How to use : Java GUI Download file and Progress Bar |
How to use : Java GUI Download file and Progress Bar บทความนี้จะเป็นตัวอย่างการเขียน Java GUI เพื่อ Download ไฟล์จาก Server มาจัดเก็บไว้ในเครื่อง Computer โดยในตัวอย่างนี้มีทั้งรูปแบบการเขียนแบบง่าย ๆ จนถึงการใช้เทคนิคขั้นสูง เช่นการใช้ SwingWorker กับ ProgressBar เพื่อให้โปรแกรมแสดงสถานะการทำงาน และไม่ค้างในขณะที่กำลังทำงาน รวมทั้งการใช้ ProgressBar แบบ Dialog เพื่อไม่ให้ User ทำอย่างอื่นจนกว่าจะ Download เสร็จสิ้น
How to use : Java GUI Download file and Progress Bar
Example 1 การเขียน Java GUI เพื่อ Download ไฟล์แบบง่าย ๆ
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
public class MyForm extends JFrame {
private JTextField txtURL;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Java GUI Tutorial");
setSize(525, 270);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Title
final JLabel lblTitle = new JLabel("Download File", JLabel.CENTER);
lblTitle.setBounds(73, 24, 370, 14);
getContentPane().add(lblTitle);
// TextField URL
txtURL = new JTextField("");
txtURL.setHorizontalAlignment(SwingConstants.CENTER);
txtURL.setBounds(73, 66, 370, 20);
getContentPane().add(txtURL);
// Button Download
JButton btnDownload = new JButton("Download");
btnDownload.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
URL url = new URL(txtURL.getText());
URLConnection conexion = url.openConnection();
conexion.connect();
int lenghtOfFile = conexion.getContentLength();
InputStream input = new BufferedInputStream(url.openStream());
// File Name
String source = txtURL.getText();
String fileName = source.substring(source.lastIndexOf('/') + 1, source.length());
// Copy file
String saveFile = null;
try {
saveFile = new File(".").getCanonicalPath() + "\\files\\" + fileName;
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
OutputStream output = new FileOutputStream(saveFile);
byte data[] = new byte[1024];
int count;
long total = 0;
while ((count = input.read(data)) != -1) {
total += count;
output.write(data, 0, count);
}
output.flush();
output.close();
input.close();
} catch (Exception ex) {
System.err.println(ex);
}
}
});
btnDownload.setBounds(217, 118, 100, 23);
getContentPane().add(btnDownload);
}
}
Output
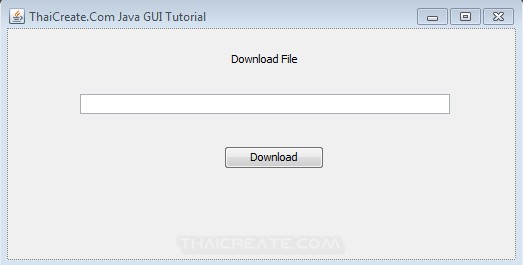
แสดง Frame สำหรับ Download ไฟล์ กรอก URL สำหรับ Download คลิก Download
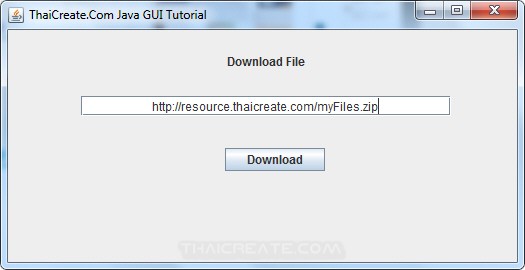
กำลัง Download ไฟล์ และจะเห็นว่าตัวอย่างนี้เมื่อโปรแกรมกำลัง Download ไฟล์ หน้าจอจะค้างจนกว่าโปรแกรมจะทำงานเสร็จ ถึงจะทำอย่างอื่นได้ต่อ (แก้ไขโดยตัวอย่างที่ 2 และ 3)
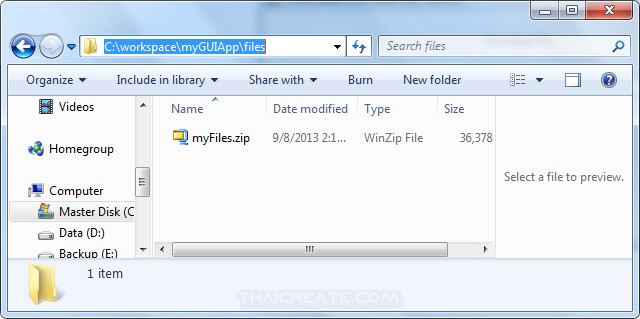
ไฟล์ถูก Save ลงในโฟเดอร์
Example 2 การเขียน Java GUI เพื่อ Download ไฟล์ ด้วยการใช้ SwingWorker และ ProgressBar
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.JProgressBar;
import javax.swing.SwingWorker;
import javax.swing.UIManager;
public class MyForm extends JFrame {
private JTextField txtURL;
private JProgressBar progressBar;
private JButton btnDownload;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Java GUI Tutorial");
setSize(525, 270);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Title
