 |
|
C# WinApp ผมอยากหารูปแบบการแปลง string ให้เป็น datetime ครับ |
|
 |
|
|
 |
 |
|
  
|
 |
 |
 |
 |
Date :
2016-11-16 16:29:02 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (VB.NET)
Public Shared Function StringToDate(ByVal strDate As String, ByVal Date_Format As String, Optional ByVal Era_Type As String = "en-US") As Date
Dim dRet As Date
Return If(Date.TryParseExact(strDate, Date_Format, New CultureInfo(Era_Type), DateTimeStyles.None, dRet), dRet, Nothing)
End Function
Caller
Code (VB.NET)
Dim str2Date1 = StringToDate("31/12/2015", "dd/MM/yyyy", "en-US") --> 12/31/2015
Dim str2Date2 = StringToDate("31/12/2558", "dd/MM/yyyy", "th-TH") --> 12/31/2015
|
 |
 |
 |
 |
Date :
2016-11-17 18:52:47 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมจะเอาไปใช้กะวันหมดอายุสารเคมีครับ
บางที
จะมีแค่ nov/2016 หรือ 10/2016 บางเจ้ามีแค่ 2016 มาให้
ก็เลยจะดึง string ที่ว่ามาแปลงเป็นวันที่(ประมาณนั้น)
|
 |
 |
 |
 |
Date :
2016-11-17 19:34:53 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้า Dim str2Date1 = "1/12/2015"
แบบนี้เราจะรู้ได้ยังไงครับว่าเป็น เดือน 1 หรือ เดือน 12
หรือ อย่างบางที User ส่ง string มา แล้วเรามาบีบที่ format ผลออกมาก็มักจะเป็นแบบนี้
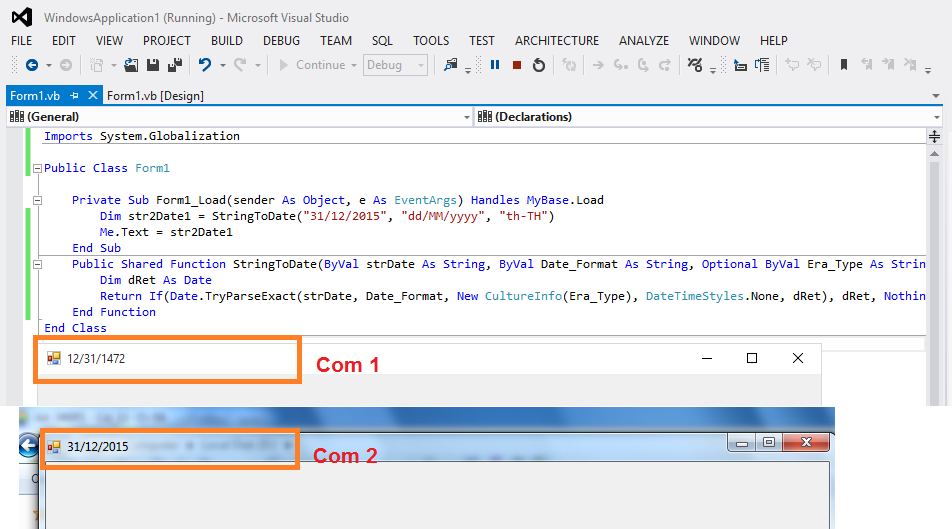
|
 |
 |
 |
 |
Date :
2016-11-18 08:51:02 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (VB.NET)
Me.Text = str2Date1
'(คุณพยายามที่จะทำให้ String = Date โดยขาดเหตุผลกำกับ)
'--- ถ้ามันถูกต้องคุณคงไม่มีรูปภาพ Com1, Com2 มาประกอบ
str2Date1 มันเป็นวันที่และวันที่ก็คือวันที่ไม่มีเป็นอย่างอื่น
--- แบบนี้เราจะรู้ได้ยังไงครับว่าเป็น เดือน 1 หรือ เดือน 12
--- ต่อให้รู้ว่ามันเป็นเดือน 1 หรือ เดือน 12 ก็ไม่มีประโยชน์อะไรเลย เหตุผลเพราะมันเป็นวันที่
Me.Text คุณคิดว่ามันเป็นวันที่หรือไม่ล่ะ?
การแสดงผลวันที่โดยมีเหตุผลกำกับ (การแปลงวันที่ให้เป็น String)
Code (VB.NET)
Me.Text = str2Date1.ToString("dd/MM/yyyy", New CultureInfo("th-TH")) 'ต้องการ พ.ศ.
Me.Text = str2Date1.ToString("dd/MM/yyyy", New CultureInfo("en-GB")) 'ต้องการ ค.ศ. (อังกฤษ)
Me.Text = str2Date1.ToString("MM/dd/yyyy", New CultureInfo("en-US")) 'ต้องการ ค.ศ. (อังกฤษ-US)
ปล. สิ่งที่ผมอธิบายมันตอบโจทย์คนได้ทั้งโลก ไม่เฉพาะตัวของคุณ
|
 |
 |
 |
 |
Date :
2016-11-18 11:36:06 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 7 เขียนโดย : หน้าฮี เมื่อวันที่ 2016-11-18 11:36:06
รายละเอียดของการตอบ ::
ผมคงเป็นส่วนน้อยที่มองว่าโลกกลมก็เป็นได้
ตอบความคิดเห็นที่ : 8 เขียนโดย : หน้าฮี เมื่อวันที่ 2016-11-18 11:50:08
รายละเอียดของการตอบ ::
ผมด่าคนเขียนไปเพราะ เขียนแล้ว user ใช้งานไม่เป็น ไม่คล่อง ไม่รู้จะมีอะไรเยอะแยะ ไม่อยากได้แบบนี้ มีแบบที่อยากได้ไม๊
เพราะฉะนั้น ผมจึงต้องเขียนเพื่อไม่ให้ผมต้องด่าตัวเองไปด้วย
ผมยังงงๆ อยู่มีซักกี่โปรแกรมที่เขียนแล้วเราไม่จะเป็นต้องไปนั่งสอน User อีกรอบในสิ่งที่ เขารู้แล้ว แต่คนเขียนทำให้มันยากขึ้น
ด้วยเหตุผลง่ายๆ สิ่งที่ผมอธิบายมันตอบโจทย์คนได้ทั้งโลก เพราะเราต้องไปนั่งอธิบาย อีกรอบนั่นเอง
ผมต้องการโปรแกรมง่ายๆ ที่ user จบ อนุบาล ก็ใช้งานได้ ขอแค่มัน รู้จัก คีย์บอร์ด และ เมาส์
หรือโปรแกรมที่ user เหมือนกับที่ งาน user ทำอยู่จน user ไม่รู้ซะด้วยซ้ำไปว่า ผมเปลี่ยน ระบบ อะไรไปบ้าง
และเป็นอีกสาเหตุที่ LIMS แพงหูดับจน lab บ้านๆไม่อาจเอื้อมทั้ง ๆ ที่จริงๆ แค่ เขามี access หรือ mysql บ้านๆ ก็สามารถเขียนระบบขึ้นมาได้แล้ว แถมบางแลบยังต้องยอมเปลี่ยนแปลงระบบตัวเองเพื่อให้เข้ากับโปรแกรมที่คนที่ไม่รู้เรื่องแลบเขียนมา
ใช้ 3 วัน แก้ ที 2 ปี แก้ 365 รอบ(ประมาณนั้น)
สำหรับผมคนนั้นก็แค่ ขายในสิ่งที่คนไม่ต้องการ(ชวนเชื่อว่ามันดี)แค่นั้นเอง
เหตุผลง่ายๆ ขนาดท่านก็ยังไม่สามารถตอบโจทย์นั้นได้ ก็คงยอมรับกรรม โยนให้ User รับผิดชอบไป
โดยที่อีก 2 วัน User ก็จะโทรมาถามว่าไอ้นี่มันยังไงรึ ไอ้นั่นทำไมไม่เหมือนเดิม ต้องทำยังไงต่อรึ ฯลฯ
แล้วท่านก็จะต้องตอบว่า อ่อๆๆๆ เดี๋ยวผมแก้ให้นะครับ จบคำสนทนา
ปล.ตั้งแต่เขียนโปรแกรมมา เคยใช้เองบ้างไม๊ครับ ท่านจะได้รู้ว่าเขียนโปรแกรมนั้นช่างไร้สาระสิ้นดี
ปล. ผมพูดจริง ที่ LIMS ไม่จำเป็นต้องเสียตังเขียน 3-10 ล้าน เพื่อให้ได้มาแค่ การจัดการตัวอย่างบ้านๆที่ปกติ User จ่ายตัง ไม่ถึงร้อยเพื่อซื้อสมุด ปากกา มาจด จริงไม๊ครับ
|
 |
 |
 |
 |
Date :
2016-11-18 13:46:19 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 7 เขียนโดย : หน้าฮี เมื่อวันที่ 2016-11-18 11:36:06
รายละเอียดของการตอบ ::
ปล. ลืมบอกไปครับ
ต่างกันเยอะนะครับ
คำถามกับคำตอบ(ไม่ได้เกี่ยวกันเลยก็ว่าได้)
ถึงแม้กระนั้นผมก็ยังอยากหาทางป้องกัน
เรื่อง string กับ datetime อีกต่อไป
ผมอยากรู้ว่า string ที่ป้อนเข้ามา สามารถทำให้เป็นรูป วันที่ได้หรือไม่ หากต่าง
หากมีใครสังเกต ใน excel ถ้าเรา พิมพ์ 1/1 หรือ 1-1 แล้ว enter จะได้ 1/1/2016 ผมไม่เห็นต้องมานั่งพิม 1/1/2016
