|
 |
|
พอกดปุ่ม ลบข้อมุล ก็จะขึ้น MessageBox ที่แสดงรายระเอียด Error ต้องการทราบว่า Error เพราะอะไร ใช้ฐานข้อมูล Access |
|
 |
|
|
 |
 |
|
นี่คือภาพที่ได้หลังจากกดปุ่ม ลบ
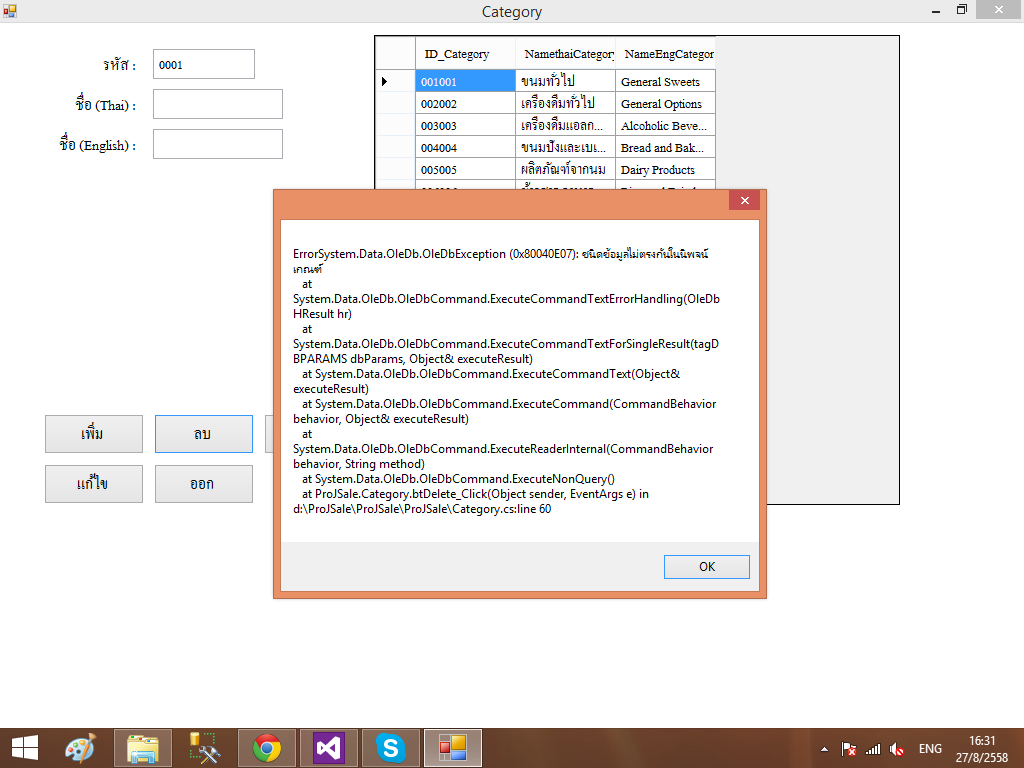
code ปุ่มลบ ข้อมูล
Code (C#)
private void btDelete_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "delete from Category where ID_Category = " + txtID_Category.Text + " ";
command.CommandText = query;
command.ExecuteNonQuery();
MessageBox.Show("Data Deleted");
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error" + ex);
}
}
รูปนี้ เป็นรูป ฐานข้อมูล Access
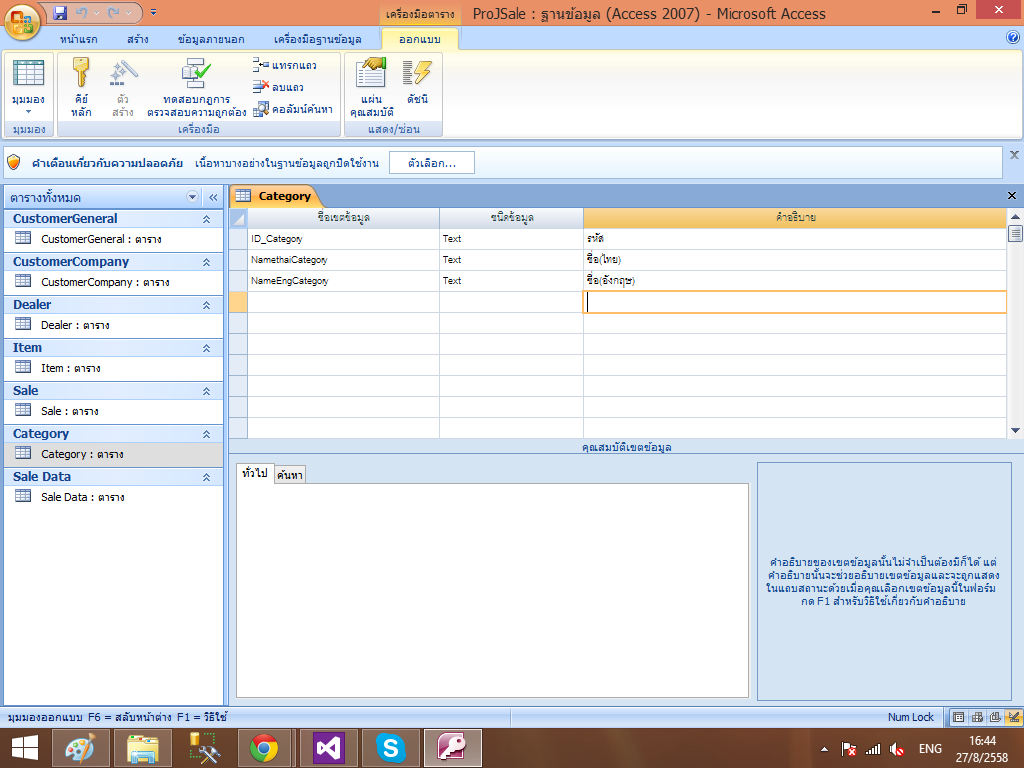
ส่วนด้านล่างนี้ เป็น code ท้ังหมดของ From นี้
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.OleDb;
namespace ProJSale
{
public partial class Category : Form
{
private OleDbConnection connection = new OleDbConnection();
public Category()
{
InitializeComponent();
connection.ConnectionString = @"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=D:\ProJSale\ProJSale\ProJSale.accdb;";
}
private void Customer_Load(object sender, EventArgs e)
{
// TODO: This line of code loads data into the 'proJSaleDataSet.Category' table. You can move, or remove it, as needed.
this.categoryTableAdapter.Fill(this.proJSaleDataSet.Category);
this.WindowState = FormWindowState.Maximized;
}
private void btInsert_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
command.CommandText = "insert into Category (ID_Category,NamethaiCategory,NameEngCategory) values('" + txtID_Category.Text + "','" + txtNameThaiCategory.Text + "','" + txtNameEngCategory.Text + "')";
command.ExecuteNonQuery();
MessageBox.Show("Data Saved");
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error" + ex);
}
}
private void btDelete_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "delete from Category where ID_Category = " + txtID_Category.Text + " ";
command.CommandText = query;
command.ExecuteNonQuery();
MessageBox.Show("Data Deleted");
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error" + ex);
