How to use : Java GUI Create Frame Form for Send Mail / Attachment |
How to use : Java GUI Create Frame Form for Send Mail บทความนี้จะเป็นตัวอย่างการเขียน Java GUI ในการออกแบบ Frame หรือ Form สำหรับส่งอีเมล์ไปยังปลายทาง ในตัวอย่างนี้จะเลือกใช้ Library ของ JavaMail และก่อนที่จะส่งจะต้องมี Email Account ที่สามารถ Authen ผ่าน Username และ Password ก่อนที่จะส่งไปยังปลายทางได้ โดยในตัวอย่างนี้จะยกตัวอย่าง 2 รูปแบบคือ แบบแรกจะเป็นการส่งอีเมล์จาก GUI Form แบบง่าย ๆ ส่วนตัวอย่างที่สองจะเป็นการ Browse เพื่อเลือกไฟล์สำหรับแนบไฟล์ไปกับอีเมล์ได้ด้วย (Attachment)
How to use : Java GUI Create Frame Form for Send Mail
Example 1 การเขียน Java GUI เพื่อส่งอีเมล์แบบง่าย ๆ
MyForm.java
package com.java.myapp;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.JTextArea;
import javax.swing.JScrollPane;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.util.Properties;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 496, 379);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// Mail Authen
final String auth_host = "mail.thaicreate.com";
final String auth_port = "25";
final String auth_email = "[email protected]";
final String auth_password = "password";
final Properties props = new Properties();
props.put("mail.smtp.host", auth_host);
props.put("mail.smtp.socketFactory.port", auth_port);
props.put("mail.smtp.socketFactory.class",
"javax.net.ssl.SSLSocketFactory");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.port", auth_port);
// Label Title Send Mail
JLabel lblSendMail = new JLabel("Send Mail");
lblSendMail.setFont(new Font("Tahoma", Font.BOLD, 13));
lblSendMail.setHorizontalAlignment(SwingConstants.CENTER);
lblSendMail.setBounds(187, 35, 78, 14);
getContentPane().add(lblSendMail);
// Label To
JLabel lblTo = new JLabel("To :");
lblTo.setBounds(109, 69, 46, 14);
getContentPane().add(lblTo);
// Text Field To
final JTextField txtTo = new JTextField();
txtTo.setBounds(229, 69, 154, 20);
getContentPane().add(txtTo);
txtTo.setColumns(10);
// Label Subject
JLabel lblSubject = new JLabel("Subject :");
lblSubject.setBounds(109, 110, 78, 14);
getContentPane().add(lblSubject);
// Text Field Subject
final JTextField txtSubject = new JTextField();
txtSubject.setColumns(10);
txtSubject.setBounds(229, 110, 179, 20);
getContentPane().add(txtSubject);
// Label Description
JLabel lblDesc = new JLabel("Description :");
lblDesc.setBounds(109, 151, 78, 14);
getContentPane().add(lblDesc);
// Text Area Description
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(229, 153, 181, 75);
getContentPane().add(scrollPane);
final JTextArea txtDesc = new JTextArea();
scrollPane.setViewportView(txtDesc);
// Button Send
JButton btnSend = new JButton("Send");
btnSend.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try {
Session mailSession = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication
getPasswordAuthentication() {
return new PasswordAuthentication
(auth_email,auth_password);
}
});
Message message = new MimeMessage(mailSession);
message.setFrom(new InternetAddress(auth_email)); // From
/*** Recipient ***/
message.setRecipients(Message.RecipientType.TO,
InternetAddress.parse(txtTo.getText())); // To
message.setSubject(txtSubject.getText());
message.setText(txtDesc.getText());
Transport.send(message);
JOptionPane.showMessageDialog(null,"Mail Send Successfully.");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
});
btnSend.setBounds(187, 264, 89, 23);
getContentPane().add(btnSend);
}
}
Output
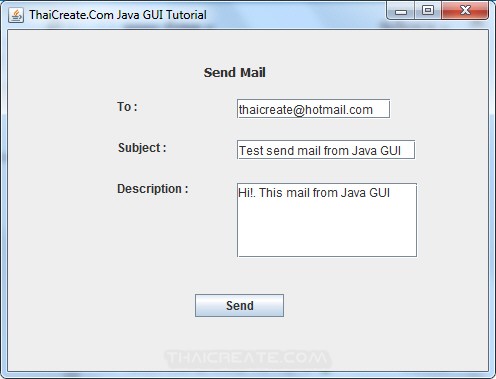
แสดง Form สำหรับส่งอีเมล์
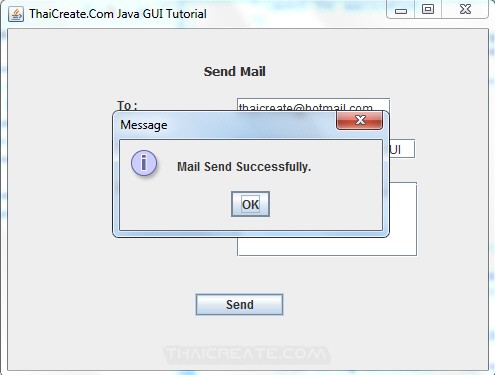
อีเมล์ถูกส่งเรียบร้อย
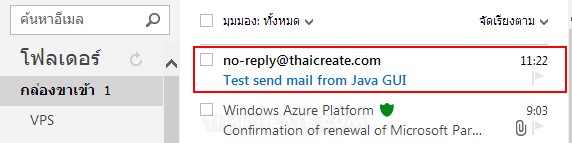
เช็คอีเมล์ปลายทาง
Example 2 การเขียน Java GUI เพื่อส่งอีเมล์แบบมีแนบไฟล์ File Attachment
MyForm.java
package com.java.myapp;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.JTextArea;
import javax.swing.JScrollPane;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.util.Properties;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 496, 435);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// Mail Authen
final String auth_host = "mail.thaicreate.com";
final String auth_port = "25";
final String auth_email = "[email protected]";
final String auth_password = "password";
final Properties props = new Properties();
props.put("mail.smtp.host", auth_host);
props.put("mail.smtp.socketFactory.port", auth_port);
props.put("mail.smtp.socketFactory.class",
"javax.net.ssl.SSLSocketFactory");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.port", auth_port);
// Label Title Send Mail
JLabel lblSendMail = new JLabel("Send Mail");
lblSendMail.setFont(new Font("Tahoma", Font.BOLD, 13));
lblSendMail.setHorizontalAlignment(SwingConstants.CENTER);
lblSendMail.setBounds(187, 35, 78, 14);
getContentPane().add(lblSendMail);
// Label To
JLabel lblTo = new JLabel("To :");
lblTo.setBounds(109, 69, 46, 14);
getContentPane().add(lblTo);
// Text Field To
final JTextField txtTo = new JTextField();
txtTo.setBounds(229, 69, 154, 20);
getContentPane().add(txtTo);
txtTo.setColumns(10);
// Label Subject
JLabel lblSubject = new JLabel("Subject :");
lblSubject.setBounds(109, 110, 78, 14);
getContentPane().add(lblSubject);
// Text Field Subject
final JTextField txtSubject = new JTextField();
txtSubject.setColumns(10);
txtSubject.setBounds(229, 110, 179, 20);
getContentPane().add(txtSubject);
// Label Description
JLabel lblDesc = new JLabel("Description :");
lblDesc.setBounds(109, 151, 78, 14);
getContentPane().add(lblDesc);
// Text Area Description
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(229, 153, 181, 75);
getContentPane().add(scrollPane);
final JTextArea txtDesc = new JTextArea();