หรือ หลายๆอย่าง ลองๆเล่นกันดู
excel ไม่ต้องถามเลยว่า คุณต้องใส่ format อย่างที่ฉันทำได้นะ เพราะความสามารถฉันมีแค่นี้ พน๊
ผมอยากให้โปรแกรม รับ string ที่ป้อนเข้ามาแล้ว
เช็คดูว่าสามารถเอามาทำเป็นวันที่ได้ไม๊ ไม่ใช่ รู้อยู่แล้วว่ามันต้องเป็น วันที่ แน่ๆ แล้วค่อยมา ทำเป็นวันที่อีกที
1ลาว1/2016 1/1/2016 1/1/xxx 1/2016 13/2016 ถ้าโปรแกรมแยกได้ string เป็นวันที่ก็ถือว่าตอบโจทย์นะ
ถ้าท่านยังงง ผมก็สามารถอธิบายให้ท่านกระจ่างได้เสมอนะ
ผมอยากให้ตอบคำถามผมมากกว่าที่จะออกเลไปแล้วก็ไม่เข้าฝั่ง
|
 |
 |
 |
 |
Date :
2016-11-18 15:14:41 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
คุณลองอ่านดูดีฯ
'(คุณพยายามที่จะทำให้ String = Date โดยขาดเหตุผลกำกับ)
'--- ถ้ามันถูกต้องคุณคงไม่มีรูปภาพ Com1, Com2 มาประกอบ
Code (VB.NET)
Me.Text = str2Date1
Code (C#)
this.Text = str2Date1
ทำไมผมถึงเน้น
Me.Text = String.Empty
Me.Text = "2016-12-32"
Me.Text = "2016-13-33"
...
...
...
ฝากให้เป็นการบ้าน ผมต้องการแปลงข้อความรูปแบบวันที่ "2559/12/31 08:30:20 AM +7" (ละเว้น D.C./B.C.)
ไปเป็นวันที่ของ US TimeZones Mountain Standard Time
ใช้ภาษาอะไรก็ได้ในการเขียน ผมอ่านออกหมดแหล่ะ
+55555
|
 |
 |
 |
 |
Date :
2016-11-18 15:31:11 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO14 คำนวณเล่นฯด้วยสายตา
วันเวลาไทย = "2559/12/31 08:30:20 AM +7"
วันเวลา US = "2016/12/31 15:30:20 AM -7"
จาก #NO13 1/1 ---> 1/1/2016
ถ้าจะทำมันก็ไม่ใช่เรื่องยาก
Code (VB.NET)
Dim dtFixed As Date = New Date(2016, 1, 1) 'yyyy/MM/dd
โลกของข้อมูลมันไม่ใช่เรื่องนึกอยากจะทำก็ทำได้ และไม่ใช่เรื่องเล่นฯ
ประเด็นก็คือ "เอาอำนาจมาจากไหนเพื่อแก้ไขข้อมูล"
--- ใครคือผู้ดำเนินการ
--- ใครคือผู้ตรวจสอบ
--- ใครคือผู้อนุมัติ
ระเบียนที่ ผู้ตรวจสอบ ผู้ดำเนินการ ผลการดำเนินการ ข้อมูลเก่า ข้อมูลใหม่ หมายเหตุ
1
2
3
...
...
...
หลักฐานทุกฯอย่างก่อนปรับปรุง/หลังปรับปรุง
ผู้อนุมัติ
ปล. การทำงานกับข้อมูลมันต้องรู้จักปกป้องตัวเองและเพื่อนร่วมงานด้วย
|
 |
 |
 |
 |
Date :
2016-11-19 10:01:34 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
วันเวลาไทย = "2559/12/31 08:30:20 AM +7"
วันเวลา US = "2016/12/31 15:30:20 AM -7"
Code (VB.NET)
Public Class Form1
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Dim str2Date1 = StringToDate("2559/12/31 08:30:20 AM +7", "dd/MM/yyyy", "th-TH")
Dim dateNow As Date = Date.Parse(str2Date1)
Me.Text = "The date and time are UTC =>" & TimeZoneInfo.ConvertTimeToUtc(dateNow)
End Sub
Public Shared Function StringToDate(ByVal strDate As String, ByVal Date_Format As String, Optional ByVal Era_Type As String = "en-US") As Date
Dim dRet As Date
Return If(Date.TryParseExact(strDate, Date_Format, New CultureInfo(Era_Type), DateTimeStyles.None, dRet), dRet, Nothing)
End Function
End Class
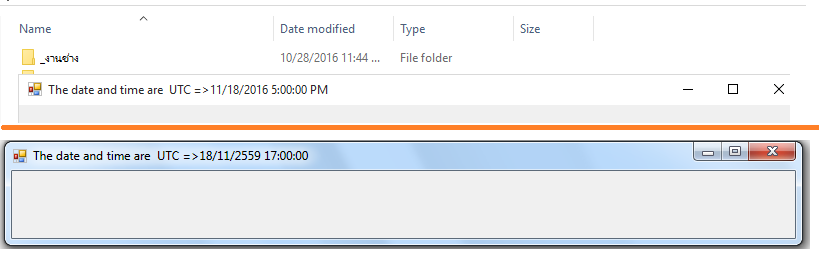
ผมก็ยังต้องหาทางแก้กันต่อไป
|
 |
 |
 |
 |
Date :
2016-11-19 11:16:58 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ที่ผมอยากได้คือความคาดเดา ที่สามารถจะเอา string มาทำเป็น วันที่ได้หรือไม่ได้
เมื่อ 100 ปีก่อนใครจะคิดว่าเราจะมี สมารทโฟนใช้กัน
คนส่วนใหญ่บอกว่าเป็นไปไม่ได้ ต้องใช้ โทรเลขเท่านั้น
แต่ก็มีคนส่วนน้อยที่รู้จัก NPN PNP และเอามันมาใช้ประโยชน์ในการ อ่านค่า 0 กับ 1
|
 |
 |
 |
 |
Date :
2016-11-19 11:23:04 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
หากจะย้อนไปไกลกว่านั้น
John Jacob Berzelius จะรู้ไม๊ว่าอีกเกือบ 200 ปี ธาตุที่เขาเป็นคนเริ่มต้นแยกไว้จะกลายมาเป็นสารกึ่งตัวนำแบบบ้านๆที่ใช้กันอยู่ทั่วไปบนโลกใบนี้
ท้ายที่สุดแล้ว ความรู้ ก็ไม่สุดสุดอยู่แค่รู้
แล้วความคิดเดิมๆก็จะค่อยๆหายไปตามกาลเวลา
|
 |
 |
 |
 |
Date :
2016-11-19 11:33:06 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ข้อมุลคุณ รูปแบบมันซ้ำซ้อนกันครับ แม้แต่คนยังแยกไม่ออกแล้ว คอมพิวเตอร์มันจะแยกออกได้ยังไงครับ
ผมเชื่อว่าสามัญสำนึกคุณบอกถูกต้องแล้วว่ามันแยกไม่ได้ อย่าไปหาเลยครับ วิธีการแยกข้อมูลที่ซ้ำซ้อนกัน
วิธีที่ง่ายที่สุดแต่โคตรนาน คือถามคนผลิดว่ารูปแบบมันเป็นยังไง
ต้องโทษที่เมือก่อนมันไม่มีมาตรฐานในการกำหนดวันที่นำเข้า มันก็เลยเป็นผลพวงให้งานต่อมามันลำบาก
(ป้ายผลิดมันมีมาตราฐานการพิมพ์อยู่ แต่คนคีย์ข้อมูลอาจจะไม่รู้ เขามีอักษรกำกับ เช่น ว / ด / ป มากับป้าย)
ข้อมูลใหม่ก็จัดรูปแบบให้ถูกต้อง ข้อมูลเก่าก็แก้กันไปเป็นเคสๆ อ่านป้ายกำกับของบริษัทให้ชัดเจน
รูปแบบมันพอแยกได้ เมื่อ
1 วันที่ มากกว่า 12
2 ถ้าปี < 2200 ให้เดาว่าเป็นปีค.ศ
3 ถ้าปี >2400 ให้เดาว่าเป็นปี พ.ศ
นอกจากนี้ต้องถามรูปแบบที่แน่นอนจากบริษัทผู้ผลิดโดยตรง
|
 |
 |
 |
 |
Date :
2016-11-19 12:42:36 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 20 เขียนโดย : Chaidhanan เมื่อวันที่ 2016-11-19 12:42:36
รายละเอียดของการตอบ ::
เอกสารผมบางอย่าง มี ว / ด / ป ไม่ครบ ครับ
