 |
|
สอบถามการลบข้อมูลใน datagridview ที่ถูก Fill หน่อยครับ |
|
 |
|
|
 |
 |
|
คำถามอยู่ในภาพครับ..
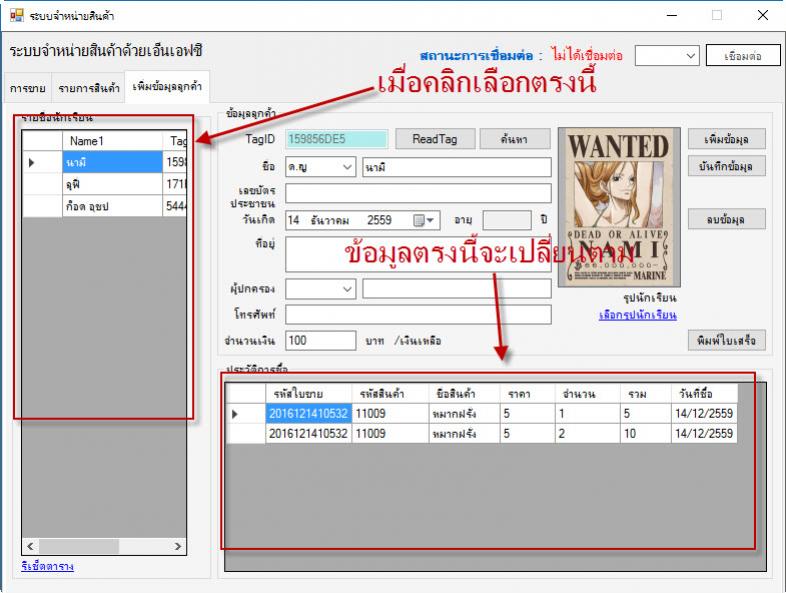
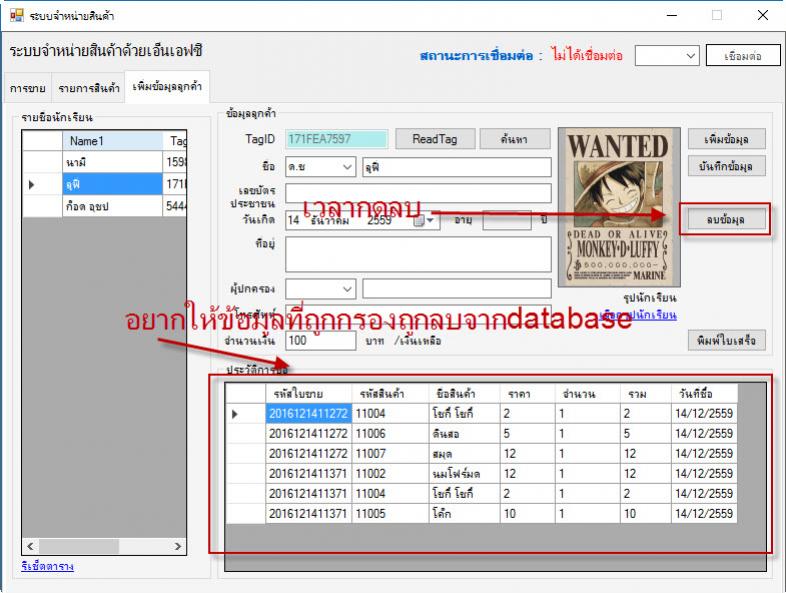
ส่วนของโค๊ด ตัวกรอง น่ะครับ..
Code (VB.NET)
Private Sub DataGridView1_CellEnter(sender As Object, e As DataGridViewCellEventArgs) Handles DataGridView1.CellEnter
Me.List2TableAdapter.Fill(Me.Database1DataSet.List2)
List2BindingSource.Filter = " TagID like '" & DataGridView1.Item(1, DataGridView1.CurrentRow.Index).Value.ToString.Trim & "%'"
End Sub
ส่วนของปุ่มลบครับ..( ควรเพิ่มคำสั่งอะไรลงในปุ่ม )
Code (VB.NET)
If MsgBox("คุณต้องการลบข้อมูลลูกค้า", MsgBoxStyle.YesNo, "ยืนยันการลบ") = MsgBoxResult.Yes Then
'List2BindingSource.RemoveSort()
'List2BindingSource.EndEdit()
'List2TableAdapter.Update(Database1DataSet)
MsgBox("ลบรายการเสร็จสิ้น", MsgBoxStyle.OkOnly, "ลบข้อมูล")
DataStudentsBindingSource.RemoveCurrent()
DataStudentsBindingSource.EndEdit()
DataStudentsTableAdapter.Update(Database1DataSet)
MsgBox("ลบข้อมูลเสร็จสิ้น", MsgBoxStyle.OkOnly, "ลบข้อมูล")
End If
รบกวนหน่อยคับ.. ติดจริงๆ
Tag : .NET, Ms Access, VB.NET, Windows
|
|
 |
 |
 |
 |
Date :
2016-12-14 12:16:15 |
By :
kongrx782 |
View :
3241 |
Reply :
14 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Dynamic Parameter (ความยืดหยุ่น)
Code (VB.NET)
Private Sub btnDeleteData_Click(sender As Object, e As EventArgs) Handles btnDeleteData.Click
'Dim strSQLSelect As String = "Select * From Products Where ProductID In ({0})"
Dim strSQLDelete As String = "Delete From TableProducts Where ProductID In ({0})"
'strSQLDelete == "Delete From TableProducts Where ProductID In(@P1, @P2, @P3, ...)"
Dim valueArray As String() = {"1, 2, 3, 4, 5, 6, 7, 8"} 'วนลูปอ่านค่า Keys จากตาราง DataGridView Filter
Dim params = valueArray.Select(Function(s, i) "@P" & i).ToArray()
Dim Inclause = String.Join(", ", params).TrimEnd(" ") 'Remove Last Blank space.
Dim connStr As String = "Data Source=yourPCName;Initial Catalog=yourDBName;User ID=sa;Password=หำ providerName=""System.Data.SqlClient"""
Dim con = New System.Data.SqlClient.SqlConnection(connStr)
'Dim cmd1 = New System.Data.SqlClient.SqlCommand(String.Format(strSQLSelect, Inclause), con)
Dim cmd2 = New System.Data.SqlClient.SqlCommand(String.Format(strSQLDelete, Inclause), con)
For i As Integer = 0 To valueArray.Length - 1
'cmd1.Parameters.AddWithValue(params(i), valueArray(i))
cmd2.Parameters.AddWithValue(params(i), valueArray(i))
Next
con.Open()
'Dim objReturn1 = cmd1.ExecuteNonQuery()
Dim objReturn2 = cmd2.ExecuteNonQuery()
End Sub
Good Luck my babe.
|
 |
 |
 |
 |
Date :
2016-12-14 13:35:03 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
วันนี้ผมขี้เกียจและถือโอกาสปรับปรุง SourceCode ของตัวเอง (ไม่ได้ปรับมานานมากแล้ว)
LINQ_LAMPDA_Extxt.vb
Code (VB.NET)
Public Module LINQ_LAMPDA_Extxt
'Returns only A where B does NOT exists for A.
<System.Runtime.CompilerServices.Extension> _
Public Function LeftExcludingJoin(Of TSource, TInner, TKey, TResult)(source As IEnumerable(Of TSource),
inner As IEnumerable(Of TInner),
pk As Func(Of TSource, TKey),
fk As Func(Of TInner, TKey),
result As Func(Of TSource, TInner, TResult)) As IEnumerable(Of TResult)
Dim _result As IEnumerable(Of TResult) = Enumerable.Empty(Of TResult)()
_result = From s In source Group Join i In inner On pk(s) Equals fk(i) Into joinData = Group
From left In joinData.DefaultIfEmpty() Where left Is Nothing
Select result(s, left)
Return _result
End Function
End Module
ตัวอย่างการใช้งาน
Code (VB.NET)
Dim leftList = {
New With {.ID = 1, .Name = "John", .Changed = False},
New With {.ID = 2, .Name = "Obama", .Changed = False},
New With {.ID = 3, .Name = "Kinton", .Changed = False}
}
Dim rightList = {
New With {.ID = 1, .Name = "John", .Changed = False},
New With {.ID = 3, .Name = "Kinton", .Changed = True},
New With {.ID = 4, .Name = "Payboy", .Changed = False},
New With {.ID = 5, .Name = "หำ", .Changed = False}
}
'ผลลัพธ์คือ {.ID = 2, .Name = "Obama", .Changed = False},
Dim resultLeftExcludingJoin = leftList.LeftExcludingJoin(rightList, Function(p) p.ID, Function(a) a.ID, _
Function(p, a) New With {Key .MyPerson = p, Key .MyAddress = a}) _
.Select(Function(a) New With { _
Key .LeftID = (If(a.MyPerson IsNot Nothing, a.MyPerson.ID & " Left", "Null-Value")), _
Key .RightID = (If(a.MyAddress IsNot Nothing, a.MyAddress.ID & " Right", "Null-Value")), _
Key .LeftName = (If(a.MyPerson IsNot Nothing, a.MyPerson.Name & " Left", "Null-Value")), _
Key .RightName = (If(a.MyAddress IsNot Nothing, a.MyAddress.Name & " Right", "Null-Value")), _
Key .LeftChanged = (If(a.MyPerson IsNot Nothing, a.MyPerson.Changed & " Left", -1)), _
Key .RightChanged = (If(a.MyAddress IsNot Nothing, a.MyAddress.Changed & " Left", -1))
}).ToList()
|
 |
 |
 |
 |
Date :
2016-12-14 13:57:30 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO6 เผื่อพวกคุณมองภาพไม่ออก
--- Concept ของผมคือ ผมจะไม่ดึงข้อมูลจาก Database เป็นครั้งที่ 2 และผมจะหลีกเลี่ยงการ Join บน Server Database.
------ เพื่ออะไร?
------ 1. ประหยัด Memory/etc..
------ 2. ความเร็ว (อย่างน้อยฯ > เดิม 10 เท่า)
ผมดึงข้อมูลที (ไม่ได้ดึงหะมอย) เป็นล้านฯระเบียน
|
 |
 |
 |
 |
Date :
2016-12-14 14:09:39 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 7 สมมุติว่าผมกลับไปเรียนใหม่อีกครั้งหนึ่ง (ทุกมหาวิทยาลัยในเมืองไทนี้)
--- ผมคงได้เกรด 0 ทุกวิชาเรียน 100%
--- (มันไม่มีความยุติธรรมสำหรับตัวของผมเลย)
ปล. ผมใช้หลักการ "ไร้ซึ่งทฤษฏีมารองรับ"
|
 |
 |
 |
 |
Date :
2016-12-14 14:14:58 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เล่าเรื่องจริงให้ฟังนะ (+55555)
โปรเจคระดับ >= 50 ล้าน <= ร้อยล้านบาท
--- ใช้ SAแค่คนเดียว (รุ่นน้องของผม/ตัวผมบ้างเป็นบางครั้ง)
--- ใช้โปรแกรมเมอร์แค่คนเดียว (ตัวตรูคนเดียวนี่แหละ)
ที่เหลือผมก็ไปจ้าง "วินมอเตอร์ไซท์ ปากซอย" สิบคนยี่สิบคนก็ว่ากันไป
--- คืนวานได้แค่ 3 ประตู (เงินไม่พอแอก)
--- แน่นอนผมก็หาสูทรและผูกไทร์ให้ครบ
ที่เหลือผมก็ไปจ้าง "น้องโบก ตามผลับตามบาร์" สิบคนยี่สิบคนก็ว่ากันไป
--- แน่นอนผมก็หาสูทรและผูกไทร์ให้ครบ
+55555 (พวกผมไปเป็นทีม) และไม่มีซ้ำหน้า (+55555)
--- มันดูดีและน่าเชื่อถือ (บริษัทแม่งโครตใหญ่โต พนักงานมีเป็น 1,000)
+55555
|
 |
 |
 |
 |
Date :
2016-12-14 14:56:37 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO10 อันนี้เป็นความรู้นอกตำราเลยนะนั่น (แม้แต่ ไอสไตล์ยังยอมสยบ)
--- ห้องประชุมมันต้องจองห้องโรงแรมใหญ่ฯ (เฉพาะทีมงานของผมก็มีเป็นพันฯ)
--- บรรยากาศในห้องประชุม
------ ผมทันยังไม่ทันเปิดคอมพิวเตอร์ (โปรเจคเตอร์ปลั๊กยังไม่ได้เสียบเลย)
------ โปรแกรมของคุณสุดยอด ตรงตามที่หน่วยงานของเราต้องการ +55555
--------- ไม่รู้ว่า "น้องโบก" มันไปแอบทำหน้าที่ตอนไหน?
|
 |
 |
 |
 |
Date :
2016-12-14 15:04:14 |
By :
หน้าอี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
[b]จาก #NO9 - #NO 11[/b]
พวกคุณดูพละกำลังสมองของผม (เมื่อวานผมแดกเบียร์ไป 2 ลัง/ วันนี้กำลังแดก ขวดที่ 7)
--- คิดไปข้างหน้าได้ ก็ต้องคิดย้อนหลังได้ (สภาวะการณ์การเอาตัวรอด)
สมมุติว่ามีคนไทยบางคนชื่อ
--- ชื่อ นายอำเพ้อ
--- ชื่อ นายผู้หวา
--- ชื่อ ผอเขต
--- ชื่อ รองผอเขต
--- ชื่อ นายสิบ
--- ชื่อ นายร้อย
--- ชื่อ นายพัน
...
...
...
บางคนก็มีชื่อว่า : ผัวกูชื่อพลอาสาฯ
บางคนก็มีชื่อว่า : ...
--- ผมจำเป็นต้องจ้าง "น้องโบก" ไหม?
------ น้องโบกทุกสำนักแย่งกันมาทำงานกับผม
------ ผม(ตรูฟันฟรีมาหมดแล้ว) และข้อเสนอเพิ่มพิเศษ "หนูให้พี่หมื่นหนึ่งนะ"
------ เจอหน้าผม น้องโบก "รีบขอบคุณ/ขอบใจผม"
ขอบคุณพี่มากนะ(น้องโบกทุกคนเลย) สำหรับโอกาสที่พี่หยิบยื่นให้/ผมก็ไม่ลืมยื่นให้เหมือนกัน
--- อยู่ที่น้องโบกจะเอามือหยิบหรือเอาปากหยิบในส่ิงที่ผมยื่นให้ (+55555 มันยื่นอะไรหว่า?)
...
...
...
|
 |
 |
 |
 |
Date :
2016-12-14 15:47:49 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รบกวนหน่อยครับ. คือว่า ผมยังลบข้อมูลของอีกตารางไม่ได้เลย.. ยัง งง..
|
 |
 |
 |
 |
Date :
2016-12-16 13:24:07 |
By :
kongrx782 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|