}
}
private void btEdit_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "Update Category set ID_Category = '" + txtID_Category.Text + "' ,NamethaiCategory = '" + txtNameThaiCategory.Text + "' , where NameEngCategory = '" + txtNameEngCategory.Text + "'";
MessageBox.Show(query);
command.CommandText = query;
command.ExecuteNonQuery();
MessageBox.Show("Data Edit Successful");
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error" + ex);
}
}
private void btLoad_Click_1(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "select * from Category ";
command.CommandText = query;
OleDbDataAdapter da = new OleDbDataAdapter(command);
DataTable dt = new DataTable();
da.Fill(dt);
dataGridView1.DataSource = dt;
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error" + ex);
}
}
}
}
Tag : Ms Access, C#

|
ประวัติการแก้ไข 2015-08-27 16:45:57
|
 |
 |
 |
 |
Date :
2015-08-27 16:42:03 |
By :
sarasakza01 |
View :
960 |
Reply :
6 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้า MS Access ลองดูพวก ' ด้วยครับ คือถ้า Number จะต้องไม่ครอบ 'xxx'
|
 |
 |
 |
 |
Date :
2015-08-27 17:26:07 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ใช่ครับ เพราะมันรับได้เฉพาะ Number ถ้า 'xxx' มันมองว่าเป็น String ครับ
|
 |
 |
 |
 |
Date :
2015-08-28 09:02:58 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
string query = "delete from Category where ID_Category = '" + txtID_Category.Text + "'";
ก็ได้ครับ
แต่ขอถามนิดครับ
ID_Category. ใน access ได้กำหนดรูปแบบไว้ไม๊ครับ
ปล.ในช่องรหัสควรจะให้ตรงกับ ID_Category นะครับเพื่อความสะดวกต่อการพัฒนาขึ้นต่อไป
ไม่งั้นเด๋วเผลอ
string query = "delete from Category where ID_Category like '%" + txtID_Category.Text + "%'";
มันจะลบ ID_Category ที่มี 001 หมดนะครับ
    
|
 |
 |
 |
 |
Date :
2015-08-28 16:27:11 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ครับผม ขอบคุนนะครับทุกคนที่ช่วย ตอนนี้ สามารถ ลบ ได้แล้วครับ
|
 |
 |
 |
 |
Date :
2015-08-29 15:15:19 |
By :
sarasakza01 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 03
|