Java GUI and Oracle Database |
Java GUI and Oracle Database (Java) หัวข้อนี้จะเป็นการเขียน Java GUI กับการติดต่อกับฐานข้อมูล Database ของ Oracle แบบง่าย ๆ โดยจะยกตัวอย่างการเขียนเพื่อติดต่อกับ Oracle เช่น การเชื่อมต่อว่ามีวิธีการอย่างไร การอ่านข้อมูลจาก Oracle มาแสดงในหน้า JFrame กับ JTable และตัวอย่าง Code สำหรับการ Insert ข้อมูล , Update ข้อมูล และการ Delete ข้อมูล ซึ่งตัวอย่างนี้สามารถนำไปประยุกต์ใช้งานกับการเขียน Java GUI กับ Databae Oracle ได้หลากหลาย
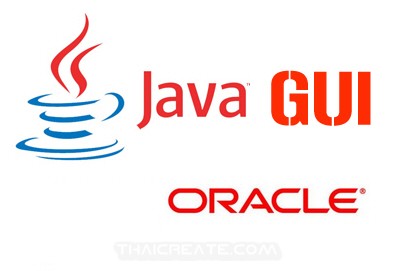
Java GUI and Oracle Database
Basic Java and Oracle Database (JDBC/OracleDriver)
โครงสร้าง JAR Library และ Oracle Database

ไฟล์ jar ซึ่งเป็๋น Connector Library ไว้ติดต่อกับ Database ของ Oracle
Table
create table customer
(
CUSTOMERID varchar2(4),
NAME varchar2(50),
EMAIL varchar2(50),
COUNTRYCODE varchar2(3),
BUDGET number,
USED number
)
tablespace SYSTEM
storage
(
initial 64K
minextents 1
maxextents unlimited
);
alter table customer
add constraint PK_CUSTOMER primary key (CUSTOMERID);
INSERT INTO customer VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO customer VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO customer VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO customer VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);

โครงสร้างของ Database
รูปแบบการเชื่อมต่อระหว่าง Java GUI กับ Oracle
Connection connect = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connect = DriverManager.getConnection("" +
"jdbc:oracle:thin:@TCDB", "myuser","mypassword");
if(connect != null){
System.out.println("Database Connected.");
} else {
System.out.println("Database Connect Failed.");
}
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
try {
connect.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
Example ตัวอย่างการเขียน Java GUI เพื่ออานข้อมูลจาก Oracle มาแสดงในหน้า JFrame กับ JTable
อ่านบทความ Java GUI กับ JTable วิธีการใช้งาน JTable พื้นฐาน (แนะนำ)
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JOptionPane;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import javax.swing.JLabel;
public class MyForm extends JFrame {
Connection connect = null;
Statement s = null;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 580, 242);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// Customer Label
JLabel lblCustomer = new JLabel("Customer List");
lblCustomer.setBounds(231, 28, 95, 14);
getContentPane().add(lblCustomer);
// ScrollPane for Table
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(33, 61, 494, 90);
getContentPane().add(scrollPane);
// Table
JTable table = new JTable();
// Model for Table
DefaultTableModel model = (DefaultTableModel)table.getModel();
model.addColumn("CustomerID");
model.addColumn("Name");
model.addColumn("Email");
model.addColumn("CountryCode");
model.addColumn("Budget");
model.addColumn("Used");
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connect = DriverManager.getConnection("" +
"jdbc:oracle:thin:@TCDB", "myuser","mypassword");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addRow(new Object[0]);
model.setValueAt(rec.getString("CustomerID"), row, 0);
model.setValueAt(rec.getString("Name"), row, 1);
model.setValueAt(rec.getString("Email"), row, 2);
model.setValueAt(rec.getString("CountryCode"), row, 3);
model.setValueAt(rec.getFloat("Budget"), row, 4);
model.setValueAt(rec.getFloat("Used"), row, 5);
row++;
/*
* model.addRow(new Object[]{
rec.getString("CustomerID")
,rec.getString("Name"),rec.getString("Email")
,rec.getString("CountryCode")
,rec.getFloat("Budget")
,rec.getFloat("Used")
});
*/
}
rec.close();
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
scrollPane.setViewportView(table);
}
}
Output
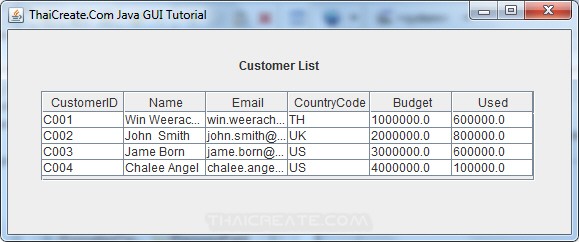
ผลลัพธ์ที่ได้จากการอ่านข้อมูงจาก Database มาแสดงใน JTable และสำหรับการใช้งานพื้นฐาน JTable แนะนำให้อ่านบทความของ JTable
ตัวอย่างรูปแบบการเชื่อมต่อและกระทำกับ Database เช่น Add Insert/Update/Delete
Insert
Connection connect = null;
Statement s = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connect = DriverManager.getConnection("" +
"jdbc:oracle:thin:@TCDB", "myuser","mypassword");
s = connect.createStatement();
String sql = "INSERT INTO customer " +
"(CustomerID,Name,Email,CountryCode,Budget,Used) " +
"VALUES ('C005','Chai Surachai','[email protected]'" +
",'TH','1000000','0') ";
s.execute(sql);
System.out.println("Record Inserted Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
Read
Connection connect = null;
Statement s = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connect = DriverManager.getConnection("" +
"jdbc:oracle:thin:@TCDB", "myuser","mypassword");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
while((rec!=null) && (rec.next()))
{
System.out.print(rec.getString("CustomerID"));
System.out.print(" - ");
System.out.print(rec.getString("Name"));
System.out.print(" - ");
System.out.print(rec.getString("Email"));
System.out.print(" - ");
System.out.print(rec.getString("CountryCode"));
System.out.print(" - ");
System.out.print(rec.getFloat("Budget"));
System.out.print(" - ");
System.out.print(rec.getFloat("Used"));
System.out.println("");
}
rec.close();
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
Update
Connection connect = null;
Statement s = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connect = DriverManager.getConnection("" +
"jdbc:oracle:thin:@TCDB", "myuser","mypassword");
s = connect.createStatement();
String sql = "UPDATE customer " +
"SET Budget = '5000000' " +
" WHERE CustomerID = 'C005' ";
s.execute(sql);
System.out.println("Record Update Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
Delete
Connection connect = null;
Statement s = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connect = DriverManager.getConnection("" +
"jdbc:oracle:thin:@TCDB", "myuser","mypassword");
s = connect.createStatement();
String sql = "DELETE FROM customer " +
" WHERE CustomerID = 'C005' ";
s.execute(sql);
System.out.println("Record Delete Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
สำหรับการใช้งาน Java GUI กับ Oracle สามารถอ่านเพิ่มเติมได้ที่บทความ Java กับ Oracle
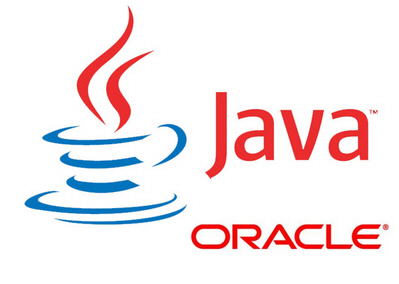
Java and Oracle Database (JDBC/OracleDriver)
Property & Method (Others Related) |
|