 |
|
ช่วยด้วยครับ้รื่อง radio button c# winapp ต้องการดึงข้อมูลจากดาต้าเบส sqlserver 2005 ตาราง ProductType |
|
 |
|
|
 |
 |
|
Design
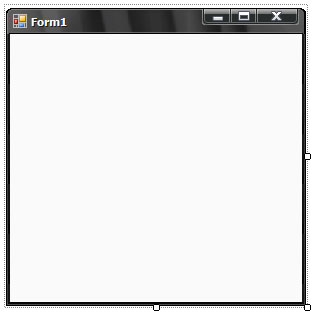
Database
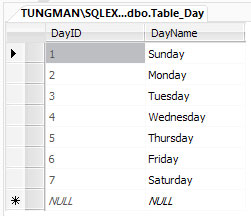
Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace MyRadioButton
{
public partial class Form1 : Form
{
private SqlDatabaseManager SqlDatabaseManager1;
private List<RadioButton> RadioArray;
public Form1()
{
InitializeComponent();
RadioArray = new List<RadioButton>();
SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.ConnectionString = "Data Source=.\\SQLEXPRESS;Initial Catalog=SqlDatabase;Integrated Security=True";
}
private void Form1_Load(object sender, EventArgs e)
{
DataTable Dt = new DataTable();
SqlDatabaseManager1.CommandString = "Select [DayID], [DayName] From [Table_Day]";
Dt = SqlDatabaseManager1.ExecuteQuery();
int point_y = 0;
foreach (DataRow Dr in Dt.Rows)
{
RadioButton aRadioButton = new RadioButton();
aRadioButton.Text = Dr["DayName"].ToString();
aRadioButton.Location = new Point(10, 10 + point_y);
this.Controls.Add(aRadioButton);
point_y += 20;
}
}
}
}
SqlDatabaseManager.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using System.Globalization;
using System.IO;
using System.Text.RegularExpressions;
namespace MyRadioButton
{
#region ========== Class SqlDatabaseManager ==========
/// <summary>
/// Summary description for SqlDatabase
/// </summary>
class SqlDatabaseManager
{
#region ========== Global Variables ==========
private SqlConnection sqlConnection;
private SqlCommand sqlCommand;
private SqlTransaction sqlTransaction;
private List<string> errorCommand;
private string sqlConnectionString = string.Empty;
private string sqlCommandString = string.Empty;
private string commandMessage = "You must execute command.";
private bool commandSuccess = false;
private bool transaction = false;
private int rowsAffected = 0;
#endregion
#region ========= Constructor ==========
/// <summary>
/// Use sql connection string from web.config configulation.
/// </summary>
public SqlDatabaseManager()
{
sqlConnection = new SqlConnection();
}
/// <summary>
/// Use sql connection string by user define.
/// </summary>
public SqlDatabaseManager(string SqlConnectionString)
{
sqlConnectionString = SqlConnectionString;
sqlConnection = new SqlConnection(sqlConnectionString);
}
#endregion
#region ========== Property ==========
/// <summary>
/// Gets or sets Sql connection.
/// </summary>
public virtual string ConnectionString
{
get { return sqlConnectionString; }
set
{
sqlConnectionString = value;
sqlConnection = new SqlConnection(sqlConnectionString);
}
}
/// <summary>
/// Gets or sets Sql command.
/// </summary>
public virtual string CommandString
{
get { return sqlCommandString; }
set
{
sqlCommandString = ConvertDateCommand(value);
sqlCommand = new SqlCommand(sqlCommandString, sqlConnection);
commandMessage = "You must execute command.";
commandSuccess = false;
rowsAffected = 0;
if (transaction)
sqlCommand.Transaction = sqlTransaction;
}
}
/// <summary>
/// Check for Sql command.
/// </summary>
public virtual bool IsSuccess
{
get { return commandSuccess; }
}
/// <summary>
/// Gets message from Sql command.
/// </summary>
public virtual string Message
{
get { return commandMessage; }
}
/// <summary>
/// Gets Number of rows affected.
