 |
|
ขอความช่วยเหลือด่วยครับ debug แล้ว Error แบบนี้คืออะไรครับ |
|
 |
|
|
 |
 |
|
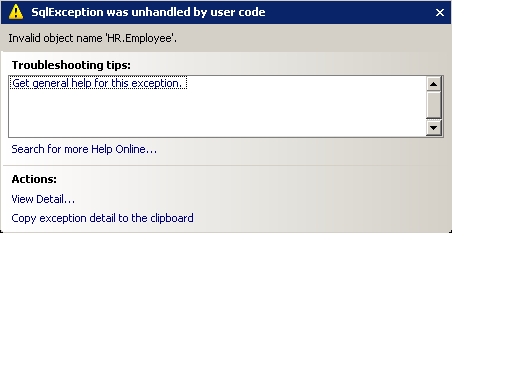
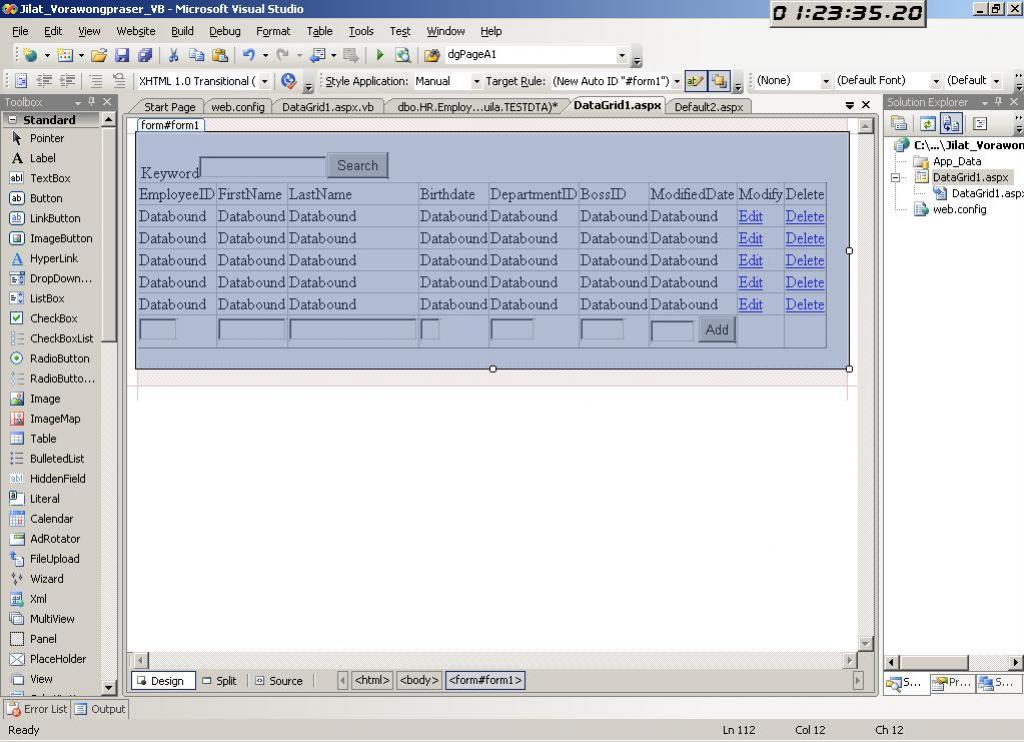
Code (ASP)
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="DataGrid1.aspx.vb" Inherits="DataGrid1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head id="Head1" runat="server">
<title>ThaiCreate.Com ASP.NET - DataGrid & SQL Server</title>
</head>
<body>
<form id="form1" runat="server">
<br />
<asp:Label ID="Label1" runat="server" Text="Keyword"></asp:Label>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
<asp:Button ID="Button1" runat="server" Text="Search" />
<asp:DataGrid id="myDataGrid" runat="server" AutoGenerateColumns="False"
ShowFooter="True"
DataKeyField="EmployeeID">
<Columns>
<asp:TemplateColumn HeaderText="EmployeeID">
<ItemTemplate>
<asp:Label id="lblEmployeeID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditEmployeeID" size="5" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddEmployeeID" size="5" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="FirstName">
<ItemTemplate>
<asp:Label id="lblFirstName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditFirstName" size="10" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddFirstName" size="10" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="LastName">
<ItemTemplate>
<asp:Label id="lblLastName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditLastName" size="20" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddLastName" size="20" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Birthdate">
<ItemTemplate>
<asp:Label id="lblBirthdate" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBirthdate" size="2" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBirthdate" size="2" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="DepartmentID">
<ItemTemplate>
<asp:Label id="lblDepartmentID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditDepartmentID" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddDepartmentID" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="BossID">
<ItemTemplate>
<asp:Label id="lblBossID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBossID" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBossID" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="ModifiedDate">
<ItemTemplate>
<asp:Label id="lblModifiedDate" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditModifiedDate" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddModifiedDate" size="6" runat="server"></asp:TextBox>
<asp:Button id="btnAdd" runat="server" Text="Add" CommandName="Add"></asp:Button>
</FooterTemplate>
</asp:TemplateColumn>
<asp:EditCommandColumn ButtonType="LinkButton" UpdateText="Update" CancelText="Cancel" EditText="Edit" HeaderText="Modify" ></asp:EditCommandColumn>
<asp:ButtonColumn Text="Delete" CommandName="Delete" HeaderText="Delete"></asp:ButtonColumn>
</Columns>
</asp:DataGrid>
<br />
</form>
</body>
</html>
Code (VB.NET)
Imports System.Data
Imports System.Data.SqlClient
Partial Class DataGrid1
Inherits System.Web.UI.Page
Dim objConn As SqlConnection
Dim objCmd As SqlCommand
Dim strSQL As String
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim strConnString As String
strConnString = "Server=AQUILA;UID=TESTUSR;PASSWORD=TEST9999;database=TESTDTA;Max Pool Size=400;Connect Timeout=600;"
objConn = New SqlConnection(strConnString)
objConn.Open()
If Not Page.IsPostBack() Then
BindData()
End If
End Sub
Protected Sub BindData()
strSQL = "SELECT * FROM HR.Employee"
Dim dtReader As SqlDataReader
objCmd = New SqlCommand(strSQL, objConn)
dtReader = objCmd.ExecuteReader()
'*** BindData to DataGrid ***'
myDataGrid.DataSource = dtReader
myDataGrid.DataBind()
dtReader.Close()
dtReader = Nothing
End Sub
Protected Sub Page_Unload(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Unload
objConn.Close()
objConn = Nothing
End Sub
Protected Sub myDataGrid_CancelCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.CancelCommand
myDataGrid.EditItemIndex = -1
myDataGrid.ShowFooter = True
BindData()
End Sub
Protected Sub myDataGrid_DeleteCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.DeleteCommand
strSQL = "DELETE FROM HR.Employee WHERE EmployeeID = '" & myDataGrid.DataKeys.Item(e.Item.ItemIndex) & "'"
objCmd = New SqlCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myDataGrid.EditItemIndex = -1
BindData()
End Sub
Protected Sub myDataGrid_EditCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.EditCommand
myDataGrid.EditItemIndex = e.Item.ItemIndex
myDataGrid.ShowFooter = False
BindData()
End Sub
Protected Sub myDataGrid_ItemCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.ItemCommand
If e.CommandName = "Add" Then
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Item.FindControl("txtEmployeeID"), TextBox)
'*** Name ***'
Dim txtName As TextBox = CType(e.Item.FindControl("txtFirstName"), TextBox)
'*** Email ***'
Dim txtEmail As TextBox = CType(e.Item.FindControl("txtLastName"), TextBox)
'*** CountryCode ***'
Dim txtCountryCode As TextBox = CType(e.Item.FindControl("txtBirthdate"), TextBox)
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Item.FindControl("txtADepartmentID"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
' Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
Dim txtAddModifiedDate As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
' Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
strSQL = "INSERT INTO HR.Employee (EmployeeID,FirstName,txtLastName,CountryCode,Budget,Used) " & _
" VALUES ('" & txtCustomerID.Text & "','" & txtName.Text & "','" & txtEmail.Text & "' " & _
" ,'" & txtCountryCode.Text & "','" & txtBudget.Text & "','" & txtUsed.Text & "') "
objCmd = New SqlCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
BindData()
End If
End Sub
Protected Sub myDataGrid_UpdateCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.UpdateCommand
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Item.FindControl("txtEditCustomerID"), TextBox)
'*** Email ***'
Dim txtName As TextBox = CType(e.Item.FindControl("txtEditName"), TextBox)
'*** Name ***'
Dim txtEmail As TextBox = CType(e.Item.FindControl("txtEditEmail"), TextBox)
'*** CountryCode ***'
Dim txtCountryCode As TextBox = CType(e.Item.FindControl("txtEditCountryCode"), TextBox)
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Item.FindControl("txtEditBudget"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Item.FindControl("txtEditUsed"), TextBox)
strSQL = "UPDATE HR.Employee SET CustomerID = '" & txtCustomerID.Text & "' " & _
" ,Name = '" & txtName.Text & "' " & _
" ,Email = '" & txtEmail.Text & "' " & _
" ,CountryCode = '" & txtCountryCode.Text & "' " & _
" ,Budget = '" & txtBudget.Text & "' " & _
" ,Used = '" & txtUsed.Text & "' " & _
" WHERE CustomerID = '" & myDataGrid.DataKeys.Item(e.Item.ItemIndex) & "'"
objCmd = New SqlCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myDataGrid.EditItemIndex = -1
myDataGrid.ShowFooter = True
BindData()
End Sub
Protected Sub Button1_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles Button1.Click
strSQL = "SELECT * FROM HR.Employee WHERE (Name like '%" & Me.TextBox1.Text & "%') "
objCmd = New SqlCommand(strSQL, objConn)
'Dim dtReader As SqlDataReader
Dim dtAdapter As SqlDataAdapter
Dim ds As New DataSet
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(ds)
myDataGrid.DataSource = ds
myDataGrid.DataBind()
End Sub
Protected Sub myDataGrid_SelectedIndexChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles myDataGrid.SelectedIndexChanged
End Sub
End Class
Tag : ASP.NET, VB.NET
|
|
 |
 |
 |
 |
Date :
2011-09-06 15:05:03 |
By :
joeycole |
View :
1254 |
Reply :
4 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แก้ไขภาพแรกครับ
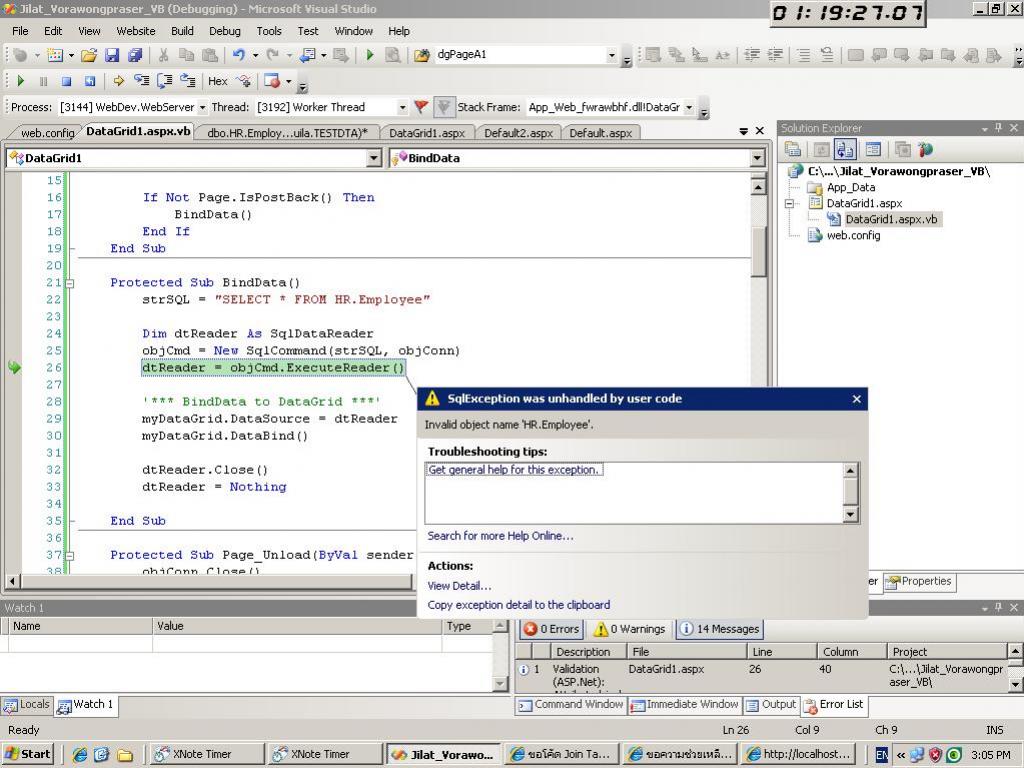
|
 |
 |
 |
 |
Date :
2011-09-06 15:07:17 |
By :
joeycole |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (VB.NET)
Imports System.Data
Imports System.Data.SqlClient
Partial Class DataGrid1
Inherits System.Web.UI.Page
Dim objConn As SqlConnection
Dim objCmd As SqlCommand
Dim strSQL As String
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim strConnString As String
strConnString = "Server=AQUILA;UID=TESTUSR;PASSWORD=TEST9999;database=TESTDTA;Max Pool Size=400;Connect Timeout=600;"
objConn = New SqlConnection(strConnString)
objConn.Open()
If Not Page.IsPostBack() Then
BindData()
DropDownListDataTable()
End If
End Sub
Sub DropDownListDataTable()
Dim objConn As SqlConnection
Dim dtAdapter As SqlDataAdapter
Dim dt As New DataTable
Dim strConnString As String
strConnString = "Server=AQUILA;UID=TESTUSR;PASSWORD=TEST9999;database=TESTDTA;Max Pool Size=400;Connect Timeout=600;"
objConn = New SqlConnection(strConnString)
objConn.Open()
Dim strSQL As String
strSQL = "SELECT * FROM [HR.Department]"
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
'*** DropDownlist ***'
With Me.myDDL1
.DataSource = dt
.DataTextField = "Name"
.DataValueField = "Name"
.DataBind()
End With
'*** Default Value ***'
' myDDL1.SelectedIndex = myDDL1.Items.IndexOf(myDDL1.Items.FindByValue("TH"))
End Sub
Protected Sub BindData()
strSQL = "SELECT * FROM [HR.Employee]"
Dim dtReader As SqlDataReader
objCmd = New SqlCommand(strSQL, objConn)
dtReader = objCmd.ExecuteReader()
'Dim dtAdapter As SqlDataAdapter
'Dim ds As New DataSet
'dtAdapter = New SqlDataAdapter(strSQL, objConn)
'dtAdapter.Fill(ds)
'myDataGrid.DataSource = ds
'myDataGrid.DataBind()
'*** BindData to DataGrid ***'
myDataGrid.DataSource = dtReader
myDataGrid.DataBind()
dtReader.Close()
dtReader = Nothing
End Sub
Protected Sub Page_Unload(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Unload
objConn.Close()
objConn = Nothing
End Sub
Protected Sub myDataGrid_CancelCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.CancelCommand
myDataGrid.EditItemIndex = -1
myDataGrid.ShowFooter = True
BindData()
End Sub
Protected Sub myDataGrid_DeleteCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.DeleteCommand
strSQL = "DELETE FROM [HR.Employee] WHERE EmployeeID = '" & myDataGrid.DataKeys.Item(e.Item.ItemIndex) & "'"
objCmd = New SqlCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myDataGrid.EditItemIndex = -1
BindData()
End Sub
Protected Sub myDataGrid_EditCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.EditCommand
myDataGrid.EditItemIndex = e.Item.ItemIndex
myDataGrid.ShowFooter = False
BindData()
End Sub
Protected Sub myDataGrid_ItemCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.ItemCommand
If e.CommandName = "Add" Then
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Item.FindControl("txtEmployeeID"), TextBox)
'*** Name ***'
Dim txtName As TextBox = CType(e.Item.FindControl("txtFirstName"), TextBox)
'*** Email ***'
Dim txtEmail As TextBox = CType(e.Item.FindControl("txtLastName"), TextBox)
'*** CountryCode ***'
Dim txtCountryCode As TextBox = CType(e.Item.FindControl("txtBirthdate"), TextBox)
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Item.FindControl("txtADepartmentID"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
' Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
Dim txtAddModifiedDate As TextBox = CType(e.Item.FindControl("txtModifiedDate"), TextBox)
' Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
strSQL = "INSERT INTO [HR.Employee] (EmployeeID,FirstName,LastName,Birthdate,DepartmentID,BossID,ModifiedDate) " & _
" VALUES ('" & txtCustomerID.Text & "','" & txtName.Text & "','" & txtEmail.Text & "' ,'" & txtCountryCode.Text & "','" & txtBudget.Text & "','" & txtUsed.Text & "','" & txtAddModifiedDate.Text & "')"
objCmd = New SqlCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
BindData()
End If
End Sub
Protected Sub myDataGrid_UpdateCommand(ByVal source As Object, ByVal e As DataGridCommandEventArgs) Handles myDataGrid.UpdateCommand
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Item.FindControl("txtEmployeeID"), TextBox)
'*** Name ***'
Dim txtName As TextBox = CType(e.Item.FindControl("txtFirstName"), TextBox)
'*** Email ***'
Dim txtEmail As TextBox = CType(e.Item.FindControl("txtLastName"), TextBox)
'*** CountryCode ***'
Dim txtCountryCode As TextBox = CType(e.Item.FindControl("txtBirthdate"), TextBox)
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Item.FindControl("txtADepartmentID"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
' Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
Dim txtAddModifiedDate As TextBox = CType(e.Item.FindControl("txtModifiedDate"), TextBox)
' Dim txtUsed As TextBox = CType(e.Item.FindControl("txtBossID"), TextBox)
strSQL = "UPDATE [HR.Employee] SET EmployeeID = '" & txtCustomerID.Text & "' " & _
" ,FirstName = '" & txtName.Text & "' " & _
" ,LastName = '" & txtEmail.Text & "' " & _
" ,Birthdate = '" & txtCountryCode.Text & "' " & _
" ,DepartmentID = '" & txtBudget.Text & "' " & _
" ,BossID = '" & txtUsed.Text & "' " & _
" ,ModifiedDate = '" & txtAddModifiedDate.Text & "' " & _
" WHERE CustomerID = '" & myDataGrid.DataKeys.Item(e.Item.ItemIndex) & "'"
objCmd = New SqlCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myDataGrid.EditItemIndex = -1
myDataGrid.ShowFooter = True
BindData()
End Sub
Protected Sub Button1_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles Button1.Click
strSQL = "SELECT * FROM [HR.Employee] WHERE (FirstName like '%" & Me.TextBox1.Text & "%')or(LastName like '%" & Me.TextBox1.Text & "%')or(EmployeeID = '%" & Me.TextBox2.Text & "%')"
' or(Employee like '%" & Me.TextBox1.Text & "%') "
objCmd = New SqlCommand(strSQL, objConn)
'Dim dtReader As SqlDataReader
Dim dtAdapter As SqlDataAdapter
Dim ds As New DataSet
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(ds)
myDataGrid.DataSource = ds
myDataGrid.DataBind()
End Sub
Protected Sub myDataGrid_SelectedIndexChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles myDataGrid.SelectedIndexChanged
End Sub
Protected Sub DropDownList1_SelectedIndexChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles myDDL1.SelectedIndexChanged
End Sub
End Class
|
 |
 |
 |
 |
Date :
2011-09-06 16:25:15 |
By :
joeycole |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ทำไมไม่รู้จัก หรือว่าไม่มีสิทธิ์ใน Table ครับ 
|
 |
 |
 |
 |
Date :
2011-09-06 16:32:18 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|