scrollPane.setViewportView(txtDesc);
// Label Attach
JLabel lblAttach = new JLabel("Attachment :");
lblAttach.setBounds(109, 259, 78, 14);
getContentPane().add(lblAttach);
// Label File
final JLabel lblFile = new JLabel("File");
lblFile.setBounds(265, 259, 179, 14);
getContentPane().add(lblFile);
// Button JFileChooser
JButton chooseFile = new JButton("...");
chooseFile.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
JFileChooser fileopen = new JFileChooser();
int ret = fileopen.showDialog(null, "Choose file");
if (ret == JFileChooser.APPROVE_OPTION) {
lblFile.setText(fileopen.getSelectedFile().toString());
}
}
});
chooseFile.setBounds(226, 255, 27, 23);
getContentPane().add(chooseFile);
// Button Send
JButton btnSend = new JButton("Send");
btnSend.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try {
Session mailSession = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication
getPasswordAuthentication() {
return new PasswordAuthentication
(auth_email,auth_password);
}
});
Message message = new MimeMessage(mailSession);
message.setFrom(new InternetAddress(auth_email)); // From
/*** Recipient ***/
message.setRecipients(Message.RecipientType.TO,
InternetAddress.parse(txtTo.getText())); // To
message.setSubject(txtSubject.getText());
// create the message part
MimeBodyPart messageBodyPart = new MimeBodyPart();
String Attach = lblFile.getText(); // File Path
//fill message
messageBodyPart.setText(txtDesc.getText());
Multipart multipart = new MimeMultipart();
multipart.addBodyPart(messageBodyPart);
// Part two is attachment
messageBodyPart = new MimeBodyPart();
DataSource source = new FileDataSource(Attach);
messageBodyPart.setDataHandler(new DataHandler(source));
String fileName = Attach.substring(Attach.lastIndexOf('\\')+1, Attach.length());
messageBodyPart.setFileName(fileName);
multipart.addBodyPart(messageBodyPart);
message.setContent(multipart);
Transport.send(message);
JOptionPane.showMessageDialog(null,"Mail Send Successfully.");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
});
btnSend.setBounds(189, 312, 89, 23);
getContentPane().add(btnSend);
}
}

Output
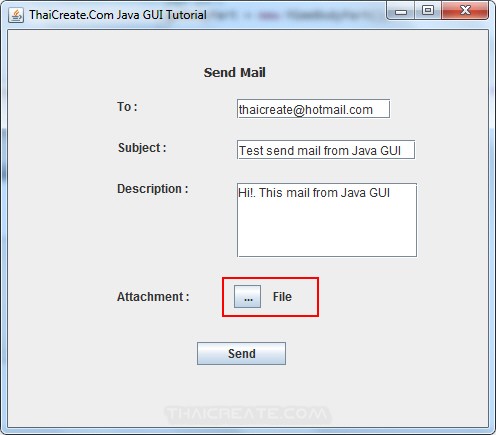
แสดง Frame หรือ Form สำหรับส่งอีเมล์ จะมีปุ่มสำหรับเลือกแนบไฟล์ (Attachment)
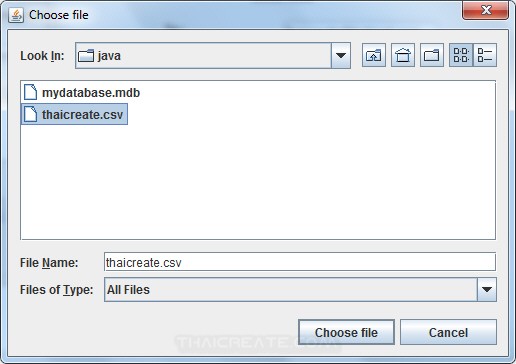
มี Dialog ของ File Chooser ให้เลือกไฟล์ที่ต้องการ
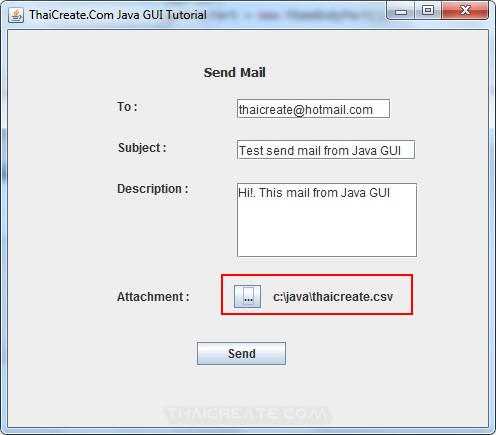
แสดง Path และไฟล์ที่เลือก
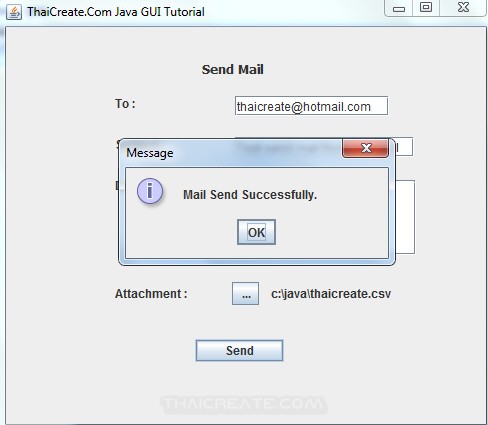
อีเมล์ถูกส่งเรียบร้อยแล้ว

เมื่อกลับไปดูที่ Email ปลายทางก็จะได้รับอีเมล์
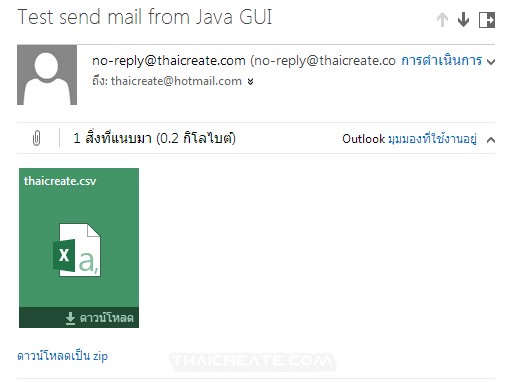
พร้อมไฟล์แนบ
อ่านเพิ่มเติม : Java Send Mail / SMTP Authen Account (JavaMail)
อ่านเพิ่มเติม : Java Send Mail / File Attachment (JavaMail)
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-09-10 10:06:12 /
2017-03-27 21:54:39 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|