|
 |
|
[c# win] มันอะไรกันนักหนาเนี่ย image + picturebox เนี่ย |
|
 |
|
|
 |
 |
|
1. สร้างโปรเจ็คใหม่เป็น win app + c# ตั้งชื่อว่า ImageGallery
2. เปิดไฟล์ชื่อ Form1.Designer.cs แล้วเอาโค้ดด้านล่างไปแปะ
Form1.Designer.cs
namespace ImageGallery
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.ImageListBox = new System.Windows.Forms.ListBox();
this.PictureBox1 = new System.Windows.Forms.PictureBox();
this.AddButton = new System.Windows.Forms.Button();
this.DeleteButton = new System.Windows.Forms.Button();
((System.ComponentModel.ISupportInitialize)(this.PictureBox1)).BeginInit();
this.SuspendLayout();
//
// ImageListBox
//
this.ImageListBox.FormattingEnabled = true;
this.ImageListBox.Location = new System.Drawing.Point(13, 42);
this.ImageListBox.Name = "ImageListBox";
this.ImageListBox.Size = new System.Drawing.Size(156, 264);
this.ImageListBox.TabIndex = 0;
this.ImageListBox.SelectedIndexChanged += new System.EventHandler(this.ImageListBox_SelectedIndexChanged);
//
// PictureBox1
//
this.PictureBox1.Location = new System.Drawing.Point(175, 13);
this.PictureBox1.Name = "PictureBox1";
this.PictureBox1.Size = new System.Drawing.Size(305, 293);
this.PictureBox1.TabIndex = 1;
this.PictureBox1.TabStop = false;
this.PictureBox1.Click += new System.EventHandler(this.PictureBox1_Click);
//
// AddButton
//
this.AddButton.Location = new System.Drawing.Point(13, 13);
this.AddButton.Name = "AddButton";
this.AddButton.Size = new System.Drawing.Size(75, 23);
this.AddButton.TabIndex = 2;
this.AddButton.Text = "Add";
this.AddButton.UseVisualStyleBackColor = true;
this.AddButton.Click += new System.EventHandler(this.AddButton_Click);
//
// DeleteButton
//
this.DeleteButton.Location = new System.Drawing.Point(94, 13);
this.DeleteButton.Name = "DeleteButton";
this.DeleteButton.Size = new System.Drawing.Size(75, 23);
this.DeleteButton.TabIndex = 3;
this.DeleteButton.Text = "Delete";
this.DeleteButton.UseVisualStyleBackColor = true;
this.DeleteButton.Click += new System.EventHandler(this.DeleteButton_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(494, 320);
this.Controls.Add(this.DeleteButton);
this.Controls.Add(this.AddButton);
this.Controls.Add(this.PictureBox1);
this.Controls.Add(this.ImageListBox);
this.Name = "Form1";
this.Text = "Image Gallery";
this.Load += new System.EventHandler(this.Form1_Load);
((System.ComponentModel.ISupportInitialize)(this.PictureBox1)).EndInit();
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.ListBox ImageListBox;
private System.Windows.Forms.PictureBox PictureBox1;
private System.Windows.Forms.Button AddButton;
private System.Windows.Forms.Button DeleteButton;
}
}
|
 |
 |
 |
 |
Date :
2010-06-03 08:19:41 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
3. เปิดไฟล์ชื่อ Form1.cs แล้วเอาโค้ดด้านล่างไปแปะ
Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
namespace ImageGallery
{
public partial class Form1 : Form
{
private const string SecretKey = "www.thaicreate.com";
private string ImageDirectoryPath;
private DataTable DtImage;
public Form1()
{
InitializeComponent();
ImageDirectoryPath = string.Format("{0}\\Images", Application.StartupPath);
DtImage = new DataTable();
DtImage.Columns.Add(new DataColumn("ImageName", System.Type.GetType("System.String")));
DtImage.Columns.Add(new DataColumn("ImagePath", System.Type.GetType("System.String")));
}
private void Form1_Load(object sender, EventArgs e)
{
DirectoryInfo ImageDirectory = new DirectoryInfo(ImageDirectoryPath);
ImageListBox.DisplayMember = "ImageName";
if (ImageDirectory.Exists)
{
foreach (FileInfo imageFile in ImageDirectory.GetFiles())
{
string ImageName = imageFile.Name.Substring(0, imageFile.Name.Length - 10);
