|
 |
|
ถามหน่อยครับ import excel ไฟล์ .xlsx ได้แต่ไม่สามารถ import ไฟล์ .xls ได้ครับ ต้องแก้ยังไงครับ |
|
 |
|
|
 |
 |
|
โค้ด
Code (C#)
try
{
int x = 1;
FileInfo excel = new FileInfo((@"D:\Application\Billpayment\FileTest\COOP_180531_012.xls"));
using (var package = new ExcelPackage(excel))
{
var workbook = package.Workbook;
//*** Sheet 1
var worksheet = workbook.Worksheets.First();
string strConnString = "Server=xxxxxxxxxx;UID=sa;PASSWORD=xxxxxx;database=mybase";
var objConn = new SqlConnection(strConnString);
objConn.Open();
//*** Loop to Insert
int totalRows = worksheet.Dimension.End.Row;
for (int i = 10; i <= totalRows; i++)
{
MessageBox.Show(worksheet.Cells[i, 1].ToString());
string strSQL = "INSERT INTO tbl_billpayment_confirm (billpayment_date,order_no,ref_no_1,ref_no_2,billpayment_name,amount,status_flag,user_id) "
+ " VALUES ("
+ "GETDATE()" + ", "
+ " '" + x + "', "
+ " '" + worksheet.Cells[i, 1].Text.ToString() + "', "
+ " '" + worksheet.Cells[i, 2].Text.ToString() + "', "
+ " '" + worksheet.Cells[i, 3].Text.ToString() + "', "
+ " '" + worksheet.Cells[i, 4].Text.ToString() + "', "
+ " '" + null + "', "
+ " '" + null + "' "
+ ")";
var objCmd = new SqlCommand(strSQL, objConn);
objCmd.ExecuteNonQuery();
}
//objConn.Close();
MessageBox.Show("เสร็จ");
x++;
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
error แจ้งตอน impor
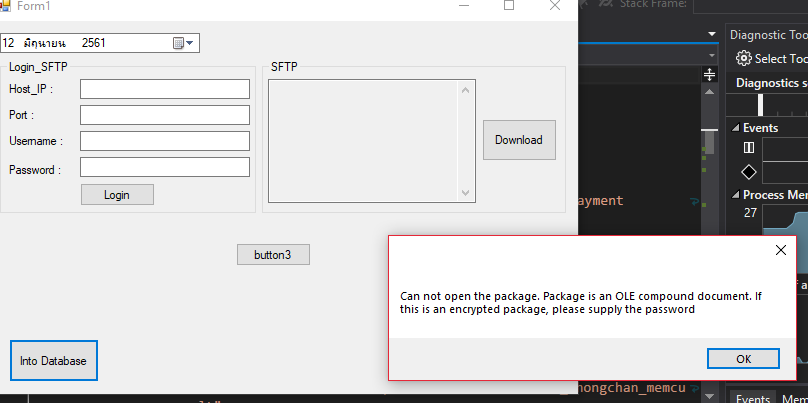
แต่ถ้าเป็นไฟล์ .xlsx มัน import เข้าbase ได้ปกติ
Tag : .NET, Win (Windows App), C#

|
|
 |
 |
 |
 |
Date :
2018-06-12 10:50:21 |
By :
darkgolfman0 |
View :
1670 |
Reply :
9 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอนนี้ แก้ได้ละครับ ต้องแปลงไฟล์ xls เป็น xlsx โดยจะต้องติดตั้ง Spire.XLS dll ที่อยู่ใน NuGet
แล้วใช้โค้ดแปลงไฟล์
Code (C#)
Workbook workbooks = new Workbook();
workbooks.LoadFromFile(@"file.xls");
workbooks.SaveToFile(@"file.xlsx", ExcelVersion.Version2013);
|
 |
 |
 |
 |
Date :
2018-06-12 17:09:22 |
By :
darkgolfman0 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|

|
 |
 |
 |
 |
Date :
2018-06-13 11:44:56 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 00
|