 |
|
Code
package newpackage;
import java.awt.EventQueue;
import javax.swing.JLabel;
import javax.swing.JFrame;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import javax.swing.ButtonGroup;
import javax.swing.JOptionPane;
import javax.swing.JButton;
import javax.swing.JRadioButton;
import java.awt.EventQueue;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JOptionPane;
import javax.swing.JFrame;
import javax.swing.JTable;
import javax.swing.JScrollPane;
import javax.swing.table.DefaultTableModel;
import javax.swing.JLabel;
import javax.swing.JTextField;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.io.*;
/**
/**
*
* @author Aong
*/
public class NewJFrame extends javax.swing.JFrame {
/**
* Creates new form NewJFrame
*/
//public NewJFrame() {
// initComponents();
// }
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
pack();
}// </editor-fold>
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
NewJFrame frame = new NewJFrame();
frame.setVisible(true);
}
});
}
public NewJFrame() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 1000, 700);
setTitle("เลือกราคา");
getContentPane().setLayout(null);
JLabel lblSearch = new JLabel("โปรดระบุช่วงราคา :");
lblSearch.setBounds(150, 60,200, 14);
getContentPane().add(lblSearch);
JLabel lblSearch1 = new JLabel("โปรระบุอุปกรณ์ที่ต้องการ :");
lblSearch1.setBounds(150,180,200, 14);
getContentPane().add(lblSearch1);
JLabel lblSearch2 = new JLabel("โปรระบุยี่ห้อที่ต้องการ :");
lblSearch2.setBounds(150, 290,200, 14);
getContentPane().add(lblSearch2);
// Radio Button
final JRadioButton radio1 = new JRadioButton("ราคาน้อยกว่า1000");
radio1.setBounds(119, 90, 150, 23);
getContentPane().add(radio1);
final JRadioButton radio2 = new JRadioButton("ราคาไม่เกิน2000");
radio2.setBounds(119,120, 150, 23);
getContentPane().add(radio2);
final JRadioButton radio3 = new JRadioButton("ราคามากกว่า2000");
radio3.setBounds(119,150, 150, 23);
getContentPane().add(radio3);
final JRadioButton radio4 = new JRadioButton("Harddisk");
radio4.setBounds(119, 200, 150, 23);
getContentPane().add(radio4);
final JRadioButton radio5 = new JRadioButton("Display Card");
radio5.setBounds(119, 230, 150, 23);
getContentPane().add(radio5);
final JRadioButton radio6 = new JRadioButton("Ram");
radio6.setBounds(119, 260, 150, 23);
getContentPane().add(radio6);
final JRadioButton radio7 = new JRadioButton("Ram");
radio7.setBounds(119,310, 150, 23);
getContentPane().add(radio7);
final JRadioButton radio8 = new JRadioButton("Ram");
radio8.setBounds(119, 340, 150, 23);
getContentPane().add(radio8);
final JRadioButton radio9 = new JRadioButton("Ram");
radio9.setBounds(119, 370, 150, 23);
getContentPane().add(radio9);
final JRadioButton radio10 = new JRadioButton("Ram");
radio10.setBounds(119, 400, 150, 23);
getContentPane().add(radio10);
// Set Group
ButtonGroup group = new ButtonGroup();
group.add(radio1);
group.add(radio2);
group.add(radio3);
ButtonGroup group2 = new ButtonGroup();
group2.add(radio4);
group2.add(radio5);
group2.add(radio6);
ButtonGroup group3 = new ButtonGroup();
group3.add(radio7);
group3.add(radio8);
group3.add(radio9);
group3.add(radio10);
// ScrollPane
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(300,100, 600, 400);
getContentPane().add(scrollPane);
// Table
JTable table = new JTable();
scrollPane.setViewportView(table);
DefaultTableModel model = (DefaultTableModel)table.getModel();
model.addColumn("id");
model.addColumn("name");
model.addColumn("price");
// Button
JButton btn = new JButton("OK");
btn.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
Connection connect = null;
Statement s = null;
if(radio1.isSelected()){
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/store" +
"?user=root&password=1234");
s = connect.createStatement();
String sql = "SELECT * FROM materiel WHERE price between 0 and 1000 ";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addRow(new Object[0]);
model.setValueAt(rec.getString("id"), row, 0);
model.setValueAt(rec.getString("name"), row, 1);
model.setValueAt(rec.getString("price"), row, 2);
row++;
}
model.removeRow(0);
connect.close();
rec.close();
}
catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
} else if (radio2.isSelected()) {
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/store" +
"?user=root&password=1234");
s = connect.createStatement();
String sql = "SELECT * FROM materiel WHERE price between 1000 and 2000 ";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addRow(new Object[0]);
model.setValueAt(rec.getString("id"), row, 0);
model.setValueAt(rec.getString("name"), row, 1);
model.setValueAt(rec.getString("price"), row, 2);
row++;
}
connect.close();
rec.close();
model.removeRow(0);
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
} else if (radio3.isSelected()) {
} else {
JOptionPane.showMessageDialog(null,
"You not select.");
}
}
});
btn.setBounds(125, 450, 89, 23);
getContentPane().add(btn);
}
}
สอบถามหน่อยครับจะทำการเคลียข้อมูลเก่ายังไงหรอครับ พอกดปุ่มราคาไม่เกิน2000บาทแล้วกลับมากดราคาไม่เกิน1000มันมีข้อมูลที่หลงเหลืออยู่4-5ข้อมูลที่ราคาเกิน1000ขึ้นไปเข้ามาแสดง ช่วยทีคับ
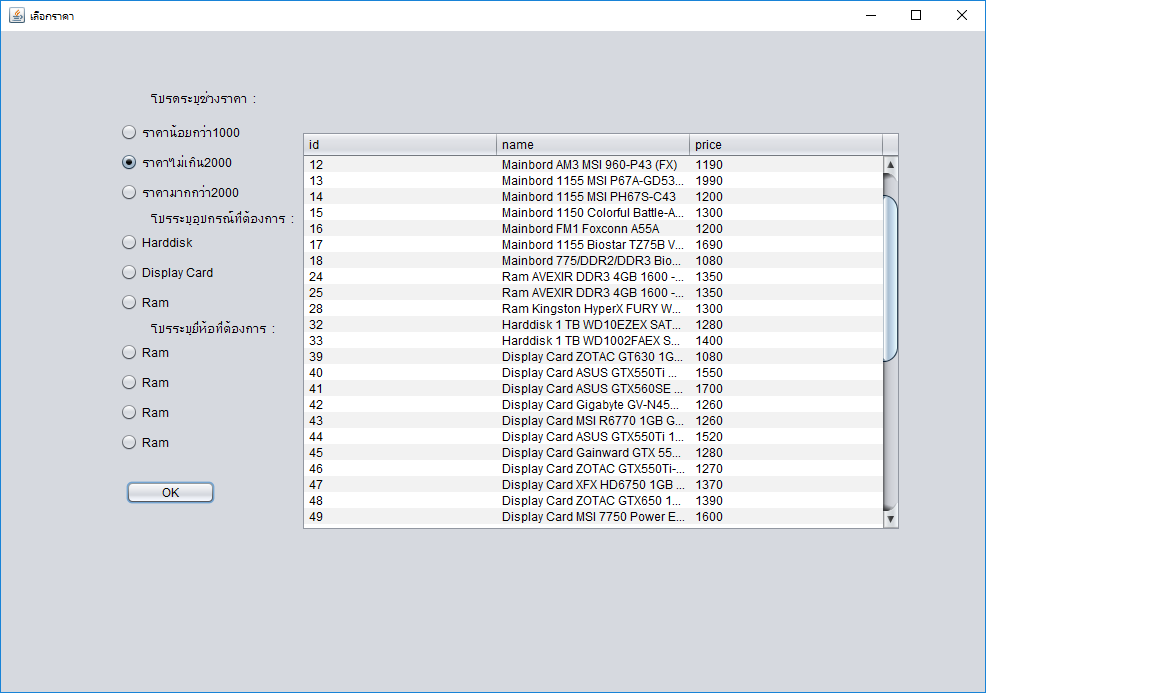
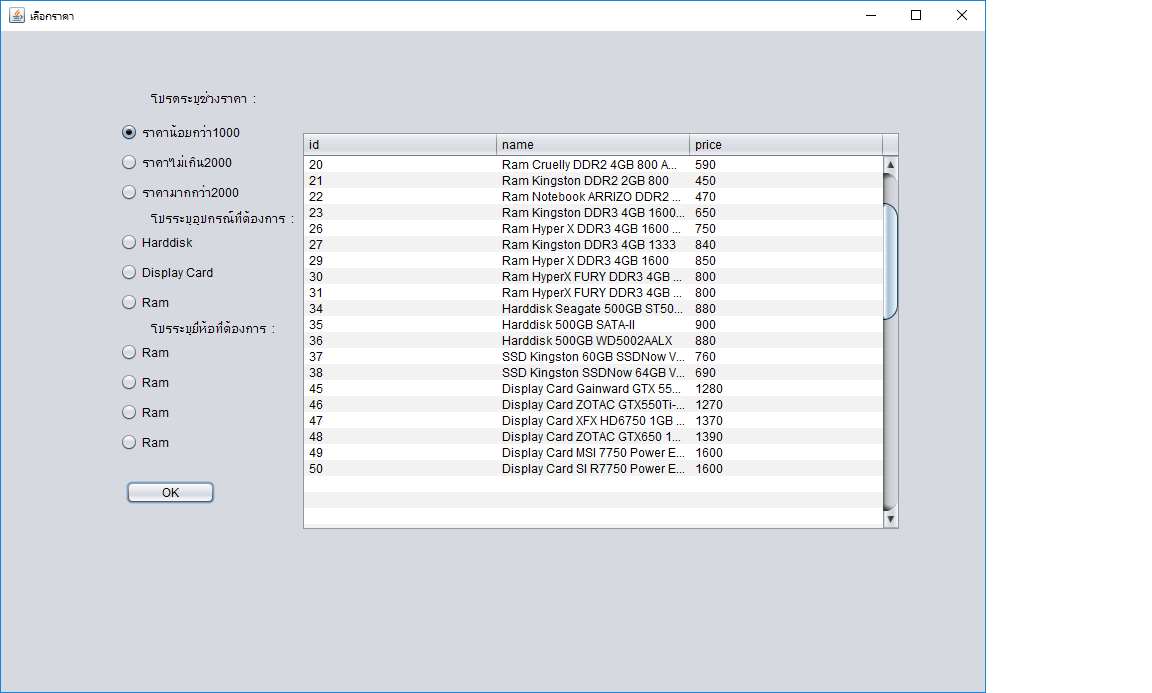
Tag : Java, MySQL, JAVA
|
|
 |
 |
 |
 |
Date :
2017-08-03 01:51:24 |
By :
1520169821336560 |
View :
1480 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |