 |
|
งานเข้าครับ Capture Screen ด้วย C# (.NET) ช่วยหน่อยครับ C# Capture Web Page , Capture Screen |
|
 |
|
|
 |
 |
|
เคยเขียนไว้ 
|
 |
 |
 |
 |
Date :
2010-08-03 11:22:26 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
วันนี้มีโอกาศได้เขียนครับ
อันนี้ Capture Windows ปัจจุบัน
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Imaging;
using System.IO;
namespace CCapture
{
class Program : Form
{
private static Bitmap bmpScreenshot;
private static Graphics gfxScreenshot;
static void Main(string[] args)
{
bmpScreenshot = new Bitmap(Screen.PrimaryScreen.Bounds.Width, Screen.PrimaryScreen.Bounds.Height, PixelFormat.Format32bppArgb);
gfxScreenshot = Graphics.FromImage(bmpScreenshot);
gfxScreenshot.CopyFromScreen(Screen.PrimaryScreen.Bounds.X, Screen.PrimaryScreen.Bounds.Y, 0, 0, Screen.PrimaryScreen.Bounds.Size, CopyPixelOperation.SourceCopy);
bmpScreenshot.Save("C:\\ThaiCreate\\Capture.jpg", ImageFormat.Jpeg);
gfxScreenshot.Dispose();
}
}
}
|
ประวัติการแก้ไข 2010-08-31 08:54:49
 |
 |
 |
 |
Date :
2010-08-30 16:08:36 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อันนี้ C# Capture Web Page ครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Imaging;
using System.IO;
namespace CCapture
{
class Program : Form
{
[STAThread]
static void Main(string[] args)
{
System.Threading.Thread t = new System.Threading.Thread(ThreadStart);
t.SetApartmentState(System.Threading.ApartmentState.STA);
t.Start();
}
private static void ThreadStart()
{
WebBrowser web = new WebBrowser();
web.ScrollBarsEnabled = false;
web.ScriptErrorsSuppressed = true;
web.Navigate("https://www.thaicreate.com");
while (web.ReadyState != System.Windows.Forms.WebBrowserReadyState.Complete)
System.Windows.Forms.Application.DoEvents();
System.Threading.Thread.Sleep(1500);
int width = web.Document.Body.ScrollRectangle.Width;
int height = web.Document.Body.ScrollRectangle.Height;
web.Width = width;
web.Height = height;
System.Drawing.Bitmap bmp = new System.Drawing.Bitmap(width, height);
web.DrawToBitmap(bmp, new System.Drawing.Rectangle(0, 0, width, height));
bmp.Save("C:\\tc\\thaicreate.jpg", ImageFormat.Jpeg);
bmp.Dispose();
}
}
}
|
ประวัติการแก้ไข 2010-08-31 08:49:55
 |
 |
 |
 |
Date :
2010-08-31 08:49:30 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
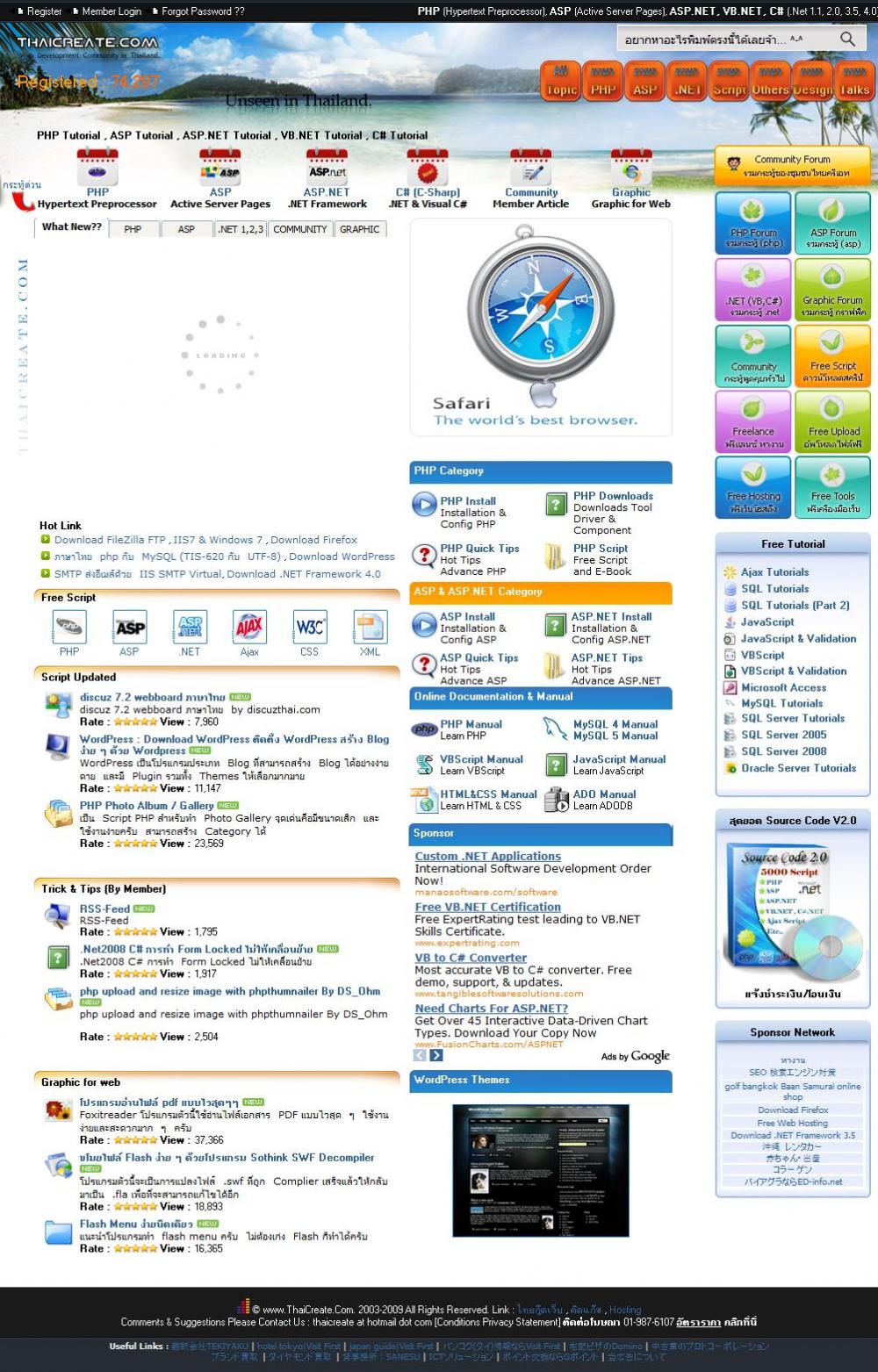
แจ๋มจริง 
|
 |
 |
 |
 |
Date :
2010-08-31 08:56:56 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อันนี้ VB.NET Capture Web page
Code (VB.NET)
Imports System
Imports System.Data
Imports System.Windows.Forms
Imports System.Drawing.Imaging
Imports System.IO
Imports System.Data.SqlServerCe
Module Module1
'By https://www.thaicreate.com (mr.win) '
<STAThread()> _
Sub Main(ByVal args As String())
Dim t As New System.Threading.Thread(AddressOf ThreadStart)
t.SetApartmentState(System.Threading.ApartmentState.STA)
t.Start()
End Sub
Private Sub ThreadStart()
'*** Capture Web Page ***//
Dim web As New WebBrowser()
web.ScrollBarsEnabled = False
web.ScriptErrorsSuppressed = True
Dim strURL As String = "https://www.thaicreate.com"
Dim fileName As String = DateTime.Now.ToString("ddMMyyyyHHmmss") & ".jpg"
web.Navigate(strURL)
While web.ReadyState <> System.Windows.Forms.WebBrowserReadyState.Complete
System.Windows.Forms.Application.DoEvents()
End While
System.Threading.Thread.Sleep(1500)
Dim width As Integer = web.Document.Body.ScrollRectangle.Width
Dim height As Integer = web.Document.Body.ScrollRectangle.Height
web.Width = width
web.Height = height
Dim bmp As New System.Drawing.Bitmap(width, height)
web.DrawToBitmap(bmp, New System.Drawing.Rectangle(0, 0, width, height))
bmp.Save(Directory.GetCurrentDirectory() & "\Img\" & fileName, ImageFormat.Jpeg)
bmp.Dispose()
End Sub
End Module
|
 |
 |
 |
 |
Date :
2010-09-02 22:37:54 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
|
 |
 |
 |
 |
Date :
2010-09-04 09:19:44 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เพิ่งมาเจอแต่อาจจะเป็นประโยชน์กับคนที่ต้องการ
ง่ายๆครับ sendkey printscreen จากนั้นให้นำไปเก็บไว้ใน picturebox และสั่งบันทึกได้เลย
|
 |
 |
 |
 |
Date :
2011-05-11 20:58:30 |
By :
คนหลงทาง |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลอง code c# ทั้งสองแบบแล้ว ครับ run ไม่ผ่านทั้งสองเลยคับ ==!
