|
 |
|
รบกวนขอวิธี สั่งพิมพ์ textbox 5 ครั้ง ให้ random ตัวเลขที่อยู่ใน textbox โดยนับจาก textbox อันแรก |
|
 |
|
|
 |
 |
|
ลองศึกษาการเพิ่มหน้ากระดาษ ดูครับ
ปล. นี่ไม่ใช่การ random นะครับ เพราะมันเพิ่มขึ้นทีละ 1 แต่คนละหน้า เหมือนเราใส่หน้ากระดาษ ครับ
|
 |
 |
 |
 |
Date :
2017-04-01 12:37:10 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace TORServices.DatabaseTor
{
public class PrintDataGridView
{
#region Variables
int iCellHeight = 0; //Used to get/set the datagridview cell height
int iTotalWidth = 0; //
int iRow = 0;//Used as counter
bool bFirstPage = false; //Used to check whether we are printing first page
bool bNewPage = false;// Used to check whether we are printing a new page
int iHeaderHeight = 0; //Used for the header height
System.Drawing.StringFormat strFormat; //Used to format the grid rows.
List<double> arrColumnLefts = new List<double>();//Used to save left coordinates of columns
List<double> arrColumnWidths = new List<double>();//Used to save column widths
private System.Drawing.Printing.PrintDocument _printDocument = new System.Drawing.Printing.PrintDocument();
private System.Windows.Forms.DataGridView gw = new System.Windows.Forms.DataGridView();
private string _ReportHeader;
int iCount = 0;
#endregion
public PrintDataGridView(System.Windows.Forms.DataGridView gridview, string ReportHeader)
{
_printDocument.PrintPage += new System.Drawing.Printing.PrintPageEventHandler(printDocument_PrintPage);
_printDocument.BeginPrint += new System.Drawing.Printing.PrintEventHandler(printDocument_BeginPrint);
gw = gridview;
_ReportHeader = ReportHeader;
}
public void PrintForm()
{
System.Windows.Forms.PrintDialog printDialog = new System.Windows.Forms.PrintDialog();
printDialog.Document = _printDocument;
printDialog.UseEXDialog = true;
//Get the document
if (System.Windows.Forms.DialogResult.OK == printDialog.ShowDialog())
{
_printDocument.DocumentName = _ReportHeader + string.Format("{0:yyyyMMdd hhmmss}", DateTime.Now);
_printDocument.Print();
}
}
#region Begin Print Event Handler
/// <span class="code-SummaryComment"><summary></span>
/// Handles the begin print event of print document
/// <span class="code-SummaryComment"></summary></span>
/// <span class="code-SummaryComment"><param name=""sender""></param></span>
/// <span class="code-SummaryComment"><param name=""e""></param></span>
private void printDocument_BeginPrint(object sender,
System.Drawing.Printing.PrintEventArgs e)
{
strFormat = new System.Drawing.StringFormat();
strFormat.Alignment = System.Drawing.StringAlignment.Near;
strFormat.LineAlignment = System.Drawing.StringAlignment.Center;
strFormat.Trimming = System.Drawing.StringTrimming.EllipsisCharacter;
if (arrColumnLefts != null) arrColumnLefts.Clear();
if (arrColumnWidths != null) arrColumnWidths.Clear();
arrColumnLefts = new List<double>();
arrColumnWidths = new List<double>();
iCellHeight = 0;
iCount = 0;
bFirstPage = true;
bNewPage = true;
// Calculating Total Widths
iTotalWidth = 0;
foreach (System.Windows.Forms.DataGridViewColumn dgvGridCol in gw.Columns)
{
iTotalWidth += dgvGridCol.Width;
}
}
#endregion
#region Print Page Event
/// <span class="code-SummaryComment"><summary></span>
/// Handles the print page event of print document
/// <span class="code-SummaryComment"></summary></span>
/// <span class="code-SummaryComment"><param name=""sender""></param></span>
/// <span class="code-SummaryComment"><param name=""e""></param></span>
private void printDocument_PrintPage(object sender,
System.Drawing.Printing.PrintPageEventArgs e)
{
//Set the left margin
int iLeftMargin = 50;// e.MarginBounds.Left;
//Set the top margin