final JLabel lblTitle = new JLabel("Download File", JLabel.CENTER);
lblTitle.setBounds(73, 24, 370, 14);
getContentPane().add(lblTitle);
// TextField URL
txtURL = new JTextField("");
txtURL.setHorizontalAlignment(SwingConstants.CENTER);
txtURL.setBounds(73, 66, 370, 20);
getContentPane().add(txtURL);
// ProgressBar
progressBar = new JProgressBar();
progressBar.setStringPainted(true);
progressBar.setMinimum(0);
progressBar.setMaximum(100);
progressBar.setBounds(166, 99, 190, 20);
getContentPane().add(progressBar);
// Button Download
btnDownload = new JButton("Download");
btnDownload.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
btnDownload.setEnabled(false);
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
new BackgroundWorker().execute();
}
});
}
});
btnDownload.setBounds(210, 152, 100, 23);
getContentPane().add(btnDownload);
}
public class BackgroundWorker extends SwingWorker<Void, Void> {
public BackgroundWorker() {
addPropertyChangeListener(new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
progressBar.setValue(getProgress());
}
});
}
@Override
protected void done() {
btnDownload.setEnabled(true);
}
@Override
protected Void doInBackground() throws Exception {
try {
URL url = new URL(txtURL.getText());
URLConnection conexion = url.openConnection();
conexion.connect();
int lenghtOfFile = conexion.getContentLength();
InputStream input = new BufferedInputStream(url.openStream());
// File Name
String source = txtURL.getText();
String fileName = source.substring(source.lastIndexOf('/') + 1, source.length());
// Copy file
String saveFile = null;
try {
saveFile = new File(".").getCanonicalPath() + "\\files\\" + fileName;
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
OutputStream output = new FileOutputStream(saveFile);
byte data[] = new byte[1024];
int count;
long total = 0;
while ((count = input.read(data)) != -1) {
total += count;
setProgress((int)((total*100)/lenghtOfFile));
output.write(data, 0, count);
}
output.flush();
output.close();
input.close();
} catch (Exception ex) {
System.err.println(ex);
}
return null;
}
}
}
Output
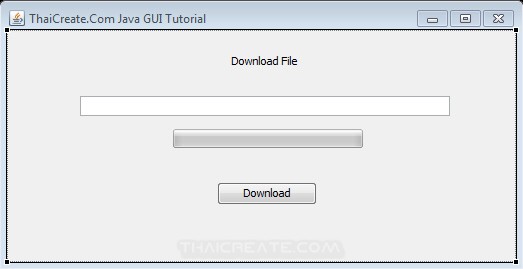
แสดง Frame สำหรับ Download ไฟล์
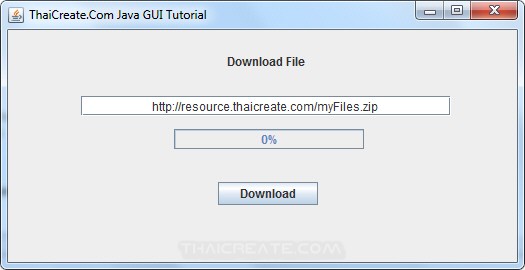
กรอกชื่อไฟล์ URL ที่ต้องการ Download
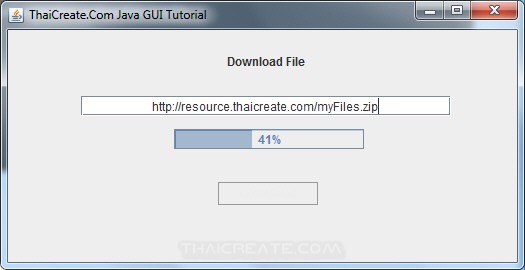
จะเห็นว่าในขณะที่โปรแกรมทำงานจะมีสถานะการ Download แจ้ง และหน้าจอโปรแกรมไม่ค้าง
Example 3 การเขียน Java GUI เพื่อ Download ไฟล์ ด้วยการใช้ SwingWorker และ ProgressBar แบบ Dialog
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.JProgressBar;
import javax.swing.SwingWorker;
import javax.swing.UIManager;
public class MyForm extends JFrame {
private JTextField txtURL;
private JButton btnDownload;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Java GUI Tutorial");
setSize(525, 270);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Title
final JLabel lblTitle = new JLabel("Download File", JLabel.CENTER);
lblTitle.setBounds(73, 24, 370, 14);
getContentPane().add(lblTitle);
// TextField URL
txtURL = new JTextField("");
txtURL.setHorizontalAlignment(SwingConstants.CENTER);
txtURL.setBounds(73, 66, 370, 20);
getContentPane().add(txtURL);
// Button Download
btnDownload = new JButton("Download");
btnDownload.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
btnDownload.setEnabled(false);
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
new BackgroundWorker().execute();
}
});
}
});
btnDownload.setBounds(210, 120, 100, 23);
getContentPane().add(btnDownload);
}
public class BackgroundWorker extends SwingWorker<Void, Void> {
private JProgressBar pb;
private JDialog dialog;
public BackgroundWorker() {
addPropertyChangeListener(new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
if ("progress".equalsIgnoreCase(evt.getPropertyName())) {
if (dialog == null) {
dialog = new JDialog();
dialog.setTitle("Downloading...");
dialog.setLayout(new GridBagLayout());
dialog.setDefaultCloseOperation(JDialog.DO_NOTHING_ON_CLOSE);
GridBagConstraints gbc = new GridBagConstraints();
gbc.insets = new Insets(2, 2, 2, 2);
gbc.weightx = 1;
gbc.gridy = 0;
dialog.add(new JLabel("Downloading..."), gbc);
pb = new JProgressBar();
pb.setStringPainted(true);
gbc.gridy = 1;
dialog.add(pb, gbc);
dialog.pack();
dialog.setLocationRelativeTo(null);
dialog.setModal(true);
JDialog.setDefaultLookAndFeelDecorated(true);
dialog.setVisible(true);
}
pb.setValue(getProgress());
}
}
});
}
@Override
protected void done() {
btnDownload.setEnabled(true);
}
@Override
protected Void doInBackground() throws Exception {
try {
URL url = new URL(txtURL.getText());
URLConnection conexion = url.openConnection();
conexion.connect();
int lenghtOfFile = conexion.getContentLength();
InputStream input = new BufferedInputStream(url.openStream());
// File Name
String source = txtURL.getText();
String fileName = source.substring(source.lastIndexOf('/') + 1, source.length());
// Copy file
String saveFile = null;
try {
saveFile = new File(".").getCanonicalPath() + "\\files\\" + fileName;
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
OutputStream output = new FileOutputStream(saveFile);
byte data[] = new byte[1024];
int count;
long total = 0;
while ((count = input.read(data)) != -1) {
total += count;
setProgress((int)((total*100)/lenghtOfFile));
output.write(data, 0, count);
}
output.flush();
output.close();
input.close();
} catch (Exception ex) {
System.err.println(ex);
}
return null;
}
}
}
Output
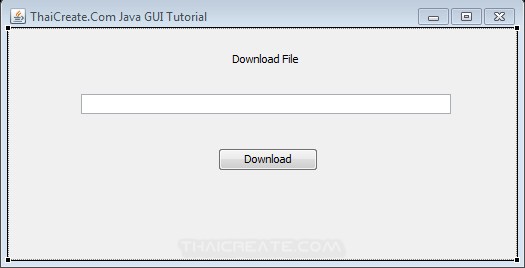
แสดง Frame สำหรับ Download ไฟล์
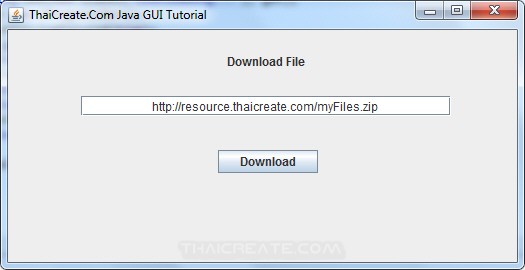
กรอกชื่อไฟล์ URL ที่ต้องการ Download
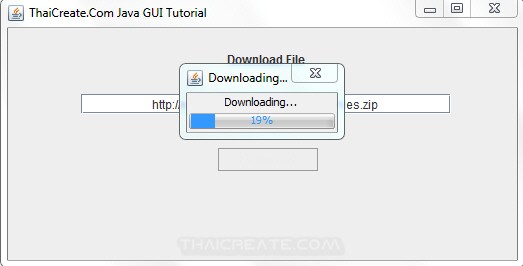
จะเห็นว่าในขณะที่โปรแกรมทำงานจะมีสถานะการ Download แบบ Dialog ProgressBar และหน้าจอโปรแกรมไม่ค้าง แต่ไม่สามารถทำอย่างอื่นได้ จนกว่าจะทำงานเสร็จ
อ่านเพิ่มเติม : Java Progress Bar (JProgressBar) - Swing Example
อ่านเพิ่มเติม : How to use : Java GUI SwingWorker and JProgressBar
อ่านเพิ่มเติม : How to use : Java GUI SwingWorker and BackgroundWorker
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2013-09-10 10:07:38 /
2017-03-27 21:59:15 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|