 |
|
C# winApp ต้องการให้จุดมันตรงกัน ใน เลขทศนิยม ครับ |
|
 |
|
|
 |
 |
|
มาอีกแล้วครับ วันนี้ ผมได้ขึ้น คณิต ม. 1 เรื่อง การบวก การลบ ทศนิยม แล้วนะครับ
คราวนี้ อยาก ให้ DrawString แบบ ใน จุด อยู่ตรงกัน ครับ
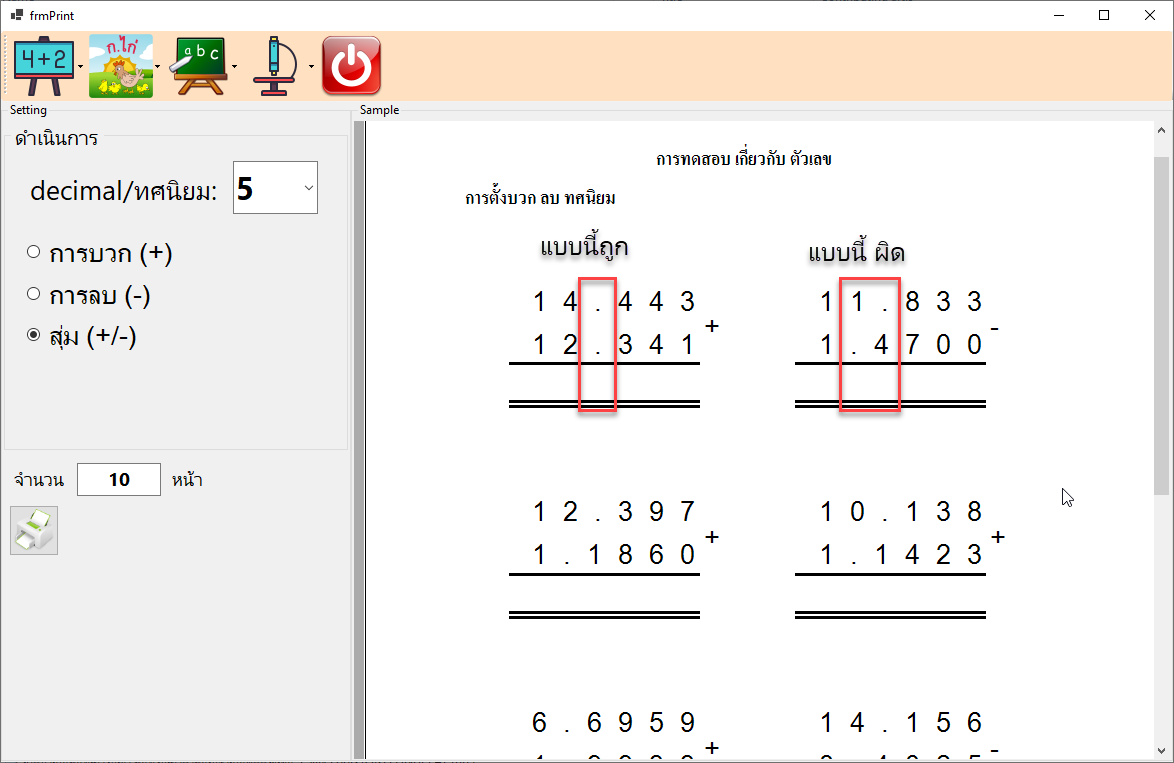
โค้ด
https://github.com/mongkonP/KidsLearning/blob/master/KidsLearning.Print/ptnMth/m02OP/op005PlusMinus_Num_03factoring.cs
https://github.com/mongkonP/KidsLearning/blob/master/KidsLearning.Classed/Exten/ExtGraphics_Maths_Operation.cs
Code (C#)
public static void DrawNumPositive(this Graphics e, Font fontDetail, double min, double max, int x, int y, string opr, int CountNum = 6)
{
var num = new Classed.Exten.RandomNumber(min, max);
string _a = TextStringExtension.SpacedString(num.MinValue.ToString());
string _b = TextStringExtension.SpacedString(num.MaxValue.ToString());
//System.Windows.Forms.MessageBox.Show(num.MinValue + "\n" + num.MaxValue + "\n" + _a + "\n" + _b);
int w = Convert.ToInt32(e.MeasureString("0 ", fontDetail).Width);
int h = Convert.ToInt32(e.MeasureString("0 ", fontDetail).Height);
//https://docs.microsoft.com/en-us/dotnet/desktop/winforms/advanced/how-to-align-drawn-text?view=netframeworkdesktop-4.8
StringFormat format = new StringFormat(StringFormatFlags.DirectionRightToLeft);
// https://stackoverflow.com/questions/11451001/why-isnt-my-text-right-aligned-when-i-custom-draw-my-strings
//https://docs.microsoft.com/en-us/dotnet/desktop/winforms/advanced/how-to-align-drawn-text?view=netframeworkdesktop-4.8&redirectedfrom=MSDN
StringFormat stringFormat = new StringFormat() { Alignment = StringAlignment.Far };
e.DrawString(_b, fontDetail, new SolidBrush(Color.Black), new Rectangle(x, y, w * CountNum, h), stringFormat);
