 |
|
คือ ผมดึงข้อมูลจากดาต้าเบส อีก ถัง หนึ่ง มาบันทึกลงอีก ถังหนึงอะครับ แต่ว่า พอรันแล้ว ข้อมุลมันไม่ยอม insert ให้อะครับ มันวิ่งข้ามไปที่ update เลย แล้วพอเช้ค ที่database ก็ไม่มีข้อมูลครับ รบกวนพี่ๆช่วยดูทีครับ
Code (VB.NET)
Imports System
Imports System.Data
Imports System.Data.SqlClient
Imports System.IO
Imports System.Text
Imports System.Data.OleDb
Public Class uBranch
Inherits uBaseClass
Private Sub Internalloaddata()
Dim dt As New DataTable
Dim _strQuery As New StringBuilder
_strQuery.Append(" select COMP_ID as COMP_ID,COMP_CODE as COMP_CODE,COMP_NAME as COMP_NAME,COMP_ADDRESS1 AS COMP_ADDRESS1 ,COMP_ADDRESS2 as COMP_ADDRESS2,COMP_TEL as COMP_TEL,COMP_FAX as COMP_FAX,COMP_REGISTRATIONNO as COMP_REGISTRATIONNO,COMP_TAXNO as COMP_TAXNO,COMP_CREATEDATE as COMP_CREATEDATE,COMP_LASTUPDATE as COMP_LASTUPDATE,COMP_CREATEDBY as COMP_CREATEDBY,COMP_UPDATEDBY as COMP_UPDATEDBY,")
_strQuery.Append(" COMP_STATUS as COMP_STATUS from COMP")
Console.WriteLine("COMP data start ...")
dt = uProcessData.QueryData_Str(uConnect.conn, _strQuery.ToString)
Console.WriteLine("COMP data Query Data ...")
If Not dt Is Nothing Then
If dt.Rows.Count > 0 Then
Console.WriteLine("COMP data have Data ...")
SaveData(dt)
Else
Console.WriteLine("COMP data not have Data ...")
End If
Else
Console.WriteLine("COMP data not have Data ...")
End If
End Sub
Sub New()
Internalloaddata()
End Sub
Private Sub SaveData(ByVal dt As DataTable)
For i As Integer = 0 To dt.Rows.Count - 1
If InsertData(dt, i) = False Then
Updatedata(dt, i)
End If
Next
End Sub
Private Function InsertData(ByVal dt As DataTable, ByVal Arow As Integer) As Boolean
Dim _strQuery As New StringBuilder
Dim COMP_ID As String = dt.Rows(Arow)("COMP_ID").ToString
Dim COMP_CODE As String = dt.Rows(Arow)("COMP_CODE").ToString
Dim COMP_NAME As String = dt.Rows(Arow)("COMP_NAME").ToString
Dim COMP_ADDRESS1 As String = dt.Rows(Arow)("COMP_ADDRESS1").ToString
Dim COMP_ADDRESS2 As String = dt.Rows(Arow)("COMP_ADDRESS2").ToString
Dim COMP_TEL As String = dt.Rows(Arow)("COMP_TEL").ToString
Dim COMP_FAX As String = dt.Rows(Arow)("COMP_FAX").ToString
Dim COMP_REGISTRATIONNO As String = dt.Rows(Arow)("COMP_REGISTRATIONNO").ToString
Dim COMP_TAXNO As String = dt.Rows(Arow)("COMP_TAXNO").ToString
Dim COMP_CREATEDATE As String = dt.Rows(Arow)("COMP_CREATEDATE").ToString
Dim COMP_LASTUPDATE As String = dt.Rows(Arow)("COMP_LASTUPDATE").ToString
Dim COMP_CREATEDBY As String = dt.Rows(Arow)("COMP_CREATEDBY").ToString
Dim COMP_UPDATEDBY As String = dt.Rows(Arow)("COMP_UPDATEDBY").ToString
Dim COMP_STATUS As String = dt.Rows(Arow)("COMP_STATUS").ToString
uConnectDestination.tr = uConnectDestination.conn.BeginTransaction
Try
_strQuery.Append(" insert into COMP")
_strQuery.Append(" (COMP_ID,COMP_CODE,COMP_NAME,COMP_ADDRESS1,COMP_ADDRESS2,COMP_TEL,COMP_FAX,COMP_REGISTRATIONNO,")
_strQuery.Append(" COMP_TAXNO,COMP_CREATEDATE,COMP_LASTUPDATE,COMP_CREATEDBY,COMP_UPDATEDBY,COMP_STATUS)")
_strQuery.Append(" values ")
_strQuery.Append("(")
_strQuery.Append("'").Append(COMP_ID).Append("',")
_strQuery.Append("'").Append(COMP_CODE).Append("',")
_strQuery.Append("'").Append(COMP_NAME).Append("',")
_strQuery.Append("'").Append(COMP_ADDRESS1).Append("',")
_strQuery.Append("'").Append(COMP_ADDRESS2).Append("',")
_strQuery.Append("'").Append(COMP_TEL).Append("',")
_strQuery.Append("'").Append(COMP_FAX).Append("',")