string ImagePath = string.Format("{0}\\{1}", ImageDirectoryPath, imageFile.Name);
DataRow Dr = DtImage.NewRow();
Dr["ImageName"] = ImageName;
Dr["ImagePath"] = ImagePath;
DtImage.Rows.Add(Dr);
}
ImageListBox.DataSource = DtImage;
if (DtImage.Rows.Count > 0)
DisplayImage(DtImage.Rows[0]["ImagePath"].ToString());
}
}
private void AddButton_Click(object sender, EventArgs e)
{
OpenFileDialog openImageDialog = new OpenFileDialog();
openImageDialog.FileName = string.Empty;
openImageDialog.Filter = "Image Files (*.gif;*.jpg;*.png;*.bmp)|*.gif;*.jpg;*.png;*.bmp";
openImageDialog.FilterIndex = 1;
openImageDialog.RestoreDirectory = true;
openImageDialog.Multiselect = false;
if (openImageDialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
if (DtImage.Select(string.Format("ImageName Like '{0}'", openImageDialog.SafeFileName)).Count() == 0)
{
string OutputFilePath = string.Format("{0}\\{1}.encrypted", ImageDirectoryPath, openImageDialog.SafeFileName);
DirectoryInfo ImageDirectory = new DirectoryInfo(ImageDirectoryPath);
if (!ImageDirectory.Exists)
ImageDirectory.Create();
Security.EncryptFile(openImageDialog.FileName, OutputFilePath, SecretKey);
DataRow Dr = DtImage.NewRow();
Dr["ImageName"] = openImageDialog.SafeFileName;
Dr["ImagePath"] = OutputFilePath;
DtImage.Rows.Add(Dr);
ImageListBox.DataSource = DtImage;
DisplayImage(DtImage.Rows[ImageListBox.SelectedIndex]["ImagePath"].ToString());
}
else
{
MessageBox.Show("ไม่สามารถเพิ่มไฟล์ได้เนื่องจากมีไฟล์นี้แล้ว", "ผิดพลาด", MessageBoxButtons.OK, MessageBoxIcon.Asterisk);
}
}
}
private void DeleteButton_Click(object sender, EventArgs e)
{
string ImagePath = DtImage.Rows[ImageListBox.SelectedIndex]["ImagePath"].ToString();
FileInfo imageFile = new FileInfo(ImagePath);
if (imageFile.Exists)
{
imageFile.Delete();
DtImage.Rows[ImageListBox.SelectedIndex].Delete();
DtImage.AcceptChanges();
ImageListBox.DataSource = DtImage;
if (ImageListBox.SelectedIndex >= 0)
DisplayImage(DtImage.Rows[ImageListBox.SelectedIndex]["ImagePath"].ToString());
else
PictureBox1.Image = null;
MessageBox.Show("ลบไฟล์รูปภาพนี้เรียบร้อยแล้ว", "ลบไฟล์", MessageBoxButtons.OK, MessageBoxIcon.None);
}
else
{
MessageBox.Show("ไม่สามารถลบไฟล์นี้ได้", "ผิดพลาด", MessageBoxButtons.OK, MessageBoxIcon.Asterisk);
}
}
private void ImageListBox_SelectedIndexChanged(object sender, EventArgs e)
{
if (ImageListBox.SelectedIndex >= 0)
DisplayImage(DtImage.Rows[ImageListBox.SelectedIndex]["ImagePath"].ToString());
else
PictureBox1.Image = null;
}
private void PictureBox1_Click(object sender, EventArgs e)
{
if (PictureBox1.Image != null)
{
byte[] imageBytes = Security.DecryptStream(DtImage.Rows[ImageListBox.SelectedIndex]["ImagePath"].ToString(), SecretKey);
Image myImage = Image.FromStream(new MemoryStream(imageBytes));
ViewForm viewForm = new ViewForm(myImage);
viewForm.ShowDialog();
}
}
private void DisplayImage(string ImagePath)
{
byte[] imageBytes = Security.DecryptStream(ImagePath, SecretKey);
Image myImage = Image.FromStream(new MemoryStream(imageBytes));
Size fitImageSize = ScaledImageDimensions(myImage.Width, myImage.Height, PictureBox1.Width, PictureBox1.Height);
Bitmap imgOutput = new Bitmap(myImage, fitImageSize.Width, fitImageSize.Height);
PictureBox1.Image = null;
PictureBox1.SizeMode = PictureBoxSizeMode.CenterImage;
PictureBox1.Image = imgOutput;
}
private Size ScaledImageDimensions(int currentImageWidth, int currentImageHeight, int desiredImageWidth, int desiredImageHeight)
{
/* First, we must calculate a multiplier that will be used
* to get the dimensions of the new, scaled image. */
double scaleImageMultiplier = 0;
/* This multiplier is defined as the ratio of the
* Desired Dimension to the Current Dimension.
