พื้นฐาน Java GUI : Dialog และ Popup สร้าง Input Dialog และ Custom Modal Dialog |
พื้นฐาน Java GUI : Dialog และ Popup สร้าง Input Dialog และ Custom Modal Dialog บทความนี้เราจะมาเรียนรู้เกี่ยวกับ Java GUI และการสร้าง Dialog และ Popuo ทั้งที่เป็น Input Dialog เช่น การสร้าง Input Dialog Popup แบบรับค่า Username / Password หรือการสร้าง Modal Dialog แบบ Confirm ที่จะต้องกด Yes/No หรือจะเป็นแบบ Custom Dialog Popup ที่ใช้การเรียก Form จาก Class อื่น แสดงเป็นแบบ Modal Dialog โดยใน Modal อาจจะสร้างเป็น Input ข้อมูล และหลังจากที่ได้ข้อมูลเรียบร้อยแล้วก็ส่งค่ากลับมายัง Form หลัก
Example 1 : ตัวอย่างการสร้าง Dialog Popup แบบ Cofirm ด้วย Yes/No
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
public class MyForm extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Tutorial");
setSize(450, 300);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Create Dialog
JButton btnButton = new JButton("Submit");
btnButton.setBounds(171, 95, 89, 23);
btnButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int n = JOptionPane.showConfirmDialog(null,
"I really like my book",
"Question (application-modal dialog)",
JOptionPane.YES_NO_OPTION,
JOptionPane.QUESTION_MESSAGE);
System.out.println(n); // Use n for response
}
});
getContentPane().add(btnButton);
}
}
Output
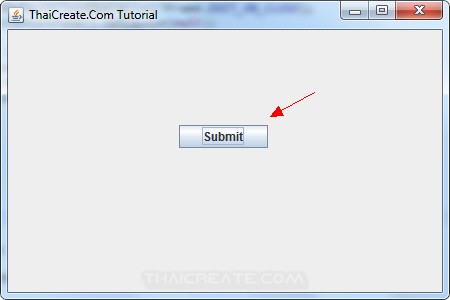
คลิกที่ Button เพื่อแสดง Dialog แบบ Yes/No
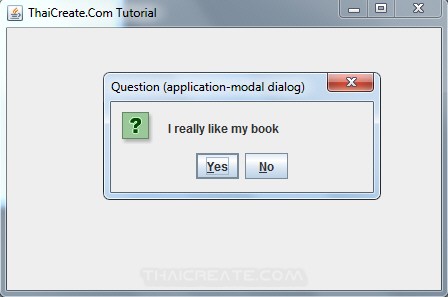
ทางเลือก Optional เป็น Yes/No โดยเมื่อกลับไปสามารถนำค่า n ไปใช้ได้
System.out.println(n);
Example 2 : ตัวอย่างการสร้าง Dialog Popup แบบมี Input ข้อมูลเช่น Name , Username และ Password
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class MyForm extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Tutorial");
setSize(450, 300);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Create Dialog
JButton btnButton = new JButton("Submit");
btnButton.setBounds(171, 95, 89, 23);
btnButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JTextField name = new JTextField();
JTextField username = new JTextField();
JPasswordField password = new JPasswordField();
final JComponent[] inputs = new JComponent[] {
new JLabel("Name"),
name,
new JLabel("Username"),
username,
new JLabel("Password"),
password
};
JOptionPane.showMessageDialog(null, inputs, "My custom dialog", JOptionPane.PLAIN_MESSAGE);
System.out.println("You entered " +
name.getText() + ", " +
username.getText() + ", " +
password.getText());
}
});
getContentPane().add(btnButton);
}
}
Output
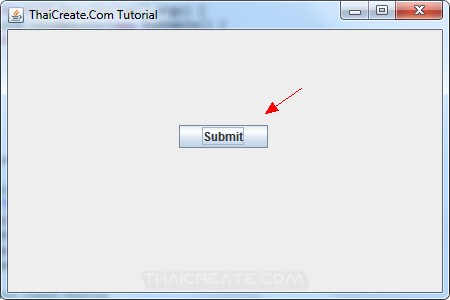
คลิกที่ Button เพื่อแสดง Dialog ที่มี Input ข้อมูล
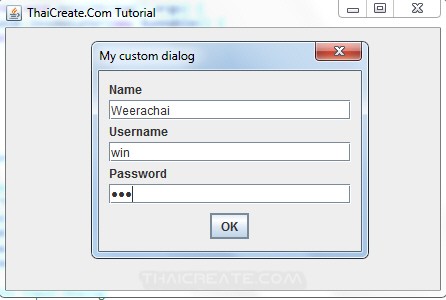
กรอกข้อมูใน Input Dialog และหลังจากที่กด OK สามารถ get ค่าได้โดยใช้คำสั่ง
System.out.println("You entered " +
name.getText() + ", " +
username.getText() + ", " +
password.getText());
Example 3 : ตัวอย่างการสร้าง Custom Modal Dialog แบบสร้าง Layout ขึ้นมาใหม่อีก Class
MyForm.java เป็น Form หลักไว้เปิด Dialog และแสดงค่าจาก Dialog
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class MyForm extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Tutorial");
setSize(450, 300);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Result
final JLabel lblResult = new JLabel("Result",JLabel.CENTER);
lblResult.setBounds(32, 53, 370, 14);
getContentPane().add(lblResult);
// Create Button Open Dialog
JButton btnButton = new JButton("Submit");
btnButton.setBounds(171, 95, 89, 23);
btnButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
MyDialogPopup dialog = new MyDialogPopup();
dialog.setModal(true);
dialog.setVisible(true);
lblResult.setText(dialog.sName);
}
});
getContentPane().add(btnButton);
}
}
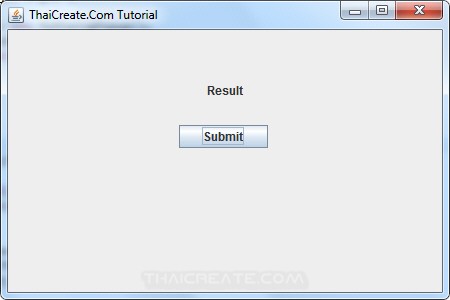
MyDialogPopup.java เป็น Dialog และ Input ข้อมูล
package com.java.myapp;
import javax.swing.JButton;
import javax.swing.JDialog;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import javax.swing.JTextField;
public class MyDialogPopup extends JDialog {
public String sName;
public static void main(String[] args) {
try {
MyDialogPopup dialog = new MyDialogPopup();
dialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
public MyDialogPopup() {
setBounds(100, 100, 296, 175);
setResizable(false);
setLocationRelativeTo(null);
getContentPane().setLayout(null);
// Create Input
final JTextField name = new JTextField();
name.setBounds(57, 36, 175, 20);
getContentPane().add(name);
// Button OK
JButton btnOK = new JButton("OK");
btnOK.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
sName = name.getText();
dispose();
}
});
btnOK.setBounds(70, 93, 78, 23);
getContentPane().add(btnOK);
// Button Cancel
JButton btnCancel = new JButton("Cancel");
btnCancel.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
sName = "";
dispose();
}
});
btnCancel.setBounds(158, 93, 74, 23);
getContentPane().add(btnCancel);
}
}
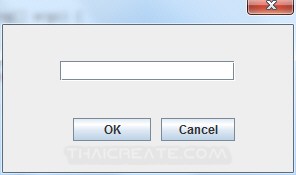
Output
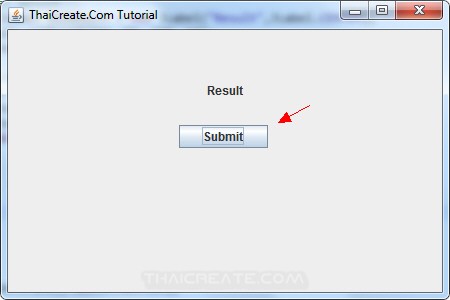
แสดง Form หลักคลิกที่ Button เพื่อเปิด Dialog Popup
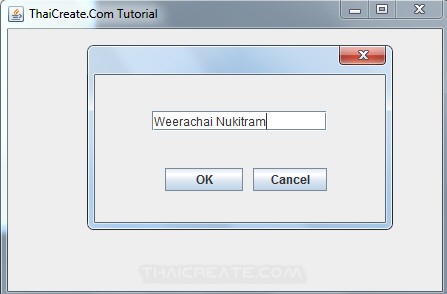
แสดง Dialog Popup และ Input ข้อมูล และเมื่อคลิกที่ OK จะมีการส่งข้อมูลไปยัง Form หลัก
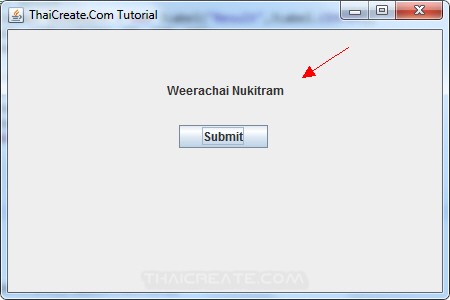
แสดงข้อมูลที่ถูกส่งมายัง Dialog Popup
|