 |
|
[WebApp] VB.Net จะสร้างฟอร์มค้นหาโดยมีเงือนไขจาก Radiobutton ค่ะ |
|
 |
|
|
 |
 |
|
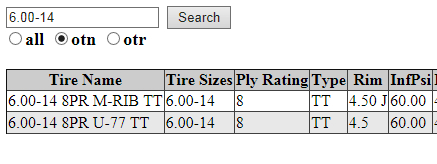
ต้องการสร้างฟอร์มค้นหาสินค้าจาก 2 โรงงานตามนี้อ่ะค่ะ
All : หาได้ทั้ง 2 โรงงาน
Database ของทั้ง 2 โรงงานแยกกันตามนี้
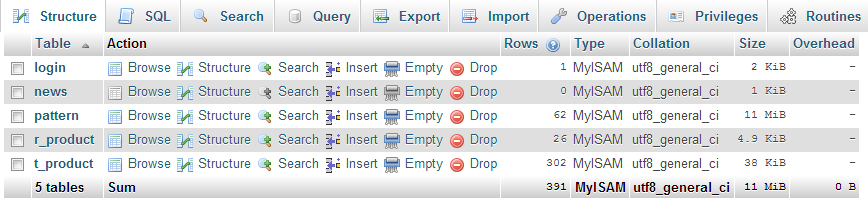
โครงสร้างของ t_product
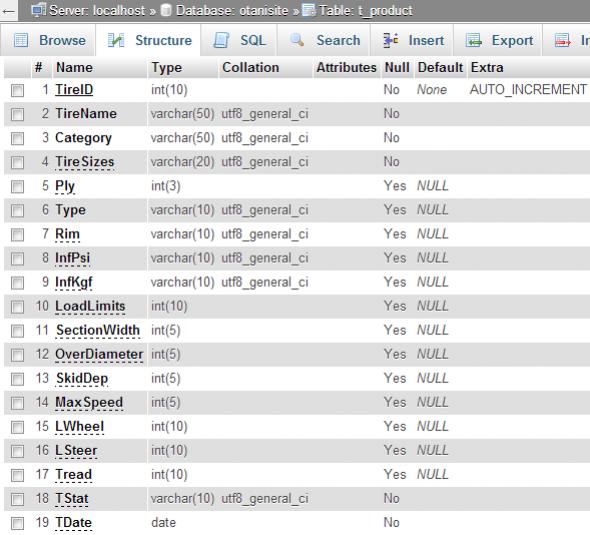
โครงสร้างของ r_product
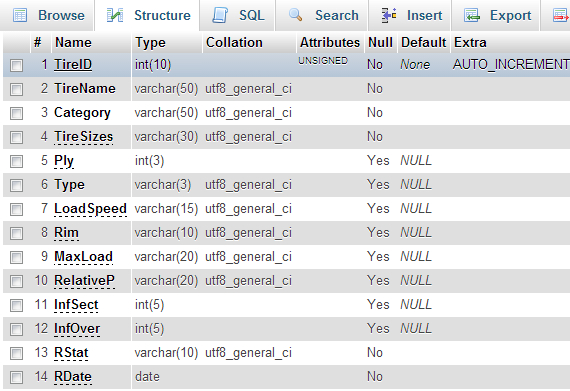
ปัญหาตอนนี้คือ มันสามารถค้นหาจาก t_product ได้เงื่อนไขเดียว รบกวนผู้รู้ดูโค้ดให้หน่อยนะค่ะ ขอบคุณค่ะ
Code (VB.NET)
<%@ Page Language="VB" %>
<%@ import Namespace="System.Data" %>
<%@ import Namespace="MySql.Data.MySqlClient" %>
<script runat="server">
Dim strKeyWord As String
Sub Page_Load(sender As Object, e As EventArgs)
strKeyWord = Me.txtKeyWord.Text
End Sub
Sub BindData()
Dim Conn As New MySqlConnection
Dim Cmd As New MySqlCommand
Dim da As New MySqlDataAdapter
Dim ds As New DataSet
Dim strConnString, strSQL As String
da = New MySqlDataAdapter("SELECT * FROM dbo.otanisite", Conn)
strConnString = "Server=localhost;User Id=root; Password=root; Database=otanisite; Pooling=false"
If Me.RadioButton1.Checked = True Then
strSQL = "SELECT TireName AS F1 FROM t_product WHERE TireName = '%" & strKeyWord & "%' UNION ALL SELECT TireName AS F1 FROM r_product WHERE TireName = '%" & strKeyWord & "%'"
End If
If Me.RadioButton2.Checked = True Then
strSQL = "SELECT * FROM t_product WHERE (TireName like '%" & strKeyWord & "%')"
End If
If Me.RadioButton3.Checked = True Then
strSQL = "SELECT * FROM r_product WHERE (TireName like '%" & strKeyWord & "%')"
End If
Conn.ConnectionString = strConnString
With Cmd
.Connection = Conn
.CommandText = strSQL
.CommandType = CommandType.Text
End With
da.SelectCommand = Cmd
da.Fill(ds)
'*** BindData to GridView ***'
myGridView.DataSource = ds
myGridView.DataBind()
da = Nothing
Conn.Close()
Conn = Nothing
End Sub
Sub myGridView_RowDataBound(sender As Object, e As GridViewRowEventArgs)
'*** Tire Name ***'
Dim lblTireName As Label = CType(e.Row.FindControl("lblTireName"), Label)
If Not IsNothing(lblTireName) Then
lblTireName.Text = e.Row.DataItem("TireName")
End If
'*** Tire Sizes ***'
Dim lblTireSizes As Label = CType(e.Row.FindControl("lblTireSizes"), Label)
If Not IsNothing(lblTireSizes) Then
lblTireSizes.Text = e.Row.DataItem("TireSizes")
End If
'*** Ply Rating ***'
Dim lblPly As Label = CType(e.Row.FindControl("lblPly"), Label)
If Not IsNothing(lblPly) Then
lblPly.Text = e.Row.DataItem("Ply")
End If
'*** Type ***'
Dim lblType As Label = CType(e.Row.FindControl("lblType"), Label)
If Not IsNothing(lblType) Then
lblType.Text = e.Row.DataItem("Type")
End If
'*** Rim ***'
Dim lblRim As Label = CType(e.Row.FindControl("lblRim"), Label)
If Not IsNothing(lblRim) Then
lblRim.Text = e.Row.DataItem("Rim")
End If
'*** InfPsi ***'
Dim lblInfPsi As Label = CType(e.Row.FindControl("lblInfPsi"), Label)
If Not IsNothing(lblInfPsi) Then
lblInfPsi.Text = FormatNumber(e.Row.DataItem("InfPsi"), 2)
End If
'*** InfKgf ***'