บางทีมาแค่ 2016 หรือ 2559 หรือ 11/2016 หรือ 11/2559 (จะมาทำไมมาแค่ปีที่ผลิต หรือปีที่หมดอายุ)
ผมเลยกำลังหาวิธีที่จะเอา string พวกนี้ มาประเมิณ แล้วก็จัดรูปแบบให้เป็นวันที่
ข้อความแบบไหน เราถึงจะบอกว่า ข้อความนี้แหละเรียกว่าวันที่ ข้อความนี้ไม่ใช่นะ
ผมยังไม่ไปถึง พ.ศ. หรือ ค.ศ. เลย ครับ
สุดท้ายก็จะได้รูปแบบที่เราจะเอามาใช้ทำเป็น วันที่ได้ หรือไม่ได้ นั่นเอง
อย่าง 1/1 1-1 11/59 11-16 หรือ อื่นๆ
ถ้าเรายกตัวอย่างคนปกติก็ต้องร้องอ๋อ อยู่แล้ว แต่ส่วนที่ยังไม่ยกตัวอย่างละมันยังมีอีกไม๊
Code
คือผมอยากได้แบบนี้ครับ
1. 2015-10-16 หรือ 20151016 หรือ 16-10-2015 หรือ 16102015 ให้เป็น 2015-10-16 เป็นเรื่อง string
2. 2015-10 หรือ 201510 หรือ 10-2015 หรือ 102015 ให้เป็น 2015-10-01 เป็นเรื่อง string
3. 2015 หรือ 2015 หรือ 2015 หรือ 2015 ให้เป็น 2015-01-01 เป็นเรื่อง string
ตอนนี้ยังงงๆ อยู่ว่า
1. เราจะเช็ค ยังไงว่า string ที่รับ เป็นรูปแบบของ วันที่ หรือไม่ ครับ ข้อนี้ที่ยังไม่ได้
2. เราจะเช็ค ยังไงว่า string ที่รับ เป็นรูปแบบของ พศ./คศ. ครับ
แต่คำตอบที่ได้กลับกลายเป็นว่า เอา string มา convert เป็น datetime หรือ เปลี่ยน พ.ศ. เป็น ค.ศ.
ซึ่งมันข้ามขั้นตอนไป
อย่างถ้า เป็น 2559 หรือ 2016 ผมก็จะทำให้เป็น 01/01/2559 หรือ 01/01/2016
1/2559 หรือ 1/2016 ผมก็จะทำให้เป็น 01/01/2559 หรือ 01/01/2016
คราวนี้เราจะเอาไปทำเป็น พศ./คศ. ก็ว่ากันไป
คำถามคือ
เอ.... มันยังมีอีกไม๊ รูปแบบ string ที่เราจะมองมันเป็นวันที่ ได้ เหมือนอย่างที่เราพิมใน excel แล้วมันก็แปลงเป็นวันที่ให้เราเลย
|
 |
 |
 |
 |
Date :
2016-11-19 13:18:20 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
คุยกับคนที่ไม่ได้จบสายคอมพิวเตอร์มาโดยตรงมักจะเป็นแบบนี้เสมอ
--- 1 คนใน 5, 000 คน ที่ไม่ได้จบสายคอมพิวเตอร์โดยตรง และสามารถทำงานกับผมได้
|
 |
 |
 |
 |
Date :
2016-11-19 15:54:02 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ไอสไตล์เคยพูดว่า "สิ่งที่ยากที่สุดในชีวิตของผมและผมไม่เคยทำได้เลยก็คือ"
--- การทำให้คนจนหรือว่าคนโง่ "พึงพอใจ"
ไอสไตล์คิดได้ถูกต้อง
|
 |
 |
 |
 |
Date :
2016-11-19 16:07:45 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถามนิดครับ database เป็น mysql หรือ sqlserver
ถ้าเป็น sqlserver หา function regular expression เพิ่มเข้าไป
และใช้ regular express check รูปแบบ update ไปที่ละรูปแบบ
ตัวอย่าง mysql
Code (SQL)
ีupdate table set new_date = date( flddate), flddate = '0'
where flddate REGEXP '^(19|20)[0-9][09]/[0-9][09]/[0-9][09]'
and flddate<>'0'
Code (SQL)
ีupdate table set new_date = date( concat( flddate, '-01-01)), flddate = '0'
where flddate REGEXP '^(19|20)[0-9][0-9]'
and flddate<>'0'
Code (SQL)
ีupdate table set new_date = date( concat( (flddate-543), '-01-01)), flddate = '0'
where flddate REGEXP '^2[4-5][0-9][0-9]'
and flddate<>'0'
มีกี่ format ที่ยอมรับได้ ก็๋ค่อยๆทำไปที่ละดัน เดี๋ยวก็หมดเอง
ถ้าอยากรู้ว่าอันไหนที่ยังไม่ทำ ก็คิวรี่อันที่ flddate ไม่เท่ากับ 0 มาดู format เพื่อตรวจสอบ
|
 |
 |
 |
 |
Date :
2016-11-19 16:23:36 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ในเชิงโปรแกรม "คิดเอง ทำเอง แก้ไขปัญหาด้วยตัวเอง" เป็นสิ่งที่จำเป็น
Code (VB.NET)
Dim s As New System.Text.StringBuilder()
s.Append(" 2016/12 4 นา 12/13 12.13 หำ 6 กิโล มี (Jan. 13, 2012) Feb. 12, 12 – น้อย ")
s.Append(" January 15, 1999 July 15, 2923 June 2 2343 7/25/23 08/22/3323 มก. 33, 2333 ")
Dim p01 As String = "(?:(\d{1,2})/(\d{1,2})/(\d{2,4}))"
Dim p02 As String = "(?:(\s\d{1,2})\s+(jan(?:uary){0,1}\.{0,1}|feb(?:ruary){0,1}\.{0,1}|mar(?:ch){0,1}\.{0,1}|apr(?:il){0,1}\.{0,1}|may\.{0,1}|jun(?:e){0,1}\.{0,1}|jul(?:y){0,1}\.{0,1}|aug(?:ust){0,1}\.{0,1}|sep(?:tember){0,1}\.{0,1}|oct(?:ober){0,1}\.{0,1}|nov(?:ember){0,1}\.{0,1}|dec(?:ember){0,1}\.{0,1})\s+(\d{2,4}))"
Dim p03 As String = "(?:(jan(?:uary){0,1}\.{0,1}|feb(?:ruary){0,1}\.{0,1}|mar(?:ch){0,1}\.{0,1}|apr(?:il){0,1}\.{0,1}|may\.{0,1}|jun(?:e){0,1}\.{0,1}|jul(?:y){0,1}\.{0,1}|aug(?:ust){0,1}\.{0,1}|sep(?:tember){0,1}\.{0,1}|oct(?:ober){0,1}\.{0,1}|nov(?:ember){0,1}\.{0,1}|dec(?:ember){0,1}\.{0,1})\s+([0-9]{1,2})[\s,]+(\d{2,4}))"
Dim p04 As String = "(?:(มก(?:ราคม){0,1}\.{0,1}|กุม(?:ภาพันธ์){0,1}\.{0,1}|มี(?:นายน){0,1}\.{0,1}|เม(?:ษายน){0,1}\.{0,1}|พฤ(?:ษภาคม){0,1}\.{0,1}|มิ(?:ถุนายน){0,1}\.{0,1}|กรก(?:ฏาคม){0,1}\.{0,1}|สิง(?:หายน){0,1}\.{0,1}|กัน(?:ยายน){0,1}\.{0,1}|ตุ(?:ลาคม){0,1}\.{0,1}|พฤศ(?:จิกายน){0,1}\.{0,1}|ธัน(?:วาคม){0,1}\.{0,1})\s+([0-9]{1,2})[\s,]+(\d{2,4}))"
Dim p05 As String = "(?:(\d{1,2})/(\d{2,4}))" 'เดือน/ปี
Dim p06 As String = "(?:(\d{2,4})/(\d{1,2}))" 'ปี/เดือน
Dim p07 As String = "(?:(\d{1,2})-(\d{2,4}))" 'เดือน-ปี
Dim p08 As String = "(?:(\d{2,4})-(\d{1,2}))" 'ปี-เดือน
'...
'...
'...
'More...
Dim mc As MatchCollection = Regex.Matches(s.ToString(), p01 + "|" + p02 + "|" + p03 + "|" + p04 + "|" + p05 + "|" + p06 + "|" + p07 + "|" + p08, RegexOptions.IgnoreCase Or RegexOptions.IgnorePatternWhitespace)
Dim lstPattern = {New With {.Name = "Jan", .Value = "01"},
New With {.Name = "Jan.", .Value = "01"},
New With {.Name = "January", .Value = "01"},
New With {.Name = "ม.ค.", .Value = "01"},
New With {.Name = "มกราคม", .Value = "01"}
}
Dim lstSeparator = New Char() {"-"c, "/"c, "."c}
Dim strArray = "นา-หำ/มี.น้อย".Split(lstSeparator)
Dim sPattern = lstPattern.ToList().FindAll(Function(x) x.Name = "MatchCollection Value").FirstOrDefault()
MsgBox(mc.Count)
การทำให้ครอบคลุมในทุกฯกรณีมันไม่ใช่หน้าที่ของผม (ถ้าจะทำมันก็ไม่ใช่เรื่องยาก)
|
 |
 |
 |
 |
Date :
2016-11-21 11:02:53 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 27 แถมให้อีกหนึ่ง Pattern เอาให้เหมือนโปรแกรม Excel
ตรวจสอบ jan14 หรือ jan2014 หรือ January2014 หรือ january2014 หรือ january14
Code (VB.NET)
Dim p09 As String = "(?:(jan(?:uary){0,1})(\d{2,4}))"
'เดือนอื่นฯก็ดู p04 ประกอบ
|
 |
 |
 |
 |
Date :
2016-11-21 12:02:03 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
jan-59 =>1/1/1959
เป็นเรื่องน่าเศร้าที่ ระบบไม่มีทางแก้
jan-59 =>1/1/1959 หรือ 1/1/2559 หรือ 1/1/2359 หรือ 1/1/2659 หรือ 1/1/2459
   
|
 |
 |
 |
 |
Date :
2016-11-21 14:28:13 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #No1 - #NO29
สะสางงานของตัวเองได้ระดับหนึ่ง ปลีกวิเวกเพื่อให้มีเวลาว่าง 1 วัน
สร้าง Control DatetimePicker เอาไว้ใช้งาน (อยากทำมาตั้งนานแล้วแต่ไม่มีโอกาส/เวลาที่จะทำ)
--- ทำไมไม่ใช้ Control DatetimePicker ของ .NET ก็เป็นที่รู้ฯกัน
สิ่งที่ทำไปแล้วและเอาอยู่
--- คำนวณตำแหน่งแสดงผลบนหน้าจอได้ถูกต้อง ดังรูปภาพ
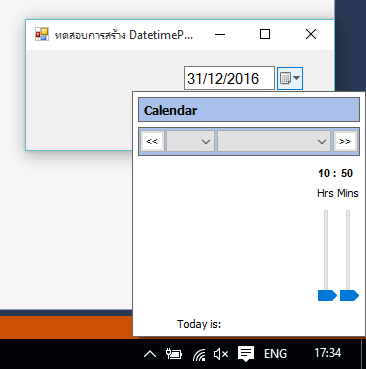
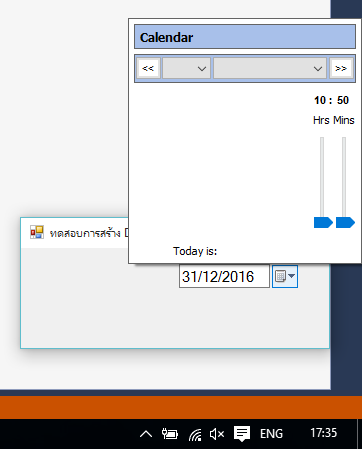
อันนี้เอาของฝรั่งมายำ
Code (VB.NET)
Imports System.Collections.Generic
Imports System.ComponentModel
Imports System.Data
Imports System.Drawing
Imports System.Text
Imports System.Windows.Forms
Imports System.Reflection
<ToolboxBitmap(GetType(System.Windows.Forms.ComboBox)), ToolboxItem(True), ToolboxItemFilter("System.Windows.Forms"), Description("Displays an editable text box with a drop-down list of permitted values.")> _
Partial Public Class myComboBox
Inherits System.Windows.Forms.ComboBox
Public Sub New()
InitializeComponent()
End Sub
Private Shared _modalMenuFilter As Type
Private Shared ReadOnly Property modalMenuFilter() As Type
Get
If _modalMenuFilter Is Nothing Then
_modalMenuFilter = Type.[GetType]("System.Windows.Forms.ToolStripManager+ModalMenuFilter")
End If
If _modalMenuFilter Is Nothing Then
_modalMenuFilter = New List(Of Type)(GetType(ToolStripManager).Assembly.GetTypes()).Find(Function(type__1) type__1.FullName = "System.Windows.Forms.ToolStripManager+ModalMenuFilter")
End If
Return _modalMenuFilter
End Get
End Property
Private Shared _suspendMenuMode As MethodInfo
Private Shared ReadOnly Property suspendMenuMode() As MethodInfo
Get
If _suspendMenuMode Is Nothing Then
Dim t As Type = modalMenuFilter
If t IsNot Nothing Then
_suspendMenuMode = t.GetMethod("SuspendMenuMode", BindingFlags.[Static] Or BindingFlags.NonPublic Or BindingFlags.[Public])
End If
End If
Return _suspendMenuMode
End Get
End Property
Private Shared Sub SuspendMenuModeX()
Dim suspendMenuMode__1 As MethodInfo = myComboBox.suspendMenuMode
If suspendMenuMode__1 IsNot Nothing Then
suspendMenuMode__1.Invoke(Nothing, Nothing)
End If
End Sub
Private Shared _resumeMenuMode As MethodInfo
Private Shared ReadOnly Property resumeMenuMode() As MethodInfo
Get
If _resumeMenuMode Is Nothing Then
Dim t As Type = modalMenuFilter
If t IsNot Nothing Then
_resumeMenuMode = t.GetMethod("ResumeMenuMode", BindingFlags.[Static] Or BindingFlags.NonPublic Or BindingFlags.[Public])
End If
End If
Return _resumeMenuMode
End Get
End Property
Private Shared Sub ResumeMenuModeX()
Dim resumeMenuMode__1 As MethodInfo = myComboBox.resumeMenuMode
If resumeMenuMode__1 IsNot Nothing Then
resumeMenuMode__1.Invoke(Nothing, Nothing)
End If
End Sub
Protected Overrides Sub OnDropDown(e As EventArgs)
MyBase.OnDropDown(e)
SuspendMenuModeX()
End Sub
Protected Overrides Sub OnDropDownClosed(e As EventArgs)
ResumeMenuModeX()
MyBase.OnDropDownClosed(e)
End Sub
End Class
อันนี้เอาของฝรั่งมายำ
Code (VB.NET)
Imports System.Drawing
Imports System.Runtime.InteropServices
Imports System.Windows.Forms
Imports System.Security
Imports System.Security.Permissions
Friend NotInheritable Class NativeMethods
Private Sub New()
End Sub
Private Shared HWND_TOPMOST As New HandleRef(Nothing, New IntPtr(-1))
Friend Const WM_NCHITTEST As Integer = &H84,
WM_NCACTIVATE As Integer = &H86,
WS_EX_TRANSPARENT As Integer = &H20,
WS_EX_TOOLWINDOW As Integer = &H80,
WS_EX_LAYERED As Integer = &H80000,
WS_EX_NOACTIVATE As Integer = &H8000000,
HTTRANSPARENT As Integer = -1,
HTLEFT As Integer = 10, HTRIGHT As Integer = 11,
HTTOP As Integer = 12, HTTOPLEFT As Integer = 13,
HTTOPRIGHT As Integer = 14,
HTBOTTOM As Integer = 15,
HTBOTTOMLEFT As Integer = 16,
HTBOTTOMRIGHT As Integer = 17,
WM_PRINT As Integer = &H317,
WM_USER As Integer = &H400,
WM_REFLECT As Integer = WM_USER + &H1C00,
WM_COMMAND As Integer = &H111,
CBN_DROPDOWN As Integer = 7,
WM_GETMINMAXINFO As Integer = &H24
<Flags> _
Friend Enum AnimationFlags As Integer
Roll = &H0
' Uses a roll animation.
HorizontalPositive = &H1
' Animates the window from left to right. This flag can be used with roll or slide animation.
HorizontalNegative = &H2
' Animates the window from right to left. This flag can be used with roll or slide animation.
VerticalPositive = &H4
' Animates the window from top to bottom. This flag can be used with roll or slide animation.
VerticalNegative = &H8
' Animates the window from bottom to top. This flag can be used with roll or slide animation.
Center = &H10
' Makes the window appear to collapse inward if Hide is used or expand outward if the Hide is not used.
Hide = &H10000
' Hides the window. By default, the window is shown.
Activate = &H20000
' Activates the window.
Slide = &H40000
' Uses a slide animation. By default, roll animation is used.
Blend = &H80000
' Uses a fade effect. This flag can be used only with a top-level window.
Mask = &HFFFFF
End Enum
<SuppressUnmanagedCodeSecurity> _
<DllImport("user32.dll", CharSet:=CharSet.Auto)> _
Private Shared Function AnimateWindow(windowHandle As HandleRef, time As Integer, flags As AnimationFlags) As Integer
End Function
Friend Shared Sub AnimateWindow(control As Control, time As Integer, flags As AnimationFlags)
Try
Dim sp As New SecurityPermission(SecurityPermissionFlag.UnmanagedCode)
sp.Demand()
AnimateWindow(New HandleRef(control, control.Handle), time, flags)
Catch generatedExceptionName As SecurityException
End Try
End Sub
<SuppressUnmanagedCodeSecurity> _
<DllImport("user32.dll", CharSet:=CharSet.Auto, ExactSpelling:=True)> _
Private Shared Function SetWindowPos(hWnd As HandleRef, hWndInsertAfter As HandleRef, x As Integer, y As Integer, cx As Integer, cy As Integer, _
flags As Integer) As Boolean
End Function
Friend Shared Sub SetTopMost(control As Control)
Try
Dim sp As New SecurityPermission(SecurityPermissionFlag.UnmanagedCode)
sp.Demand()
SetWindowPos(New HandleRef(control, control.Handle), HWND_TOPMOST, 0, 0, 0, 0, _
&H13)
Catch generatedExceptionName As SecurityException
End Try
End Sub
Friend Shared Function HIWORD(n As Integer) As Integer
Return CShort((n >> 16) And &HFFFF)
End Function
Friend Shared Function HIWORD(n As IntPtr) As Integer
Return HIWORD(CInt(CLng(n)))
End Function
Friend Shared Function LOWORD(n As Integer) As Integer
Return CShort(n And &HFFFF)
End Function
Friend Shared Function LOWORD(n As IntPtr) As Integer
Return LOWORD(CInt(CLng(n)))
End Function
<StructLayout(LayoutKind.Sequential)> _
Friend Structure MINMAXINFO
Public reserved As Point
Public maxSize As Size
Public maxPosition As Point
Public minTrackSize As Size
Public maxTrackSize As Size
End Structure
Private Shared _isRunningOnMono As System.Nullable(Of Boolean)
Public Shared ReadOnly Property IsRunningOnMono() As Boolean
Get
If Not _isRunningOnMono.HasValue Then
_isRunningOnMono = Type.[GetType]("Mono.Runtime") IsNot Nothing
End If
Return _isRunningOnMono.Value
End Get
End Property
End Class
|
 |
 |
 |
 |
Date :
2016-11-23 18:01:35 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ต่อจาก #NO 30
เกริ่นเล่าให้ฟังสักเล็กน้อย คิดในเชิงอุคมคติ "คนบางคนเกิดมาเพื่อเป็นผู้นำ คนบางคนเกิดมาเพื่อเป็นผู้ตาม"
--- ในชีวิตจริงมันอาจจะไม่เป็นอย่างนี้ก็ได้ อาจให้เหตุผลกำกับได้ว่า "โชคและวาสนาของคนมันแตกต่างกัน"
------ (คนคำนวณ หรือ จะสู้ฟ้าลิขิต)
--- คนบางคนเกิดมาเพื่องานโปรแกรมมิ่ง (แน่นอนว่าแทบทุกคน ส่ายหน้าเมื่ออายุมากขึ้น)
Code (VB.NET)
Imports System.Collections.Generic
Imports System.ComponentModel
Imports System.Data
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Text
Imports System.Windows.Forms
Imports System.Security.Permissions
Imports System.Runtime.InteropServices
Imports VS = System.Windows.Forms.VisualStyles
<CLSCompliant(True), ToolboxItem(False)> _
Partial Public Class Popup
Inherits ToolStripDropDown
#Region " Fields & Properties "
''' <summary>
''' Gets the content of the pop-up.
''' </summary>
Public Property Content() As Control
Get
Return m_Content
End Get
Private Set(value As Control)
m_Content = value
End Set
End Property
Private m_Content As Control
''' <summary>
''' Determines which animation to use while showing the pop-up window.
''' </summary>
Public Property ShowingAnimation() As PopupAnimations
Get
Return m_ShowingAnimation
End Get
Set(value As PopupAnimations)
m_ShowingAnimation = value
End Set
End Property
Private m_ShowingAnimation As PopupAnimations
''' <summary>
''' Determines which animation to use while hiding the pop-up window.
''' </summary>
Public Property HidingAnimation() As PopupAnimations
Get
Return m_HidingAnimation
End Get
Set(value As PopupAnimations)
m_HidingAnimation = value
End Set
End Property
Private m_HidingAnimation As PopupAnimations
''' <summary>
''' Determines the duration of the animation.
''' </summary>
Public Property AnimationDuration() As Integer
Get
Return m_AnimationDuration
End Get
Set(value As Integer)
m_AnimationDuration = value
End Set
End Property
Private m_AnimationDuration As Integer
''' <summary>
''' Gets or sets a value indicating whether the content should receive the focus after the pop-up has been opened.
''' </summary>
''' <value><c>true</c> if the content should be focused after the pop-up has been opened; otherwise, <c>false</c>.</value>
''' <remarks>If the FocusOnOpen property is set to <c>false</c>, then pop-up cannot use the fade effect.</remarks>
Public Property FocusOnOpen() As Boolean
Get
Return m_FocusOnOpen
End Get
Set(value As Boolean)
m_FocusOnOpen = value
End Set
End Property
Private m_FocusOnOpen As Boolean
''' <summary>
''' Gets or sets a value indicating whether pressing the alt key should close the pop-up.
''' </summary>
''' <value><c>true</c> if pressing the alt key does not close the pop-up; otherwise, <c>false</c>.</value>
Public Property AcceptAlt() As Boolean
Get
Return m_AcceptAlt
End Get
Set(value As Boolean)
m_AcceptAlt = value
End Set
End Property
Private m_AcceptAlt As Boolean
Private _host As ToolStripControlHost
Private _opener As Control
Private _ownerPopup As Popup
Private _childPopup As Popup
Private _resizableTop As Boolean
Private _resizableLeft As Boolean
Private _isChildPopupOpened As Boolean
Private _resizable As Boolean
''' <summary>
''' Gets or sets a value indicating whether the <see cref="PopupControl.Popup" /> is resizable.
''' </summary>
''' <value><c>true</c> if resizable; otherwise, <c>false</c>.</value>
Public Property Resizable() As Boolean
Get
Return _resizable AndAlso Not _isChildPopupOpened
End Get
Set(value As Boolean)
_resizable = value
End Set
End Property
Private _nonInteractive As Boolean
''' <summary>
''' Gets or sets a value indicating whether the <see cref="PopupControl.Popup"></see> acts like a transparent windows (so it cannot be clicked).
''' </summary>
''' <value>
''' <c>true</c> if the popup is noninteractive; otherwise, <c>false</c>.</value>
Public Property NonInteractive() As Boolean
Get
Return _nonInteractive
End Get
Set(value As Boolean)
If value <> _nonInteractive Then
_nonInteractive = value
If IsHandleCreated Then
RecreateHandle()
End If
End If
End Set
End Property
''' <summary>
''' Gets or sets a minimum size of the pop-up.
''' </summary>
''' <returns>An ordered pair of type <see cref="T:System.Drawing.Size" /> representing the width and height of a rectangle.</returns>
Public Shadows Property MinimumSize() As Size
Get
Return m_MinimumSize
End Get
Set(value As Size)
m_MinimumSize = value
End Set
End Property
Private Shadows m_MinimumSize As Size
''' <summary>
''' Gets or sets a maximum size of the pop-up.
''' </summary>
''' <returns>An ordered pair of type <see cref="T:System.Drawing.Size" /> representing the width and height of a rectangle.</returns>
Public Shadows Property MaximumSize() As Size
Get
Return m_MaximumSize
End Get
Set(value As Size)
m_MaximumSize = value
End Set
End Property
Private Shadows m_MaximumSize As Size
''' <summary>
''' Gets parameters of a new window.
''' </summary>
''' <returns>An object of type <see cref="T:System.Windows.Forms.CreateParams" /> used when creating a new window.</returns>
Protected Overrides ReadOnly Property CreateParams() As CreateParams
<SecurityPermission(SecurityAction.LinkDemand, Flags:=SecurityPermissionFlag.UnmanagedCode)> _
Get
Dim cp As CreateParams = MyBase.CreateParams
cp.ExStyle = cp.ExStyle Or NativeMethods.WS_EX_NOACTIVATE
If NonInteractive Then
cp.ExStyle = cp.ExStyle Or NativeMethods.WS_EX_TRANSPARENT Or NativeMethods.WS_EX_LAYERED Or NativeMethods.WS_EX_TOOLWINDOW
End If
Return cp
End Get
End Property
#End Region
#Region " Constructors "
''' <summary>
''' Initializes a new instance of the <see cref="PopupControl.Popup"/> class.
''' </summary>
''' <param name="content">The content of the pop-up.</param>
''' <remarks>
''' Pop-up will be disposed immediately after disposion of the content control.
''' </remarks>
''' <exception cref="T:System.ArgumentNullException"><paramref name="content" /> is <code>null</code>.</exception>
Public Sub New(content__1 As Control)
If content__1 Is Nothing Then
Throw New ArgumentNullException("content")
End If
Content = content__1
FocusOnOpen = True
AcceptAlt = True
ShowingAnimation = PopupAnimations.SystemDefault
HidingAnimation = PopupAnimations.None
AnimationDuration = 100
InitializeComponent()
AutoSize = False
DoubleBuffered = True
ResizeRedraw = True
_host = New ToolStripControlHost(content__1)
Padding = InlineAssignHelper(Margin, InlineAssignHelper(_host.Padding, InlineAssignHelper(_host.Margin, Padding.Empty)))
If NativeMethods.IsRunningOnMono Then
content__1.Margin = Padding.Empty
End If
MinimumSize = content__1.MinimumSize
content__1.MinimumSize = content__1.Size
MaximumSize = content__1.MaximumSize
content__1.MaximumSize = content__1.Size
Size = content__1.Size
If NativeMethods.IsRunningOnMono Then
_host.Size = content__1.Size
End If
TabStop = InlineAssignHelper(content__1.TabStop, True)
content__1.Location = Point.Empty
Items.Add(_host)
AddHandler content__1.Disposed, Sub(sender, e)
content__1 = Nothing
Dispose(True)
End Sub
AddHandler content__1.RegionChanged, Sub(sender, e)
UpdateRegion()
End Sub
AddHandler content__1.Paint, Sub(sender, e)
PaintSizeGrip(e)
End Sub
UpdateRegion()
End Sub
#End Region
#Region " Methods "
''' <summary>
''' Raises the <see cref="E:System.Windows.Forms.ToolStripItem.VisibleChanged"/> event.
''' </summary>
''' <param name="e">An <see cref="T:System.EventArgs"/> that contains the event data.</param>
Protected Overrides Sub OnVisibleChanged(e As EventArgs)
MyBase.OnVisibleChanged(e)
If NativeMethods.IsRunningOnMono Then
Return
End If
' in case of non-Windows
If (Visible AndAlso ShowingAnimation = PopupAnimations.None) OrElse (Not Visible AndAlso HidingAnimation = PopupAnimations.None) Then
Return
End If
Dim flags As NativeMethods.AnimationFlags = If(Visible, NativeMethods.AnimationFlags.Roll, NativeMethods.AnimationFlags.Hide)
Dim _flags As PopupAnimations = If(Visible, ShowingAnimation, HidingAnimation)
If _flags = PopupAnimations.SystemDefault Then
If SystemInformation.IsMenuAnimationEnabled Then
If SystemInformation.IsMenuFadeEnabled Then
_flags = PopupAnimations.Blend
Else
_flags = PopupAnimations.Slide Or (If(Visible, PopupAnimations.TopToBottom, PopupAnimations.BottomToTop))
End If
Else
_flags = PopupAnimations.None
End If
End If
If (_flags And (PopupAnimations.Blend Or PopupAnimations.Center Or PopupAnimations.Roll Or PopupAnimations.Slide)) = PopupAnimations.None Then
Return
End If
If _resizableTop Then
' popup is “inverted”, so the animation must be
If (_flags And PopupAnimations.BottomToTop) <> PopupAnimations.None Then
_flags = (_flags And Not PopupAnimations.BottomToTop) Or PopupAnimations.TopToBottom
ElseIf (_flags And PopupAnimations.TopToBottom) <> PopupAnimations.None Then
_flags = (_flags And Not PopupAnimations.TopToBottom) Or PopupAnimations.BottomToTop
End If
End If
If _resizableLeft Then
' popup is “inverted”, so the animation must be
If (_flags And PopupAnimations.RightToLeft) <> PopupAnimations.None Then
_flags = (_flags And Not PopupAnimations.RightToLeft) Or PopupAnimations.LeftToRight
ElseIf (_flags And PopupAnimations.LeftToRight) <> PopupAnimations.None Then
_flags = (_flags And Not PopupAnimations.LeftToRight) Or PopupAnimations.RightToLeft
End If
End If
flags = flags Or (NativeMethods.AnimationFlags.Mask And DirectCast(CInt(_flags), NativeMethods.AnimationFlags))
NativeMethods.SetTopMost(Me)
NativeMethods.AnimateWindow(Me, AnimationDuration, flags)
End Sub
''' <summary>
''' Processes a dialog box key.
''' </summary>
''' <param name="keyData">One of the <see cref="T:System.Windows.Forms.Keys" /> values that represents the key to process.</param>
''' <returns>
''' true if the key was processed by the control; otherwise, false.
''' </returns>
<UIPermission(SecurityAction.LinkDemand, Window:=UIPermissionWindow.AllWindows)> _
Protected Overrides Function ProcessDialogKey(keyData As Keys) As Boolean
If AcceptAlt AndAlso ((keyData And Keys.Alt) = Keys.Alt) Then
If (keyData And Keys.F4) <> Keys.F4 Then
Return False
Else
Close()
End If
End If
Dim processed As Boolean = MyBase.ProcessDialogKey(keyData)
If Not processed AndAlso (keyData = Keys.Tab OrElse keyData = (Keys.Tab Or Keys.Shift)) Then
Dim backward As Boolean = (keyData And Keys.Shift) = Keys.Shift
Content.SelectNextControl(Nothing, Not backward, True, True, True)
End If
Return processed
End Function
''' <summary>
''' Updates the pop-up region.
''' </summary>
Protected Sub UpdateRegion()
If Region IsNot Nothing Then
Region.Dispose()
Region = Nothing
End If
If Content.Region IsNot Nothing Then
Region = Content.Region.Clone()
End If
End Sub
''' <summary>
''' Shows the pop-up window below the specified control.
''' </summary>
''' <param name="control">The control below which the pop-up will be shown.</param>
''' <remarks>
''' When there is no space below the specified control, the pop-up control is shown above it.
''' </remarks>
''' <exception cref="T:System.ArgumentNullException"><paramref name="control"/> is <code>null</code>.</exception>
Public Overloads Sub Show(control As Control)
If control Is Nothing Then
Throw New ArgumentNullException("control")
End If
Show(control, control.ClientRectangle)
End Sub
''' <summary>
''' Shows the pop-up window below the specified area.
''' </summary>
''' <param name="area">The area of desktop below which the pop-up will be shown.</param>
''' <remarks>
''' When there is no space below specified area, the pop-up control is shown above it.