_strQuery.Append("'").Append(COMP_REGISTRATIONNO).Append("',")
_strQuery.Append("'").Append(COMP_TAXNO).Append("',")
_strQuery.Append("'").Append(COMP_CREATEDATE).Append("',")
_strQuery.Append("'").Append(COMP_LASTUPDATE).Append("',")
_strQuery.Append("'").Append(COMP_CREATEDBY).Append("',")
_strQuery.Append("'").Append(COMP_STATUS).Append("'")
_strQuery.Append(")")
uProcessData.ModifyData_Str(uConnectDestination.conn, uConnectDestination.tr, _
_strQuery.ToString)
uConnectDestination.tr.Commit()
Console.WriteLine("Insert New COMP @Row" & Arow + 1 & "/" & dt.Rows.Count)
Return True
Catch ex As Exception
uConnectDestination.tr.Rollback()
Return False
End Try
End Function
Private Function Updatedata(ByVal dt As DataTable, ByVal Arow As Integer) As Boolean
Dim _strQuery As New StringBuilder
Dim COMP_ID As String = dt.Rows(Arow)("COMP_ID").ToString
Dim COMP_CODE As String = dt.Rows(Arow)("COMP_CODE").ToString
Dim COMP_NAME As String = dt.Rows(Arow)("COMP_NAME").ToString
Dim COMP_ADDRESS1 As String = dt.Rows(Arow)("COMP_ADDRESS1").ToString
Dim COMP_ADDRESS2 As String = dt.Rows(Arow)("COMP_ADDRESS2").ToString
Dim COMP_TEL As String = dt.Rows(Arow)("COMP_TEL").ToString
Dim COMP_FAX As String = dt.Rows(Arow)("COMP_FAX").ToString
Dim COMP_REGISTRATIONNO As String = dt.Rows(Arow)("COMP_REGISTRATIONNO").ToString
Dim COMP_TAXNO As String = dt.Rows(Arow)("COMP_TAXNO").ToString
Dim COMP_CREATEDATE As String = dt.Rows(Arow)("COMP_CREATEDATE").ToString
Dim COMP_LASTUPDATE As String = dt.Rows(Arow)("COMP_LASTUPDATE").ToString
Dim COMP_CREATEDBY As String = dt.Rows(Arow)("COMP_CREATEDBY").ToString
Dim COMP_UPDATEDBY As String = dt.Rows(Arow)("COMP_UPDATEDBY").ToString
Dim COMP_STATUS As String = dt.Rows(Arow)("COMP_STATUS").ToString
uConnectDestination.tr = uConnectDestination.conn.BeginTransaction
Try
_strQuery.Append(" update COMP")
_strQuery.Append(" set ")
_strQuery.Append(" COMP_ID = '").Append(COMP_ID).Append("',")
_strQuery.Append(" COMP_CODE = '").Append(COMP_CODE).Append("',")
_strQuery.Append(" COMP_NAME = '").Append(COMP_NAME).Append("',")
_strQuery.Append(" COMP_ADDRESS1 = '").Append(COMP_ADDRESS1).Append("',")
_strQuery.Append(" COMP_ADDRESS2 = '").Append(COMP_ADDRESS2).Append("',")
_strQuery.Append(" COMP_TEL = '").Append(COMP_TEL).Append("',")
_strQuery.Append(" COMP_FAX = '").Append(COMP_FAX).Append("',")
_strQuery.Append(" COMP_REGISTRATIONNO = '").Append(COMP_REGISTRATIONNO).Append("',")
_strQuery.Append(" COMP_TAXNO = '").Append(COMP_TAXNO).Append("',")
_strQuery.Append(" COMP_CREATEDATE = '").Append(COMP_CREATEDATE).Append("',")
_strQuery.Append(" COMP_LASTUPDATE = '").Append(COMP_LASTUPDATE).Append("',")
_strQuery.Append(" COMP_CREATEDBY = '").Append(COMP_CREATEDBY).Append("',")
_strQuery.Append(" COMP_UPDATEDBY = '").Append(COMP_UPDATEDBY).Append("',")
_strQuery.Append(" COMP_STATUS = '").Append(COMP_STATUS).Append("'")
_strQuery.Append(" where COMP_ID = '").Append(COMP_ID).Append("'")
uProcessData.ModifyData_Str(uConnectDestination.conn, uConnectDestination.tr, _
_strQuery.ToString)
uConnectDestination.tr.Commit()
Console.WriteLine("Update COMP @Row" & Arow + 1 & "/" & dt.Rows.Count)
Return True
Catch ex As Exception
uConnectDestination.tr.Rollback()
Return False
End Try
End Function
End Class
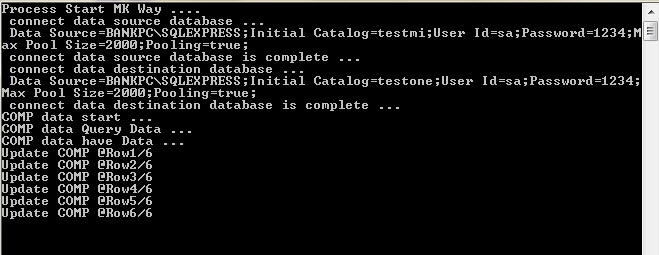
Tag : - - - -
|
|
 |
 |
 |
 |
Date :
2010-02-02 11:35:52 |
By :
songpons |
View :
1628 |
Reply :
4 |
|
 |
 |
 |
 |
|
|
|
 |