Dim lblInfKgf As Label = CType(e.Row.FindControl("lblInfKgf"), Label)
If Not IsNothing(lblInfKgf) Then
lblInfKgf.Text = FormatNumber(e.Row.DataItem("InfKgf"), 2)
End If
'*** Load Limits ***'
Dim lblLoadLimits As Label = CType(e.Row.FindControl("lblLoadLimits"), Label)
If Not IsNothing(lblLoadLimits) Then
lblLoadLimits.Text = e.Row.DataItem("LoadLimits")
End If
'*** Section Width ***'
Dim lblSectionWidth As Label = CType(e.Row.FindControl("lblSectionWidth"), Label)
If Not IsNothing(lblSectionWidth) Then
lblSectionWidth.Text = e.Row.DataItem("SectionWidth")
End If
'*** Overall Diameter ***'
Dim lblOverDiameter As Label = CType(e.Row.FindControl("lblOverDiameter"), Label)
If Not IsNothing(lblOverDiameter) Then
lblOverDiameter.Text = e.Row.DataItem("OverDiameter")
End If
'*** Skid Depth ***'
Dim lblSkidDep As Label = CType(e.Row.FindControl("lblSkidDep"), Label)
If Not IsNothing(lblSkidDep) Then
lblSkidDep.Text = e.Row.DataItem("SkidDep")
End If
End Sub
Sub btnSearch_Click(sender As Object, e As EventArgs)
BindData()
End Sub
</script>
<html>
<head>
<title>Test - Search From DB</title>
</head>
<form id="form1" runat="server">
<asp:TextBox id="txtKeyWord" runat="server"></asp:TextBox>
<asp:Button id="btnSearch" onclick="btnSearch_Click" runat="server" Text="Search"></asp:Button>
<br />
<asp:RadioButton ID="RadioButton1" GroupName="Searchby" Value="all" runat="server"
Text="all" Font-Bold="True" Font-Size="Large" />
<asp:RadioButton ID="RadioButton2" GroupName="Searchby" Value="otn" runat="server"
Text="otn" Font-Bold="True" Font-Size="Large" Font-Underline="False" />
<asp:RadioButton ID="RadioButton3" GroupName="Searchby" Value="otr" runat="server"
Text="otr" Font-Bold="True" Font-Size="Large" />
<br />
<br />
<asp:GridView id="myGridView" runat="server"
AutoGenerateColumns="False" onRowDataBound="myGridView_RowDataBound">
<HeaderStyle backcolor="#cccccc"></HeaderStyle>
<AlternatingRowStyle backcolor="#e8e8e8"></AlternatingRowStyle>
<Columns>
<asp:TemplateField HeaderText="Tire Name">
<ItemTemplate>
<asp:Label id="lblTireName" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Tire Sizes">
<ItemTemplate>
<asp:Label id="lblTireSizes" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Ply Rating">
<ItemTemplate>
<asp:Label id="lblPly" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Type">
<ItemTemplate>
<asp:Label id="lblType" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Rim">
<ItemTemplate>
<asp:Label id="lblRim" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="InfPsi">
<ItemTemplate>
<asp:Label id="lblInfPsi" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="InfKgf">
<ItemTemplate>
<asp:Label id="lblInfKgf" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Load Limits">
<ItemTemplate>
<asp:Label id="lblLoadLimits" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Section Width">
<ItemTemplate>
<asp:Label id="lblSectionWidth" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Overall Diameter">
<ItemTemplate>
<asp:Label id="lblOverDiameter" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Skid Depth">
<ItemTemplate>
<asp:Label id="lblSkidDep" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="MaxSpeed">
<ItemTemplate>
<asp:Label id="lblMaxSpeed" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="LWheel">
<ItemTemplate>
<asp:Label id="lblLWheel" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="LSteer">
<ItemTemplate>
<asp:Label id="lblSteer" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Tread">
<ItemTemplate>
<asp:Label id="lblTread" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</form>
</body>
</html>
Tag : .NET, MySQL, Web (ASP.NET), VB.NET, Windows
|
|
 |
 |
 |
 |
Date :
2013-10-28 09:39:50 |
By :
benz |
View :
1097 |
Reply :
5 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ช่วยหน่อยนะค่ะ อ่อน vb.net มากๆ
|
 |
 |
 |
 |
Date :
2013-10-28 09:50:25 |
By :
benz |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ช่วยหน่อยนะค่ะ ขอร้อง
|
 |
 |
 |
 |
Date :
2013-10-29 08:24:02 |
By :
benz |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ดูแล้วงงน่ะครับ ปกติก็น่าจะใช้พวก JOIN ได้น่ะครับ
|
 |
 |
 |
 |
Date :
2013-10-29 08:57:55 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ใช้ if เพื่อต่อ String คำสั่ง sql ครับ
ประมาณนี้ครับ ปรับปรุงเอา
Code (SQL)
sql = @"SELECT รายการขายสินค้า.วันที่นำมาขาย,รายการขายสินค้า.เลขที่รายการขาย,รายการขายสินค้า.ฤดูกาล,member.mem_id AS รหัสลูกค้า,member.hname+' '+member.fname+' '+member.lname AS ชื่อ,รายการขายสินค้า.สินค้า,รายการขายสินค้า.น้ำหนัก,รายการขายสินค้า.ราคากิโลกรัมละ,รายการขายสินค้า.เป็นเงินทั้งหมด FROM member,รายการขายสินค้า ";
sql += "WHERE member.fname LIKE '%" + txtname2.Text + "%' ";
sql += "AND member.mem_id LIKE '%" + txtmem2.Text + "%'";
if (goods.Checked == true)
{
sql += "AND รายการขายสินค้า.สินค้า = '" + สินค้า.Text + "'";
}
if (น้ำหนัก.Checked == true && bt1.Checked == false)
{
sql += "AND รายการขายสินค้า.น้ำหนัก " + นน1.Text + " " + นน2.Text + "";
}
if (น้ำหนัก.Checked == true && bt1.Checked == true)
{
sql += "AND รายการขายสินค้า.น้ำหนัก BETWEEN " + นน2.Text + " AND " + นน3.Text + " ";
}
if (เป็นเงิน.Checked == true && bt2.Checked == false)
{
sql += "AND รายการขายสินค้า.เป็นเงินทั้งหมด " + mn1.Text + " " + mn2.Text + "";
}
if (เป็นเงิน.Checked == true && bt2.Checked == true)
{
sql += "AND รายการขายสินค้า.เป็นเงินทั้งหมด BETWEEN " + mn2.Text + " AND " + mn3.Text + " ";
}
if (ราคา.Checked == true && bt3.Checked == false)
{
sql += "AND รายการขายสินค้า.ราคากิโลกรัมละ " + pr1.Text + " " + pr2.Text + "";
}
if (ราคา.Checked == true && bt3.Checked == true)
{
sql += "AND รายการขายสินค้า.ราคากิโลกรัมละ BETWEEN " + pr2.Text + " AND " + pr3.Text + " ";
}
if (ses.Checked == true)
{
sql += "AND รายการขายสินค้า.ฤดูกาล = " + ฤดูกาล.Text + "";
}
if (วันที่ขาย.Checked == true)
{
sql += "AND รายการขายสินค้า.วันที่นำมาขาย BETWEEN '" + d1 + "' AND '" + d2 + "'";
}
sql += "AND รายการขายสินค้า.mem_id = member.mem_id ORDER BY รายการขายสินค้า.วันที่นำมาขาย ASC";
|
 |
 |
 |
 |
Date :
2013-10-29 09:47:51 |
By :
54645 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|