|
 |
 |
 |
 |
Date :
2011-09-06 23:16:20 |
By :
telesis |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อยากได้ C ธรรมดา
หรือ พ่วงกับ OpenCV ด้วยจะเป็นการดีมากๆเรยครับ
ขอขอบคุณมากครับ
|
 |
 |
 |
 |
Date :
2011-12-01 22:52:51 |
By :
ding_iumv |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Imaging;
using System.IO;
namespace CCapture
{
class Program : Form
{
private static Bitmap bmpScreenshot;
private static Graphics gfxScreenshot;
static void Main(string[] args)
{
bmpScreenshot = new Bitmap(Screen.PrimaryScreen.Bounds.Width, Screen.PrimaryScreen.Bounds.Height, PixelFormat.Format32bppArgb);
gfxScreenshot = Graphics.FromImage(bmpScreenshot);
gfxScreenshot.CopyFromScreen(Screen.PrimaryScreen.Bounds.X, Screen.PrimaryScreen.Bounds.Y, 0, 0, Screen.PrimaryScreen.Bounds.Size, CopyPixelOperation.SourceCopy);
bmpScreenshot.Save("C:\\ThaiCreate\\Capture.jpg", ImageFormat.Jpeg);
gfxScreenshot.Dispose();
}
}
}
ผมลองโค็ดนี้แล้วครับ แต่มัน eror ขอพี่ช่วย แสดงขั้นตอนิธีการทำหน่อยครับ ผมมือใหม่เพิ่งเริ่มเขียนครับ
|
 |
 |
 |
 |
Date :
2011-12-09 10:40:55 |
By :
พูลศักดิ์ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมก็รันได้ปกติครับ หรือไม่ลองไปตามลิ้งค์แล้ว Download ไฟล์ตามที่ผมแนะนำให้ครับ 
|
 |
 |
 |
 |
Date :
2011-12-09 17:03:06 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ได้เปลี่ยน path ตรงนี้รึเปล่าครับ "C:\\ThaiCreate\\Capture.jpg"
|
 |
 |
 |
 |
Date :
2011-12-12 18:09:46 |
By :
Kotakin |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ไม่ทราบว่าถ้าต้องการ ให้บันทึกรูปโดย ที่ก่อนทำการบันทึกรูปภาพ
เราต้องใช้ mouse ลากกรอบตามที่เราต้องการ crop รูปแล้วค่อยทำการเซฟเป็นรูป
ไม่ทราบว่าาต้องเพิ่มเติมยังไงบ้างครับ ขอบคุณครับ
|
 |
 |
 |
 |
Date :
2012-06-26 01:49:09 |
By :
Nat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมอยากได้ วิธีเอา source code print screen อ้ะครับนั่งหาวิธีมานานแล้วทำไม ไม่ได้ซักที ๕๕๕๕๕+
แล้วผมอยากทราบครับ ว่าผมจะเขียนโปรแกรมเป็น auto screen นี่ละครับจับภาพไปเรื่อยๆ สามารถตั้งค่าว่าจับทุกๆกี่วิ แยกเไฟล์ป็นแต่ละวัน
ผมควรใช้โปรแกรมอะไรเขียนดีครับ ^^
รบกวน แนะนำทีนะครับ
|
 |
 |
 |
 |
Date :
2015-08-27 14:32:21 |
By :
ปอนด์ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อยากถามว่าอยากได้แบบนี้แต่เป็นภาษาJAVAได้มั้ยครับ
|
 |
 |
 |
 |
Date :
2019-01-09 00:15:30 |
By :
มิ้ว |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|