* Specifically which dimension is used depends on the larger
* dimension of the image, as this will be the constraining dimension
* when we fit to the window. */
/* Determine if Image is Portrait or Landscape. */
if (currentImageHeight > currentImageWidth) /* Image is Portrait */
{
/* Calculate the multiplier based on the heights. */
if (desiredImageHeight > desiredImageWidth)
{
scaleImageMultiplier = (double)desiredImageWidth / (double)currentImageWidth;
}
else
{
scaleImageMultiplier = (double)desiredImageHeight / (double)currentImageHeight;
}
}
else /* Image is Landscape */
{
/* Calculate the multiplier based on the widths. */
if (desiredImageWidth > desiredImageHeight)
{
scaleImageMultiplier = (double)desiredImageWidth / (double)currentImageWidth;
}
else
{
scaleImageMultiplier = (double)desiredImageHeight / (double)currentImageHeight;
}
}
/* Generate and return the new scaled dimensions.
* Essentially, we multiply each dimension of the original image
* by the multiplier calculated above to yield the dimensions
* of the scaled image. The scaled image can be larger or smaller
* than the original. */
int outputWidth = (int)(currentImageWidth * scaleImageMultiplier);
int outputHight = (int)(currentImageHeight * scaleImageMultiplier);
return new Size((currentImageWidth > outputWidth) ? outputWidth : currentImageWidth, (currentImageHeight > outputHight) ? outputHight : currentImageHeight);
}
}
}
|
 |
 |
 |
 |
Date :
2010-06-03 08:20:33 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
4. คลิกขวาที่ชื่อโปรเจ็ค ใน solution explorer เลือก add new item สร้าง class ใหม่ชื่อ Security.cs ก็อบโค้ดด้านล่างไปแปะ
Security.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.IO;
using System.Runtime.InteropServices;
using System.Security.Cryptography;
using System.Text;
/// <summary>
/// Summary description for Security
/// </summary>
public class Security
{
/// <summary>
/// สำหรับเข้ารหัส และถอดรหัส
/// </summary>
public Security()
{
//
// TODO: Add constructor logic here
//
}
/// <summary>
/// สร้าง Code สำหรับเปลี่ยนแปลงข้อมูลเพื่อความปลอดภัย
/// </summary>
/// <param name="EncryptString">ข้อความที่ต้องการเข้ารหัส</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>ข้อความที่เข้ารหัสแล้ว</returns>
public static string EncryptString(string EncryptString, string SecretKey)
{
MemoryStream msEncrypt = new MemoryStream();
DESCryptoServiceProvider DES = new DESCryptoServiceProvider();
string secretKey = (SecretKey.Length == 8) ? SecretKey : MD5(SecretKey);
// Key ที่ต้องใช้งานกันทั้งสองฝ่าย ข้อมูลลับ
DES.Key = ASCIIEncoding.ASCII.GetBytes(secretKey);
DES.IV = ASCIIEncoding.ASCII.GetBytes(secretKey);
// ใช้ CreateEncryptor ในการเข้ารหัส
ICryptoTransform myEncryptor = DES.CreateEncryptor();
// ตัวแปร array สำหรับรับข้อความที่ต้องการเข้ารหัส ต้องแปลงเป็น ASCII
byte[] pwd = ASCIIEncoding.ASCII.GetBytes(EncryptString);
// เข้ารหัสข้อมูล ข้อมูลที่เข้ารหัสเรียบร้อยแล้วจะเก็บไว้ที่ msEncrypt
CryptoStream myCryptoStream = new CryptoStream(msEncrypt, myEncryptor, CryptoStreamMode.Write);
myCryptoStream.Write(pwd, 0, pwd.Length);
myCryptoStream.Close();
// ส่งค่าที่ encrypt แล้วกลับไป
return Convert.ToBase64String(msEncrypt.ToArray());
}
/// <summary>
/// ถอดรหัส Code
/// </summary>
