|
 |
|
C# จะเขียน thread แบบนี้ ในรูปแบบ lambda ได้ยังไง ครับ |
|
 |
|
|
 |
 |
|
Code (C#)
/* void ShowThreadInformation(Object state)
{
Object obj = new Object();
lock (obj)
{
var th = Thread.CurrentThread;
Console.WriteLine("Managed thread #{0}: ", th.ManagedThreadId);
Console.WriteLine(" Background thread: {0}", th.IsBackground);
Console.WriteLine(" Thread pool thread: {0}", th.IsThreadPoolThread);
Console.WriteLine(" Priority: {0}", th.Priority);
Console.WriteLine(" Culture: {0}", th.CurrentCulture.Name);
Console.WriteLine(" UI culture: {0}", th.CurrentUICulture.Name);
Console.WriteLine();
}
}*/
private void ThreadClass_4()
{
Action<object> ShowThreadInformation =(state) =>
{
Object obj = new Object();
lock (obj)
{
var th = Thread.CurrentThread;
Console.WriteLine("Managed thread #{0}: ", th.ManagedThreadId);
Console.WriteLine(" Background thread: {0}", th.IsBackground);
Console.WriteLine(" Thread pool thread: {0}", th.IsThreadPoolThread);
Console.WriteLine(" Priority: {0}", th.Priority);
Console.WriteLine(" Culture: {0}", th.CurrentCulture.Name);
Console.WriteLine(" UI culture: {0}", th.CurrentUICulture.Name);
Console.WriteLine();
}
};
ThreadPool.QueueUserWorkItem(ShowThreadInformation);
var th1 = new Thread(ShowThreadInformation);
th1.Start();
var th2 = new Thread(ShowThreadInformation);
th2.IsBackground = true;
th2.Start();
Thread.Sleep(500);
ShowThreadInformation(null);
}
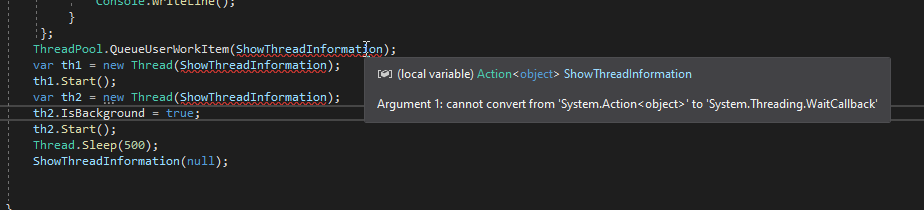

ประเด็นคือ อยากลองเขียนให้เป็น แบบ action หรือ func ครับ
ในกรณีที่ไม่มี parameter จะใช้ได้ครับ
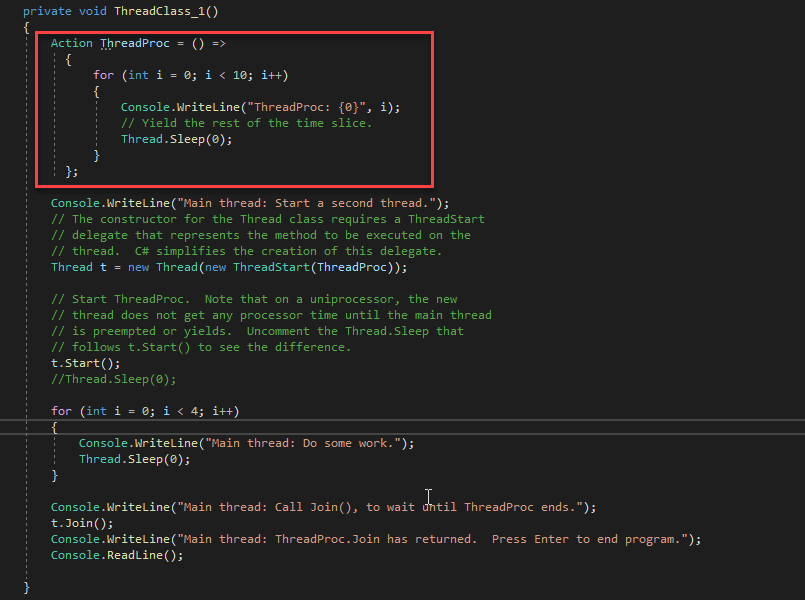
แต่พอเพิ่ม parameter กลับ Error เลยอยากรู้ว่าต้องเขียนยังไง ครับ
Tag : .NET, Web (ASP.NET), Win (Windows App), C#, VS 2017 (.NET 4.x)

|
ประวัติการแก้ไข 2020-04-12 23:00:49
|
 |
 |
 |
 |
Date :
2020-04-12 16:24:50 |
By :
lamaka.tor |
View :
1474 |
Reply :
6 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Action เป็น Delegate ที่ไม่คืนค่า และสามารถ
กำหนด Type ของ Parameter ได้อย่างอิสระ
ในขณะเดียวกัน Action ยังเป็น Type เองด้วย
การเริ่ม Thread โดยใส่ parameter จึง
ต้องใช้ Delegate ตาม Constructors
ที่สนับสนุน Method Signature ของ Thread Class
Thread Class Constructors:
Thread(ParameterizedThreadStart)
Thread(ParameterizedThreadStart, Int32)
Thread(ThreadStart)
Thread(ThreadStart, Int32)
Thread Class มี delegate argument 2 ตัว คือ ThreadStart กับ ParameterizedThreadStart
ThreadStart สำหรับ signature ที่ไม่มี argument
[System.Runtime.InteropServices.ComVisible(true)]
public delegate void ThreadStart();
ส่วน ParameterizedThreadStart แบบมี 1 argument ซึ่งก็คือ object
[System.Runtime.InteropServices.ComVisible(false)]
public delegate void ParameterizedThreadStart(object obj);
ในทำนองเดียวกันการใช้เมธอด QueueUserWorkItem ของ ThreadPool
ก็ต้องใช้ delegate ที่มี type ตาม Overloads ของมัน
ThreadPool.QueueUserWorkItem Method Overloads:
QueueUserWorkItem(WaitCallback)
QueueUserWorkItem(WaitCallback, Object)
argument ของ QueueUserWorkItem ที่เป็น delegate มีแค่ WaitCallback
ซึ่งมี method signature เป็นแบบ 1 argument ที่มี type เป็น object
[System.Runtime.InteropServices.ComVisible(true)]
public delegate void WaitCallback(object state);
ตัวอย่างการใช้งาน
Code (C#)
using System;
using System.Threading;
namespace ProgramConsole {
public class Program {
public static void Main(string[] args) {
ThreadClass_4();
}
private static void ThreadClass_4() {
WaitCallback ShowThreadInformationW = (state) => MyMethod("Test " + state);
ParameterizedThreadStart ShowThreadInformation = (state) => MyMethod(state);
ThreadPool.QueueUserWorkItem(ShowThreadInformationW, "ThreadPoolState");
var th1 = new Thread(ShowThreadInformation);
th1.Start("th1");
var th2 = new Thread(ShowThreadInformation);
th2.IsBackground = true;
th2.Start("th2");
Thread.Sleep(500);
ShowThreadInformation(null);
}
private static void MyMethod (Object o) {
Object obj = new Object();
lock(obj) {
var th = Thread.CurrentThread;
Console.WriteLine("Managed thread #{0}: ", th.ManagedThreadId);
Console.WriteLine(" Background thread: {0}", th.IsBackground);
Console.WriteLine(" Thread pool thread: {0}", th.IsThreadPoolThread);
Console.WriteLine(" Priority: {0}", th.Priority);
Console.WriteLine(" Culture: {0}", th.CurrentCulture.Name);
Console.WriteLine(" UI culture: {0}", th.CurrentUICulture.Name);
Object ol = (o == null) ? "Parameter is null" : o;
Console.WriteLine(ol);
Console.WriteLine();
}
}
}
}
หรือ แนวขี้เกียจ โดยใช้ wrapper ของ delegate จำเป็น (Invoke เทียม)
(ParameterizedThreadStart ของ Thread Class กับ WaitCallback ของ ThreadPool.QueueUserWorkItem Method)
ด้วยคำสั่ง : {ชื่อ Action delegate}.Invoke
Code (C#)
using System;
using System.Threading;
namespace ProgramConsole {
public class Program {
public static void Main(string[] args) {
ThreadClass_4();
}
private static void ThreadClass_4() {
Action < object > ShowThreadInformation = (state) => {
Object obj = new Object();
lock(obj) {
var th = Thread.CurrentThread;
Console.WriteLine("Managed thread #{0}: ", th.ManagedThreadId);
Console.WriteLine(" Background thread: {0}", th.IsBackground);
Console.WriteLine(" Thread pool thread: {0}", th.IsThreadPoolThread);
Console.WriteLine(" Priority: {0}", th.Priority);
Console.WriteLine(" Culture: {0}", th.CurrentCulture.Name);
Console.WriteLine(" UI culture: {0}", th.CurrentUICulture.Name);
Console.WriteLine();
}
};
ThreadPool.QueueUserWorkItem(ShowThreadInformation.Invoke);
var th1 = new Thread(ShowThreadInformation.Invoke);
th1.Start();
var th2 = new Thread(ShowThreadInformation.Invoke);
th2.IsBackground = true;
th2.Start();
Thread.Sleep(500);
ShowThreadInformation(null);
}
}
}
|
ประวัติการแก้ไข 2020-04-14 00:35:03 2020-04-14 00:38:12 2020-04-14 00:42:13
 |
 |
 |
 |
Date :
2020-04-14 00:31:06 |
By :
PhrayaDev |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 05
|