|
ASP.NET สร้าง WebBoard (เว็บบอร์ด) กระทู้หรือกระดานถาม-ตอบ (by VB.NET , C#) |
|
|
ASP.NET กับการสร้าง Webboard (เว็บบอร์ด) กระทู้หรือกระดานถาม-ตอบ บทความ asp.net กับการสร้างระบบ webboard หรือกระดานถามตอบ สำหรับการตั้งกระทู้ และการแสดงความคิดเห็นในกระทู้ สำหรับตัวอย่างนี้ไม่มีอะไรซับซ้อน โดยใน Webboard นี้จะประกอบด้วย 2 ตารางคือ webboard (เก็บคำถาม) และ reply (เก็บรายละเอียดผู้ตอบหรือผู้แสดงความคิดเห็น) โดยในตัวอย่างได้ในกระทู้ได้เก็บจำนวนผู้อ่าน และแสดงความคิดเห็นของแต่ล่ะกระทู้ด้วย มีตัวอย่างให้ดูหรือดาวน์โหลดทั้งภาษา VB.NET และ C#
Screenshot
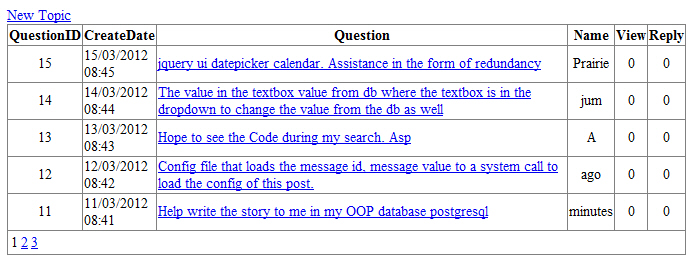
คุณสมบัติและความสามารถของตัวอย่างนี้
- แสดงกระทู้ จำนวนคนอ่าน / จำนวนคนตอบ
- สามารถแสดงความคิดเห็น
- แบบฟอร์มสำหรับตั้งกระทู้ใหม่
ตารางประกอบด้วย 2 ตารางคือ
- webboard
- reply
โครงสร้างตาราง webboard เก็บรายละเอียดคำถาม
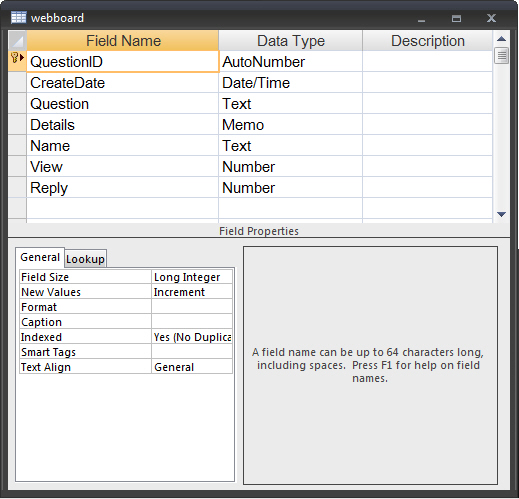
โครงสร้างตาราง reply เก็บรายละเอียดการแสดงความคดิเห็น
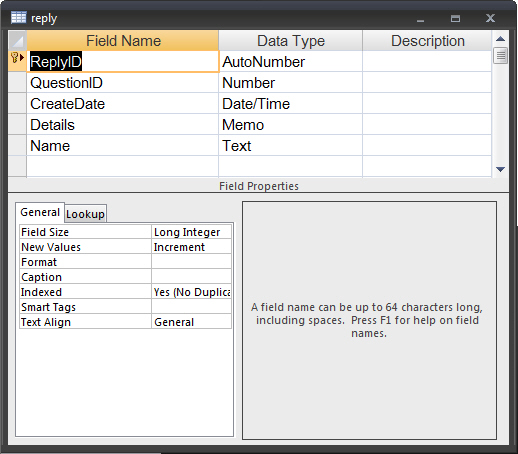
เริ่มต้นสร้าง Project
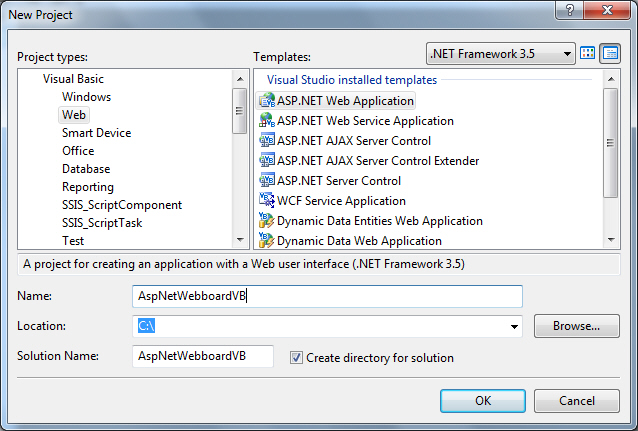
เลือกเป็น ASP.NET Web Application สำหรับภาษาก็แล้วแต่ถนัด อาจจะเป็น VB.NET หรือ C#
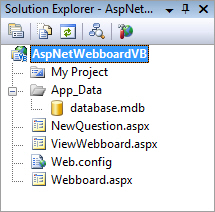
สำหรับฐานข้อมูลใช้ Microsoft Access ชื่อฐานข้อมูลว่า mydatabase.mdb จัดเก็บไว้ในโฟเดอร์ App_Data

สำหรับไฟล์จะประกอบด้วย
- Webboard.aspx (แสดงคำถาม)
- ViewWebboard.aspx (แสดงรายละเอียดผู้แสดงความคิดเห็น)
- NewQuestion.aspx (ไว้สำหรับตั้งกระทู้ใหม่)
(สามารถดาวน์โหลดได้จากข้างล่าง)
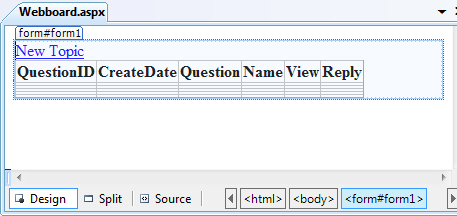
Form สำหรับ Webboard.aspx
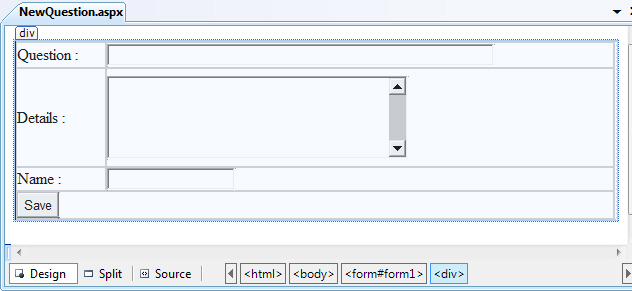
Form สำหรับ ViewWebboard.aspx
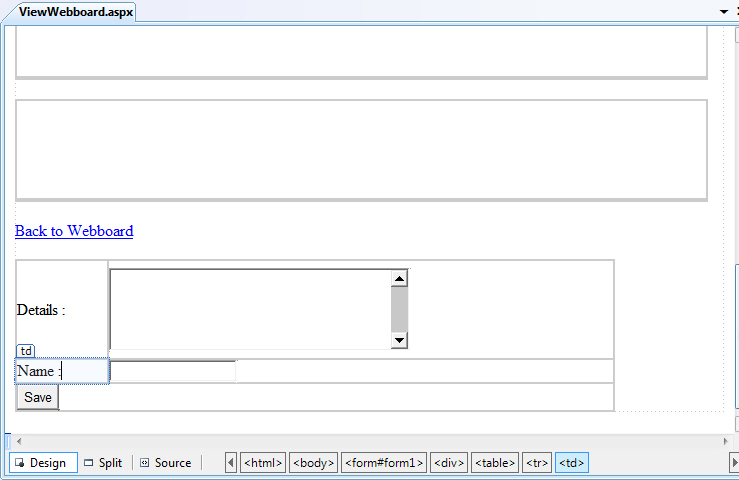
Form หน้าจอ ViewWebboard.aspx
Code สำหรับภาษา VB.NET
Webboard.aspx.vb
Imports System.Data
Imports System.Data.OleDb
Partial Public Class Webboard
Inherits System.Web.UI.Page
Protected objConn As OleDbConnection
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim strConnString As String
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|DataDirectory|\database.mdb"
objConn = New OleDbConnection(strConnString)
objConn.Open()
If Not Page.IsPostBack() Then
ShowWebboard()
End If
End Sub
Protected Sub ShowWebboard()
Dim dtAdapter As New OleDbDataAdapter
Dim objCmd As New OleDbCommand
Dim ds As New DataSet
Dim strSQL As New StringBuilder
strSQL.Append(" SELECT * FROM webboard ")
strSQL.Append(" ORDER BY QuestionID DESC ")
With objCmd
.Connection = objConn
.CommandText = strSQL.ToString
