|
 |
|
เรื่องการส่งค่า parameter ในตอนกด Next Page Crystal Report |
|
 |
|
|
 |
 |
|
Code (C#)
if(!Page.IsPostBack())
{
ConfigureCrystalReports();
}
แบบนี้ได้ไหม๊ครับ
|
 |
 |
 |
 |
Date :
2015-11-24 15:07:19 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
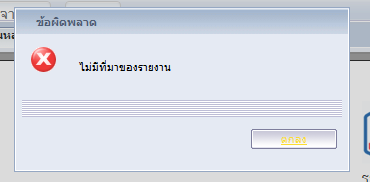
ขึ้นแบบนี้แทนตอนกด Next นะครับ ตอนนี้แก้จนงงหมดแล้วครับ
|
ประวัติการแก้ไข 2015-11-24 15:39:22
 |
 |
 |
 |
Date :
2015-11-24 15:38:39 |
By :
toey1 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอนนี้ถ้าไม่ต้องค่า parameter ไป สามารถใช้งานกด Next ได้ปกติ แต่ถ้าส่งค่า parameter ไปด้วย จะขึ้นหน้าให้กรอก parameter เวลากด Next
|
 |
 |
 |
 |
Date :
2015-11-24 16:12:09 |
By :
toey1 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รบกวนด้วยคราบ
|
 |
 |
 |
 |
Date :
2015-11-25 14:58:30 |
By :
toey1 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มีใครพอทราบไหมครับ
|
 |
 |
 |
 |
Date :
2015-11-26 15:35:21 |
By :
toey1 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 04
|