/// <param name="DecryptString">ข้อความที่ต้องการถอดรหัส</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>ข้อความที่ถอดรหัสแล้ว</returns>
public static string DecryptString(string DecryptString, string SecretKey)
{
MemoryStream msDecrypt = new MemoryStream();
DESCryptoServiceProvider DES = new DESCryptoServiceProvider();
string secretKey = (SecretKey.Length == 8) ? SecretKey : MD5(SecretKey);
// Key ที่ต้องใช้งานกันทั้งสองฝ่าย ข้อมูลลับ
DES.Key = ASCIIEncoding.ASCII.GetBytes(secretKey);
DES.IV = ASCIIEncoding.ASCII.GetBytes(secretKey);
// ใช้ CreateDecryptor ในการเข้าถอดรหัส
ICryptoTransform myDecryptor = DES.CreateDecryptor();
// ตัวแปร array สำหรับรับข้อความที่ต้องการถอดรหัส ไม่ต้องแปลงเป็น ASCII
byte[] pwd = Convert.FromBase64String(DecryptString);
// เข้ารหัสข้อมูล ข้อมูลที่เข้ารหัสเรียบร้อยแล้วจะเก็บไว้ที่ msDecrypt
CryptoStream cCryptoStream = new CryptoStream(msDecrypt, myDecryptor, CryptoStreamMode.Write);
cCryptoStream.Write(pwd, 0, pwd.Length);
cCryptoStream.Close();
// ส่งค่าที่ decrypt แล้วกลับไป ต้องแปลงจาก ASCII ให้กลับเป็น string ก่อน
return ASCIIEncoding.ASCII.GetString(msDecrypt.ToArray());
}
/// <summary>
/// เข้ารหัสเอกสาร โดยส่งออกมาเป็น Binary Stream
/// </summary>
/// <param name="InputFilePath">ชื่อเอกสารที่ต้องการเข้ารหัส</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>Binary Stream ที่เข้ารหัสแล้ว</returns>
public static byte[] EncryptStream(string InputFilePath, string SecretKey)
{
// read an file to new byte array
byte[] byteArray = File.ReadAllBytes(InputFilePath);
// write data to the new stream
MemoryStream memoryStream = new MemoryStream(byteArray);
return EncryptStream(memoryStream, SecretKey);
}
/// <summary>
/// เข้ารหัสเอกสาร โดยส่งออกมาเป็น Binary Stream
/// </summary>
/// <param name="BinaryStream">Stream ที่ต้องการเข้ารหัส</param>
/// <param name="StreamLength">ขนาดของ Stream</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>Binary Stream ที่เข้ารหัสแล้ว</returns>
public static byte[] EncryptStream(Stream BinaryStream, string SecretKey)
{
BinaryReader BinaryRead = new BinaryReader(BinaryStream);
byte[] binaryData = BinaryRead.ReadBytes(Convert.ToInt32(BinaryStream.Length));
string secretKey = (SecretKey.Length == 8) ? SecretKey : MD5(SecretKey);
//A 64 bit key and IV is required for this provider.
//Set secret key For DES algorithm.
DESCryptoServiceProvider DES = new DESCryptoServiceProvider();
DES.Key = ASCIIEncoding.ASCII.GetBytes(secretKey);
DES.IV = ASCIIEncoding.ASCII.GetBytes(secretKey);
//Create a DES decryptor from the DES instance.
ICryptoTransform desencrypt = DES.CreateEncryptor();
//Create crypto stream set to read and do a DES decryption transform on incoming bytes.
MemoryStream memoryStream = new MemoryStream();
CryptoStream cryptostream = new CryptoStream(memoryStream, desencrypt, CryptoStreamMode.Write);
cryptostream.Write(binaryData, 0, binaryData.Length);
cryptostream.FlushFinalBlock();
cryptostream.Close();
return memoryStream.ToArray();
}
/// <summary>
/// เข้ารหัสเอกสาร
/// </summary>
/// <param name="InputFilePath">ชื่อเอกสารที่ต้องการเข้ารหัส</param>
/// <param name="OutputFilePath">ชื่อเอกสารที่เข้ารหัสแล้ว</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
public static void EncryptFile(string InputFilePath, string OutputFilePath, string SecretKey)
{
//Create a encrypted file.
FileStream fsEncrypted = new FileStream(OutputFilePath, FileMode.Create, FileAccess.Write);
//Print the contents of the Encrypted file.
byte[] byteArray = EncryptStream(InputFilePath, SecretKey);
fsEncrypted.Write(byteArray, 0, byteArray.Length);
fsEncrypted.Close();
}
/// <summary>
/// เข้ารหัสเอกสาร
/// </summary>
/// <param name="BinaryStream">Stream ที่ต้องการเข้ารหัส</param>
/// <param name="StreamLength">ขนาดของ Stream</param>
/// <param name="OutputFilePath">ชื่อเอกสารที่เข้ารหัสแล้ว</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
public static void EncryptFile(Stream BinaryStream, string OutputFilePath, string SecretKey)
{
//Create a encrypted file.
FileStream fsEncrypted = new FileStream(OutputFilePath, FileMode.Create, FileAccess.Write);
//Print the contents of the Encrypted file.
byte[] byteArray = EncryptStream(BinaryStream, SecretKey);
fsEncrypted.Write(byteArray, 0, byteArray.Length);
fsEncrypted.Close();
}
/// <summary>
/// ถอดรหัสเอกสาร โดยส่งออกมาเป็น Binary Stream
/// </summary>
/// <param name="InputFilePath">ชื่อเอกสารที่ต้องการถอดรหัส</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>Binary Stream ที่ถอดรหัสแล้ว</returns>
public static byte[] DecryptStream(string InputFilePath, string SecretKey)
{
// read an file to new byte array
byte[] byteArray = File.ReadAllBytes(InputFilePath);
// write data to the new stream
MemoryStream memoryStream = new MemoryStream(byteArray);
return DecryptStream(memoryStream, SecretKey);
}
/// <summary>
/// ถอดรหัสเอกสาร โดยส่งออกมาเป็น Binary Stream
/// </summary>
/// <param name="BinaryStream">Stream ที่ต้องการถอดรหัส</param>
/// <param name="StreamLength">ขนาดของ Stream</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>Binary Stream ที่ถอดรหัสแล้ว</returns>
public static byte[] DecryptStream(Stream BinaryStream, string SecretKey)
{
BinaryReader BinaryRead = new BinaryReader(BinaryStream);
byte[] binaryData = BinaryRead.ReadBytes(Convert.ToInt32(BinaryStream.Length));
string secretKey = (SecretKey.Length == 8) ? SecretKey : MD5(SecretKey);
//A 64 bit key and IV is required for this provider.
//Set secret key For DES algorithm.
DESCryptoServiceProvider DES = new DESCryptoServiceProvider();
DES.Key = ASCIIEncoding.ASCII.GetBytes(secretKey);
DES.IV = ASCIIEncoding.ASCII.GetBytes(secretKey); //Set initialization vector.
//Create a DES decryptor from the DES instance.
ICryptoTransform desdecrypt = DES.CreateDecryptor();
//Create crypto stream set to read and do a DES decryption transform on incoming bytes.
MemoryStream memoryStream = new MemoryStream();
CryptoStream cryptostreamDecr = new CryptoStream(memoryStream, desdecrypt, CryptoStreamMode.Write);
cryptostreamDecr.Write(binaryData, 0, binaryData.Length);
cryptostreamDecr.FlushFinalBlock();
cryptostreamDecr.Close();
return memoryStream.ToArray();
}
/// <summary>
/// ถอดรหัสเอกสาร
/// </summary>
/// <param name="InputFilePath">ชื่อเอกสารที่ต้องการถอดรหัส</</param>
/// <param name="OutputFilePath">ชื่อเอกสารที่ถอดรหัสแล้ว</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
public static void DecryptFile(string InputFilePath, string OutputFilePath, string SecretKey)
{
//Create a file stream to read the encrypted file back.