int iTopMargin = e.MarginBounds.Top;
//Whether more pages have to print or not
bool bMorePagesToPrint = false;
int iTmpWidth = 0;
//For the first page to print set the cell width and header height
if (bFirstPage)
{
foreach (System.Windows.Forms.DataGridViewColumn GridCol in gw.Columns)
{
iTmpWidth = (int)(System.Math.Floor((double)((double)GridCol.Width /
(double)iTotalWidth * (double)iTotalWidth *
((double)e.MarginBounds.Width / (double)iTotalWidth))));
iHeaderHeight = (int)(e.Graphics.MeasureString(GridCol.HeaderText,
GridCol.InheritedStyle.Font, iTmpWidth).Height) + 11;
// Save width and height of headers
arrColumnLefts.Add(iLeftMargin);
arrColumnWidths.Add(iTmpWidth);
iLeftMargin += iTmpWidth;
}
}
//Loop till all the grid rows not get printed
while (iRow <= gw.Rows.Count - 1)
{
System.Windows.Forms.DataGridViewRow GridRow = gw.Rows[iRow];
//Set the cell height
iCellHeight = GridRow.Height + 5;
int iCount = 0;
//Check whether the current page settings allows more rows to print
if (iTopMargin + iCellHeight >= e.MarginBounds.Height + e.MarginBounds.Top)
{
bNewPage = true;
bFirstPage = false;
bMorePagesToPrint = true;
break;
}
else
{
if (bNewPage)
{
//Draw Header
e.Graphics.DrawString(_ReportHeader,
new System.Drawing.Font(gw.Font, System.Drawing.FontStyle.Bold),
System.Drawing.Brushes.Black, e.MarginBounds.Left,
e.MarginBounds.Top - e.Graphics.MeasureString(_ReportHeader,
new System.Drawing.Font(gw.Font, System.Drawing.FontStyle.Bold),
e.MarginBounds.Width).Height - 13);
String strDate = DateTime.Now.ToLongDateString() + " " +
DateTime.Now.ToShortTimeString();
//Draw Date
e.Graphics.DrawString(strDate,
new System.Drawing.Font(gw.Font, System.Drawing.FontStyle.Bold), System.Drawing.Brushes.Black,
e.MarginBounds.Left +
(e.MarginBounds.Width - e.Graphics.MeasureString(strDate,
new System.Drawing.Font(gw.Font, System.Drawing.FontStyle.Bold),
e.MarginBounds.Width).Width),
e.MarginBounds.Top - e.Graphics.MeasureString(_ReportHeader,
new System.Drawing.Font(new System.Drawing.Font(gw.Font, System.Drawing.FontStyle.Bold),
System.Drawing.FontStyle.Bold), e.MarginBounds.Width).Height - 13);
//Draw Columns
iTopMargin = e.MarginBounds.Top;
foreach (System.Windows.Forms.DataGridViewColumn GridCol in gw.Columns)
{
e.Graphics.FillRectangle(new System.Drawing.SolidBrush(System.Drawing.Color.LightGray),
new System.Drawing.Rectangle((int)arrColumnLefts[iCount], iTopMargin,
(int)arrColumnWidths[iCount], iHeaderHeight));
e.Graphics.DrawRectangle(System.Drawing.Pens.Black,
new System.Drawing.Rectangle((int)arrColumnLefts[iCount], iTopMargin,
(int)arrColumnWidths[iCount], iHeaderHeight));
e.Graphics.DrawString(GridCol.HeaderText,
GridCol.InheritedStyle.Font,
new System.Drawing.SolidBrush(GridCol.InheritedStyle.ForeColor),
new System.Drawing.RectangleF((int)arrColumnLefts[iCount], iTopMargin,
(int)arrColumnWidths[iCount], iHeaderHeight), strFormat);
iCount++;
}
bNewPage = false;
iTopMargin += iHeaderHeight;
}
iCount = 0;
//Draw Columns Contents
foreach (System.Windows.Forms.DataGridViewCell Cel in GridRow.Cells)
{
if (Cel.Value != null)
{
e.Graphics.DrawString(Cel.Value.ToString(),
Cel.InheritedStyle.Font,
new System.Drawing.SolidBrush(Cel.InheritedStyle.ForeColor),
new System.Drawing.RectangleF((int)arrColumnLefts[iCount],
(float)iTopMargin,
(int)arrColumnWidths[iCount], (float)iCellHeight),
strFormat);
}
//Drawing Cells Borders
e.Graphics.DrawRectangle(System.Drawing.Pens.Black,
new System.Drawing.Rectangle((int)arrColumnLefts[iCount], iTopMargin,
(int)arrColumnWidths[iCount], iCellHeight));
iCount++;
}
}
iRow++;
iTopMargin += iCellHeight;
}
//If more lines exist, print another page.
if (bMorePagesToPrint)
e.HasMorePages = true;
else
e.HasMorePages = false;
}
#endregion
}
}
มองประเด็นไปที่ Graphics.DrawString หรือพวก Draw ต่างของ Document
มองว่าการพิมพ์งานก็คือ การ ใช้งาน Image/picture/Graphics หรือการเรารูปมายัดลงเครื่องปริ้นนั่นเองครับ
แรกผมไปมุ่งที่ Document เล่นเอางง แต่พอมองว่ามันมาจาก รูปภาพ ทุกอย่างที่ทำเป็นรูปได้ก็พิมได้ ก็เลยง่ายขึ้น ครับ
|
 |
 |
 |
 |
Date :
2017-04-01 12:43:39 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
บอกตรง ๆ ว่าผมอ่านแล้วไม่เข้าใจ
อ้างอิงจากคุณ TOR_CHEMISTRY น่ะครับ
ถ้าอยากพิมพ์หมายเลขในกระดาษ
และให้มันเพิ่มไปเรื่อย ๆ
ผมว่าทำใน Crystal Report ก็ได้มั้งครับ
ส่งค่าไป แล้วให้บวกกับเลขหน้าปัจจุบัน
ก็น่าจะทำได้
|
 |
 |
 |
 |
Date :
2017-04-01 15:50:57 |
By :
fonfire |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
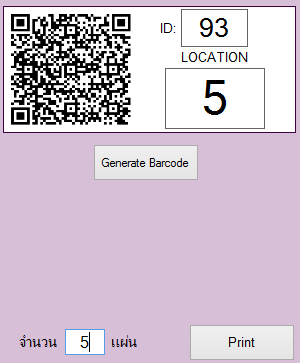
ตามภาพครับ
ID ตอนนี้เท่ากับ 93
1.เมื่อกด print ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 93 ให้เป็น 94 จำนวนแผ่นจาก 5 เหลือ 4
2.ถ้าจำนวนแผ่นเท่ากับ 4 เหลือ ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 93 ให้เป็น 94 จำนวนแผ่นจาก 5 เหลือ 4
3.เมื่อกด print ให้พิมพ์ QRcode ออกมาแล้ว จำนวนแผ่นจาก 3 เหลือ 2 ID เปลี่ยนเป็น 96
4.เมื่อกด print ให้พิมพ์ QRcode ออกมาแล้ว จำนวนแผ่นจาก 2 เหลือ 1 ID เปลี่ยนเป็น 97
5.เมื่อกด print ให้พิมพ์ QRcode ออกมาแล้ว จำนวนแผ่นจาก 1 เหลือ 0 ID เปลี่ยนเป็น 98
ต่อไปถ้ากด print อีกจะไม่สามารถพิมพ์ได้เพราะจำนวนแผ่น = 0
|
 |
 |
 |
 |
Date :
2017-04-01 16:41:05 |
By :
sakkapong |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แก้ไขข้างบนก่อนหน้านี้นะครับ
ตามภาพครับ
ID ตอนนี้เท่ากับ 93
1.เมื่อกด print ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 93 ให้เป็น 94 จำนวนแผ่นจาก 5 เหลือ 4
2.ถ้าจำนวนแผ่นเท่ากับ 4 ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 94 ให้เป็น 95 จำนวนแผ่นจาก 4 เหลือ 3
3.ถ้าจำนวนแผ่นเท่ากับ 3 ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 95 ให้เป็น 96 จำนวนแผ่นจาก 3 เหลือ 2
4.ถ้าจำนวนแผ่นเท่ากับ 2 ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 96 ให้เป็น 97 จำนวนแผ่นจาก 2 เหลือ 1
5.ถ้าจำนวนแผ่นเท่ากับ 1 ให้พิมพ์ QRcode ออกมาแล้ว ID จาก 97 ให้เป็น 98 จำนวนแผ่นจาก 1 เหลือ 0
ถ้าจำนวนแผ่นเท่ากับ 0 ให้หยุดพิมพ์ไม่ต้องทำอะไร
แบบนี้ครับ
ขอบคุณครับ
|
 |
 |
 |
 |
Date :
2017-04-01 16:45:46 |
By :
sakkapong |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมเคยด่าคนต่อหน้าและลับหลัง
ผมถามว่า : ตรูแอบด่า/ด่าตรงฯ ทำไมเอ็งถึงยังหัวเราะได้ว่ะ?
คนถูกด่า: ผมเป็นคนมองโลกในแง่ดี ทุกฯคำชม/คำด่า ผมถือว่าเป็นประสบการ์ณ
+++++ 55555
ผมอยากได้ลูกพี่ที่คิดได้แบบนี้จริงฯ ไม่ต้องมากขอแค่มีแค่คนเดียวบนโลกใบนี้
|
 |
 |
 |
 |
Date :
2017-04-01 17:32:45 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
โลกใบนี้มันกว้าง แต่ความรู้ด้านคอมพิวเตอร์(ในเมืองไทย) มันยังคงคำว่า "แคบ"
--- โอกาสที่เราจะเจอกันด้วยความบังเอิญมันก็เป็นไปได้
--- ไม่รู้ใครเป็นใคร จริงหรือโกหก มันก็ยังไม่ได้นิยามให้ตรงกัน
พึงระมัดระวัง
ปล. +55555
|
 |
 |
 |
 |
Date :
2017-04-01 17:43:52 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
555    ยังไม่เข้าใจขอเอากลับไปนอนคิดก่อน
|
 |
 |
 |
 |
Date :
2017-04-01 17:44:47 |
By :
sakkapong |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 03
|