''' </remarks>
Public Overloads Sub Show(ByVal area As Rectangle)
_resizableTop = InlineAssignHelper(_resizableLeft, False)
Dim location As New Point(area.Left, area.Top + area.Height)
Dim screen__1 As Rectangle = Screen.FromControl(Me).WorkingArea
If location.X + Size.Width > (screen__1.Left + screen__1.Width) Then
_resizableLeft = True
location.X = (screen__1.Left + screen__1.Width) - Size.Width
End If
If location.Y + Size.Height > (screen__1.Top + screen__1.Height) Then
_resizableTop = True
location.Y -= Size.Height + area.Height
End If
'location = control.PointToClient(location);
Show(location, ToolStripDropDownDirection.BelowRight)
End Sub
''' <summary>
''' Shows the pop-up window below the specified area of the specified control.
''' </summary>
''' <param name="control">The control used to compute screen location of specified area.</param>
''' <param name="area">The area of control below which the pop-up will be shown.</param>
''' <remarks>
''' When there is no space below specified area, the pop-up control is shown above it.
''' </remarks>
''' <exception cref="T:System.ArgumentNullException"><paramref name="control"/> is <code>null</code>.</exception>
Public Overloads Sub Show(ByVal control As Control, ByVal area As Rectangle)
If control Is Nothing Then
Throw New ArgumentNullException("control")
End If
SetOwnerItem(control)
_resizableTop = InlineAssignHelper(_resizableLeft, False)
Dim location As Point = control.PointToScreen(New Point(area.Left, area.Top + area.Height))
Dim screen__1 As Rectangle = Screen.FromControl(control).WorkingArea
If location.X + Size.Width > (screen__1.Left + screen__1.Width) Then
_resizableLeft = True
location.X = (screen__1.Left + screen__1.Width) - Size.Width
End If
If location.Y + Size.Height > (screen__1.Top + screen__1.Height) Then
_resizableTop = True
location.Y -= Size.Height + area.Height
End If
location = control.PointToClient(location)
Show(control, location, ToolStripDropDownDirection.BelowRight)
End Sub
Private Sub SetOwnerItem(control As Control)
If control Is Nothing Then
Return
End If
If TypeOf control Is Popup Then
Dim popupControl As Popup = TryCast(control, Popup)
_ownerPopup = popupControl
_ownerPopup._childPopup = Me
OwnerItem = popupControl.Items(0)
Return
ElseIf _opener Is Nothing Then
_opener = control
End If
If control.Parent IsNot Nothing Then
SetOwnerItem(control.Parent)
End If
End Sub
''' <summary>
''' Raises the <see cref="E:System.Windows.Forms.Control.SizeChanged" /> event.
''' </summary>
''' <param name="e">An <see cref="T:System.EventArgs" /> that contains the event data.</param>
Protected Overrides Sub OnSizeChanged(e As EventArgs)
If Content IsNot Nothing Then
Content.MinimumSize = Size
Content.MaximumSize = Size
Content.Size = Size
Content.Location = Point.Empty
End If
MyBase.OnSizeChanged(e)
End Sub
''' <summary>
''' Raises the <see cref="E:System.Windows.Forms.Control.Layout" /> event.
''' </summary>
''' <param name="e">A <see cref="T:System.Windows.Forms.LayoutEventArgs" /> that contains the event data.</param>
Protected Overrides Sub OnLayout(e As LayoutEventArgs)
If Not NativeMethods.IsRunningOnMono Then
MyBase.OnLayout(e)
Return
End If
Dim suggestedSize As Size = GetPreferredSize(Size.Empty)
If AutoSize AndAlso suggestedSize <> Size Then
Size = suggestedSize
End If
SetDisplayedItems()
OnLayoutCompleted(EventArgs.Empty)
Invalidate()
End Sub
''' <summary>
''' Raises the <see cref="E:System.Windows.Forms.ToolStripDropDown.Opening" /> event.
''' </summary>
''' <param name="e">A <see cref="T:System.ComponentModel.CancelEventArgs" /> that contains the event data.</param>
Protected Overrides Sub OnOpening(e As CancelEventArgs)
If Content.IsDisposed OrElse Content.Disposing Then
e.Cancel = True
Return
End If
UpdateRegion()
MyBase.OnOpening(e)
End Sub
''' <summary>
''' Raises the <see cref="E:System.Windows.Forms.ToolStripDropDown.Opened" /> event.
''' </summary>
''' <param name="e">An <see cref="T:System.EventArgs" /> that contains the event data.</param>
Protected Overrides Sub OnOpened(e As EventArgs)
If _ownerPopup IsNot Nothing Then
_ownerPopup._isChildPopupOpened = True
End If
If FocusOnOpen Then
Content.Focus()
End If
MyBase.OnOpened(e)
End Sub
''' <summary>
''' Raises the <see cref="E:System.Windows.Forms.ToolStripDropDown.Closed"/> event.
''' </summary>
''' <param name="e">A <see cref="T:System.Windows.Forms.ToolStripDropDownClosedEventArgs"/> that contains the event data.</param>
Protected Overrides Sub OnClosed(e As ToolStripDropDownClosedEventArgs)
_opener = Nothing
If _ownerPopup IsNot Nothing Then
_ownerPopup._isChildPopupOpened = False
End If
MyBase.OnClosed(e)
End Sub
#End Region
#Region " Resizing Support "
''' <summary>
''' Processes Windows messages.
''' </summary>
''' <param name="m">The Windows <see cref="T:System.Windows.Forms.Message" /> to process.</param>
<SecurityPermission(SecurityAction.LinkDemand, Flags:=SecurityPermissionFlag.UnmanagedCode)> _
Protected Overrides Sub WndProc(ByRef m As Message)
'if (m.Msg == NativeMethods.WM_PRINT && !Visible)
'{
' Visible = true;
'}
If InternalProcessResizing(m, False) Then
Return
End If
MyBase.WndProc(m)
End Sub
''' <summary>
''' Processes the resizing messages.
''' </summary>
''' <param name="m">The message.</param>
''' <returns>true, if the WndProc method from the base class shouldn't be invoked.</returns>
<SecurityPermission(SecurityAction.LinkDemand, Flags:=SecurityPermissionFlag.UnmanagedCode)> _
Public Function ProcessResizing(ByRef m As Message) As Boolean
Return InternalProcessResizing(m, True)
End Function
<SecurityPermission(SecurityAction.LinkDemand, Flags:=SecurityPermissionFlag.UnmanagedCode)> _
Private Function InternalProcessResizing(ByRef m As Message, contentControl As Boolean) As Boolean
If m.Msg = NativeMethods.WM_NCACTIVATE AndAlso m.WParam <> IntPtr.Zero AndAlso _childPopup IsNot Nothing AndAlso _childPopup.Visible Then
_childPopup.Hide()
End If
If Not Resizable AndAlso Not NonInteractive Then
Return False
End If
If m.Msg = NativeMethods.WM_NCHITTEST Then
Return OnNcHitTest(m, contentControl)
ElseIf m.Msg = NativeMethods.WM_GETMINMAXINFO Then
Return OnGetMinMaxInfo(m)
End If
Return False
End Function
<SecurityPermission(SecurityAction.LinkDemand, Flags:=SecurityPermissionFlag.UnmanagedCode)> _
Private Function OnGetMinMaxInfo(ByRef m As Message) As Boolean
Dim minmax As NativeMethods.MINMAXINFO = DirectCast(Marshal.PtrToStructure(m.LParam, GetType(NativeMethods.MINMAXINFO)), NativeMethods.MINMAXINFO)
If Not MaximumSize.IsEmpty Then
minmax.maxTrackSize = MaximumSize
End If
minmax.minTrackSize = MinimumSize
Marshal.StructureToPtr(minmax, m.LParam, False)
Return True
End Function
Private Function OnNcHitTest(ByRef m As Message, contentControl As Boolean) As Boolean
If NonInteractive Then
'm.Result = DirectCast(NativeMethods.HTTRANSPARENT, IntPtr)
m.Result = New IntPtr(NativeMethods.HTTRANSPARENT)
Return True
End If
Dim x As Integer = Cursor.Position.X
' NativeMethods.LOWORD(m.LParam);
Dim y As Integer = Cursor.Position.Y
' NativeMethods.HIWORD(m.LParam);
Dim clientLocation As Point = PointToClient(New Point(x, y))
Dim gripBouns As New GripBounds(If(contentControl, Content.ClientRectangle, ClientRectangle))
Dim transparent As New IntPtr(NativeMethods.HTTRANSPARENT)
If _resizableTop Then
If _resizableLeft AndAlso gripBouns.TopLeft.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTTOPLEFT, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTTOPLEFT))
Return True
End If
If Not _resizableLeft AndAlso gripBouns.TopRight.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTTOPRIGHT, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTTOPRIGHT))
Return True
End If
If gripBouns.Top.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTTOP, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTTOP))
Return True
End If
Else
If _resizableLeft AndAlso gripBouns.BottomLeft.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTBOTTOMLEFT, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTBOTTOMLEFT))
Return True
End If
If Not _resizableLeft AndAlso gripBouns.BottomRight.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTBOTTOMRIGHT, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTBOTTOMRIGHT))
Return True
End If
If gripBouns.Bottom.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTBOTTOM, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTBOTTOM))
Return True
End If
End If
If _resizableLeft AndAlso gripBouns.Left.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTLEFT, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTLEFT))
Return True
End If
If Not _resizableLeft AndAlso gripBouns.Right.Contains(clientLocation) Then
'm.Result = If(contentControl, transparent, DirectCast(NativeMethods.HTRIGHT, IntPtr))
m.Result = If(contentControl, transparent, New IntPtr(NativeMethods.HTRIGHT))
Return True
End If
Return False
End Function
Private _sizeGripRenderer As VS.VisualStyleRenderer
''' <summary>
''' Paints the sizing grip.
''' </summary>
''' <param name="e">The <see cref="System.Windows.Forms.PaintEventArgs" /> instance containing the event data.</param>
Public Sub PaintSizeGrip(e As PaintEventArgs)
If e Is Nothing OrElse e.Graphics Is Nothing OrElse Not _resizable Then
Return
End If
Dim clientSize As Size = Content.ClientSize
Using gripImage As New Bitmap(&H10, &H10)
Using g As Graphics = Graphics.FromImage(gripImage)
If Application.RenderWithVisualStyles Then
If _sizeGripRenderer Is Nothing Then
_sizeGripRenderer = New VS.VisualStyleRenderer(VS.VisualStyleElement.Status.Gripper.Normal)
End If
_sizeGripRenderer.DrawBackground(g, New Rectangle(0, 0, &H10, &H10))
Else
ControlPaint.DrawSizeGrip(g, Content.BackColor, 0, 0, &H10, &H10)
End If
End Using
Dim gs As GraphicsState = e.Graphics.Save()
e.Graphics.ResetTransform()
If _resizableTop Then
If _resizableLeft Then
e.Graphics.RotateTransform(180)
e.Graphics.TranslateTransform(-clientSize.Width, -clientSize.Height)
Else
e.Graphics.ScaleTransform(1, -1)
e.Graphics.TranslateTransform(0, -clientSize.Height)
End If
ElseIf _resizableLeft Then
e.Graphics.ScaleTransform(-1, 1)
e.Graphics.TranslateTransform(-clientSize.Width, 0)
End If
e.Graphics.DrawImage(gripImage, clientSize.Width - &H10, clientSize.Height - &H10 + 1, &H10, &H10)
e.Graphics.Restore(gs)
End Using
End Sub
Private Shared Function InlineAssignHelper(Of T)(ByRef target As T, value As T) As T
target = value
Return value
End Function
#End Region
End Class
|
 |
 |
 |
 |
Date :
2016-11-23 19:52:18 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
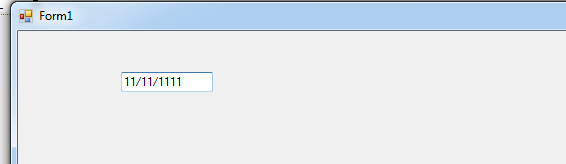
คือถ้าผมอยากให้ MaskedTextBox กด uo/down เพื่อเปลี่ยน วันที่ กับเดือนได้
ต้องทำยังไงรึครับ
เห็นบางโปรแกรม หรือแม่แต่ใน bios เองก็ทำได้
|
 |
 |
 |
 |
Date :
2016-11-23 19:54:51 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO xxx- 33
จริงฯแล้วต้นฉบับ Source Code ของผมเขียนด้วย C# เป็นหลัก (ผมแปลงเป็น VB.NET เล่นฯไปอย่างนั้นแหละ)
|
 |
 |
 |
 |
Date :
2016-11-23 20:23:56 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอนนี้ผมหันมาทำ Uncer ครับ
กำลังคิดอยู่ว่าถ้าเราใช้ Uncer จาก สารละลายมาตรฐานแบบตั้งต้น คำนวนผ่าน pipett และ flask
เช่น Cd 0.02 mg/L จะดึงเครื่องแก้วประจำปีมาใช้ได้ยังไง
แถมต้องทำ databasee แบบ save as โดยดึงข้อมูลเก่ามาแก้ไขแล้วก็ save as ดูท่าจะไม่ต่ำกว่า 8 ตาราง เป็นแน่
วุ่นวายพอดู 555
แต่ก็ดีที่รู้ว่า น่าจะเป็นโปรแกรมคำนวน Uncer 1 เดียวในไทย
เสร็จโปรแกรมนี้คงต้องกลับไปเขียนแบบ wood clock ต่อ ค้างเติ่งไว้หลายเพลาแล้วยังไม่ขึ้นเป็นรูปเป็นร่างซักที
|
 |
 |
 |
 |
Date :
2016-11-23 20:45:50 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
@lamaka.tor
--- ตามสะดวกครับ (โอกาสข้างหน้าที่เราจะมาชนกันมันยากยิ่งกว่าคำว่า "บังเอิญ")
อันนี้สุดท้ายและคิดว่าสมบูรณ์แล้ว (ผมอาจจะเอา TreeView หรือ DataGridView ยัดลงไปใน ComboBox/ตามที่ผมต้องการ)
Code (VB.NET)
<ToolboxBitmap(GetType(System.Windows.Forms.ComboBox)), ToolboxItem(True), ToolboxItemFilter("System.Windows.Forms"), Description("Displays an editable text box with a drop-down list of permitted values.")> _
Partial Public Class PopupComboBox
Inherits myComboBox
Public Sub New()
'this.dropDownHideTime = DateTime.UtcNow;
InitializeComponent()
Me.dropDownHideTime = DateTime.UtcNow
MyBase.DropDownHeight = InlineAssignHelper(MyBase.DropDownWidth, 1)
MyBase.IntegralHeight = False
End Sub
Private _dropDown As PopupControl.Popup
Private m_dropDownControl As Control
Public Property DropDownControl() As Control
Get
Return m_dropDownControl
End Get
Set(value As Control)
If m_dropDownControl Is value Then
Return
End If
m_dropDownControl = value
If _dropDown IsNot Nothing Then
RemoveHandler _dropDown.Closed, AddressOf dropDown_Closed
_dropDown.Dispose()
End If
_dropDown = New Popup(value)
AddHandler _dropDown.Closed, AddressOf dropDown_Closed
End Set
End Property
Private dropDownHideTime As DateTime
Private Sub dropDown_Closed(sender As Object, e As ToolStripDropDownClosedEventArgs)
dropDownHideTime = DateTime.UtcNow
End Sub
Public Shadows Property DroppedDown() As Boolean
Get
Return _dropDown.Visible
End Get
Set(value As Boolean)
If DroppedDown Then
HideDropDown()
Else
ShowDropDown()
End If
End Set
End Property
Public Shadows Event DropDown As EventHandler
Public Sub ShowDropDown()
If _dropDown IsNot Nothing Then
If (DateTime.UtcNow - dropDownHideTime).TotalSeconds > 0.5 Then
RaiseEvent DropDown(Me, EventArgs.Empty)
_dropDown.Show(Me)
Else
dropDownHideTime = DateTime.UtcNow.Subtract(New TimeSpan(0, 0, 1))
Focus()
End If
End If
End Sub
Public Shadows Event DropDownClosed As EventHandler
Public Sub HideDropDown()
If _dropDown IsNot Nothing Then
_dropDown.Hide()
RaiseEvent DropDownClosed(Me, EventArgs.Empty)
End If
End Sub
<SecurityPermission(SecurityAction.LinkDemand, Flags:=SecurityPermissionFlag.UnmanagedCode)> _
Protected Overrides Sub WndProc(ByRef m As Message)
If m.Msg = (NativeMethods.WM_COMMAND + NativeMethods.WM_REFLECT) AndAlso NativeMethods.HIWORD(m.WParam) = NativeMethods.CBN_DROPDOWN Then
BeginInvoke(New MethodInvoker(AddressOf ShowDropDown))
Return
End If
MyBase.WndProc(m)
End Sub
End Class
Good Luck.
|
 |
 |
 |
 |
Date :
2016-11-23 21:36:20 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|