 |
|
มัน error แบบนี้อะครับ ต้องแก้ code ตรงไหนหรอครับ
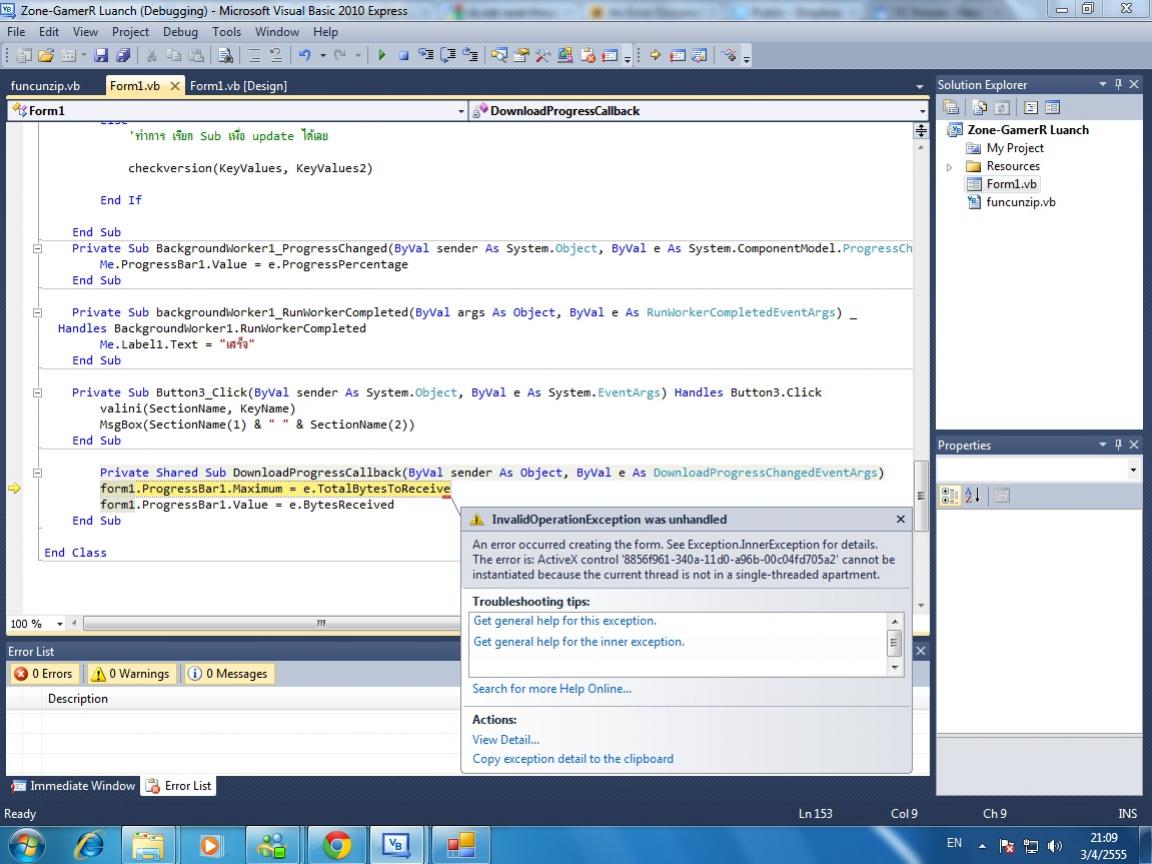
นี้ code ครับ
Code (VB.NET)
Imports System.IO
Imports System.Net
Imports System.ComponentModel
Public Class Form1
Dim SectionName(2) As String
Dim KeyName(5) As String
Dim KeyValues(5) As String
Dim KeyValues2(5) As String
Dim client As WebClient = New WebClient
Dim directory As String = Application.StartupPath & "/"
Public Shared WithEvents httpclient As WebClient
Public Sub New()
InitializeComponent()
BackgroundWorker1.WorkerReportsProgress = True
BackgroundWorker1.WorkerSupportsCancellation = True
End Sub
Private Declare Unicode Function GetPrivateProfileString Lib "kernel32" _
Alias "GetPrivateProfileStringW" (ByVal lpApplicationName As String, _
ByVal lpKeyName As String, ByVal lpDefault As String, _
ByVal lpReturnedString As String, ByVal nSize As Int32, _
ByVal lpFileName As String) As Int32
'Delagate เพื่อใช้ในการชี้ตำแหน่งของ Function หรือ Sub
Private Delegate Sub UpdateTextBox(ByVal data As String(), ByVal data2 As String())
Public Overloads Sub ReadINIFile(ByVal INIPath As String, _
ByVal SectionName As String, ByVal KeyName As String(), _
ByRef KeyValue As String())
Dim Length As Integer
Dim strData As String
strData = Space$(1024)
For i As Integer = 1 To KeyName.Length - 1
If KeyName(i) <> "" Then
'This will read the ini file using Section Name and Key
Length = GetPrivateProfileString(SectionName, KeyName(i), KeyValue(i), _
strData, strData.Length, LTrim(RTrim((INIPath))))
If Length > 0 Then
KeyValue(i) = strData.Substring(0, Length)
Else
KeyValue(i) = "error"
End If
End If
Next
End Sub
Private Sub valini(ByRef SectionName As String(), ByRef KeyName As String())
SectionName(1) = "client"
SectionName(2) = "server"
KeyName(1) = "serverurl"
KeyName(2) = "launchversion"
KeyName(3) = "mcversion"
KeyName(4) = "urlnewclient"
KeyName(5) = "urlpatch"
'KeyName(6) = ""
End Sub
Private Sub startgame(ByVal keyvalue As String(), ByVal keyvalue2 As String())
Dim url As String = keyvalue(1)
Dim launchversion As String = keyvalue(2)
Dim mcversion As String = keyvalue(3)
End Sub
Private Sub checkversion(ByVal keyvalue As String(), ByVal keyvalue2 As String())
'keyvalue = client : keyvalue2 = server
Dim mcversion As Double = keyvalue(3)
If keyvalue(2) = keyvalue2(2) Then
If keyvalue(3) < keyvalue2(3) Then
download(keyvalue2(5) & "/patch" & mcversion + "1" & ".zip")
End If
Else
'download(keyvalue2(5) + "zgmlaunch.zip")
End If
End Sub
Private Sub download(ByVal url As String)
Me.Label1.Text = "กำลังดาวโหลดไฟล์"
client.DownloadFileAsync(New Uri(url), directory + "zgmpatch.zip")
Try
AddHandler client.DownloadProgressChanged, AddressOf DownloadProgressCallback
Do While client.IsBusy
Application.DoEvents()
Loop
'install()
Catch ex As Exception
Label1.Text = "การDownloadผิดพลาด อาจไม่ได้เชื่อมต่ออินเตอร์เน็ตหรือไม่สามารถเข้าถึงเว็บเซิฟเวอร์"
End Try
End Sub
Private Sub install()
Dim args(2) As Object
args(0) = Application.StartupPath + "zgmpatch.zip"
args(1) = directory
funcunzip.UnzipFile(args)
End Sub
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
Me.Close()
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Me.Label1.Text = "กำลังเช็คเวอร์ชั่น"
valini(SectionName, KeyName)
ReadINIFile(Application.StartupPath & ("/config.ini"), SectionName(1), KeyName, KeyValues)
'MessageBox.Show(KeyValues(1) & " " & KeyValues(2))
'Run Work
If BackgroundWorker1.IsBusy <> True Then
BackgroundWorker1.RunWorkerAsync(KeyValues)
End If
End Sub
Private Sub bw_Dowork(ByVal sender As System.Object, ByVal e As DoWorkEventArgs) Handles BackgroundWorker1.DoWork
Dim KeyValues As Object() = DirectCast(e.Argument, Object())
valini(SectionName, KeyName)
'MsgBox(KeyValues(1))
AddHandler client.DownloadProgressChanged, AddressOf DownloadProgressCallback
client.DownloadFileAsync(New Uri(KeyValues(1) + "server.ini"), directory + "/server.ini")
Do While client.IsBusy
Application.DoEvents()
Loop
ReadINIFile(Application.StartupPath & ("/server.ini"), "server", KeyName, KeyValues2)
'MsgBox(KeyValues2(2))
If Label1.InvokeRequired Then
'สร้าง Delagate และส่งชื่อ Sub ที่ต้องการจะชี้ให้ไปทำงาน
Dim check As New UpdateTextBox(AddressOf checkversion)
'ทำการเรียก Sub และส่ง string value
Me.Invoke(check, New Object() {KeyValues, KeyValues2})
'ถ้าอยู่ใน MainThread
Else
'ทำการ เรียก Sub เพื่อ update ได้เลย
checkversion(KeyValues, KeyValues2)
End If
End Sub
Private Sub BackgroundWorker1_ProgressChanged(ByVal sender As System.Object, ByVal e As System.ComponentModel.ProgressChangedEventArgs) Handles BackgroundWorker1.ProgressChanged
Me.ProgressBar1.Value = e.ProgressPercentage
End Sub
Private Sub backgroundWorker1_RunWorkerCompleted(ByVal args As Object, ByVal e As RunWorkerCompletedEventArgs) _
Handles BackgroundWorker1.RunWorkerCompleted
Me.Label1.Text = "เสร็จ"
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
valini(SectionName, KeyName)
MsgBox(SectionName(1) & " " & SectionName(2))
End Sub
Private Shared Sub DownloadProgressCallback(ByVal sender As Object, ByVal e As DownloadProgressChangedEventArgs)
form1.ProgressBar1.Maximum = e.TotalBytesToReceive
form1.ProgressBar1.Value = e.BytesReceived
End Sub
End Class
Tag : .NET, VB.NET, VS 2010 (.NET 4.x)
|
|
 |
 |
 |
 |
Date :
2012-04-03 21:09:10 |
By :
theballkyo |
View :
1418 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |