Java Convert HashMap/ArrayList Write to CSV file |
Java Convert HashMap/ArrayList Write to CSV file บทความการเขียนโปรแกรมภาษา Java เพื่อทำการอ่านข้อมูลจากตัวแปรในภาษา Java เช่น HashMap/ArrayList ซึ่งประกอบด้วย Key และ Value หลาย ๆ Index แล้วนำข้อมูลเหล่านั้นเขียนลงใน CSV
Example การอ่านข้อมูลจาก HashMap/ArrayList แล้งเขียนลง CSV
MyClass.java
package com.java.myapp;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
public class MyClass {
public static void main(String[] args) {
String path = "C:\\java\\thaicreate.csv";
try {
ArrayList<HashMap<String, String>> myArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
/*** Rows 1 ***/
map = new HashMap<String, String>();
map.put("CustomerID","C001");
map.put("Name", "Win Weerachai");
map.put("Email", "[email protected]");
map.put("CountryCode", "TH");
map.put("Budget", "1000000");
map.put("Used", "600000");
myArrList.add(map);
/*** Rows 2 ***/
map = new HashMap<String, String>();
map.put("CustomerID","C002");
map.put("Name", "John Smith");
map.put("Email", "[email protected]");
map.put("CountryCode", "UK");
map.put("Budget", "2000000");
map.put("Used", "800000");
myArrList.add(map);
/*** Rows 3 ***/
map = new HashMap<String, String>();
map.put("CustomerID","C003");
map.put("Name", "Jame Born");
map.put("Email", "[email protected]");
map.put("CountryCode", "US");
map.put("Budget", "3000000");
map.put("Used", "600000");
myArrList.add(map);
/*** Rows 4 ***/
map = new HashMap<String, String>();
map.put("CustomerID","C004");
map.put("Name", "Chalee Angel");
map.put("Email", "[email protected]");
map.put("CountryCode", "US");
map.put("Budget", "4000000");
map.put("Used", "100000");
myArrList.add(map);
FileWriter writer;
writer = new FileWriter(path, true); //True = Append to file, false = Overwrite
// Write CSV
for (int i = 0; i < myArrList.size(); i++) {
writer.write(myArrList.get(i).get("CustomerID").toString());
writer.write(",");
writer.write(myArrList.get(i).get("Name").toString());
writer.write(",");
writer.write(myArrList.get(i).get("Email").toString());
writer.write(",");
writer.write(myArrList.get(i).get("CountryCode").toString());
writer.write(",");
writer.write(myArrList.get(i).get("Budget").toString());
writer.write(",");
writer.write(myArrList.get(i).get("Used").toString());
writer.write("\r\n");
}
System.out.println("Write success!");
writer.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Output
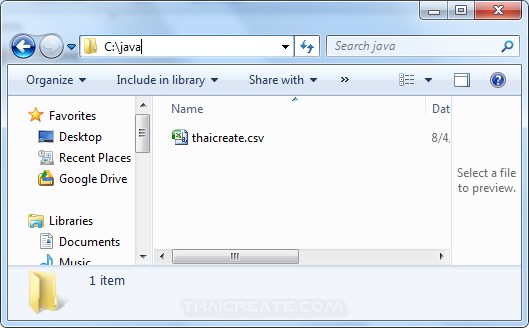
ไฟล์ CSV ที่ถูกสร้าง
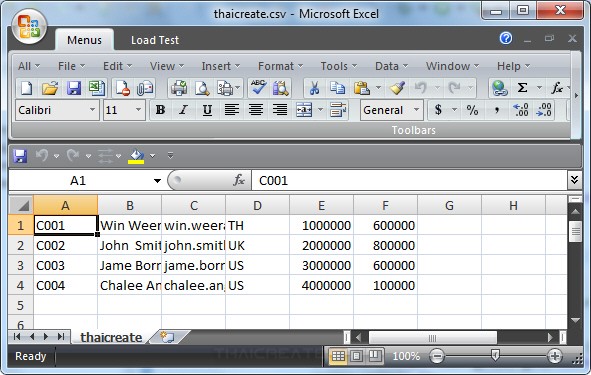
ไฟล์ CSV เมื่อเปิดผ่านโปรแกรม Excel
thaicreate.csv
C001,Win Weerachai,[email protected],TH,1000000,600000
C002,John Smith,[email protected],UK,2000000,800000
C003,Jame Born,[email protected],US,3000000,600000
C004,Chalee Angel,[email protected],US,4000000,100000

|