|
 |
|
สอบถามมือใหม่ครับผม อยากดึงข้อมูลในดาต้าเบส มาแสดงใน Datagridview ครับ ทำตามตัวอย่างมันไม่ขึ้น |
|
 |
|
|
 |
 |
|
จากตัวอย่างที่มีในนี้ครับ
https://www.thaicreate.com/dotnet/c-sharp-dotnet-windows-form-application-winapp.html
ผมต้องการนำ ค่าใน ดาต้าเบสมาแสดงใน datagridview เฉยๆ ครับ ในที่นี้ทำแล้ว datagridview มันไม่ขึ้นอะไรเรย
แต่กดปุ่ม Check SQL สามารถเชื่อมต่อได้ ครับ
ผมทำมาหน้า frmHome ได้ดังนี้
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using MySql.Data.MySqlClient;
namespace WindowsFormsApplication1
{
public partial class frmHome : Form
{
public frmHome()
{
InitializeComponent();
}
private void frmHome_Load(object sender, EventArgs e)
{
BindDataGrid();
}
private void BindDataGrid()
{
DataTable dt = new DataTable();
string myConnection = "datasource=localhost;port=3306;username=root;password=1234;database=aprdb;";
MySqlConnection myConn = new MySqlConnection(myConnection);
MySqlDataAdapter myDataAdapter = new MySqlDataAdapter();
myConn.Open();
/*myDataAdapter.SelectCommand = new MySqlCommand("select * from table;", myConn);
MySqlCommandBuilder cb = new MySqlCommandBuilder(myDataAdapter);*/
MySqlCommand myCommand = myConn.CreateCommand();
myCommand.CommandText = "SELECT * FROM table;";
myCommand.CommandType = CommandType.Text;
myDataAdapter = new MySqlDataAdapter(myCommand);
myDataAdapter.Fill(dt);
//DataSet ds = new DataSet();
//MessageBox.Show("Connected");
myConn.Close();
this.dgName.DataSource = dt;
this.dgName.Columns.Clear();
DataGridViewTextBoxColumn column;
column = new DataGridViewTextBoxColumn();
column.DataPropertyName = "Username";
column.HeaderText = "Username";
column.Width = 50;
this.dgName.Columns.Add(column);
column = new DataGridViewTextBoxColumn();
column.DataPropertyName = "Name";
column.HeaderText = "Name";
column.Width = 100;
this.dgName.Columns.Add(column);
column = new DataGridViewTextBoxColumn();
column.DataPropertyName = "Email";
column.HeaderText = "Email";
column.Width = 150;
this.dgName.Columns.Add(column);
dt = null;
}
private void btn_add_Click(object sender, EventArgs e)
{
}
private void btn_edit_Click(object sender, EventArgs e)
{
}
private void btn_del_Click(object sender, EventArgs e)
{
}
private void btn_exit_Click(object sender, EventArgs e)
{
if (MessageBox.Show("Are you sure to exit program?", "Confirm.", MessageBoxButtons.YesNo, MessageBoxIcon.Question, MessageBoxDefaultButton.Button1) == DialogResult.Yes)
{
Application.Exit();
}
}
private void btn_check_Click(object sender, EventArgs e)
{
try
{
string myConnection = "datasource=localhost;port=3306;username=root;password=1234;database=aprdb;";
MySqlConnection myConn = new MySqlConnection(myConnection);
MySqlDataAdapter myDataAdapter = new MySqlDataAdapter();
myDataAdapter.SelectCommand = new MySqlCommand("select * from table;",myConn);
MySqlCommandBuilder cb = new MySqlCommandBuilder(myDataAdapter);
myConn.Open();
DataSet ds = new DataSet();
MessageBox.Show("Connected");
myConn.Close();
}
catch(Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
}
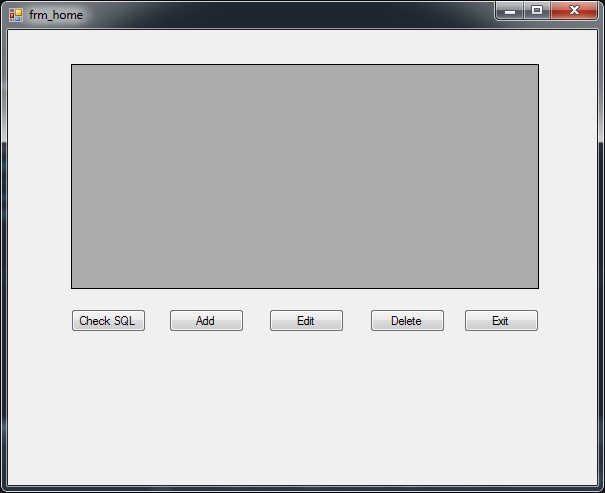
Tag : .NET, MySQL, Win (Windows App), C#, Windows

|
ประวัติการแก้ไข 2014-11-03 14:29:14
|
 |
 |
 |
 |
Date :
2014-11-03 14:28:19 |
By :
angelkiller9 |
View :
1150 |
Reply :
7 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
บรรทัดนี้ ลองเอาไปใส่ข้างล่างดูไหมครับ
myConn.Close();
this.dgName.DataSource = dt; <<<<< อันนี้ใส่ data เข้า gridview
this.dgName.Columns.Clear(); <<<<<< ส่วนอันนี้ clear มัน???
|
 |
 |
 |
 |
Date :
2014-11-03 15:03:34 |
By :
nongpaoza |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมว่าโค๊ดส่วนนี้
Code (C#)
this.dgName.Columns.Clear();
DataGridViewTextBoxColumn column;
column = new DataGridViewTextBoxColumn();
column.DataPropertyName = "Username";
column.HeaderText = "Username";
column.Width = 50;
this.dgName.Columns.Add(column);
column = new DataGridViewTextBoxColumn();
column.DataPropertyName = "Name";
column.HeaderText = "Name";
column.Width = 100;
this.dgName.Columns.Add(column);
column = new DataGridViewTextBoxColumn();
column.DataPropertyName = "Email";
column.HeaderText = "Email";
column.Width = 150;
this.dgName.Columns.Add(column);
แก้เป็นแบบนี้ดีไม๊ครับ
Code (C#)
this.dgName.Columns[0].Width = 50;
this.dgName.Columns[1].Width = 100;
this.dgName.Columns[2].Width = 150;
สั้นและแก้ปัญหา Columns Width ด้วยส่วน datatable ก็
Code (C#)
System.Data.DataTable GetTable( )
{
DataTable dt = new DataTable();
string myConnection = "datasource=localhost;port=3306;username=root;password=1234;database=aprdb;";
MySqlConnection myConn = new MySqlConnection(myConnection);
MySqlDataAdapter myDataAdapter = new MySqlDataAdapter();
myConn.Open();
/*myDataAdapter.SelectCommand = new MySqlCommand("select * from table;", myConn);
MySqlCommandBuilder cb = new MySqlCommandBuilder(myDataAdapter);*/
MySqlCommand myCommand = myConn.CreateCommand();
myCommand.CommandText = "SELECT * FROM table;";
myCommand.CommandType = CommandType.Text;
myDataAdapter = new MySqlDataAdapter(myCommand);
myDataAdapter.Fill(dt);
return dt;
}
|
 |
 |
 |
 |
Date :
2014-11-03 15:24:56 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
นายเขียนสลับกัน
นายต้องกำหนด column property ก่อน bind data
ปล. no.4 มั่วอีกแระ
|
 |
 |
 |
 |
Date :
2014-11-03 16:14:58 |
By :
ห้ามตอบเกินวันละ 2 กระทู้ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แสดงว่านายไม่รู้จัก autogeneratecolumn = false อ่ะดิ
|
 |
 |
 |
 |
Date :
2014-11-03 19:31:16 |
By :
ห้ามตอบเกินวันละ 2 กระทู้ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 00
|