.CommandType = CommandType.Text
End With
dtAdapter.SelectCommand = objCmd
dtAdapter.Fill(ds)
myGridView.AllowPaging = True
myGridView.PageSize = 5
myGridView.DataSource = ds.Tables(0)
myGridView.DataBind()
End Sub
Protected Sub myGridView_PageIndexChanging(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewPageEventArgs) Handles myGridView.PageIndexChanging
myGridView.PageIndex = e.NewPageIndex
ShowWebboard()
End Sub
Protected Sub myGridView_RowDataBound(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewRowEventArgs) Handles myGridView.RowDataBound
If e.Row.RowType = DataControlRowType.DataRow Then
'*** QuestionID ***'
Dim lblQuestionID As Label = DirectCast(e.Row.FindControl("lblQuestionID"), Label)
If Not IsNothing(lblQuestionID) Then
lblQuestionID.Text = e.Row.DataItem("QuestionID")
End If
'*** CreateDate ***'
Dim lblCreateDate As Label = DirectCast(e.Row.FindControl("lblCreateDate"), Label)
If Not IsNothing(lblCreateDate) Then
lblCreateDate.Text = CDate(e.Row.DataItem("CreateDate")).ToString("dd/MM/yyyy HH:mm")
End If
'*** Question ***'
Dim hplQuestion As HyperLink = DirectCast(e.Row.FindControl("hplQuestion"), HyperLink)
If Not IsNothing(hplQuestion) Then
hplQuestion.Text = e.Row.DataItem("Question")
hplQuestion.NavigateUrl = "ViewWebboard.aspx?QuestionID=" & e.Row.DataItem("QuestionID")
End If
'*** Name ***'
Dim lblName As Label = DirectCast(e.Row.FindControl("lblName"), Label)
If Not IsNothing(lblName) Then
lblName.Text = e.Row.DataItem("Name")
End If
'*** View ***'
Dim lblView As Label = DirectCast(e.Row.FindControl("lblView"), Label)
If Not IsNothing(lblView) Then
lblView.Text = e.Row.DataItem("View")
End If
'*** Reply ***'
Dim lblReply As Label = DirectCast(e.Row.FindControl("lblReply"), Label)
If Not IsNothing(lblReply) Then
lblReply.Text = e.Row.DataItem("Reply")
End If
End If
End Sub
Protected Sub Page_Unload(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Unload
objConn.Close()
objConn = Nothing
End Sub
End Class
NewQuestion.aspx.vb
Imports System.Data
Imports System.Data.OleDb
Partial Public Class NewQuestion
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
End Sub
Protected Sub btnSave_Click(ByVal sender As Object, ByVal e As EventArgs) Handles btnSave.Click
Dim objConn As OleDbConnection
Dim strSQL As New StringBuilder
Dim objCmd As OleDbCommand
Dim strConnString As String
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|DataDirectory|\database.mdb"
objConn = New OleDbConnection(strConnString)
objConn.Open()
'*** Insert Reply ***'
strSQL.Append(" INSERT INTO webboard (CreateDate,Question,Details,Name,[View],Reply) ")
strSQL.Append(" VALUES ")
strSQL.Append(" (@sCreateDate,@sQuestion,@sDetails,@sName,@sView,@sReply) ")
objCmd = New OleDbCommand(strSQL.ToString(), objConn)
objCmd.Parameters.Add("@sCreateDate", OleDbType.Date).Value = Now()
objCmd.Parameters.Add("@sQuestion", OleDbType.VarChar).Value = Me.txtQuestion.Text
objCmd.Parameters.Add("@sDetails", OleDbType.VarChar).Value = Me.txtDetails.Text
objCmd.Parameters.Add("@sName", OleDbType.VarChar).Value = Me.txtName.Text
objCmd.Parameters.Add("@sView", OleDbType.Integer).Value = 0
objCmd.Parameters.Add("@sReply", OleDbType.Integer).Value = 0
objCmd.ExecuteNonQuery()
objConn.Close()
objConn = Nothing
Response.Redirect("Webboard.aspx")
End Sub
End Class
ViewWebboard.aspx.vb
Imports System.Data
Imports System.Data.OleDb
Partial Public Class ViewWebboard
Inherits System.Web.UI.Page
Protected objConn As OleDbConnection
Protected strQuestionID As String = HttpContext.Current.Request.QueryString("QuestionID")
Protected strReplyNo As Integer = 1
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim strConnString As String
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|DataDirectory|\database.mdb"
objConn = New OleDbConnection(strConnString)
objConn.Open()
ShowQuestion()
ShowReply()
End Sub
Protected Sub ShowQuestion()
Dim dtAdapter As OleDbDataAdapter
Dim objCmd As OleDbCommand
Dim dt As New DataTable
Dim strSQL As New StringBuilder
strSQL.Append("SELECT * FROM webboard ")
strSQL.Append(" WHERE QuestionID = " & strQuestionID & " ")
dtAdapter = New OleDbDataAdapter(strSQL.ToString, objConn)
dtAdapter.Fill(dt)
If dt.Rows.Count > 0 Then
Me.lblQuestion.Text = dt.Rows(0)("Question")
Me.lblDetails.Text = dt.Rows(0)("Details")
Me.lblName.Text = "<b>Name : </b>" & dt.Rows(0)("Name")
Me.lblCreateDate.Text = "<b>CreateDate : </b>" & CDate(dt.Rows(0)("CreateDate")).ToString("dd/MM/yyyy HH:mm")
Me.lblReply.Text = "<b>Reply : </b>" & dt.Rows(0)("Reply")
Me.lblView.Text = "<b>View : </b>" & dt.Rows(0)("View")
End If
'*** Update Number of View ***'
strSQL.Remove(0, strSQL.Length)
strSQL.Append(" UPDATE webboard SET [View] = [View] + 1 ")
strSQL.Append(" WHERE QuestionID = @sQuestionID ")
objCmd = New OleDbCommand(strSQL.ToString(), objConn)
objCmd.Parameters.Add("@sQuestionID", OleDbType.VarChar).Value = strQuestionID.ToString()
objCmd.ExecuteNonQuery()
End Sub
Protected Sub ShowReply()
Dim strSQL As New StringBuilder
Dim objCmd As OleDbCommand
strSQL.Append(" SELECT * FROM reply ")
strSQL.Append(" WHERE QuestionID = " & strQuestionID & " ")
Dim dtReader As OleDbDataReader
objCmd = New OleDbCommand(strSQL.ToString, objConn)
dtReader = objCmd.ExecuteReader()
'*** BindData to Repeater ***'
myRepeater.DataSource = dtReader
myRepeater.DataBind()
dtReader.Close()
dtReader = Nothing
End Sub
Protected Sub ViewWebboard_Unload(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Unload
objConn.Close()
objConn = Nothing
End Sub
Protected Sub btnSave_Click(ByVal sender As Object, ByVal e As EventArgs) Handles btnSave.Click
Dim strSQL As New StringBuilder
Dim objCmd As OleDbCommand
'*** Insert Reply ***'
strSQL.Append(" INSERT INTO reply (QuestionID,CreateDate,Details,Name) ")
strSQL.Append(" VALUES ")
strSQL.Append(" (@sQuestionID,@sCreateDate,@sDetails,@sName) ")
objCmd = New OleDbCommand(strSQL.ToString(), objConn)
objCmd.Parameters.Add("@sQuestionID", OleDbType.VarChar).Value = strQuestionID.ToString()
objCmd.Parameters.Add("@sCreateDate", OleDbType.Date).Value = Now()
objCmd.Parameters.Add("@sDetails", OleDbType.VarChar).Value = Me.txtDetails.Text
objCmd.Parameters.Add("@sName", OleDbType.VarChar).Value = Me.txtName.Text
objCmd.ExecuteNonQuery()
'*** Update Number of Reply ***'
strSQL.Remove(0, strSQL.Length)
strSQL.Append(" UPDATE webboard SET Reply = Reply + 1 ")
strSQL.Append(" WHERE QuestionID = @sQuestionID ")
objCmd = New OleDbCommand(strSQL.ToString(), objConn)
objCmd.Parameters.Add("@sQuestionID", OleDbType.VarChar).Value = strQuestionID.ToString()
objCmd.ExecuteNonQuery()
Response.Redirect("ViewWebboard.aspx?QuestionID=" & strQuestionID)
End Sub
Protected Sub myRepeater_ItemDataBound(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.RepeaterItemEventArgs) Handles myRepeater.ItemDataBound
If e.Item.ItemType = ListItemType.Item Or e.Item.ItemType = ListItemType.AlternatingItem Then
'*** No ***'
Dim lblReplyNo As Label = DirectCast(e.Item.FindControl("lblReplyNo"), Label)
If Not IsNothing(lblReplyNo) Then
lblReplyNo.Text = "No : " & strReplyNo
strReplyNo = strReplyNo + 1
End If
'*** Details ***'
Dim lblReplyDetails As Label = DirectCast(e.Item.FindControl("lblReplyDetails"), Label)
If Not IsNothing(lblReplyDetails) Then
lblReplyDetails.Text = e.Item.DataItem("Details")
End If
'*** Name ***'
Dim lblReplyName As Label = DirectCast(e.Item.FindControl("lblReplyName"), Label)
If Not IsNothing(lblReplyName) Then
lblReplyName.Text = e.Item.DataItem("Name")
End If
'*** CreateDate ***'
Dim lblReplyCreateDate As Label = DirectCast(e.Item.FindControl("lblReplyCreateDate"), Label)
If Not IsNothing(lblReplyCreateDate) Then
lblReplyCreateDate.Text = CDate(e.Item.DataItem("CreateDate")).ToString("dd/MM/yyyy HH:mm")
End If
End If
End Sub
End Class
Code สำหรับภาษา C#
Webboard.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Diagnostics;
using System.Data.OleDb;
using System.Text;
namespace AspNetWebboardCS
{
public partial class Webboard : System.Web.UI.Page
{
protected OleDbConnection objConn;
protected void Page_Load(object sender, EventArgs e)
{
string strConnString = null;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|DataDirectory|\\database.mdb";
objConn = new OleDbConnection(strConnString);
objConn.Open();
if (!Page.IsPostBack)
{
ShowWebboard();
}
}
protected void ShowWebboard()
{
OleDbDataAdapter dtAdapter = new OleDbDataAdapter();
OleDbCommand objCmd = new OleDbCommand();
DataSet ds = new DataSet();
StringBuilder strSQL = new StringBuilder();
strSQL.Append(" SELECT * FROM webboard ");
strSQL.Append(" ORDER BY QuestionID DESC ");
var _with1 = objCmd;
_with1.Connection = objConn;
_with1.CommandText = strSQL.ToString();
_with1.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
myGridView.AllowPaging = true;
myGridView.PageSize = 5;
myGridView.DataSource = ds.Tables[0];
myGridView.DataBind();
}
protected void myGridView_PageIndexChanging(object sender, GridViewPageEventArgs e)
{
myGridView.PageIndex = e.NewPageIndex;
ShowWebboard();
}
protected void myGridView_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
//*** QuestionID ***'
Label lblQuestionID = (Label)e.Row.FindControl("lblQuestionID");
if ((lblQuestionID != null))
{
lblQuestionID.Text = DataBinder.Eval(e.Row.DataItem, "QuestionID").ToString();
}
//*** CreateDate ***'
Label lblCreateDate = (Label)e.Row.FindControl("lblCreateDate");
if ((lblCreateDate != null))
{
lblCreateDate.Text = Convert.ToDateTime(DataBinder.Eval(e.Row.DataItem, "CreateDate")).ToString("dd/MM/yyyy HH:mm");
}
//*** Question ***'
HyperLink hplQuestion = (HyperLink)e.Row.FindControl("hplQuestion");
if ((hplQuestion != null))
{
hplQuestion.Text = (string)DataBinder.Eval(e.Row.DataItem, "Question");
hplQuestion.NavigateUrl = "ViewWebboard.aspx?QuestionID=" + DataBinder.Eval(e.Row.DataItem, "QuestionID").ToString();
}
//*** Name ***'
Label lblName = (Label)e.Row.FindControl("lblName");
if ((lblName != null))
{
lblName.Text = (string)DataBinder.Eval(e.Row.DataItem, "Name");
}
//*** View ***'
Label lblView = (Label)e.Row.FindControl("lblView");
if ((lblView != null))
{
lblView.Text = DataBinder.Eval(e.Row.DataItem, "View").ToString(); ;
}
//*** Reply ***'
Label lblReply = (Label)e.Row.FindControl("lblReply");
if ((lblReply != null))
{
lblReply.Text = DataBinder.Eval(e.Row.DataItem, "Reply").ToString(); ;
}
}
}
protected void Page_UnLoad(object sender, EventArgs e)
{
objConn.Close();
objConn = null;
}
}
}
NewQuestion.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Diagnostics;
using System.Data.OleDb;
using System.Text;
namespace AspNetWebboardCS
{
public partial class NewQuestion : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnSave_Click(object sender, EventArgs e)
{
OleDbConnection objConn = null;
StringBuilder strSQL = new StringBuilder();
OleDbCommand objCmd = null;
string strConnString = null;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|DataDirectory|\\database.mdb";
objConn = new OleDbConnection(strConnString);
objConn.Open();
//*** Insert Reply ***'
strSQL.Append(" INSERT INTO webboard (CreateDate,Question,Details,Name,[View],Reply) ");
strSQL.Append(" VALUES ");
strSQL.Append(" (@sCreateDate,@sQuestion,@sDetails,@sName,@sView,@sReply) ");
objCmd = new OleDbCommand(strSQL.ToString(), objConn);
objCmd.Parameters.Add("@sCreateDate", OleDbType.Date).Value = DateTime.Now.ToString();
objCmd.Parameters.Add("@sQuestion", OleDbType.VarChar).Value = this.txtQuestion.Text;
objCmd.Parameters.Add("@sDetails", OleDbType.VarChar).Value = this.txtDetails.Text;
objCmd.Parameters.Add("@sName", OleDbType.VarChar).Value = this.txtName.Text;
objCmd.Parameters.Add("@sView", OleDbType.Integer).Value = 0;
objCmd.Parameters.Add("@sReply", OleDbType.Integer).Value = 0;
objCmd.ExecuteNonQuery();
objConn.Close();
objConn = null;
Response.Redirect("Webboard.aspx");
}
}
}
ViewWebboard.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Diagnostics;
using System.Data.OleDb;
using System.Text;
namespace AspNetWebboardCS
{
public partial class ViewWebboard : System.Web.UI.Page
{
protected OleDbConnection objConn;
protected string strQuestionID = HttpContext.Current.Request.QueryString["QuestionID"];
protected int strReplyNo = 1;
protected void Page_Load(object sender, EventArgs e)
{
string strConnString = null;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|DataDirectory|\\database.mdb";
objConn = new OleDbConnection(strConnString);
objConn.Open();
ShowQuestion();
ShowReply();
}
protected void ShowQuestion()
{
OleDbDataAdapter dtAdapter = null;
OleDbCommand objCmd = null;
DataTable dt = new DataTable();
StringBuilder strSQL = new StringBuilder();
strSQL.Append("SELECT * FROM webboard ");
strSQL.Append(" WHERE QuestionID = " + strQuestionID + " ");
dtAdapter = new OleDbDataAdapter(strSQL.ToString(), objConn);
dtAdapter.Fill(dt);
if (dt.Rows.Count > 0)
{
this.lblQuestion.Text = dt.Rows[0]["Question"].ToString();
this.lblDetails.Text = dt.Rows[0]["Details"].ToString();
this.lblName.Text = "<b>Name : </b>" + dt.Rows[0]["Name"].ToString();
this.lblCreateDate.Text = "<b>CreateDate : </b>" + Convert.ToDateTime(dt.Rows[0]["CreateDate"]).ToString("dd/MM/yyyy HH:mm").ToString();
this.lblReply.Text = "<b>Reply : </b>" + dt.Rows[0]["Reply"].ToString();
this.lblView.Text = "<b>View : </b>" + dt.Rows[0]["View"].ToString();
}
//*** Update Number of View ***'
strSQL.Remove(0, strSQL.Length);
strSQL.Append(" UPDATE webboard SET [View] = [View] + 1 ");
strSQL.Append(" WHERE QuestionID = @sQuestionID ");
objCmd = new OleDbCommand(strSQL.ToString(), objConn);
objCmd.Parameters.Add("@sQuestionID", OleDbType.VarChar).Value = strQuestionID.ToString();
objCmd.ExecuteNonQuery();
}
protected void ShowReply()
{
StringBuilder strSQL = new StringBuilder();
OleDbCommand objCmd = null;
strSQL.Append(" SELECT * FROM reply ");
strSQL.Append(" WHERE QuestionID = " + strQuestionID + " ");
OleDbDataReader dtReader = null;
objCmd = new OleDbCommand(strSQL.ToString(), objConn);
dtReader = objCmd.ExecuteReader();
//*** BindData to Repeater ***'
myRepeater.DataSource = dtReader;
myRepeater.DataBind();
dtReader.Close();
dtReader = null;
}
protected void Page_UnLoad(object sender, EventArgs e)
{
objConn.Close();
objConn = null;
}
protected void btnSave_Click(object sender, EventArgs e)
{
StringBuilder strSQL = new StringBuilder();
OleDbCommand objCmd = null;
//*** Insert Reply ***'
strSQL.Append(" INSERT INTO reply (QuestionID,CreateDate,Details,Name) ");
strSQL.Append(" VALUES ");
strSQL.Append(" (@sQuestionID,@sCreateDate,@sDetails,@sName) ");
objCmd = new OleDbCommand(strSQL.ToString(), objConn);
objCmd.Parameters.Add("@sQuestionID", OleDbType.VarChar).Value = strQuestionID.ToString();
objCmd.Parameters.Add("@sCreateDate", OleDbType.Date).Value = DateTime.Now.ToString();
objCmd.Parameters.Add("@sDetails", OleDbType.VarChar).Value = this.txtDetails.Text;
objCmd.Parameters.Add("@sName", OleDbType.VarChar).Value = this.txtName.Text;
objCmd.ExecuteNonQuery();
//*** Update Number of Reply ***'
strSQL.Remove(0, strSQL.Length);
strSQL.Append(" UPDATE webboard SET Reply = Reply + 1 ");
strSQL.Append(" WHERE QuestionID = @sQuestionID ");
objCmd = new OleDbCommand(strSQL.ToString(), objConn);
objCmd.Parameters.Add("@sQuestionID", OleDbType.VarChar).Value = strQuestionID.ToString();
objCmd.ExecuteNonQuery();
Response.Redirect("ViewWebboard.aspx?QuestionID=" + strQuestionID);
}
protected void myRepeater_ItemDataBound(object sender, RepeaterItemEventArgs e)
{
if (e.Item.ItemType == ListItemType.Item | e.Item.ItemType == ListItemType.AlternatingItem)
{
//*** No ***'
Label lblReplyNo = (Label)e.Item.FindControl("lblReplyNo");
if ((lblReplyNo != null))
{
lblReplyNo.Text = "No : " + strReplyNo;
strReplyNo = strReplyNo + 1;
}
//*** Details ***'
Label lblReplyDetails = (Label)e.Item.FindControl("lblReplyDetails");
if ((lblReplyDetails != null))
{
lblReplyDetails.Text = (string)DataBinder.Eval(e.Item.DataItem, "Details");
}
//*** Name ***'
Label lblReplyName = (Label)e.Item.FindControl("lblReplyName");
if ((lblReplyName != null))
{
lblReplyName.Text = (string)DataBinder.Eval(e.Item.DataItem, "Name");
}
//*** CreateDate ***'
Label lblReplyCreateDate = (Label)e.Item.FindControl("lblReplyCreateDate");
if ((lblReplyCreateDate != null))
{
lblReplyCreateDate.Text = Convert.ToDateTime(DataBinder.Eval(e.Item.DataItem, "CreateDate")).ToString("dd/MM/yyyy HH:mm");
}
}
}
}
}

Screenshot
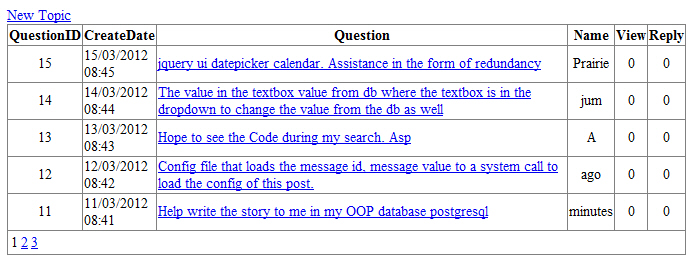
หน้าจอแสดงคำถามหรือกระทู้
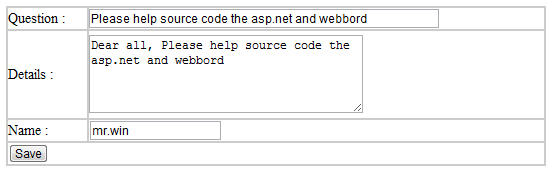
หน้าจอสำหรับตั้งกระทู้ใหม่
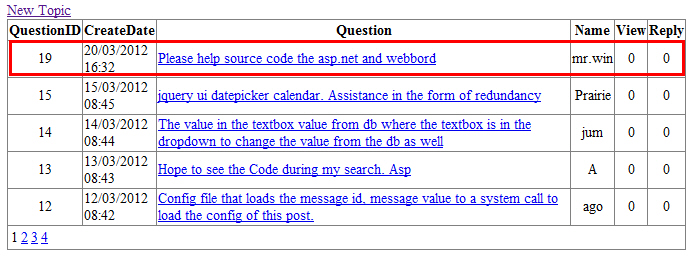
กระทู้ที่ถูกตั้งขึ้นมาใหม่
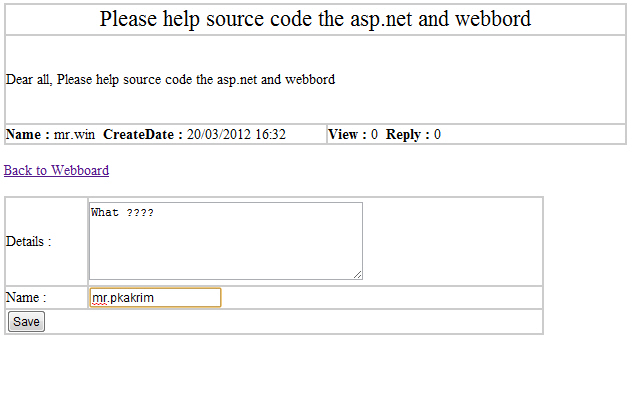
สำหรับแสดงความคิดเห็น
Download Code !!
|
|
|
Score Rating : |
 |
|
Create Date : |
2012-03-21 20:17:05 |
|
View : |
32,981 |
|
Download : |
No files
|
|
|
|
|
|
|
|