 |
|
จะทำปุ่มแก้ไขในGridviewค่ะ โดยวันที่ผ่านมาแล้วจะไม่สามารถทำการแก้ไขได้ |
|
 |
|
|
 |
 |
|
ทำได้มากกว่า 1 วิธีค่ะ เอา code มาลงสิคะ จะดูว่าเพิ่มเติมอะไรได้บ้าง
|
 |
 |
 |
 |
Date :
2011-02-12 21:14:46 |
By :
สาวเอ๋อ (ก้อคนมานเอ๋อ) |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Data;
using System.Configuration;
namespace Dentist
{
public partial class CheckHistory : System.Web.UI.Page
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["Dentist_Pool"].ConnectionString);
SqlCommand com = new SqlCommand();
SqlDataAdapter da = null;
DataTable dt = null;
protected void Page_Load(object sender, EventArgs e)
{
con.Open();
String sqlSelect = "select Assign.AssignId, Assign.AssignDate, Clinic.Clinicname, Staff.Staffname, Listhealty.Listname, Assign.AssignTimestart, Assign.AssignTimefinish, Assign.Assigndetail, Listhealty.Listprice from Assign, Clinic, Staff, Listhealty " +
" where (Assign.ClinicId = Clinic.ClinicId AND Assign.StaffId = Staff.StaffId AND Assign.ListId = Listhealty.ListId) AND PatientId = '" + Session["sessionPatientId"] + "' ORDER BY AssignDate";
da = new SqlDataAdapter(sqlSelect, con);
con.Close();
BindData();
/////////////////////////////////////////////////////
con.Open();
//แสดงชื่อคลินิกให้เลือกใน dropdown
if (!IsPostBack)
{
ddlChkCli.SelectedValue = "";
String sqlSelect2 = "select ClinicName from Clinic";
com.CommandText = sqlSelect2;
com.Connection = con;
dt = new DataTable();
dt.Load(com.ExecuteReader(CommandBehavior.CloseConnection));
ddlChkCli.DataTextField = ("ClinicName");
ddlChkCli.DataValueField = ("ClinicName");
ddlChkCli.DataSource = dt;
ddlChkCli.DataBind();
}
con.Close();
//เอาชื่อที่เลือกจาก dropdown มาหา ClinicId
con.Open();
String sqlSelect3 = "select ClinicId from Clinic where ClinicName = '" + ddlChkCli.Text + "'";
com.CommandText = sqlSelect3;
com.Connection = con;
using (IDataReader reader = com.ExecuteReader())
{
while (reader.Read())
{
Session["sessionAssCli"] = reader.GetInt32(0);
break;
}
reader.Close();
con.Close();
}
// ส่วนของcalender
con.Open();
if (IsPostBack)
{
ChkDate.Style.Add("display", "none");
}
con.Close();
//เลือกid ของทันตแพทย์จากชื่อที่เลือก
con.Open();
String sqlSelect4 = "select StaffId from Staff where Staffname = '" + ddlChkStaff.Text + "'";
com.CommandText = sqlSelect4;
com.Connection = con;
using (IDataReader reader = com.ExecuteReader())
{
while (reader.Read())
{
Session["sessionAssSta"] = reader.GetInt32(0);
break;
}
reader.Close();
con.Close();
}
}
//เปลี่ยนรายละเอียดข้อมูลตามชื่อคลินิกที่เลือก
protected void ddlAssCli_SelectedIndexChanged(object sender, EventArgs e)
{
con.Open();
String sqlSelect6 = "select ListId, Listname, Listtime2, Listprice from Listhealty where ClinicId = '" + Session["sessionAssCli"] + "'";
da = new SqlDataAdapter(sqlSelect6, con);
con.Close();
dt = new DataTable();
da.Fill(dt);
grdChkList.DataSource = dt;
grdChkList.DataBind();
con.Open();
String sqlselect7 = "select Staffname from Staff where ClinicId = '" + Session["sessionAssCli"] + "'";
com.CommandText = sqlselect7;
com.Connection = con;
dt = new DataTable();
dt.Load(com.ExecuteReader(CommandBehavior.CloseConnection));
ddlChkStaff.DataTextField = ("Staffname");
ddlChkStaff.DataValueField = ("Staffname");
ddlChkStaff.DataSource = dt;
ddlChkStaff.DataBind();
con.Close();
}
void BindData()
{
dt = new DataTable();
da.Fill(dt);
grdChkHistory.DataSource = dt;
grdChkHistory.DataBind();
dt = null;
con.Close();
}
void Page_Unload()
{
con.Close();
con = null;
}
//Edit in Gridview
protected void modEditCommand(object sender, GridViewEditEventArgs e)
{
tblChk.Style.Remove("display");
con.Open();
String sqlSelect = "select Clinic.ClinicName, Listhealty.ListName, Staff.StaffName, Assign.AssignTimestart, Assign.AssignDate, Assign.Assigndetail from Assign, Clinic, Staff, Listhealty " +
" where (Assign.ClinicId = Clinic.ClinicId AND Assign.StaffId = Staff.StaffId AND Assign.ListId = Listhealty.ListId) AND AssignId = '" + grdChkHistory.DataKeys[e.NewEditIndex].Value + "'";
SqlCommand com = new SqlCommand(sqlSelect, con);
SqlDataReader dr;
dr = com.ExecuteReader();
dr.Read();
ddlChkCli.SelectedValue = dr["ClinicName"].ToString();
ddlChkStaff.SelectedValue = dr["StaffName"].ToString();
txtChkTime.Text = dr["AssignTimestart"].ToString();
txtChkDate.Text = dr["AssignDate"].ToString();
txtChkdetail.Text = dr["Assigndetail"].ToString();
}
//Delete in Gridview
protected void modDeleteCommand(object sender, GridViewDeleteEventArgs e)
{
con.Open();
String sqlDelete = "delete from Assign where AssignId = '" + grdChkHistory.DataKeys[e.RowIndex].Value + "'";
SqlCommand com = new SqlCommand(sqlDelete, con);
com.ExecuteNonQuery();
con.Close();
grdChkHistory.EditIndex = -1;
BindData();
}
//กดเพื่อแสดงปฏิทิน
protected void imbChkDate_Click(object sender, EventArgs e)
{
ChkDate.Style.Remove("display");
}
//เลือกวันที่จะนัด
protected void calChkDate_SelectionChanged(object sender, EventArgs e)
{
txtChkDate.Text = calChkDate.SelectedDate.ToString("d/MM/yyyy");
}
//ตงลงเมื่อแก้ไข
protected void btnChksubmit_Click(object sender, EventArgs e)
{
}
//ยกเลิกเมื่อแก้ไข
protected void btnChkcancel_Click(object sender, EventArgs e)