/// </summary>
public virtual int RowsAffected
{
get { return rowsAffected; }
}
#endregion
#region ========== Method ==========
/// <summary>
/// Add the parameter value to the sql command.
/// </summary>
/// <param name="ParameterName">The name of Parameter.</param>
/// <param name="ParameterValue">The value to be added.</param>
public virtual void AddParameter(string ParameterName, object ParameterValue)
{
sqlCommand.Parameters.AddWithValue(ParameterName, ParameterValue);
}
/// <summary>
/// Start Sql Transaction.
/// </summary>
public virtual void TransactionStart()
{
transaction = true;
errorCommand = new List<string>();
if (sqlConnection.State != ConnectionState.Open)
sqlConnection.Open();
sqlTransaction = sqlConnection.BeginTransaction(IsolationLevel.ReadCommitted);
}
/// <summary>
/// Execute Sql Transaction.
/// </summary>
/// <returns>Result of transaction.</returns>
public virtual bool ExecuteTransaction()
{
transaction = false;
if (errorCommand.Count == 0)
{
sqlTransaction.Commit();
commandMessage = "All command is successfully. Transaction Commited.";
commandSuccess = true;
}
else
{
sqlTransaction.Rollback();
string ErrorText = "Some command has error. Transaction RollBack. Error in: ";
foreach (string aErrorSqlCommand in errorCommand)
{
ErrorText += aErrorSqlCommand + "\n";
}
commandMessage = ErrorText;
commandSuccess = false;
}
errorCommand.Clear();
if (sqlConnection.State == ConnectionState.Open)
sqlConnection.Close();
sqlTransaction.Dispose();
sqlCommand.Dispose();
sqlConnection.Dispose();
return commandSuccess;
}
/// <summary>
/// Execute Query Sql command.
/// </summary>
/// <returns>Query data in DataTable.</returns>
public virtual DataTable ExecuteQuery()
{
DataTable dataTable = new DataTable();
try
{
SqlDataAdapter sqlDataAdapter = new SqlDataAdapter(sqlCommand);
sqlDataAdapter.Fill(dataTable);
sqlDataAdapter.Dispose();
if (!transaction)
{
sqlCommand.Dispose();
sqlConnection.Dispose();
}
commandMessage = "Command is successfully.";
commandSuccess = true;
}
catch (Exception ex)
{
commandMessage = ErrorMessage(ex.Message);
commandSuccess = false;
}
rowsAffected = dataTable.Rows.Count;
return dataTable;
}
/// <summary>
/// Execute Scalar Sql command.
/// </summary>
/// <returns>Object of value.</returns>
public virtual object ExecuteScalar()
{
object Result = 0;
try
{
if (transaction)
{
Result = sqlCommand.ExecuteScalar();
}
else
{
if (sqlConnection.State != ConnectionState.Open)
sqlConnection.Open();
Result = sqlCommand.ExecuteScalar();
sqlConnection.Close();
sqlCommand.Dispose();
sqlConnection.Dispose();
}
commandMessage = "Command is successfully.";
commandSuccess = true;
}
catch (Exception ex)
{
commandMessage = ErrorMessage(ex.Message);
commandSuccess = false;
AddErrorCommand(sqlCommandString, ex.Message);
}
return Result;
}
/// <summary>
/// Execute Non Query Sql command.
/// </summary>
/// <returns>Result of execute command.</returns>
public virtual bool ExecuteNonQuery()
{
rowsAffected = 0;
try
{
if (transaction)
{
rowsAffected = sqlCommand.ExecuteNonQuery();
}
else
{
if (sqlConnection.State != ConnectionState.Open)
sqlConnection.Open();
rowsAffected = sqlCommand.ExecuteNonQuery();
sqlConnection.Close();
sqlCommand.Dispose();
sqlConnection.Dispose();
}
commandMessage = "Command is successfully.";
commandSuccess = true;
}
catch (Exception ex)
{
commandMessage = ErrorMessage(ex.Message);
commandSuccess = false;
AddErrorCommand(sqlCommandString, ex.Message);
}
return commandSuccess;
}
/// <summary>
/// Build error message.
