How to use : Java GUI List (JList) from Database |
How to use : Java GUI List (JList) from Database บทความนี้จะเป็นตัวอย่างการเขียน Java GUI กับ List (JList) โดยจะเป็นการอ่านข้อมูลจาก Database มาแสดงใน Item ของ List แบบง่าย ๆ พร้อมกับตัวอย่างการเลือกรายการมาแสดงจากรายการที่ได้เลือกไว้
How to use : Java GUI List (JList) from Database
Syntax
DefaultListModel model = new DefaultListModel();
while((rec!=null) && (rec.next()))
{
model.addElement(rec.getString("CustomerID") + " - " + rec.getString("Name"));
}
JList list = new JList(model);
JList list = new JList(model);
list.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent evt) {
lblResult.setText(list.getSelectedValue().toString());
}
});
ในตัวอย่างนี้จะเลือกใช้ Database ของ MySQL แต่ในกรณีที่จะใช้ร่วมกับ Database อื่น ๆ ก็สามารถทำได้ง่าย ๆ เพียงแค่เปลี่ยน Connector และ Connection String เท่านั้น สามารถอ่านเพิ่มเติมได้ที่บทความนี้
Java Connect to MySQL Database (JDBC)
โครงสร้างของ MySQL และ Table
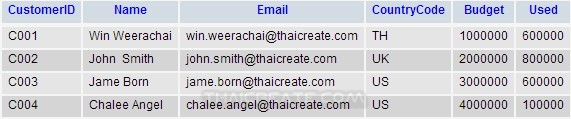
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
คำสั่งของ SQL ที่สามารถนำไปรันบน Query เพื่อสร้าง Table และ Rows ได้ทันที

Example ตัวอย่างการสร้าง List (JList) จาก Database แบบง่าย ๆ
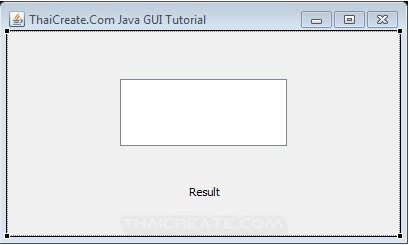
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.DefaultListModel;
import javax.swing.JList;
import javax.swing.JOptionPane;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
import javax.swing.JScrollPane;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 409, 243);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// Model
DefaultListModel model = new DefaultListModel();
Connection connect = ConnectDB();
Statement s = null;
try {
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addElement(rec.getString("CustomerID") + " - " + rec.getString("Name"));
}
rec.close();
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Label Result
final JLabel lblResult = new JLabel("Result");
lblResult.setHorizontalAlignment(SwingConstants.CENTER);
lblResult.setBounds(62, 154, 272, 14);
getContentPane().add(lblResult);
// Scroll Pane
JScrollPane scrollPane = new JScrollPane();
final JList list = new JList(model);
list.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent evt) {
lblResult.setText(list.getSelectedValue().toString());
}
});
scrollPane.setViewportView(list);
scrollPane.setBounds(113, 48, 167, 67);
getContentPane().add(scrollPane);
}
// Connection
private Connection ConnectDB() {
try {
Class.forName("com.mysql.jdbc.Driver");
return DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
}
Output
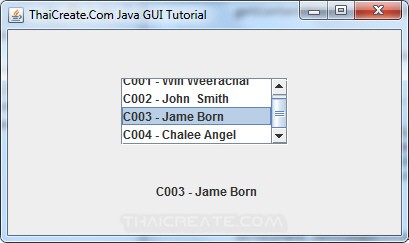
แสดง List (JList) จาก Database
อ่านเพิ่มเติม : Java List (JList) - Swing Example
กรณีที่ใช้ร่วมกับ Database อื่น ๆ สามารถดูวิธีการใช้ Connector และ Connection String ได้ที่นี่
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-09-10 10:12:40 /
2017-03-27 22:07:50 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|