodes underneath the root node
while (reader.Read())
{
SiteMapNode node = new SiteMapNode(this, reader.GetInt32(id).ToString(), reader.IsDBNull(url) ? null : reader.GetString(url),
reader.GetString(title), reader.IsDBNull(desc) ? null : reader.GetString(desc));
if (!reader.IsDBNull(roles))
{
string rolenames = reader.GetString(roles).Trim ();
if (!String.IsNullOrEmpty(rolenames))
{
string[] rolelist = rolenames.Split(new char[] { ',', ';' }, 512);
node.Roles = rolelist;
}
}
// If the node lacks roles information, "inherit" that
// information from its parent
SiteMapNode parentnode = nodes[reader.GetInt32(parent)];
if (node.Roles == null)
node.Roles = parentnode.Roles;
// Record the node in the dictionary
if (nodes.ContainsKey(reader.GetInt32(id)))
throw new ConfigurationErrorsException(_errmsg2);
nodes.Add(reader.GetInt32(id), node);
// Add the node to the site map
AddNode(node, parentnode);
}
}
}
// Return the root SiteMapNode
return _root;
}
protected override SiteMapNode GetRootNodeCore ()
{
BuildSiteMap ();
return _root;
}
}
Tag : - - - -
|
|
 |
 |
 |
 |
Date :
2009-07-07 11:45:59 |
By :
keyte |
View :
3497 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |