|
 |
|
Java ช่วยหน่อยค่ะ ด่วนๆๆ ใครที่เก่งเขียนโปรแกรมโดยเรียกใช้ ไฟล์ มั้งค่ะ |
|
 |
|
|
 |
 |
|
ต้องการเพิ่มชนิดกาแฟ แล้วให้ระบบบันทึกข้อมูลของชนิดกาแฟ 4 บรรทัดแรก (Mocca 25 30 35 ) พร้อมกำหนดหมายเลข ต่อท้ายรายชื่อกาแฟทั้งหมดที่มีอยู่แล้วในไฟล์ coffee.txt
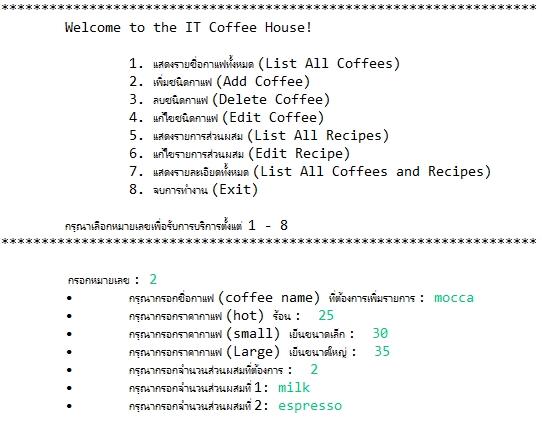
Code (Java)
//ไฟล์ coffee.txt
1. Cappuccino 25 30 40
2. Espresso 30 30 45
Code (Java)
//คลาสแสดงรายชื่อกาแฟ
public class ListAllCoffees {
private String number;
private String coffeeName;
private int priceHot;
private int priceSmall;
private int priceLarge;
public ListAllCoffees(String number, String coffeeName, int price1, int price2,
int price3) {
this.number = number;
this.coffeeName = coffeeName;
this.priceHot = price1;
this.priceSmall = price2;
this.priceLarge = price3;
}
public String toString(){
return " "+number +" "+coffeeName +" "+priceHot +" "+priceSmall +" "+priceLarge;
}
}
Code (Java)
//คลาสเพิ่มชนิดกาแฟ
import java.util.Scanner;
public class AddCoffee {
private String coffeeName;
private int priceHot;
private int priceSmall;
private int priceLarge;
private int count;
private String ingredient;
public void AddCoffee() {
Scanner scan = new Scanner(System.in);
System.out.print("\t• กรุณากรอกชื่อกาแฟ (coffee name) ที่ต้องการเพิ่มรายการ : ");
coffeeName = scan.next();
System.out.print("\t• กรุณากรอกราคากาแฟ (hot) ร้อน : ");
priceHot = scan.nextInt();
System.out.print("\t• กรุณากรอกราคากาแฟ (small) เย็นขนาดเล็ก : ");
priceSmall = scan.nextInt();
System.out.print("\t• กรุณากรอกราคากาแฟ (Large) เย็นขนาดใหญ่ : ");
priceLarge = scan.nextInt();
System.out.print("\t• กรุณากรอกจำนวนส่วนผสมที่ต้องการ : ");
count = scan.nextInt();
for (int i = 0; i < count; i++) {
System.out.print("\t• กรุณากรอกจำนวนส่วนผสมที่ "+(i+1)+": ");
ingredient = scan.next();
}
}
}
Code (Java)
//คลาสรัน
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Run {
public static void main(String[] args) throws Exception {
ListAllCoffees[] listCof = new ListAllCoffees[2];
AddCoffee add = new AddCoffee();
ListAllRecipes[] listRe = new ListAllRecipes[1];
DeleteCoffee del = new DeleteCoffee();
System.out
.println("*******************************************************************\n"
+ "\tWelcome to the IT Coffee House!\n\n"
+ "\t\t1. แสดงรายชื่อกาแฟทั้งหมด (List All Coffees)\n"
+ "\t\t2. เพิ่มชนิดกาแฟ (Add Coffee)\n"
+ "\t\t3. ลบชนิดกาแฟ (Delete Coffee)\n"
+ "\t\t4. แก้ไขชนิดกาแฟ (Edit Coffee)\n"
+ "\t\t5. แสดงรายการส่วนผสม (List All Recipes)\n"
+ "\t\t6. แก้ไขรายการส่วนผสม (Edit Recipe)\n"
+ "\t\t7. แสดงรายละเอียดทั้งหมด (List All Coffees and Recipes)\n"
+ "\t\t8. จบการทำงาน (Exit)\n\n"
+ "\tกรุณาเลือกหมายเลขเพื่อรับการบริการตั้งแต่ 1 - 8\n"
+ "*******************************************************************\n");
Scanner scan = new Scanner(System.in);
System.out.print("\t กรอกหมายเลข : ");
int menu = scan.nextInt();
if (menu == 1) {
try {
File file = new File("coffee.txt");
Scanner inputRead = new Scanner(file);
int i = 0;
while (inputRead.hasNext()) {
listCof[i] = new ListAllCoffees(inputRead.next(),
inputRead.next(), inputRead.nextInt(),
inputRead.nextInt(), inputRead.nextInt());
i++;
}
inputRead.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
for (int i = 0; i < listCof.length; i++) {
System.out.println(" \t" + listCof[i].toString());
}
} else if (menu == 2) {
add.AddCoffee();
}
}
}
Tag : Java, C#, JAVA

|
ประวัติการแก้ไข 2015-05-09 14:18:43
|
 |
 |
 |
 |
Date :
2015-05-09 14:18:01 |
By :
iamja |
View :
1783 |
Reply :
3 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (Java)
public class ListAllCoffees {
public String number;
public String coffeeName;
public int priceHot;
public int priceSmall;
public int priceLarge;
public ListAllCoffees() {
}
public ListAllCoffees(String number, String coffeeName, int price1, int price2,
int price3) {
this.number = number;
this.coffeeName = coffeeName;
this.priceHot = price1;
this.priceSmall = price2;
this.priceLarge = price3;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getCoffeeName() {
return coffeeName;
}
public void setCoffeeName(String coffeeName) {
this.coffeeName = coffeeName;
}
public int getPriceHot() {
return priceHot;
}
public void setPriceHot(int priceHot) {
this.priceHot = priceHot;
}
public int getPriceSmall() {
return priceSmall;
}
public void setPriceSmall(int priceSmall) {
this.priceSmall = priceSmall;
}
public int getPriceLarge() {
return priceLarge;
}
public void setPriceLarge(int priceLarge) {
this.priceLarge = priceLarge;
}
public String toString() {
return " " + number + " " + coffeeName + " " + priceHot + " " + priceSmall + " " + priceLarge;
}
}
Code (Java)
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scan = new Scanner(System.in);
ListAllCoffees list = new ListAllCoffees();
list.number = scan.next();
list.coffeeName = scan.next();
list.priceHot = scan.nextInt();
list.priceSmall = scan.nextInt();
list.priceLarge = scan.nextInt();
System.out.println(list.toString());
}
|
 |
 |
 |
 |
Date :
2015-05-09 23:41:24 |
By :
ipstarone |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 03
|