Java Generate Excel Report from Database (jExcelAPI) |
Java Generate Excel Report from Database (jExcelAPI) บทความนี้จะเป็นการสร้าง Excel Report ด้วย Java และ Library ของ jExcelAPI ซึ่งข้อมูลของ Report จะทำการอ่านมาจาก Database ส่วนวิธีการนั้นก็คือ จะทำการอ่านข้อมูลมาจาก Database และหลังจากที่ได้ข้อมูลจาก Database แล้วเราจะใช้การ Loop เพื่อเขียนลงในไฟล์ Excel ซึ่งจะเป็นการ Apply การใช้งานจากบทความก่อน ๆ
Java Excel and Create Excel file (jExcelAPI)
Example Excel Report
Example ตัวอย่างการสร้าง Excel Report ที่ข้อมูลถูกส่งออกมาจาก Database
MySQL Database
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ MySQL Database
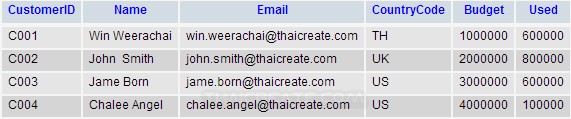
โครงสร้างของ MySQL Database
MyClass.java
package com.java.myapp;
import java.io.File;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import jxl.Workbook;
import jxl.format.Alignment;
import jxl.format.Border;
import jxl.format.BorderLineStyle;
import jxl.format.Colour;
import jxl.format.VerticalAlignment;
import jxl.write.Label;
import jxl.write.WritableCellFormat;
import jxl.write.WritableFont;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
public class MyClass {
public static void main(String[] args) {
try{
//*** for Database Connected ***//
Connection connect = null;
Statement s = null;
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
//*** for Excel Report ***//
String fileName = "C:\\java\\myExcel.xls";
WritableWorkbook workbook = Workbook.createWorkbook(new File(fileName));
//*** Create Font ***//
WritableFont fontBlue = new WritableFont(WritableFont.TIMES, 10);
fontBlue.setColour(Colour.BLUE);
WritableFont fontRed = new WritableFont(WritableFont.TIMES, 10);
fontRed.setColour(Colour.RED);
//*** Sheet 1 ***//
WritableSheet ws1 = workbook.createSheet("mySheet1", 0);
//*** Header ***//
WritableCellFormat cellFormat1 = new WritableCellFormat(fontRed);
//cellFormat2.setBackground(Colour.ORANGE);
cellFormat1.setAlignment(Alignment.CENTRE);
cellFormat1.setVerticalAlignment(VerticalAlignment.CENTRE);
cellFormat1.setBorder(Border.ALL, BorderLineStyle.THIN);
//*** Data ***//
WritableCellFormat cellFormat2 = new WritableCellFormat(fontBlue);
// cellFormat2.setWrap(true);
cellFormat2.setAlignment(jxl.format.Alignment.CENTRE);
cellFormat2.setVerticalAlignment(VerticalAlignment.CENTRE);
cellFormat2.setWrap(true);
cellFormat2.setBorder(jxl.format.Border.ALL, jxl.format.BorderLineStyle.HAIR,
jxl.format.Colour.BLACK);
ws1.mergeCells(0, 0, 5, 0);
Label lable = new Label(0, 0,"Customer Report", cellFormat1);
ws1.addCell(lable);
//*** Header ***//
ws1.setColumnView(0, 10); // Column CustomerID
ws1.addCell(new Label(0,1,"CustomerID",cellFormat1));
ws1.setColumnView(1, 15); // Column Name
ws1.addCell(new Label(1,1,"Name",cellFormat1));
ws1.setColumnView(2, 25); // Column Email
ws1.addCell(new Label(2,1,"Email",cellFormat1));
ws1.setColumnView(3, 12); // Column CountryCode
ws1.addCell(new Label(3,1,"CountryCode",cellFormat1));
ws1.setColumnView(4, 10); // Column Budget
ws1.addCell(new Label(4,1,"Budget",cellFormat1));
ws1.setColumnView(5, 10); // Column Used
ws1.addCell(new Label(5,1,"Used",cellFormat1));
int iRows = 2;
while((rec!=null) && (rec.next()))
{
ws1.addCell(new Label(0,iRows,rec.getString("CustomerID"),cellFormat2));
ws1.addCell(new Label(1,iRows,rec.getString("Name"),cellFormat2));
ws1.addCell(new Label(2,iRows,rec.getString("Email"),cellFormat2));
ws1.addCell(new Label(3,iRows,rec.getString("CountryCode"),cellFormat2));
ws1.addCell(new Label(4,iRows,rec.getString("Budget"),cellFormat2));
ws1.addCell(new Label(5,iRows,rec.getString("Used"),cellFormat2));
++iRows;
}
workbook.write();
workbook.close();
System.out.println("Excel file created.");
// Close
try {
if(connect != null){
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
catch (Exception e) {
e.printStackTrace();
}
}
}
Output
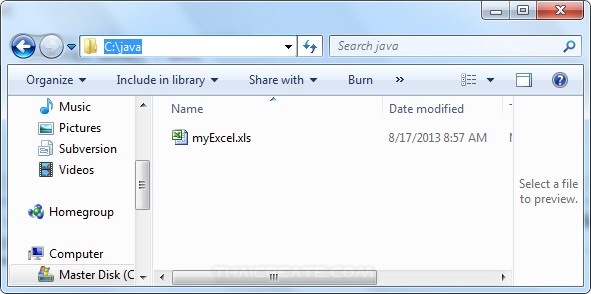
ไฟล์ Excel ที่ได้
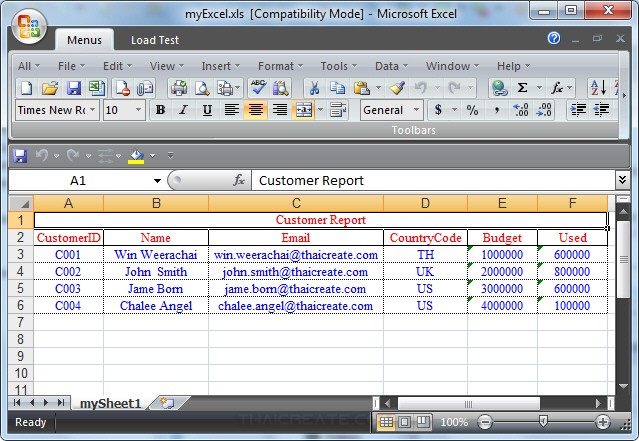
เปิดเปิดไฟล์ Excel
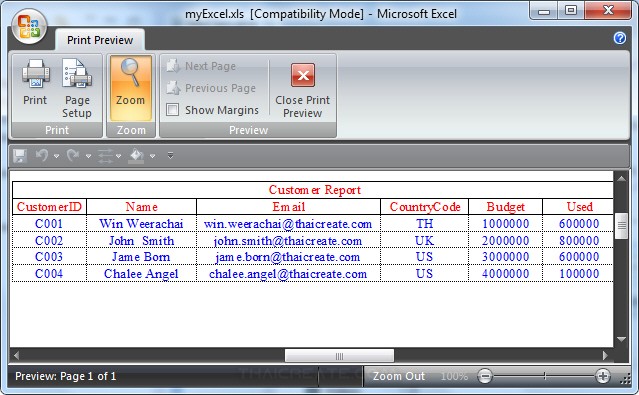
ผลลัพธ์ของ Excel ทีได้เมื่อผ่านการ Print Preview บน Excel
|