 |
|
ผมได้เขียนหน้าฟอรม์ให้ค้นหาข้อมูลอ่ะครับ เป็นหน้าฟอรม์โปรแกรมอะครับแล้ว
ข้อมูลมันไม่ขึ้นให้ แล้วตอนเขียนโค๊ดมันมี error ตรง e.row ด้วยควรแก้เป็นอะไรครับ
และผิดตรงไหนบ้างครับ
นี่คือหน้าที่ออกแบบครับ
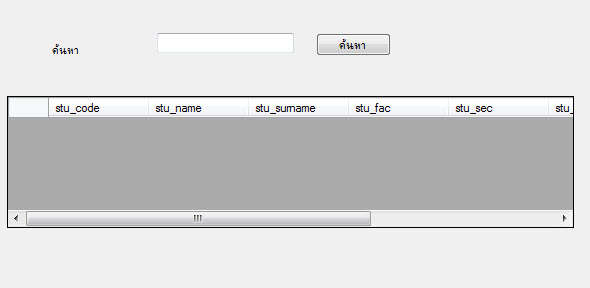
นี่คือ errorที่ code
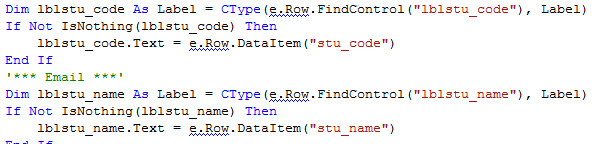
นี่คือผลลัพเวลากด ค้นหาคือไม่ขึ้นอะไรเลย
Code (VB.NET)
Imports System.Data
Imports System.Data.SqlClient
Public Class Form2
Dim strKeyWord As String
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
strKeyWord = Me.txtKeyWord.Text
End Sub
Sub BindData()
Dim objConn As New SqlConnection
Dim objCmd As New SqlCommand
Dim dtAdapter As New SqlDataAdapter
Dim ds As New DataSet
Dim strConnString, strSQL As String
strConnString = "Data Source=BANKPC\SQLEXPRESS;Initial Catalog=studen; User Id=sa;Password=1234"
strSQL = "SELECT * FROM stu WHERE (stu_code like '%" & strKeyWord & "%' OR stu_name like '%" & strKeyWord & "%') "
objConn.ConnectionString = strConnString
With objCmd
.Connection = objConn
.CommandText = strSQL
.CommandType = CommandType.Text
End With
dtAdapter.SelectCommand = objCmd
dtAdapter.Fill(ds)
'*** BindData to GridView ***'
DataGridView1.DataSource = ds
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
BindData()
End Sub
Private Sub Form2_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
'TODO: This line of code loads data into the 'StudenDataSet.stu' table. You can move, or remove it, as needed.
Me.StuTableAdapter.Fill(Me.StudenDataSet.stu)
End Sub
Private Sub DataGridView1_CellContentClick(ByVal sender As System.Object, ByVal e As System.Windows.Forms.DataGridViewCellEventArgs) Handles DataGridView1.CellContentClick
Dim lblstu_code As Label = CType(e.Row.FindControl("lblstu_code"), Label)
If Not IsNothing(lblstu_code) Then
lblstu_code.Text = e.Row.DataItem("stu_code")
End If
'*** Email ***'
Dim lblstu_name As Label = CType(e.Row.FindControl("lblstu_name"), Label)
If Not IsNothing(lblstu_name) Then
lblstu_name.Text = e.Row.DataItem("stu_name")
End If
'*** Name ***'
Dim lblstu_surname As Label = CType(e.Row.FindControl("lblstu_surname"), Label)
If Not IsNothing(lblstu_surname) Then
lblstu_surname.Text = e.Row.DataItem("stu_surname")
End If
'*** CountryCode ***'
Dim lblstu_fac As Label = CType(e.Row.FindControl("lblstu_fac"), Label)
If Not IsNothing(lblstu_fac) Then
lblstu_fac.Text = e.Row.DataItem("stu_fac")
End If
'*** Budget ***'
Dim lblstu_sec As Label = CType(e.Row.FindControl("lblstu_sec"), Label)
If Not IsNothing(lblstu_sec) Then
lblstu_sec.Text = e.Row.DataItem("stu_sec")
End If
'*** Used ***'
Dim lblstu_address As Label = CType(e.Row.FindControl("lblstu_address"), Label)
If Not IsNothing(lblstu_address) Then
lblstu_address.Text = e.Row.DataItem("stu_address")
End If
Dim lblgrade As Label = CType(e.Row.FindControl("lblgrade"), Label)
If Not IsNothing(lblgrade) Then
lblgrade.Text = e.Row.DataItem("grade")
End If
Dim lbldate As Label = CType(e.Row.FindControl("lbldate"), Label)
If Not IsNothing(lbldate) Then
lbldate.Text = e.Row.DataItem("date")
End If
End Sub
End Class
Tag : - - - -
|
|
 |
 |
 |
 |
Date :
2010-02-23 17:09:09 |
By :
songpons |
View :
1410 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |