 |
|
สอบถามปัญหาคำสั่งเรื่อง VB ช่วยหน่อยครับ ต้องเอาไปสอบวันพรุ่งนี้ รายละเอียดอยู่ด่านในครับ VB.net |
|
 |
|
|
 |
 |
|
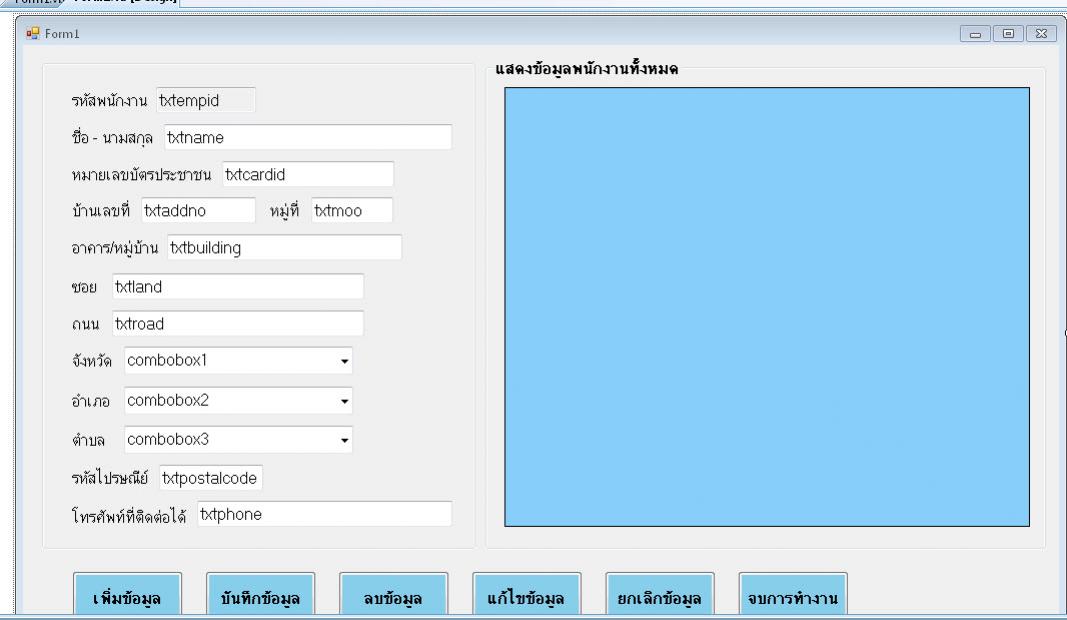
Code (VB.NET)
Imports System.Data.OleDb
Public Class Form1
Dim Conn As New OleDbConnection
Dim dc As New OleDbCommand
Dim dr As OleDbDataReader
Dim da As OleDbDataAdapter
Dim ds As New DataSet
Dim dt As DataTable
Dim currentrecord As Integer = 0
Dim recordcount As Integer = 0
Dim actionflag As String = ""
Dim IsFind As Boolean = False
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
With Conn
If .State = ConnectionState.Open Then .Close()
.ConnectionString = ConDB
.Open()
End With
formatdatagrid()
clearall()
enableall()
showdata()
End Sub
Sub showdata() 'การแสดงข้อมูล
Dim sqlshow As String = ""
sqlshow = "SELECT Employee.EmpId, Employee.EmpName, Employee.CardId, "
sqlshow &= " Employee.Addno, Employee.Building, Employee.Moo, "
sqlshow &= " Employee.Land, Employee.Road, Locality.LName, "
sqlshow &= " Amphur.AName, Province.ProName, Amphur.Code, Employee.Phone"
sqlshow &= " FROM ((Amphur INNER JOIN Employee ON Amphur.AID = Employee.AID)"
sqlshow &= " INNER JOIN Province ON Amphur.ProID = Province.ProID) INNER JOIN "
sqlshow &= " Locality ON (Amphur.AID = Locality.AID) AND "
sqlshow &= " (Employee.LID = Locality.LID);"
With dc
.CommandType = CommandType.Text
.CommandText = sqlshow
.Connection = Conn
dr = .ExecuteReader
If dr.HasRows Then
dt = New DataTable
dt.Load(dr)
DataGridView1.DataSource = dt
formatdatagrid()
Else
DataGridView1.DataSource = Nothing
formatdatagrid()
End If
End With
dr.Close()
End Sub
Sub formatdatagrid()
With DataGridView1
.ReadOnly = True
If .RowCount > 0 Then
.Font = New Font("Ms Sans Serif", 10.0)
.Columns(0).HeaderText = "รหัสพนักงาน"
.Columns(1).HeaderText = "ชื่อ-นามสกุลพนักงาน"
.Columns(2).HeaderText = "หมายเลขบัตรประชาชน"
.Columns(3).HeaderText = "บ้านเลขที่"
.Columns(4).HeaderText = "หมู่ที่"
.Columns(5).HeaderText = "อาคาร/หมู่บ้าน"
.Columns(6).HeaderText = "ซอย"
.Columns(7).HeaderText = "ถนน"
.Columns(8).HeaderText = "ตำบล"
.Columns(9).HeaderText = "อำเภอ"
.Columns(10).HeaderText = "จังหวัด"
.Columns(11).HeaderText = "รหัสไปรษณีย์"
.Columns(12).HeaderText = "โทรศัพท์ที่ติดต่อได้"
.Columns(0).Width = 150
.Columns(1).Width = 250
.Columns(2).Width = 200
.Columns(3).Width = 100
.Columns(4).Width = 100
.Columns(5).Width = 150
.Columns(6).Width = 200
.Columns(7).Width = 200
.Columns(8).Width = 200
.Columns(9).Width = 200
.Columns(10).Width = 200
.Columns(11).Width = 100
.Columns(12).Width = 150
End If
End With
End Sub
Sub clearall()
txtempid.Clear()
txtname.Clear()
txtcardid.Clear()
txtaddno.Clear()
txtmoo.Clear()
txtbuilding.Clear()
txtland.Clear()
txtroad.Clear()
combobox1.Text = "กรุณาเลือก"
combobox2.Text = "กรุณาเลือก"
combobox3.Text = "กรุณาเลือก"
txtpostalcode.Text = ""
txtphone.Text = ""
End Sub
Sub enableall()
txtname.Enabled = False
txtcardid.Enabled = False
txtaddno.Enabled = False
txtmoo.Enabled = False
txtbuilding.Enabled = False
txtland.Enabled = False
txtroad.Enabled = False
combobox1.Enabled = False
combobox2.Enabled = False
combobox3.Enabled = False
txtpostalcode.Enabled = False
txtphone.Enabled = False
End Sub
Sub autoid()
Dim sqlauto As String = ""
Dim nid As String
Dim tauauto As Integer = 0
sqlauto = "SELECT Top 1 EmpId FROM Employee ORDER BY EmpId DESC;"
Try
With dc
.CommandType = CommandType.Text
.CommandText = sqlauto
.Connection = conn
dr = .ExecuteReader
dr.Read()
nid = dr.Item("EmpId")
tauauto = CInt(nid) + 1
txtempid.Text = Format(tauauto.ToString("000000"))
End With
Catch ex As Exception
txtempid.Text = "000001"
End Try
dr.Close()
End Sub
Sub showcombo()
Dim sqlPro As String = "SELECT ProId,ProName FROM Province WHERE status='0' Order By ProId"
da = New OleDbDataAdapter(sqlPro, Conn)
da.Fill(ds, "Province")
If ds.Tables("Province").Rows.Count <> 0 Then
With combobox1
.DataSource = ds.Tables("Province")
.DisplayMember = "ProName"
.ValueMember = "ProId"
End With
End If
End Sub
Private Sub combobox2_SelectionChangeCommitted(ByVal sender As Object, ByVal e As System.EventArgs) Handles combobox2.SelectionChangeCommitted
Dim da3 As OleDbDataAdapter
Dim ds3 As New DataSet
ds3.Tables.Clear()
Dim sql1 As String = "SELECT Locality.LID, Locality.LName FROM Locality INNER JOIN Amphur ON Locality.AID = Amphur.AID WHERE Amphur.status = '" & 0 & "' and Locality.AID = TextBox1.Text;"
da3 = New OleDbDataAdapter(sql1, Conn)
da3.Fill(ds3, "Locality")
If ds3.Tables("Locality").Rows.Count <> 0 Then
With combobox3
.DataSource = ds3.Tables("Locality")
.DisplayMember = "LName"
.ValueMember = "LID"
End With
End If
End Sub
Private Sub btnadd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnadd.Click
autoid() : unenableall()
actionflag = "ADD"
txtname.Focus()
showcombo()
End Sub
Private Sub btnsave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnsave.Click
If Not IsNumeric(txtcardid.Text) Then
MessageBox.Show("ต้องกรอกข้อมูลเป็นตัวเลขเท่านั้น", "ตรวจสอบข้อมูล", MessageBoxButtons.OK, MessageBoxIcon.Warning)
txtcardid.Clear() : txtcardid.Focus() : Exit Sub
ElseIf txtcardid.TextLength < 13 Then
MessageBox.Show("กรุณากรอกข้อมูล หมายเลขบัตรประชาชนบัตรประชาชน เป็นตัวเลข 13 หลัก", "ตรวจสอบข้อมูล", MessageBoxButtons.OK, MessageBoxIcon.Warning)
txtcardid.Clear() : txtcardid.Focus() : Exit Sub
End If
Dim save As String = ""
Dim msgb As String = ""
Select Case actionflag
Case "ADD"
save = "Insert Into Employee (EmpId,EmpName,CardId,Addno,Building,Moo,Land,Road,LID,AID,ProID,Phone) "
save &= " values ('" & txtempid.Text & "','" & txtname.Text & "','" & txtcardid.Text & "','" & txtaddno.Text & "','" & txtbuilding.Text & "',"
save &= "'" & txtmoo.Text & "','" & txtland.Text & "','" & txtroad.Text & "',"
save &= "'" & combobox3.SelectedValue & "','" & combobox2.SelectedValue & "','" & combobox1.SelectedValue & "','" & txtphone.Text & "')"
End Select
With Conn
If .State = ConnectionState.Open Then .Close()
.ConnectionString = ConDB
.Open()
End With
If actionflag = "add." Then
msgb = "คุณต้องการเพิ่มข้อมูลใช่หรือไม่"
End If
If MessageBox.Show(msgb & "บันทึกข้อมูล", "คำยืนยัน", MessageBoxButtons.YesNo, MessageBoxIcon.Question, MessageBoxDefaultButton.Button1) = Windows.Forms.DialogResult.Yes Then
With dc
.CommandType = CommandType.Text
.CommandText = save
.Connection = Conn
.ExecuteNonQuery()
End With
End If
clearall()
showdata()
dr.Close()
End Sub
Sub unenableall()
txtname.Enabled = True : txtcardid.Enabled = True
txtaddno.Enabled = True
txtmoo.Enabled = True
txtbuilding.Enabled = True
txtland.Enabled = True
txtroad.Enabled = True
combobox1.Enabled = True
combobox2.Enabled = True
combobox3.Enabled = True
txtphone.Enabled = True
End Sub
Private Sub btncancel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btncancel.Click
clearall()
enableall()
End Sub
Private Sub btnexit_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnexit.Click
End
End Sub
Private Sub GroupBox1_Enter(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles GroupBox1.Enter
End Sub
End Class
โดยรายละเอียดมีดังนี้ครับ(ตรงที่ผมติดปัญหานะครับ)
- combobox1 จะแสดงข้อมูลจังหวัด โดยเชื่อมต่อกับตาราง Province เมื่อผู้ใช่เลือกจังหวัดแล้วโปรแกรมจะแสดงข้อมูลอำเภอของจังหวัดที่เลือกใน combobox2 โดยเชื่อมต่อกับตาราง Amphur
- เมื่อผู้ใช่เลือกอำเภอแล้ว โปรแกรมจะแสดงข้อมูลตำบลของอำเภอที่เลือกใน combobox3 โดยเชื่อมต่อกับตาราง Locality และจะแสดงข้อมูลรัหัสไปษณีย์ของแต่ละอำเภออัตโนมัติ
โหลดฐานข้อมูลและตัวอย่างโปรแกรมครับ
- ฐานข้อมูล
http://upload.one2car.com/download.aspx?pku=177B6FA04AH7KUDX53483[GVXN6VBD
- ตัวอย่างโปรแกรม
http://upload.one2car.com/download.aspx?pku=177B6FA04AE9VWO6VBAVYHZO67M8N4
Tag : .NET, VBScript, VB.NET
|
|
 |
 |
 |
 |
Date :
2012-08-10 16:19:31 |
By :
Wuttipong |
View :
1773 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
หน้าตาโปรแกรมครับ
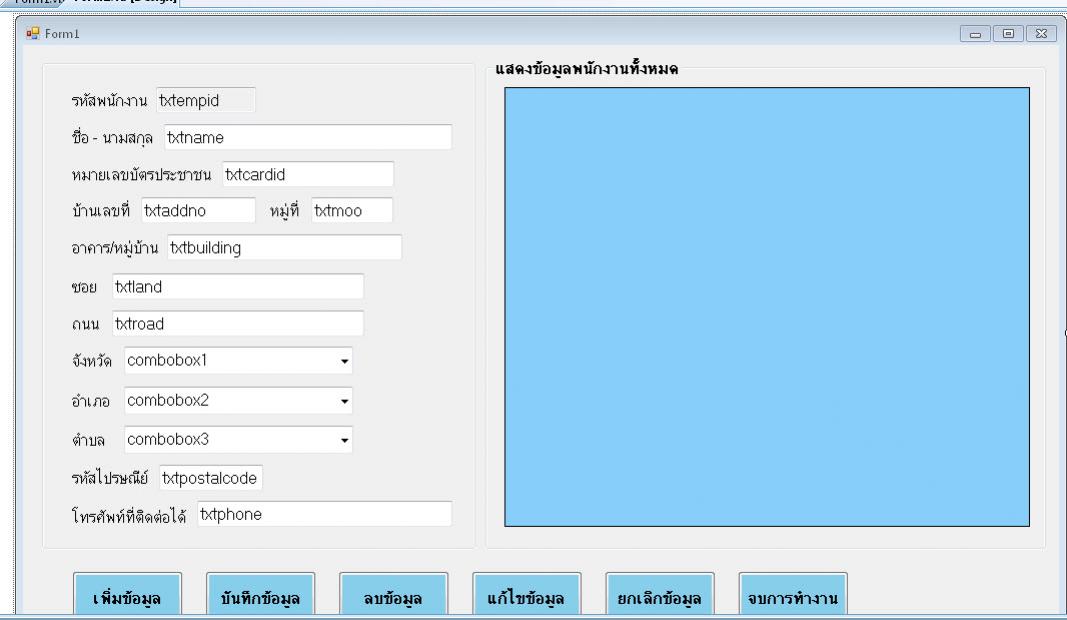
Code (VB.NET)
Imports System.Data.OleDb
Public Class Form1
Dim Conn As New OleDbConnection
Dim dc As New OleDbCommand
Dim dr As OleDbDataReader
Dim da As OleDbDataAdapter
Dim ds As New DataSet
Dim dt As DataTable
Dim currentrecord As Integer = 0
Dim recordcount As Integer = 0
Dim actionflag As String = ""
Dim IsFind As Boolean = False
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
With Conn
If .State = ConnectionState.Open Then .Close()
.ConnectionString = ConDB
.Open()
End With
formatdatagrid()
clearall()
enableall()
showdata()
End Sub
Sub showdata() 'การแสดงข้อมูล
Dim sqlshow As String = ""
sqlshow = "SELECT Employee.EmpId, Employee.EmpName, Employee.CardId, "
sqlshow &= " Employee.Addno, Employee.Building, Employee.Moo, "
sqlshow &= " Employee.Land, Employee.Road, Locality.LName, "
sqlshow &= " Amphur.AName, Province.ProName, Amphur.Code, Employee.Phone"
sqlshow &= " FROM ((Amphur INNER JOIN Employee ON Amphur.AID = Employee.AID)"
sqlshow &= " INNER JOIN Province ON Amphur.ProID = Province.ProID) INNER JOIN "
sqlshow &= " Locality ON (Amphur.AID = Locality.AID) AND "
sqlshow &= " (Employee.LID = Locality.LID);"
With dc
.CommandType = CommandType.Text
.CommandText = sqlshow
.Connection = Conn
dr = .ExecuteReader
If dr.HasRows Then
dt = New DataTable
dt.Load(dr)
DataGridView1.DataSource = dt
formatdatagrid()
Else
DataGridView1.DataSource = Nothing
formatdatagrid()
End If
End With
dr.Close()
End Sub
Sub formatdatagrid()
With DataGridView1
.ReadOnly = True
If .RowCount > 0 Then
.Font = New Font("Ms Sans Serif", 10.0)
.Columns(0).HeaderText = "รหัสพนักงาน"
.Columns(1).HeaderText = "ชื่อ-นามสกุลพนักงาน"
.Columns(2).HeaderText = "หมายเลขบัตรประชาชน"
.Columns(3).HeaderText = "บ้านเลขที่"
.Columns(4).HeaderText = "หมู่ที่"
.Columns(5).HeaderText = "อาคาร/หมู่บ้าน"
.Columns(6).HeaderText = "ซอย"
.Columns(7).HeaderText = "ถนน"
.Columns(8).HeaderText = "ตำบล"
.Columns(9).HeaderText = "อำเภอ"
.Columns(10).HeaderText = "จังหวัด"
.Columns(11).HeaderText = "รหัสไปรษณีย์"
.Columns(12).HeaderText = "โทรศัพท์ที่ติดต่อได้"
.Columns(0).Width = 150
.Columns(1).Width = 250
.Columns(2).Width = 200
.Columns(3).Width = 100
.Columns(4).Width = 100
.Columns(5).Width = 150
.Columns(6).Width = 200
.Columns(7).Width = 200
.Columns(8).Width = 200
.Columns(9).Width = 200
.Columns(10).Width = 200
.Columns(11).Width = 100
.Columns(12).Width = 150
End If
End With
End Sub
Sub clearall()
txtempid.Clear()
txtname.Clear()
txtcardid.Clear()
txtaddno.Clear()
txtmoo.Clear()
txtbuilding.Clear()
txtland.Clear()
txtroad.Clear()
combobox1.Text = "กรุณาเลือก"
combobox2.Text = "กรุณาเลือก"
combobox3.Text = "กรุณาเลือก"
txtpostalcode.Text = ""
txtphone.Text = ""
End Sub
Sub enableall()
txtname.Enabled = False
txtcardid.Enabled = False
txtaddno.Enabled = False
txtmoo.Enabled = False
txtbuilding.Enabled = False
txtland.Enabled = False
txtroad.Enabled = False
combobox1.Enabled = False
combobox2.Enabled = False
combobox3.Enabled = False
txtpostalcode.Enabled = False
txtphone.Enabled = False
End Sub
Sub autoid()
Dim sqlauto As String = ""
Dim nid As String
Dim tauauto As Integer = 0
sqlauto = "SELECT Top 1 EmpId FROM Employee ORDER BY EmpId DESC;"
Try
With dc
.CommandType = CommandType.Text
.CommandText = sqlauto
.Connection = conn
dr = .ExecuteReader
dr.Read()
nid = dr.Item("EmpId")
tauauto = CInt(nid) + 1
txtempid.Text = Format(tauauto.ToString("000000"))
End With
Catch ex As Exception
txtempid.Text = "000001"
End Try
dr.Close()
End Sub
Sub showcombo()
Dim sqlPro As String = "SELECT ProId,ProName FROM Province WHERE status='0' Order By ProId"
da = New OleDbDataAdapter(sqlPro, Conn)
da.Fill(ds, "Province")
If ds.Tables("Province").Rows.Count <> 0 Then
With combobox1
.DataSource = ds.Tables("Province")
.DisplayMember = "ProName"
.ValueMember = "ProId"
End With
End If
End Sub
Private Sub combobox2_SelectionChangeCommitted(ByVal sender As Object, ByVal e As System.EventArgs) Handles combobox2.SelectionChangeCommitted
Dim da3 As OleDbDataAdapter
Dim ds3 As New DataSet
ds3.Tables.Clear()
Dim sql1 As String = "SELECT Locality.LID, Locality.LName FROM Locality INNER JOIN Amphur ON Locality.AID = Amphur.AID WHERE Amphur.status = '" & 0 & "' and Locality.AID = TextBox1.Text;"
da3 = New OleDbDataAdapter(sql1, Conn)
da3.Fill(ds3, "Locality")
If ds3.Tables("Locality").Rows.Count <> 0 Then
With combobox3
.DataSource = ds3.Tables("Locality")
.DisplayMember = "LName"
.ValueMember = "LID"
End With
End If
End Sub
Private Sub btnadd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnadd.Click
autoid() : unenableall()
actionflag = "ADD"
txtname.Focus()
showcombo()
End Sub
Private Sub btnsave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnsave.Click
If Not IsNumeric(txtcardid.Text) Then
MessageBox.Show("ต้องกรอกข้อมูลเป็นตัวเลขเท่านั้น", "ตรวจสอบข้อมูล", MessageBoxButtons.OK, MessageBoxIcon.Warning)
txtcardid.Clear() : txtcardid.Focus() : Exit Sub
ElseIf txtcardid.TextLength < 13 Then
MessageBox.Show("กรุณากรอกข้อมูล หมายเลขบัตรประชาชนบัตรประชาชน เป็นตัวเลข 13 หลัก", "ตรวจสอบข้อมูล", MessageBoxButtons.OK, MessageBoxIcon.Warning)
txtcardid.Clear() : txtcardid.Focus() : Exit Sub
End If
Dim save As String = ""
Dim msgb As String = ""
Select Case actionflag
Case "ADD"
save = "Insert Into Employee (EmpId,EmpName,CardId,Addno,Building,Moo,Land,Road,LID,AID,ProID,Phone) "
save &= " values ('" & txtempid.Text & "','" & txtname.Text & "','" & txtcardid.Text & "','" & txtaddno.Text & "','" & txtbuilding.Text & "',"
save &= "'" & txtmoo.Text & "','" & txtland.Text & "','" & txtroad.Text & "',"
save &= "'" & combobox3.SelectedValue & "','" & combobox2.SelectedValue & "','" & combobox1.SelectedValue & "','" & txtphone.Text & "')"
End Select
With Conn
If .State = ConnectionState.Open Then .Close()
.ConnectionString = ConDB
.Open()
End With
If actionflag = "add." Then
msgb = "คุณต้องการเพิ่มข้อมูลใช่หรือไม่"
End If
If MessageBox.Show(msgb & "บันทึกข้อมูล", "คำยืนยัน", MessageBoxButtons.YesNo, MessageBoxIcon.Question, MessageBoxDefaultButton.Button1) = Windows.Forms.DialogResult.Yes Then
With dc
.CommandType = CommandType.Text
.CommandText = save
.Connection = Conn
.ExecuteNonQuery()
End With
End If
clearall()
showdata()
dr.Close()
End Sub
Sub unenableall()
txtname.Enabled = True : txtcardid.Enabled = True
txtaddno.Enabled = True
txtmoo.Enabled = True
txtbuilding.Enabled = True
txtland.Enabled = True
txtroad.Enabled = True
combobox1.Enabled = True
combobox2.Enabled = True
combobox3.Enabled = True
txtphone.Enabled = True
End Sub
Private Sub btncancel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btncancel.Click
clearall()
enableall()
End Sub
Private Sub btnexit_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnexit.Click
End
End Sub
Private Sub GroupBox1_Enter(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles GroupBox1.Enter
End Sub
End Class
โดยรายละเอียดมีดังนี้ครับ(ตรงที่ผมติดปัญหานะครับ)
- combobox1 จะแสดงข้อมูลจังหวัด โดยเชื่อมต่อกับตาราง Province เมื่อผู้ใช่เลือกจังหวัดแล้วโปรแกรมจะแสดงข้อมูลอำเภอของจังหวัดที่เลือกใน combobox2 โดยเชื่อมต่อกับตาราง Amphur
- เมื่อผู้ใช่เลือกอำเภอแล้ว โปรแกรมจะแสดงข้อมูลตำบลของอำเภอที่เลือกใน combobox3 โดยเชื่อมต่อกับตาราง Locality และจะแสดงข้อมูลรัหัสไปษณีย์ของแต่ละอำเภออัตโนมัติ
โหลดฐานข้อมูลและตัวอย่างโปรแกรมครับ
- ฐานข้อมูล
http://upload.one2car.com/download.aspx?pku=177B6FA04AH7KUDX53483[GVXN6VBD
- ตัวอย่างโปรแกรม
http://upload.one2car.com/download.aspx?pku=177B6FA04AE9VWO6VBAVYHZO67M8N4
|
 |
 |
 |
 |
Date :
2012-08-10 16:23:00 |
By :
Wuttipong |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|