FileStream fsDecrypted = new FileStream(OutputFilePath, FileMode.Create, FileAccess.Write);
//Print the contents of the Decrypted file.
byte[] byteArray = DecryptStream(InputFilePath, SecretKey);
fsDecrypted.Write(byteArray, 0, byteArray.Length);
fsDecrypted.Close();
}
/// <summary>
/// ถอดรหัสเอกสาร
/// </summary>
/// <param name="BinaryStream">Stream ที่ต้องการถอดรหัส</param>
/// <param name="StreamLength">ขนาดของ Stream</param>
/// <param name="OutputFilePath">ชื่อเอกสารที่ถอดรหัสแล้ว</param>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
public static void DecryptFile(Stream BinaryStream, string OutputFilePath, string SecretKey)
{
//Create a file stream to read the encrypted file back.
FileStream fsDecrypted = new FileStream(OutputFilePath, FileMode.Create, FileAccess.Write);
//Print the contents of the Decrypted file.
byte[] byteArray = DecryptStream(BinaryStream, SecretKey);
fsDecrypted.Write(byteArray, 0, byteArray.Length);
fsDecrypted.Close();
}
/// <summary>
/// สร้าง 64 bits Key สำหรับเข้ารหัสและถอดรหัส
/// </summary>
/// <returns>64 bits Key สำหรับเข้ารหัสและถอดรหัส</returns>
public static string GenerateKey()
{
// Create an instance of Symetric Algorithm. Key and IV is generated automatically.
DESCryptoServiceProvider desCrypto = (DESCryptoServiceProvider)DESCryptoServiceProvider.Create();
// Use the Automatically generated key for Encryption.
return ASCIIEncoding.ASCII.GetString(desCrypto.Key);
}
/// <summary>
/// ลบข้อมูล Key ออกจากหนวยความจำ
/// </summary>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
public static void RemoveKey(string SecretKey)
{
// For additional security Pin the key.
GCHandle gch = GCHandle.Alloc(SecretKey, GCHandleType.Pinned);
// Remove the Key from memory.
ZeroMemory(gch.AddrOfPinnedObject(), SecretKey.Length * 2);
gch.Free();
}
/// <summary>
/// สร้าง 64 bits Key สำหรับเข้ารหัสและถอดรหัส
/// </summary>
/// <param name="SecretKey">Key สำหรับเข้ารหัสและถอดรหัส</param>
/// <returns>64 bits Key สำหรับเข้ารหัสและถอดรหัส</returns>
private static string MD5(string SecretKey)
{
string strReturn = string.Empty;
byte[] ByteSourceText = ASCIIEncoding.ASCII.GetBytes(SecretKey);
MD5CryptoServiceProvider Md5Hash = new MD5CryptoServiceProvider();
byte[] ByteHash = Md5Hash.ComputeHash(ByteSourceText);
foreach (byte b in ByteHash)
strReturn = strReturn + b.ToString("x2");
return strReturn.Substring(0, 8);
}
// Call this function to remove the key from memory after use for security
[DllImport("KERNEL32.DLL", EntryPoint = "RtlZeroMemory")]
private static extern bool ZeroMemory(IntPtr Destination, int Length);
}
|
 |
 |
 |
 |
Date :
2010-06-03 08:23:17 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
5. คลิกขวาที่ชื่อโปรเจ็ค ใน solution explorer เลือก add new item สร้าง form ใหม่ชื่อ ViewForm
6. เปิดไฟล์ชื่อ ViewForm.Designer.cs แล้วเอาโค้ดด้านล่างไปแปะ
ViewForm.Designer.cs
namespace ImageGallery
{
partial class ViewForm
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.ViewPictureBox = new System.Windows.Forms.PictureBox();
((System.ComponentModel.ISupportInitialize)(this.ViewPictureBox)).BeginInit();
this.SuspendLayout();
//
// ViewPictureBox
//
this.ViewPictureBox.Location = new System.Drawing.Point(12, 12);
this.ViewPictureBox.Name = "ViewPictureBox";
this.ViewPictureBox.Size = new System.Drawing.Size(268, 242);
this.ViewPictureBox.TabIndex = 0;
this.ViewPictureBox.TabStop = false;
this.ViewPictureBox.Click += new System.EventHandler(this.ViewPictureBox_Click);
//
// ViewForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.Add(this.ViewPictureBox);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
this.MaximizeBox = false;
this.MinimizeBox = false;
this.Name = "ViewForm";
this.Text = "View Image";
this.Load += new System.EventHandler(this.ViewForm_Load);
((System.ComponentModel.ISupportInitialize)(this.ViewPictureBox)).EndInit();
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.PictureBox ViewPictureBox;
}
}
|
 |
 |
 |
 |
Date :
2010-06-03 08:25:11 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
7. เปิดไฟล์ชื่อ ViewForm.cs แล้วเอาโค้ดด้านล่างไปแปะ
ViewForm.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ImageGallery
{
public partial class ViewForm : Form
{
private Image theImage;
public ViewForm(Image MyImage)
{
InitializeComponent();
theImage = MyImage;
this.ClientSize = new System.Drawing.Size(theImage.Width + 24, theImage.Height + 24);
ViewPictureBox.Size = new System.Drawing.Size(theImage.Width, theImage.Height);
}
private void ViewForm_Load(object sender, EventArgs e)
{
ViewPictureBox.Image = null;
ViewPictureBox.SizeMode = PictureBoxSizeMode.CenterImage;
ViewPictureBox.Image = theImage;
}
private void ViewPictureBox_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
|
 |
 |
 |
 |
Date :
2010-06-03 08:26:24 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
8. ลองรันดู
ss อยู่ที่บ้าน กลับบ้านก่อนเดี๋ยวเอามาแปะให้ ตอนนี้ก็จินตนาการกันไปก่อนแล้วกัน
|
 |
 |
 |
 |
Date :
2010-06-03 08:28:36 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
===== browse เพื่อ add รูป =====
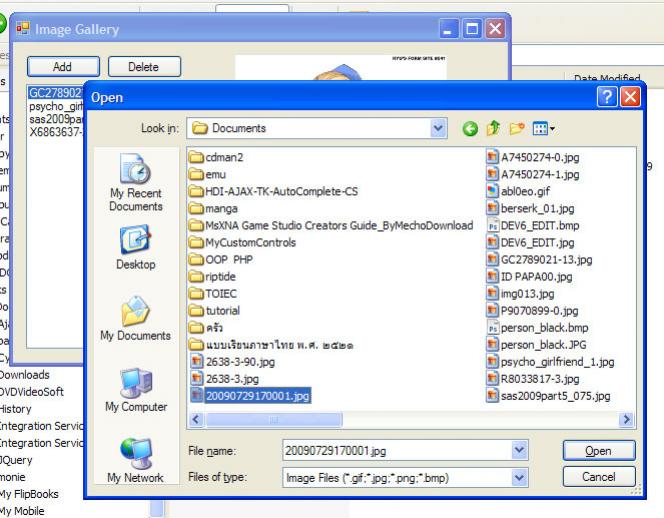
===== ไฟล์ที่ encrypt ใน folder =====
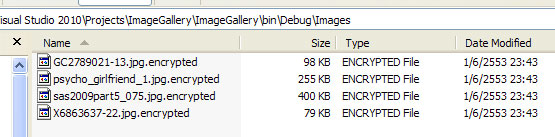
===== ตัวอย่างรูปใน thumbnail =====

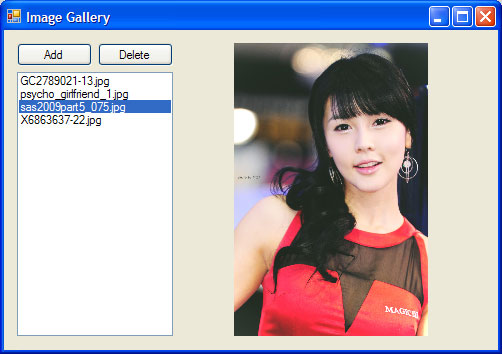
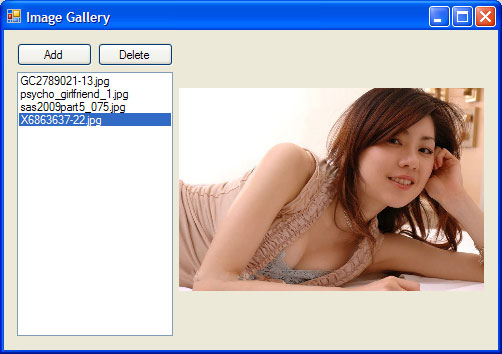
===== คลิกที่ thumbnail เพื่อขยายรูปเท่าขนาดจริง =====
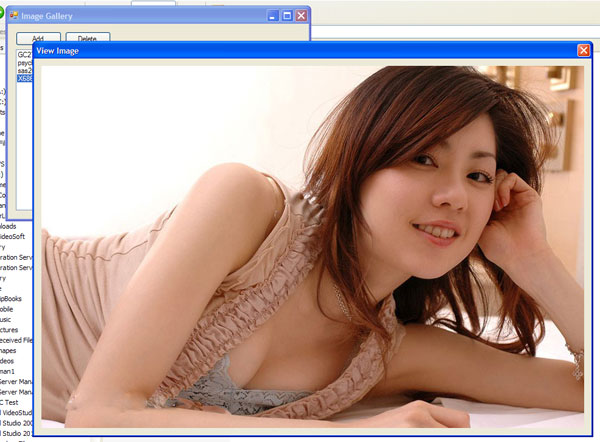

|
 |
 |
 |
 |
Date :
2010-06-19 17:58:58 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แจ๋มครับ 
|
 |
 |
 |
 |
Date :
2010-06-19 22:59:09 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พูดตามตรง ไม่ได้สนใจโน็ตบุคเลยอะ 
|
 |
 |
 |
 |
Date :
2010-06-20 07:57:46 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อ่ะเห็นด้วย ไม่ได้สนใจ notebook เลย แวมาดู เกี่ยวกับ picturebox เคลิ้ม ตรงนางแบบ notebook นี่ล่ะ อยู่หน้านี้นานเลยยย รู้สึกตัวอีกที
ลืมว่าเข้ามาทำอะไร
|
 |
 |
 |
 |
Date :
2010-08-13 14:14:06 |
By :
คนผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แล้วถ้าจะทำการติดตั้งแบบ install โปรแกรม ทั่วไปทำไงคะ แบบให้เปนรูปไอคอนที่กำหนดเลยอ่ะค่ะ บอกทีนะคะ ขอบคุนค่ะ ^^
|
 |
 |
 |
 |
Date :
2010-09-19 01:05:53 |
By :
poychan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เอารูปมาเป็นสือในการสอน น่าสนใจมากครับ 
|
 |
 |
 |
 |
Date :
2010-09-19 09:22:51 |
By :
nooknoname |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เยี่ยมมากเลยครับ ขอบคุณมานะคับ
|
 |
 |
 |
 |
Date :
2010-12-27 22:03:46 |
By :
นักศึกษา ปวช วิทยาลัยแห่งหนึ่ง |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
หารูปหมีควาย น่ารักๆไม่มีง่ะ ได้รูปนี้มา
|
 |
 |
 |
 |
Date :
2010-12-29 22:03:50 |
By :
kabarobace |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แล้วถ้าเราต้องการ add รูปทีเดียวทั้งโฟล์เดอร์ทำได้มั้ย
|
 |
 |
 |
 |
Date :
2012-01-20 21:41:29 |
By :
Khun |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้าต้องการ add ทั้ง folder จะต้องแก้ตรงไหนค่ะ
|
 |
 |
 |
 |
Date :
2012-02-13 22:12:20 |
By :
lala |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอบคุณมากคร้า กำลังหาโค้ดแบบนี้เลย
ได้ความรู้อีกเยอะเลยค่ะ
|
 |
 |
 |
 |
Date :
2013-01-28 16:41:58 |
By :
ใหม่ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พี่ค่ะ ถ้าต้องการทำปุ่มบันทึกรูปเพิ่ม
โดยให้เก้บpathรูปไปไว้ในโฟลเดออร์เรากำหนด
ต้องเขียนโค้ดประมาณไหนเหรอค่ะ
ขอบคุณค่ะ
|
 |
 |
 |
 |
Date :
2013-02-02 14:01:57 |
By :
chibi |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อยากได้ตัวอย่างแบบ ดึงข้อมูลรูปที่มีทั้งโฟลเดอร์มาแสดงครับ
โดย USER สามารถเลือกเพื่อรูปไปเพื่อบันทึกเป็นชื่อใหม่ โดยเลือกได้ครั้งละรูปหรือมากกว่า 1 รูปได้ (VB.NET)
รบกวนหน่อยนะครับ
|
 |
 |
 |
 |
Date :
2015-07-14 19:18:23 |
By :
golfgee12 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 00
|