e.DrawString(_a, fontDetail, new SolidBrush(Color.Black), new Rectangle(x, y + h + 10, w * CountNum, h), stringFormat);
e.DrawLine(new Pen(Color.Black, 3), x, y + 2 * h + 10, x + (CountNum) * w, y + 2 * h + 10);
e.DrawString(opr, fontDetail, new SolidBrush(Color.Black), x + (CountNum) * w, y + 10 + h / 2);
e.DrawLine(new Pen(Color.Black, 3), x, y + h * 3 + 15, x + (CountNum) * w, y + h * 3 + 15);
e.DrawLine(new Pen(Color.Black, 3), x, y + h * 3 + 20, x + (CountNum) * w, y + h * 3 + 20);
}
Tag : .NET, Win (Windows App), C#
|
|
 |
 |
 |
 |
Date :
2022-09-29 16:43:00 |
By :
lamaka.tor |
View :
741 |
Reply :
7 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ง่ายสุดคือเคาะ space ครับ
ถ้าไม่รับ แปลงจำนวนเต็มให้มีจำนวนเท่ากัน
ด้วยวิธีการ string interpolation เช่น
101.xxx ทำให้เป็น 4 หลักได้ 0101.xxx
แต่แทนที่จะใส่ 0 ก็ใส่เป็น whitespace character แทน
https://en.wikipedia.org/wiki/Whitespace_character
อีกวิธีคำนวณหาพิกัด x ของ . แล้วเลื่อนตัวเลขทั้งดุ้น ไปทางซ้ายหรือขวาตามจำนวนที่ขาด
|
 |
 |
 |
 |
Date :
2022-09-29 17:04:17 |
By :
009 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
หรือลอง
Code (C#)
using System;
namespace x009
{
class Program
{
static void Main(string[] args)
{
double i = 1.42;
double j = 123.56;
Console.WriteLine(i.ToString().PadLeft(6, 'x'));
Console.WriteLine(j.ToString().PadLeft(6, 'x'));
}
}
}
ตอน draw ก็เปลี่ยนสีอักษรที่ pad เป็นสีกระดาษ ตอนปริ้นต์ก็จะไม่เห็น
PadLeft ใช้กับจำนวนลบไม่ได้
|
 |
 |
 |
 |
Date :
2022-09-29 17:47:12 |
By :
009 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
double a = GetRandomNumber(min, max, random.Next(1, 5));
double b = GetRandomNumber(min, max, random.Next(1, 5));
int c = Math.Max((a.ToString().Contains(".")) ? a.ToString().Split('.')[1].Length : 0, (b.ToString().Contains(".")) ? b.ToString().Split('.')[1].Length : 0);
string _a = a.ToString().PadLeft(c, 'x');
string _b = b.ToString().PadLeft(c, 'x');
ได้ออกมาแบบนี้ ครับ
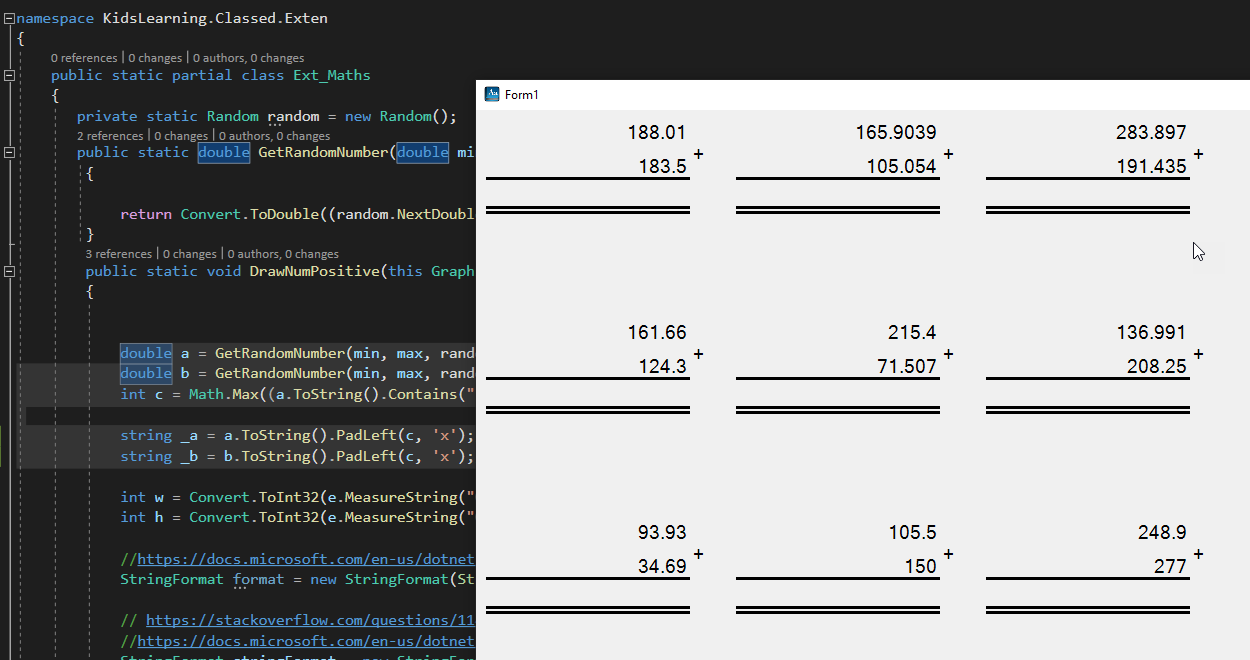
ไม่แน่ใจว่าผมทำผิดในส่วนไหน ครับ
|
 |
 |
 |
 |
Date :
2022-09-29 18:50:09 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
น่าจะได้แล้วมั้งครับ(รึปล่าว ทอสอบแค่ไม่กี่ที)
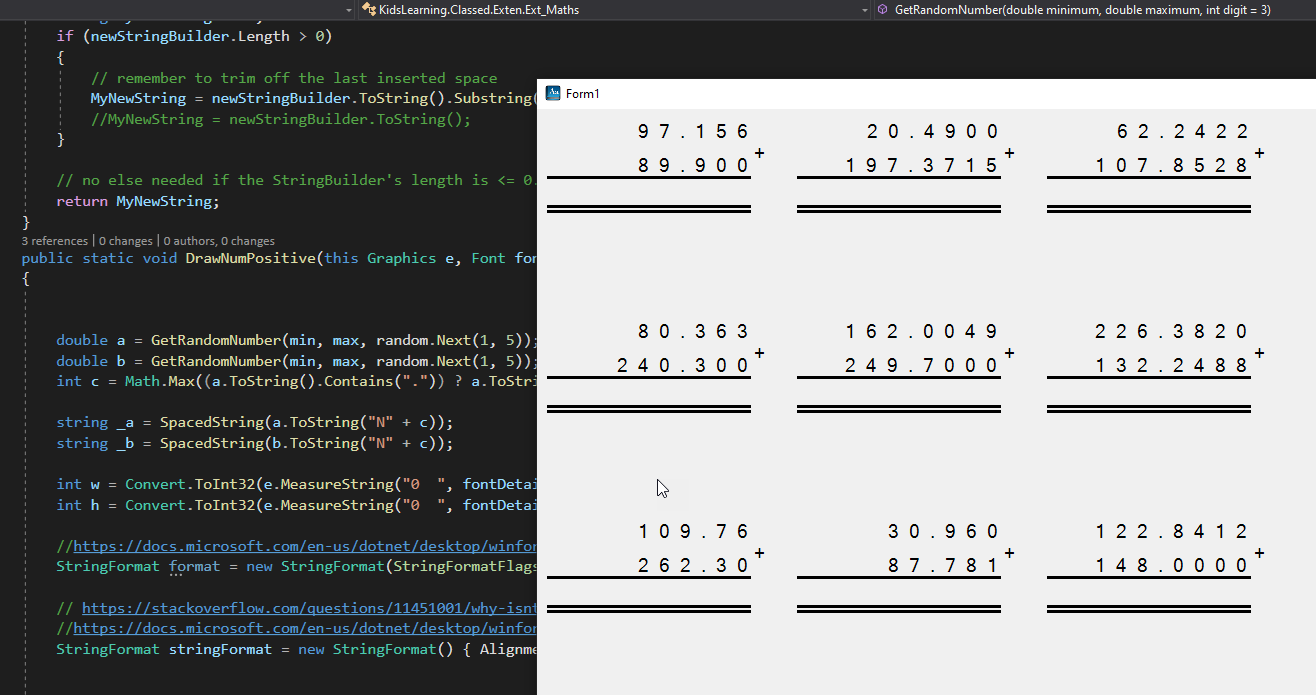
Code (C#)
public static string SpacedString(string myOldString)
{
//ref
//https://stackoverflow.com/questions/2969143/c-sharp-drawstring-letter-spacing
System.Text.StringBuilder newStringBuilder = new System.Text.StringBuilder("");
foreach (char c in myOldString.ToCharArray())
{
newStringBuilder.Append(c.ToString() + " ");
}
string MyNewString = "";
if (newStringBuilder.Length > 0)
{
// remember to trim off the last inserted space
MyNewString = newStringBuilder.ToString().Substring(0, newStringBuilder.Length - 1).Trim();
//MyNewString = newStringBuilder.ToString();
}
// no else needed if the StringBuilder's length is <= 0... The resultant string would just be "", which is what it was intitialized to when declared.
return MyNewString;
}
public static void DrawNumPositive(this Graphics e, Font fontDetail, double min, double max, int x, int y, string opr, int CountNum = 6)
{
double a = GetRandomNumber(min, max, random.Next(1, 5));
double b = GetRandomNumber(min, max, random.Next(1, 5));
int c = Math.Max((a.ToString().Contains(".")) ? a.ToString().Split('.')[1].Length : 0, (b.ToString().Contains(".")) ? b.ToString().Split('.')[1].Length : 0);
string _a = SpacedString(a.ToString("N" + c));
string _b = SpacedString(b.ToString("N" + c));
int w = Convert.ToInt32(e.MeasureString("0 ", fontDetail).Width);
int h = Convert.ToInt32(e.MeasureString("0 ", fontDetail).Height);
//https://docs.microsoft.com/en-us/dotnet/desktop/winforms/advanced/how-to-align-drawn-text?view=netframeworkdesktop-4.8
StringFormat format = new StringFormat(StringFormatFlags.DirectionRightToLeft);
// https://stackoverflow.com/questions/11451001/why-isnt-my-text-right-aligned-when-i-custom-draw-my-strings
//https://docs.microsoft.com/en-us/dotnet/desktop/winforms/advanced/how-to-align-drawn-text?view=netframeworkdesktop-4.8&redirectedfrom=MSDN
StringFormat stringFormat = new StringFormat() { Alignment = StringAlignment.Far };
e.DrawString(_b, fontDetail, new SolidBrush(Color.Black), new Rectangle(x, y, w * CountNum, h), stringFormat);
e.DrawString(_a, fontDetail, new SolidBrush(Color.Black), new Rectangle(x, y + h + 10, w * CountNum, h), stringFormat);
e.DrawLine(new Pen(Color.Black, 3), x, y + 2 * h + 10, x + (CountNum) * w, y + 2 * h + 10);
e.DrawString(opr, fontDetail, new SolidBrush(Color.Black), x + (CountNum) * w, y + 10 + h / 2);
e.DrawLine(new Pen(Color.Black, 3), x, y + h * 3 + 15, x + (CountNum) * w, y + h * 3 + 15);
e.DrawLine(new Pen(Color.Black, 3), x, y + h * 3 + 20, x + (CountNum) * w, y + h * 3 + 20);
}
ก็ผ่านไปอีก เรื่องหนึ่งครับ เรื่องหน้าค่อยว่ากัน
อีกกี่ปีน๊ จะจบ ม.6
  
|
 |
 |
 |
 |
Date :
2022-09-29 19:04:08 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ค่อยๆ ทำไปครับ ถ้าไม่ได้ส่งเข้าประกวด ทำแบบเบสิคไปก็ได้ 
|
 |
 |
 |
 |
Date :
2022-09-29 19:18:12 |
By :
009 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|