{
tblChk.Style.Add("display", "none");
}
}
}
ประมาณนี้ค่ะ รบกวนด้วยนะค่ะ
|
 |
 |
 |
 |
Date :
2011-02-12 21:23:10 |
By :
1234 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
protected void Page_Load(object sender, EventArgs e)
{
System.Text.StringBuilder cmdBuilder = new System.Text.StringBuilder();
cmdBuilder.AppendLine("SELECT Assign.AssignId, Assign.AssignDate, Clinic.Clinicname ");
cmdBuilder.AppendLine(" , Staff.Staffname, Listhealty.Listname, Assign.AssignTimestart ");
cmdBuilder.AppendLine(" , Assign.AssignTimefinish, Assign.Assigndetail, Listhealty.Listprice ");
// ADD NEW COLUMN FOR ALLOW EDIT THIS ROW
cmdBuilder.AppendLine(" , CASE ");
// จากโจทย์เฉพาะวันที่ก่อนปัจจุบัน 1 วัน และวันที่ยังไม่ถึง
// ถ้าเป็นวันก่อนหน้า 1 วันก่อนจะถึงวันปัจจุบันค่าที่ได้คือ -1
// ฉะนั้น ถ้ามากกว่า -1 จะถูก SET เป็น TRUE นั่นคือแก้ไขได้
cmdBuilder.AppendLine(" WHEN DATEADD(DAY ,Assign.AssignDate ,GETDATE()) >= -1 ");
cmdBuilder.AppendLine(" THEN TRUE ");
cmdBuilder.AppendLine(" ELSE FALSE ");
cmdBuilder.AppendLine(" END AS [EDITABLE] ");
// END ADD NEW COLUMN
cmdBuilder.AppendLine("FROM [Assign], [Clinic], [Staff], [Listhealty]");
cmdBuilder.AppendLine("WHERE (Assign.ClinicId = Clinic.ClinicId AND Assign.StaffId = Staff.StaffId ");
cmdBuilder.AppendLine(" AND Assign.ListId = Listhealty.ListId)");
cmdBuilder.AppendLine(" AND PatientId = '" + Session["sessionPatientId"].ToString() + "' ");
cmdBuilder.AppendLine("ORDER BY AssignDate ;");
string sqlSelect = cmdBuilder.ToString() ;
con.Open();
da = new SqlDataAdapter(sqlSelect, con);
con.Close();
BindData();
.......
......
ทีนี้เราจะได้ field ชื่อ editable ที่บอกว่า row นี้แก้ไขได้หรือไม่
การกันไม่ให้แก้ไขได้แบบง่ายคือ block ที่ ui หรือที่ gridview
จะได้ไม่เสียเวลาวิ่งกลับมา check ว่า แก้ไข row ได้หรือไม่ที่ server-side code
หมายถึงควรจะทำให้ ปุ่ม edit enabled/disabled ด้วยค่าของ field editable นี่เลย
ซึ่งปกติเราจะเอา ปุ่ม add / edit / delete ไว้ใน template column ของ gridview
ไหนเอา design mode ของคุณเฉพาะช่วง gridview มาดิคะ
|
ประวัติการแก้ไข 2011-02-12 23:39:49 2011-02-16 16:33:19 2011-02-16 16:37:58 2011-02-16 16:45:42
 |
 |
 |
 |
Date :
2011-02-12 23:37:15 |
By :
blurEyes |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เอารูปมาให้ดูค่ะ
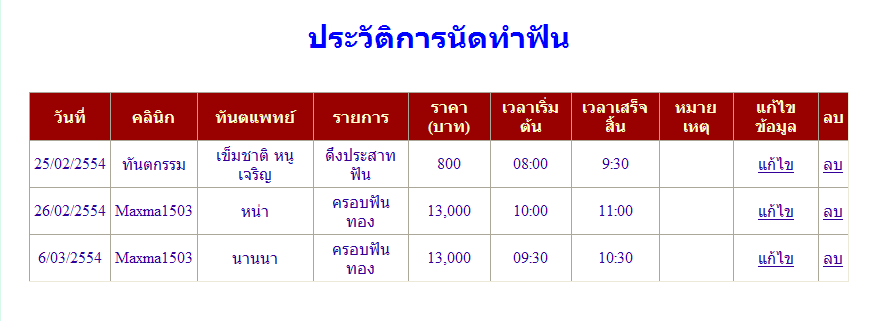
ประมาณนี้ค่ะ
รบกวนด้วยนะค่ะ ขอบคุณค่ะ
|
 |
 |
 |
 |
Date :
2011-02-13 12:51:11 |
By :
1234 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ที่อยากให้เอามาลงคือ code ของ design mode น่ะค่ะ จะได้ปรับแก้ได้ง่าย
ส่วนที่คุนเอามาคือ screen shot ตอนรันแล้ว มันติดวันหยุดและก้อพรีเส้นงานด้วยเลยทิ้งช่วงไปหน่อย
จะเอาตัวอย่างให้คุณดูละกันนะคะ ลองเอาไปปรับแก้ดู
จิงๆจะทำตัวอย่างทีเดียวหลายๆอยางไปเลยจะได้ไม่ต้องถามตอบกันบ่อยๆ
วิธีแก้ปัญหาของคุณคือค่อยๆ ทำให้ตัวปัญหาง่ายขึ้นทีละขั้น
ขั้นแรก ให้คุณสร้าง field ที่ใช้อ้างอิงว่า row นั้นแก้ไขได้หรือไม่
ขั้นสอง นำ field ค่าที่ได้จากข้างต้นมากำหนดให้ปุ่มแก้ไขนั้นมีสถานะ enabled/disabled
ดังนี้ค่ะ
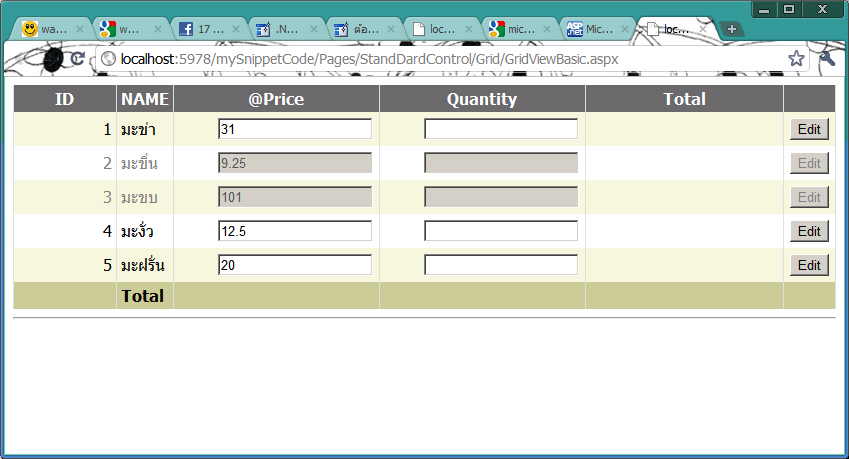
ในตัวอย่างนี่จะกำหนด datasource มาจาก object เอง เพื่อให้ง่ายต่อความเข้าใจ
และพยายามจะทำ auto calculate total price on client ไปด้วยเลย
คือป้อนจำนวนแล้วจะคำนวนราคารวมให้เลย แต่เดวจะยกไปอีกกระทูนึงแล้วกัน
ส่วนที่ comment ไปก้อช่างไว้ก่อนนะคะ
Code (ASP)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="GridViewBasic.aspx.cs" Inherits="Pages_StandDardControl_Grid_GridViewBasic" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
<script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.4.4.min.js" type="text/javascript"></script>
<style>
.DisbledLabel
{
color: Gray;
}
.EnabledLabel
{
color: Black;
}
</style>
</head>
<body>
<script language="javascript" type="text/javascript"></script>
<form id="form1" runat="server">
<div style="margin: auto auto;">
<asp:GridView ID="GridView1" runat="server" Width="100%" BackColor="White" BorderColor="#DEDFDE"
BorderWidth="1px" CellPadding="4" ForeColor="Black" GridLines="Vertical" AutoGenerateColumns="False"
BorderStyle="None" ShowFooter="true" ShowHeader="true">
<AlternatingRowStyle BackColor="White" />
<Columns>
<asp:TemplateField HeaderText="ID" ItemStyle-Width="100" ItemStyle-HorizontalAlign="Right">
<ItemTemplate>
<asp:Label ID="LabelID" runat="server" Text='<%# Eval("ID") %>' CssClass='<%# Convert.ToBoolean(Eval("Status"))?"EnabledLabel":"DisbledLabel" %>'></asp:Label>
</ItemTemplate>
<ItemStyle Width="100px"></ItemStyle>
</asp:TemplateField>
<asp:TemplateField HeaderText="NAME">
<ItemTemplate>
<asp:Label ID="LabelName" runat="server" Text='<%# Eval("Name") %>' CssClass='<%# Convert.ToBoolean(Eval("Status"))?"EnabledLabel":"DisbledLabel" %>'></asp:Label>
</ItemTemplate>
<FooterTemplate>
<b>Total</b>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="@Price" ItemStyle-Width="200" ItemStyle-HorizontalAlign="Right">
<ItemTemplate>
<asp:TextBox ID="TextBoxPricePerUnit" runat="server" Text='<%# Eval("PricePerUnit") %>'
Enabled='<%# Eval("Status") %>'></asp:TextBox>
</ItemTemplate>
<ItemStyle HorizontalAlign="Right" Width="200px"></ItemStyle>
</asp:TemplateField>
<asp:TemplateField HeaderText="Quantity" ItemStyle-Width="200" ItemStyle-HorizontalAlign="Right">
<ItemTemplate>
<asp:TextBox ID="TextBoxQuantity" runat="server" Enabled='<%# Eval("Status") %>'></asp:TextBox>
</ItemTemplate>
<ItemStyle HorizontalAlign="Right" Width="200px"></ItemStyle>
</asp:TemplateField>
<asp:TemplateField HeaderText="Total" ItemStyle-Width="200" ItemStyle-HorizontalAlign="Right">
<ItemTemplate>
<asp:Label ID="LabelTotalPrice" runat="server" Text=''></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Right" Width="200px"></ItemStyle>
</asp:TemplateField>
<asp:TemplateField>
<ItemTemplate>
<asp:Button ID="Button1" runat="server" Text="Edit" CommandName="Edit" CommandArgument='<%# Eval("ID") %>'
Enabled='<%# Eval("Status") %>' />
</ItemTemplate>
</asp:TemplateField>
</Columns>
<FooterStyle BackColor="#CCCC99" />
<HeaderStyle BackColor="#6B696B" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#F7F7DE" ForeColor="Black" HorizontalAlign="Right" />
<RowStyle BackColor="#F7F7DE" />
<SelectedRowStyle BackColor="#CE5D5A" ForeColor="White" Font-Bold="True" />
<SortedAscendingCellStyle BackColor="#FBFBF2" />
<SortedAscendingHeaderStyle BackColor="#848384" />
<SortedDescendingCellStyle BackColor="#EAEAD3" />
<SortedDescendingHeaderStyle BackColor="#575357" />
</asp:GridView>
<hr />
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Pages_StandDardControl_Grid_GridViewBasic : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
//InitClientScript();
if (!IsPostBack)
{
Session["PG_DATASOURCE"] = null;
GridView1.DataSource = InitDataSource();
GridView1.DataBind();
}
}
private List<SimpleItem> InitDataSource()
{
if (Session["PG_DATASOURCE"] == null)
{
List<SimpleItem> myList = new List<SimpleItem>();
int i = 01;
myList.Add(new SimpleItem(i++, "มะข่า", 31, true));
myList.Add(new SimpleItem(i++, "มะขิ่น", 9.25, false));
myList.Add(new SimpleItem(i++, "มะขบ", 101, false));
myList.Add(new SimpleItem(i++, "มะงั่ว", 12.50, true));
myList.Add(new SimpleItem(i++, "มะฝรั่น", 20, true));
Session["PG_DATASOURCE"] = myList;
}
return Session["PG_DATASOURCE"] as List<SimpleItem>;
}
protected void Page_Init(object sender, EventArgs e) // Init(object sender, EventArgs e)
{
//GridView1.RowDataBound += new GridViewRowEventHandler(this.OnRowDataBound);
}
//private void InitClientScript()
//{
// System.Text.StringBuilder scriptBuilder = new System.Text.StringBuilder();
// scriptBuilder.AppendLine();
// scriptBuilder.AppendLine(" function CalcRowTotal(varLabelTotalPrice ");
// scriptBuilder.AppendLine(" ,varTextBoxPricePerUnit ,varTextBoxQuantity)");
// scriptBuilder.AppendLine(" {");
// scriptBuilder.AppendLine(" alert('ok');");
// scriptBuilder.AppendLine(" var clLabelTotalPrice = document.getElementById(varLabelTotalPrice) ;");
// scriptBuilder.AppendLine(" if(clLabelTotalPrice==null) alert('nothing founded!');");
// scriptBuilder.AppendLine(" alert('01 | ' + clLabelTotalPrice.value );");
// scriptBuilder.AppendLine(" var clTextBoxPricePerUnit = document.getElementById(varTextBoxPricePerUnit) ;");
// scriptBuilder.AppendLine(" var clTextBoxQuantity = document.getElementById(varTextBoxQuantity) ;");
// scriptBuilder.AppendLine(" ");
// scriptBuilder.AppendLine(" alert('02');");
// //scriptBuilder.AppendLine(" var RowTotal = clTextBoxPricePerUnit.value * clTextBoxQuantity.value ;");
// scriptBuilder.AppendLine(" var RowTotal = 'test'");
// scriptBuilder.AppendLine(" alert('03 | ' + RowTotal );");
// scriptBuilder.AppendLine(" clLabelTotalPrice.value = RowTotal ;");
// scriptBuilder.AppendLine(" ");
// scriptBuilder.AppendLine(" }");
// scriptBuilder.AppendLine();
// System.Web.UI.HtmlControls.HtmlGenericControl ScriptParser
// = new System.Web.UI.HtmlControls.HtmlGenericControl("script");
// ScriptParser.Attributes.Add("type", "text/javascript");
// ScriptParser.Attributes.Add("language", "javascript");
// ScriptParser.InnerHtml = scriptBuilder.ToString();
// this.Form.Controls.Add(ScriptParser);
//}
//protected void OnRowDataBound(object sender, GridViewRowEventArgs e)
//{
// if (e.Row.RowType == DataControlRowType.DataRow)
// {
// TextBox txbPricePerUnit = (TextBox)e.Row.FindControl("TextBoxPricePerUnit");
// TextBox txbQuantity = (TextBox)e.Row.FindControl("TextBoxQuantity");
// Label lbTotalPrice = (Label)e.Row.FindControl("LabelTotalPrice");
// string scriptLn = string.Format("CalcRowTotal({0},{1},{2})"
// , lbTotalPrice.ClientID, txbPricePerUnit.ClientID, txbQuantity.ClientID);
// txbQuantity.Attributes.Add("onkeyup", scriptLn);
// txbPricePerUnit.Attributes.Add("onkeyup", scriptLn);
// txbQuantity.Attributes.Add("onfocusout", scriptLn);
// txbPricePerUnit.Attributes.Add("onfocusout", scriptLn);
// }
//}
//private double Convert2DoubleEx(string varStr)
//{
// double retValue = 0;
// double.TryParse(varStr, out retValue);
// return retValue;
//}
}
public class SimpleItem
{
public int ID { get; set; }
public string Name { get; set; }
public double PricePerUnit { get; set; }
public bool Status { get; set; }
public SimpleItem(int varID, string varName, double varPricePerUnit, bool varStatus)
{
this.ID = varID;
this.Name = varName;
this.PricePerUnit = varPricePerUnit;
this.Status = varStatus;
}
}
|
 |
 |
 |
 |
Date :
2011-02-15 16:14:28 |
By :
สาวเอ๋อ (ก้อคนมานเอ๋อ) |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอนแรกลองเขียนโค้ดตาม กระทู้ที่3ดูค่ะ เขียนไปแบบนี้
Code (C#)
con.Open();
String sqlSelect = "select Assign.AssignId, Assign.AssignDate, Clinic.Clinicname, Staff.Staffname, " +
"Listhealty.Listname, Assign.AssignTimestart, Assign.AssignTimefinish, Assign.Assigndetail, " +
"Listhealty.Listprice, Assign.Status CASE WHEN DATEADD(DAY ,Assign.AssignDate ,GETDATE()) >= -1 THEN TRUE ELSE FALSE END AS [EDITABLE] " +
"from Assign, Clinic, Staff, Listhealty " +
" where (Assign.ClinicId = Clinic.ClinicId AND Assign.StaffId = Staff.StaffId AND Assign.ListId = Listhealty.ListId) AND PatientId = '" + Session["sessionPatientId"] + "' ORDER BY AssignDate DESC ,AssignTimestart ASC";
da = new SqlDataAdapter(sqlSelect, con);
con.Close();
BindData();
พอรันแล้วมันแจ้งว่าแบบนี้ค่ะ
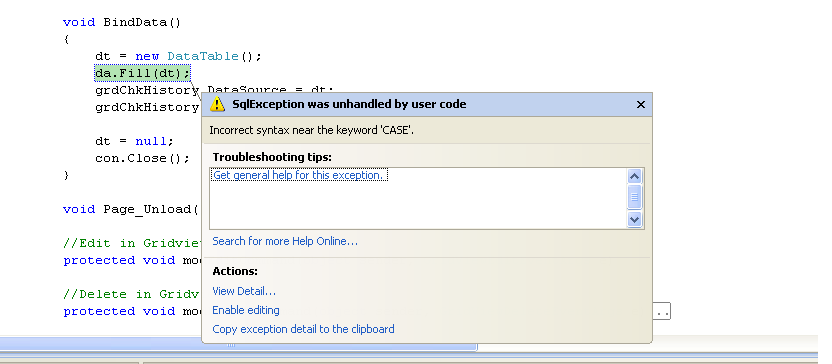
แต่วิธีข้างบนนี้ยังไม่ได้ลองทำดูค่ะ เพราะง่วนกับตารางนัดอยู่
ขอบคุณค่ะ
|
 |
 |
 |
 |
Date :
2011-02-16 16:23:52 |
By :
1234 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
System.Text.StringBuilder cmdBuilder = new System.Text.StringBuilder();
cmdBuilder.AppendLine("SELECT Assign.AssignId, Assign.AssignDate, Clinic.Clinicname ");
cmdBuilder.AppendLine(" , Staff.Staffname, Listhealty.Listname, Assign.AssignTimestart ");
cmdBuilder.AppendLine(" , Assign.AssignTimefinish, Assign.Assigndetail, Listhealty.Listprice ");
// ADD NEW COLUMN FOR ALLOW EDIT THIS ROW
cmdBuilder.AppendLine(" , CASE ");
// จากโจทย์เฉพาะวันที่ก่อนปัจจุบัน 1 วัน และวันที่ยังไม่ถึง
// ถ้าเป็นวันก่อนหน้า 1 วันก่อนจะถึงวันปัจจุบันค่าที่ได้คือ -1
// ฉะนั้น ถ้ามากกว่า -1 จะถูก SET เป็น TRUE นั่นคือแก้ไขได้
cmdBuilder.AppendLine(" WHEN DATEADD(DAY ,Assign.AssignDate ,GETDATE()) >= -1 ");
cmdBuilder.AppendLine(" THEN TRUE ");
cmdBuilder.AppendLine(" ELSE FALSE ");
cmdBuilder.AppendLine(" END AS [EDITABLE] ");
// END ADD NEW COLUMN
cmdBuilder.AppendLine("FROM [Assign], [Clinic], [Staff], [Listhealty]");
cmdBuilder.AppendLine("WHERE (Assign.ClinicId = Clinic.ClinicId AND Assign.StaffId = Staff.StaffId ");
cmdBuilder.AppendLine(" AND Assign.ListId = Listhealty.ListId)");
cmdBuilder.AppendLine(" AND PatientId = '" + Session["sessionPatientId"].ToString() + "' ");
cmdBuilder.AppendLine("ORDER BY AssignDate ;");
string sqlSelect = cmdBuilder.ToString() ;
con.Open();
da = new SqlDataAdapter(sqlSelect, con);
con.Close();
BindData();
แทน code ของคุนตั้งกะบรรทัดที่ 2 - 10 ด้วย code ข้างบนค่ะ
ตัว sql statement ของคุณเขียนผิดค่ะ
|
 |
 |
 |
 |
Date :
2011-02-16 16:48:23 |
By :
blurEyes |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|