/// </summary>
/// <param name="Message">Message string.</param>
/// <returns>Error message string.</returns>
public virtual string ErrorMessage(string MessageString)
{
return "Command error. " + MessageString;
}
/// <summary>
/// Add error sql command to string collections.
/// </summary>
/// <param name="commandString">The sql command.</param>
/// <param name="errorMessage">The error message.</param>
private void AddErrorCommand(string commandString, string errorMessage)
{
errorCommand.Add(commandString + " [Error message: " + errorMessage + "]");
}
/// <summary>
/// Convert native command to sql command.
/// </summary>
/// <param name="commandString">The native sql command.</param>
/// <returns>The standard sql command.</returns>
private string ConvertDateCommand(string commandString)
{
string SmallDateTimePattern = "[sS][mM][aA][lL][lL][dD][aA][tT][eE][tT][iI][mM][eE]\\([@][0-9a-zA-Z\\s]{1,}\\)";
Regex SmallDateTimeRgx = new Regex(SmallDateTimePattern);
foreach (Match SmallDateTimeMatchCase in SmallDateTimeRgx.Matches(commandString))
{
string MatchCasePattern = "^[sS][mM][aA][lL][lL][dD][aA][tT][eE][tT][iI][mM][eE]";
Regex MatchCaseRgx = new Regex(MatchCasePattern);
Match RemoveMatch = MatchCaseRgx.Match(SmallDateTimeMatchCase.Value);
string TempMatchCase = SmallDateTimeMatchCase.Value.Replace(RemoveMatch.Value, "");
commandString = commandString.Replace(SmallDateTimeMatchCase.Value, TempMatchCase.Replace("(", "Convert(SmallDateTime, ").Replace(")", ", 103)"));
}
string DateTimePattern = "[dD][aA][tT][eE][tT][iI][mM][eE]\\([@][0-9a-zA-Z\\s]{1,}\\)";
Regex DateTimeRgx = new Regex(DateTimePattern);
foreach (Match DateTimeMatchCase in DateTimeRgx.Matches(commandString))
{
string MatchCasePattern = "^[dD][aA][tT][eE][tT][iI][mM][eE]";
Regex MatchCaseRgx = new Regex(MatchCasePattern);
Match RemoveMatch = MatchCaseRgx.Match(DateTimeMatchCase.Value);
string TempMatchCase = DateTimeMatchCase.Value.Replace(RemoveMatch.Value, "");
commandString = commandString.Replace(DateTimeMatchCase.Value, TempMatchCase.Replace("(", "Convert(DateTime, ").Replace(")", ", 103)"));
}
return commandString;
}
#endregion
}
#endregion
#region ========== Class SqlVarBinary ==========
/// <summary>
/// Summary description for BinaryData
/// </summary>
public class SqlVarBinary
{
public SqlVarBinary()
{
//
// TODO: Add constructor logic here
//
}
/// <summary>
/// Convert to byte[].
/// </summary>
public static byte[] Convert(Stream BinaryStream, int StreamLength)
{
BinaryReader BinaryRead = new BinaryReader(BinaryStream);
byte[] binaryData = BinaryRead.ReadBytes(StreamLength);
return binaryData;
}
}
#endregion
#region ========== Class SqlDateTime ==========
/// <summary>
/// Summary description for SqlDateTime
/// </summary>
public class SqlDateTime
{
public SqlDateTime()
{
//
// TODO: Add constructor logic here
//
}
/// <summary>
/// Convert to DataTime DataType with d/M/yyyy format.
/// </summary>
public static DateTime Convert(string DateString)
{
return DateTime.ParseExact(DateString, "d/M/yyyy", CultureInfo.InvariantCulture);
}
/// <summary>
/// Convert to DataTime DataType with user define format.
/// </summary>
public static DateTime Convert(string DateString, string DateFormat)
{
return DateTime.ParseExact(DateString, DateFormat, CultureInfo.InvariantCulture);
}
}
#endregion
}
Run
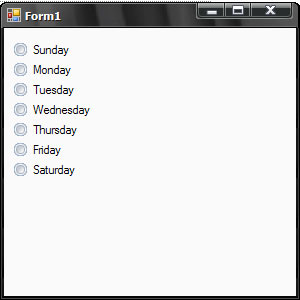
|
 |
 |
 |
 |
Date :
2010-03-29 21:19:23 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แก้ใหม่ ลืมอ่านโจทย์
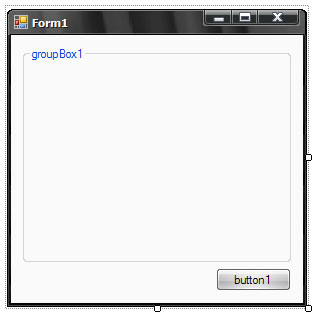
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace MyRadioButton
{
public partial class Form1 : Form
{
private SqlDatabaseManager SqlDatabaseManager1;
private List<RadioButton> RadioArray;
public Form1()
{
InitializeComponent();
RadioArray = new List<RadioButton>();
SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.ConnectionString = "Data Source=.\\SQLEXPRESS;Initial Catalog=SqlDatabase;Integrated Security=True";
}
private void Form1_Load(object sender, EventArgs e)
{
DataTable Dt = new DataTable();
SqlDatabaseManager1.CommandString = "Select [DayID], [DayName] From [Table_Day]";
Dt = SqlDatabaseManager1.ExecuteQuery();
int point_y = 0;
foreach (DataRow Dr in Dt.Rows)
{
RadioButton aRadioButton = new RadioButton();
aRadioButton.Name = "Day" + Dr["DayID"].ToString();
aRadioButton.Text = Dr["DayName"].ToString();
aRadioButton.Location = new Point(10, 20 + point_y);
groupBox1.Controls.Add(aRadioButton);
point_y += 25;
RadioArray.Add(aRadioButton);
}
}
private void button1_Click(object sender, EventArgs e)
{
int IsChecked = 0;
foreach (RadioButton aRadioButton in RadioArray)
{
if (aRadioButton.Checked == true)
{
MessageBox.Show("You choose: " + aRadioButton.Text, "Message", MessageBoxButtons.OK, MessageBoxIcon.Information);
IsChecked++;
}
}
if (IsChecked == 0)
MessageBox.Show("You must choose radio button", "Message", MessageBoxButtons.OK, MessageBoxIcon.Asterisk);
}
}
}
หวังว่าเขียน sql command สำหรับ insert data ลง database ได้นะ
|
 |
 |
 |
 |
Date :
2010-03-29 22:02:30 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เดี๋ยวลองดูครับขอบพระคุณมากเลยครับ
|
 |
 |
 |
 |
Date :
2010-03-29 23:51:01 |
By :
noknok |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ให้คะแนนยังไงหว่า
|
 |
 |
 |
 |
Date :
2010-03-29 23:51:41 |
By :
noknok |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
error SqlDatabaseManager1 = new SqlDatabaseManager(); ครับ
และจะดึงข้อมูลที่เก็บไปมาโชว์ไงครับ
|
 |
 |
 |
 |
Date :
2010-03-30 01:26:51 |
By :
noknok |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พระคุณนี้จะไม่ลืม ขออีเมล์ด้วยครับ เดวแจกโค้ดร้อยจอ
|
 |
 |
 |
 |
Date :
2010-03-30 01:39:46 |
By :
noknok |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ช่วยอีกนิดน้า
|
 |
 |
 |
 |
Date :
2010-03-30 11:56:53 |
By :
noknok |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
และจะดึงข้อมูลที่เก็บ เป็น 12345 แยกแล้ว check ตาม ไอดี มาโชว์ยังไงครับ
|
 |
 |
 |
 |
Date :
2010-03-30 12:52:40 |
By :
noknok |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|